Send an SMS Message with Kotlin in 30 Seconds
Time to read: 2 minutes
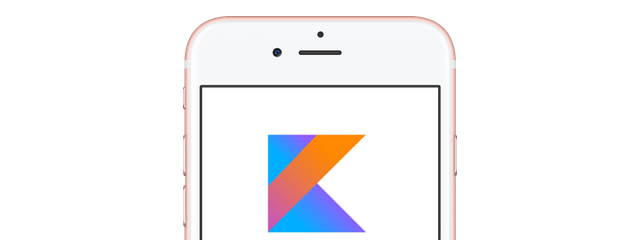
You’re building a Kotlin app, and you need to send SMS messages. What if I said you can get it done in 30 seconds with the Twilio API? Here’s a video showing you how quick it is to send an SMS message with Kotlin and the Twilio API.
Video: How To Send SMS with Kotlin in 30 Seconds
But you can’t copy and paste from a video, so here’s all the code you will need. Or you can just get it from this repository.
Add the Twilio helper library for Java to your Gradle or Maven file.
Initialise the Twilio REST Client passing your Account SID and Auth Token, which are available on the Twilio Console.
You will need three things now:
- The phone number you are sending the message to
- The Twilio number you are sending the message from
- The body of the message
Add those into the Message.creator
method and it will send a text message:
Now run it by pressing the green arrow next to your function definition.

Just wait for the magic to happen.
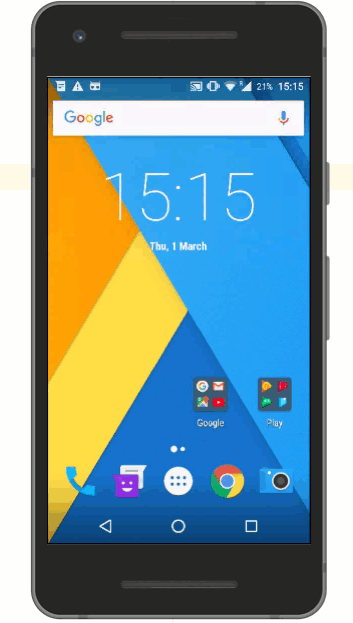
We can’t wait to see what you build
You’ve sent an SMS message, and now you’re ready to take on the world of communications. Take a look at the documentation for the Twilio REST API and for working with the Java helper library to see what else you can do. Then check out our tutorials to see some more examples, like sending SMS notifications, masking phone numbers for user privacy or two-factor authentication for user security.
Excited about the possibilities? Then let me know! Hit me up on Twitter @marcos_placona or just give me a shout in the comments below.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.