Using Natural Language Processing for Better SMS Interfaces Using Twilio and Python’s TextBlob
Time to read:
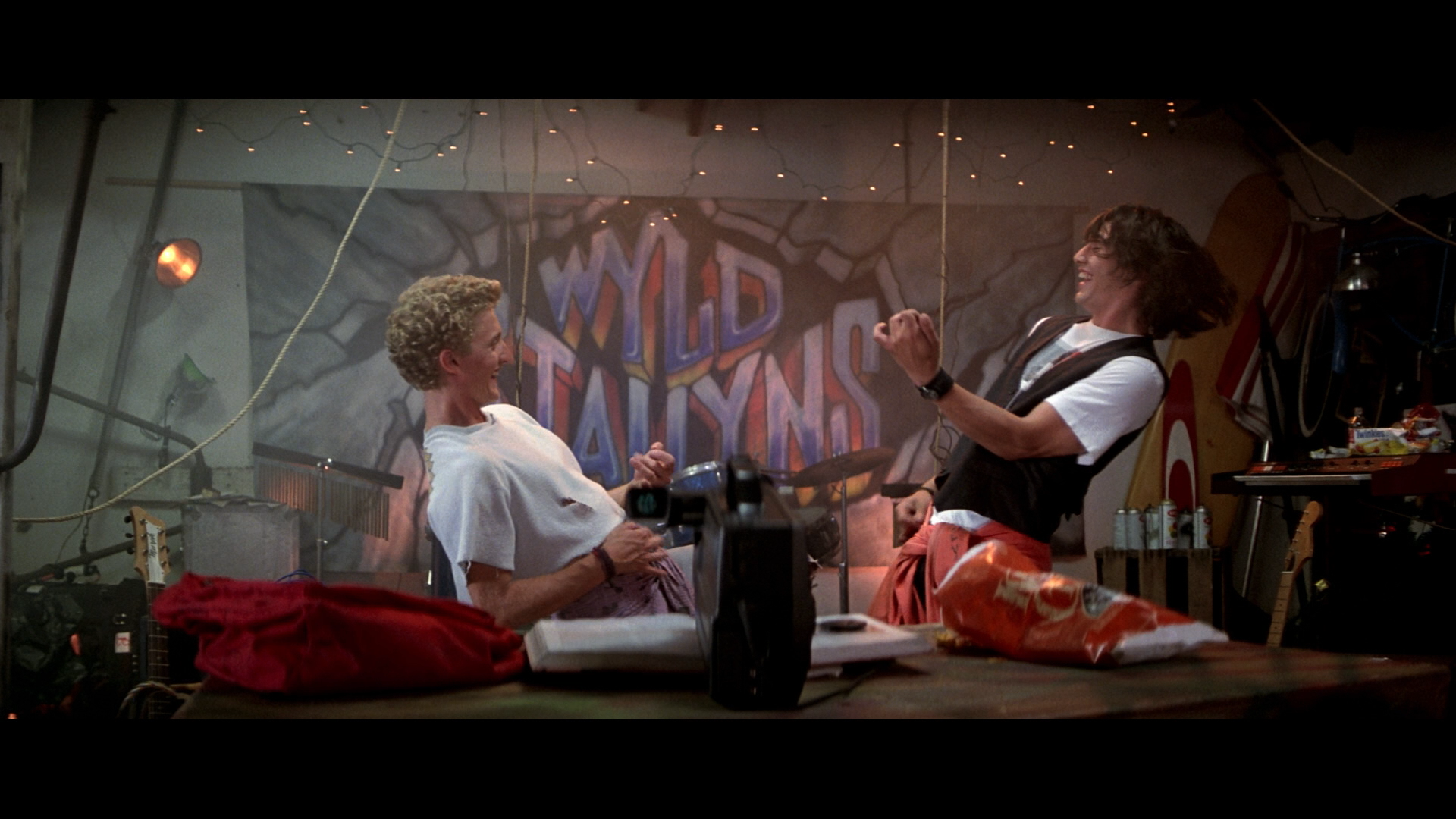
The International Telecommunications Union, the telecom agency for the United Nations, recently released some data suggesting for every 100 people on Planet Earth, 96 of them have a subscription to a cellular service. That means there are nearly as many cell subscriptions out there as human beings – a stonking 6.8 billion.
