Simple Twilio Scripts Using Webscript.io
Time to read: 7 minutes
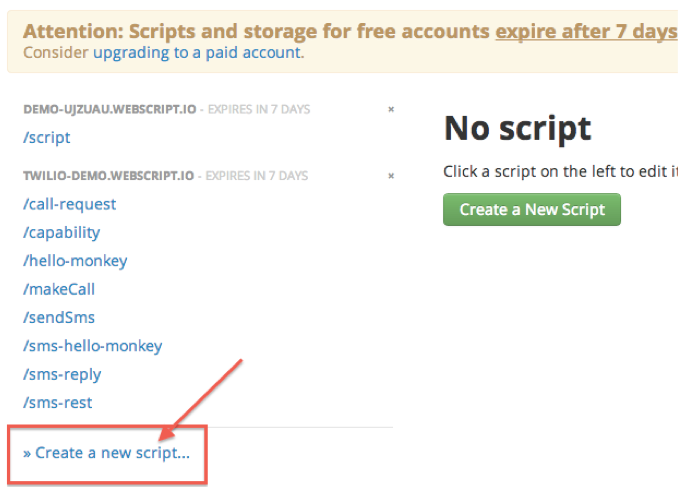
OK. I admit it, when it comes to writing apps, I’m pretty lazy. And while I love writing Twilio apps writing the Twilio code always turns out to be the easiest part. The part I’m not such a fan of is all the other stuff I have to do in order to get my app up and running. Especially when most of the time the bulk of what my app needs to do is make a couple of HTTP requests or spit out some TwiML. Even for the most basic app, I have to choose a language I want to use, setup a development environment for that language, and figure out where and how to deploy my app. Blah. That goes very much against my lazy developer ethos. And that sucks.
Thats why when John Sheehan pointed me at a service called webscript.io, which offers a service that makes it super easy to stand up new URLs and write small scripts that will run when I request the URLs, I immediately wanted to try it out. The scripts are written using a language called Lua, a simple but powerful lightweight scripting language which makes standing up simple Twilio apps, or even prototyping larger apps super simple.
In this post I’ll show you how you can use webscript.io and Lua to interact with the Twilio REST API and generate TwiML responses.
Getting started with webscript.io
Getting started with webscript.io is really simple, just sign up for a free trial account. The only restriction for trial accounts is that scripts expire after 7 days, which should be plenty of time to try out some fun stuff.
Once you’ve signed up and logged in, create your first script by clicking Create a new script:

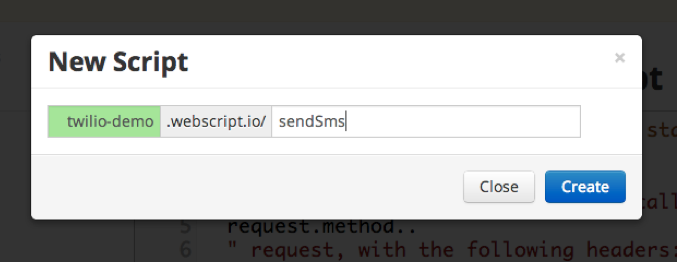
The subdomain must be unique across all of webscript.io but once you’ve used a specific subdomain in your account, you can reuse that for as many scripts as you like.
Once you enter the subdomain and path, click OK to close the dialog and webscript.io will present you with an editor that you can start writing your Lua script in. To learn more about Lua and some of the clever things you can do with it, check out the webscript.io documentation and examples.
Receiving Incoming Text Messages
So now that you’re signed up, you can start using webscript.io to set up some Twilio apps. For example, it is trivial to use the service to respond to an incoming SMS message by sending a reply. To do this you create a script that returns some TwiML that includes the verb. This tells Twilio to send an SMS message back to the the person who sent the message:
The script starts by setting the Content-Type header to application/xml, which tells Twilio the response is going to be XML and then returns the string of TwiML containing the verb.
Save the script and then try loading it in a browser and you will see that it returns the TwiML as you’d expect. Try assigning the webscript.io URL to a Twilio phone number and sending a text message to it. You should get a reply with the message “Hello, Mobile Monkey”.
Replying to Text Messages
While its easy to send what is essentially static TwiML back to Twilio, that’s not really taking advantage what webscript.io brings to the table. The power of webscript.io really starts to show when you start using Lua and the input parameters passed into the script to create logic that dynamically generates the TwiML returned to Twilio.
