Introducing the Add-on Management API
Time to read: 5 minutes
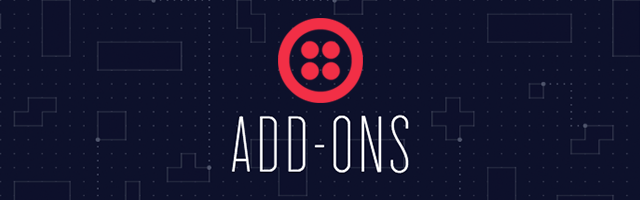
- API to programmatically manage Twilio Add-ons.
- Lets you associate a number or messaging Add-on with specific Twilio phone numbers.
- Limited access during developer preview.
Today, we are excited to announce the Twilio Add-on Management API. It is designed to help you install & configure Twilio Add-ons from your application – no web interface necessary!
Now using the API, you can install, configure, and enable Add-ons programmatically. It also allows you to associate a number or messaging Add-on with specific Twilio phone numbers so they’re invoked only for incoming calls or messages to that number. You can request early access now to Add-on Management API developer preview.
What are Twilio Add-ons?
Add-ons are products from our partners that are pre-integrated into the Twilio APIs. They help you quickly add new capabilities such as advanced caller identification, spam detection, compliance, multi-lingual speech to text, audio search, and more to Twilio. They’re super easy to use and are designed to reduce the time of getting a new capability into production. Here’s a quick recap of what Add-ons can do.
Add-ons available in the Marketplace today fall into one of 3 buckets:
- Number Add-ons that provide you with more data for a given phone number
- Messaging Add-ons that help you analyze the contents of a text message
- Recording Add-ons that help you analyze recordings you make with Twilio.
In case you haven’t checked them out before, here are some helpful links: Twilio Add-ons catalog, Number Add-ons guide for Programmable Voice, Recording Add-ons guide.
Getting rid of manual configuration & email interactions
One pattern we’ve seen emerge with Add-ons is that our customers offer them as features in their own products to their customers. For example, digital marketing agencies use Twilio to route leads to their clients. When a lead calls one of the agency’s Twilio numbers, the call gets routed to the right client. The agency uses enhanced Caller ID to offer their clients more information about the caller such as their name, industry, and even household income. Their customers love this functionality because knowing more about who’s calling lets them close more leads.
This works great, but the problem is agencies can’t enable their clients fast enough. When they release a new feature like this they’ll have 100’s or 1000’s of clients who all want access. They’ll provide a button in their software to “add enhanced caller id.” But when the user clicks it they get a message that says, “Thanks, we got your request and we’ll be in touch soon.” This is because the agency then has to go into the Twilio Console and manually enable an Add-on for all those customers. Additionally, some clients want to only enable add-ons for some of their phone numbers, but not all of them. So far the relationship between Add-ons and phone numbers has been “all or nothing.” It’s a poor experience for both the agency and their clients. Well, today that all changes.
We’re excited to ship the Add-on Management API. It lets you enable and configure Add-ons for your primary or sub-accounts programmatically. Additionally, it supports per-number configuration for Number & Messaging Add-ons. This means you can build Add-ons into your software, and now your users can install with a click!
Note that if you only need to install the Add-on into a small number of accounts, it may still be easier to do it via the Console.
Here’s how it works…
Installing an Add-on
Let’s start by finding an Add-on we want to use from the Console, and install an instance of it via the API. To do this, we need the Available Add-on SID for that Add-on. Note that you can also query for a list of available Add-ons via the API to get this SID.
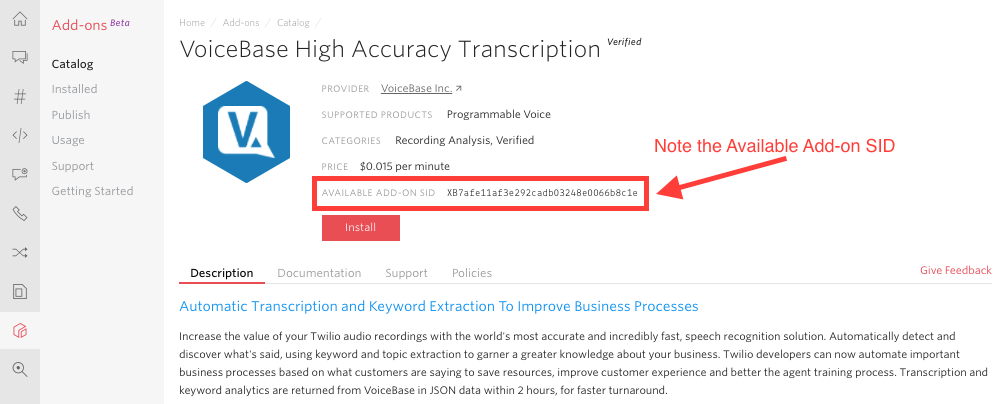
Once you have the SID of the Add-On you want it’s easy to install an instance of that Add-on. Let’s install the VoiceBase High Accuracy Transcription Add-On using its Available Add-On SID, shown in the image above.
Note – These examples use the alpha version of Twilio’s next-gen Python helper libraries, so install those if you’d like to play along.
Note that installing it does not automatically configure or enable it. We’ll have to do both separately. You will typically want to save the Installed Add-on SID so that you can configure, enable or disable it in the future – it’s a unique identifier for each instance of Add-on that is installed.
Configuring an Add-on
With an instance of the Add-On installed, it’s ready to be configured. We can see a list of its configuration parameters from its Console page or by querying for them using the API. You can also pull up the configuration for an installed Add-on using this API.
Note that configuration parameters vary from Add-on to Add-on, and that not all Add-ons need a configuration. If the Configuration schema for an Add-on is empty, you can simply skip this step.
Enabling an Installed Add-on
Now, we’re going to enable this Add-on for the “Record Verb” extension, which means it gets invoked every time you create a recording using the TwiML verb.
Associating an Add-on with a Twilio Number
At this point the Add-On will execute every time any number in your account creates a recording. In case you are using a Number or Messaging Add-on, you can limit it to execute only when specific phone numbers receive calls or messages by associating it with one or more Twilio phone numbers. (This is currently not supported for Recording Add-ons).
For our next example, we’ll switch to a Number Add-on – Nomorobo Spam Score Add-on that tells us if the caller or texter is likely to be a robocall.
Wrap up
To recap, the Add-on Management API is designed to help you install & configure Twilio Add-ons programmatically so that you can build features in your application that can be enabled without manual intervention, creating a better customer experience for your users.
For more information and to get started with the Add-on Management API, check out the docs. Note that this API is in developer preview currently, which means:
- In order to use it, you will need to request access here.
- This API is only supported by alpha version of next-gen helper libraries
- If you associate a number or messaging Add-on with a specific phone number, the association will not be visible in the Twilio Console.
We can’t wait to see what you build with Add-ons!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.