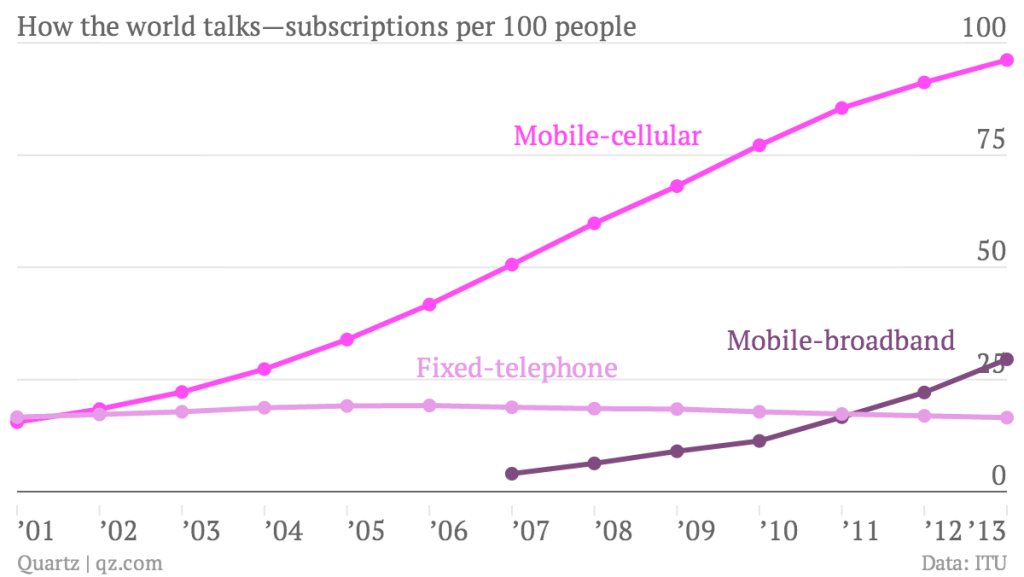
Holy biscuits – that means there might well be more cell phones out there than people.
With Twilio, you can build apps with that touch every last one of them. Voice and SMS are the only apps that come preinstalled on each of the devices those 6.8 billion people use. However, with that many types of phones and languages out there to interface with, building interfaces that work for every human can be a fascinating engineering challenge.
Take SMS for example. We’ve all come accustomed to text interfaces that ask for a KEYWORD in all caps and then take some action or deliver some information. But with the wealth of tools at our fingertips as software people, surely we can do better than applications that hinge on single words or phrases in all caps like “Text HELP to do something useful.”
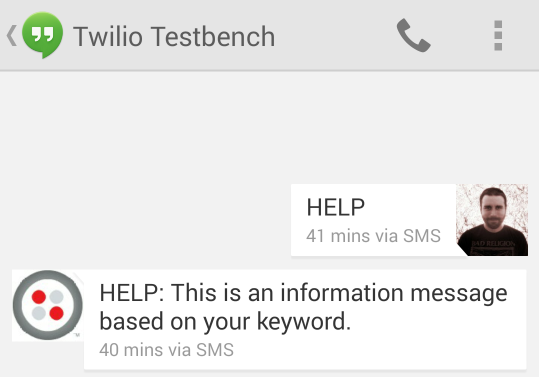
One of those tools is natural language processing – the field of computer science, artificial intelligence and linguistics dedicated to improving the way computers interpret human input. NLP is an endlessly riveting and complex field that mixes very deep comprehension and dissection of the way humans speak in different languages with stonkingly labyrinthine statistical models to makes computers interact with us the way people do. That rabbit hole goes deep and can definitely be intimidating for the mere web hacker, but fortunately the ecosystem around the field is producing some helpful open source projects that take the same discipline that powers Siri, Google Now, Cortana and Watson, and makes it accessible to the lay programmer.
One such project in the Python community is called TextBlob. It’s a library I found through the excellent Pycoder’s Weekly aiming to provide a dead simple interface to many common natural language processing tasks. In this post, we’ll use this tool to improve a few common SMS interfaces to create a more useful, more human experience for our Twilio SMS apps.
What You’ll Need
Here’s the ingredients list to cook up a tasty NLP souffle for our Twilio SMS app.
- Twilio SMS – sign up for a free trial account here.
- Python – the programming language our tutorial will be in.
- Flask – a Python web microframework that will make it easy to accept incoming SMS messages. Used here only for illustration, you’re welcome to use any web framework that works for you.
- TextBlob – our natural language processing library we’ll use to improve our SMS interfaces.
Setting Up The Project
First we need to sign up for our free Twilio account. Once your signup is complete and at your Twilio Account Dashboard, let’s click on the Numbers tab, select theTwilio phone number we would like to use for the project and configure it to point to the Python Twilio app we’ll be creating.
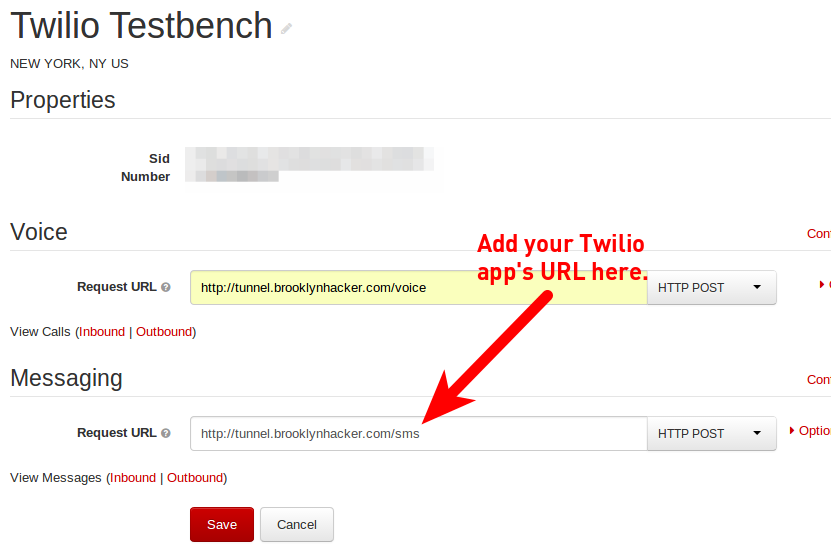
Next, we need to set up our project environment to get hacking. First, we’ll install the Twilio Python module from the command line using Pip.