For example, if you wanted to personalize the SMS reply sent from Twilio you could create a simple table in Lua and use the From parameter passed in by Twilio, which is the phone number that sent the message, to locate the senders name to inject into the response.
To do that, first create the table containing the phone numbers and names of the possible callers:
Lua includes a type called Table that lets you create an associative array. In the sample code the key is the phone number of a potential sender and the value is their name.
Then get the value of the From parameter from the request and see if you can find a match in the table. The found match (or a default value if not match is found) will be placed into a local variable called name:
The code above is checking both the form data parameters and the query string parameters for a key named From. This lets the script handle both GET and POST requests from Twilio.
Now create the TwiML response, injecting the name into the string:
Here the script uses the Format function to place the name into the TwiML being returned.
As with the previous script, you can load this URL into a browser to test it. Simply add the From parameter and a phone number value from the Table to the URL as a querystring value:
Changing the From value should change the TwiML returned.
Handle Incoming Calls
In addition to handling incoming SMS messages, it’s also possible to handle incoming phone calls. As was done to handle an incoming SMS message, you will need to generate and return some TwiML to tell Twilio how to process with the phone call once it is answered. In the script below, the TwiML returned tells Twilio to convert the text inside of the verb into speech that the caller hears.
This script also demonstrates one other important concept: verifying that the incoming request is only coming from Twilio. You don’t necessarily want just anyone who figured out the URL to your script to be able to execute it, so this script first validates the the incoming request is in fact coming from Twilio. It does this by first calling the require function to including a library provided by webscript.io named twilio, and then calling the verify function, passing the incoming request and your accounts AuthToken, which you can get from your Twilio dashboard.
The verify method uses validation token that is included in every request Twilio makes to the script to make sure the request actually came from Twilio. If the verification fails, the function returns false, and in this case the script returns a HTTP 403 status, indicating the request is forbidden.
Sending SMS Messages
Its also possible to send text messages from webscript.io by sending requests to the Twilio REST API. The process of sending text messages is greatly simplified by using the twilio library proved by webscript.io, which you can see is included in this script:
Once the twilio library is included you can call the libraries sms function, passing in your Twilio Account SID, AuthToken, From and To phone numbers and the message you want to send.
Making Outgoing Calls
Like sending text messages, you can also make outgoing phone calls from webscript.io by using the twilio helper library. The call function is used to initiate the outgoing phone call:
Also notice that instead of a message, the call function requires a URL parameter. This URL will be requested when the outgoing phone call is answered, and allows you to provide the TwiML instructions that Twilio needs to handle the phone call.
Getting SMS Messages
The Twilio library provided by webscript.io makes it really easy to send SMS messages, but if you want to get other information from the Twilio REST API, you’ll need to use the lower level http request API’s. For example, lets say you wanted to get a list of the SMS messages you had sent to a specific phone number. To do that you need to make a request to the correct Twilio REST API url, passing in the To parameter, and providing your account credentials:
Twilio will return a response formatted as JSON upon which the script can then parse and act.
Generating Capability Tokens
Lastly, let’s look at how you can use webscript.io to create capability tokens for use with Twilio Client. Creating capability tokens using webscript.io is especially power when building mobile apps because it means not having to set up an entire backend system for the app.
Creating capability tokens in webscript.io is made simple by using the capability token generator library. To use it simply include it in your script:
Once the capability token library is added you can initialize it with your Twilio account credentials and indicate if the Twilio Client should allow incoming and/or outgoing phone calls.
Call the generateToken method to generate the token and return it as the result from the script and and use it to power Twilio Client in your mobile application.
Wrap Up
Twilio lets you build really powerful phone apps, but even before you get to the Twilio part of your app, you have to climb over a mountain of other choices and problems. Maybe you just need to whip up a simple SMS alerts app or you’re trying to quickly prototype a simple IVR. In those cases creating and deploying an app that requires setting up an entire backend platform can be overkill. Instead services like webscript.io make it easy to create URL addressable code that you can use to power your Twilio app, freeing you from the burden of managing an entire software stack.
Whether making outgoing phone calls, returning TwiML to Twilio or generating a capability token for Twilio Client in my iOS app, webscript.io is one of my favorite ways to creating simple Twilio apps that I can get up and running fast.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.