Next we’ll install the two other Python packages we’ll use for this project. The first is Flask, a web microframework that makes building Twilio apps very quick. The second is TextBlob, the library we’ll use for our natural language processing.
Many NLP classifiers do depend on a collection of pre-classified data called a corpus. TextBlob makes it easy to download the collections it relies on for natural language processing with a handy function:
With our requirements installed, we’ll set up a quick Hello World app that we can modify to build our humanized SMS interfaces. Here’s a quick, non-production example:
We’ll then run the Flask app to make it ready to receive an SMS message:
Let’s send a text message to the Twilio phone number we configured up in the first step and see if it worked.
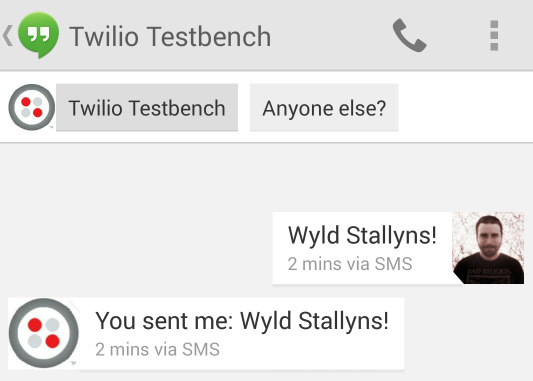
Excellent! Now we’re ready to implement some natural language processing to improve the interfaces to our Twilio SMS apps.
Keeping Customers Happy with Analyzing Sentiment
A common application for NLP is sentiment analysis: determining programmatically whether user input is positive or negative. Let’s create a quick stub app that simulates a service that provides customers support via texting.
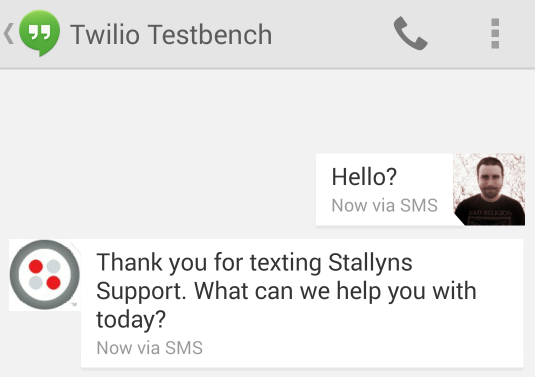
To make the experience more personal, we could add some sentiment analysis to the application. Based on whether or not the customer is happy or not, we can have our app respond accordingly.
Let’s add a little conditional logic to our app to supply different SMS replies based on the positivity or negativity of the user’s text message.
Let’s give the app a try. We’ll try emulating an unhappy customer.
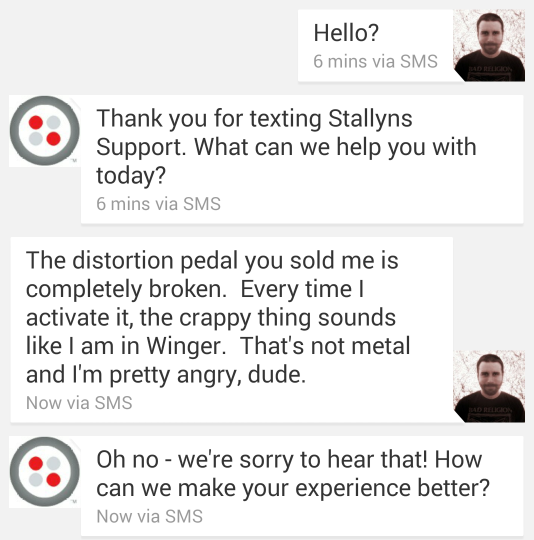
Then try emulating a happy customer.
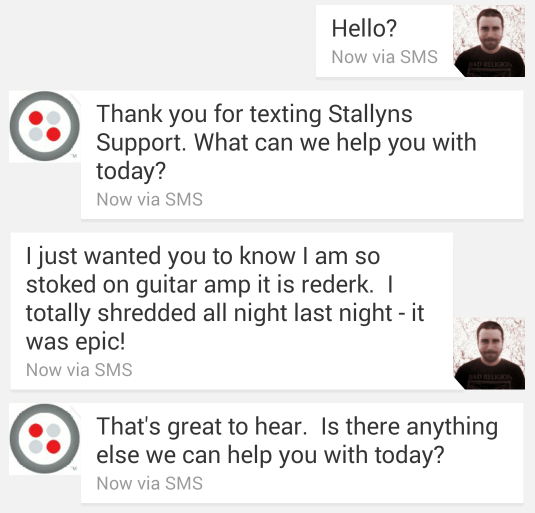
Then try a behavior that is a little more “meh.”
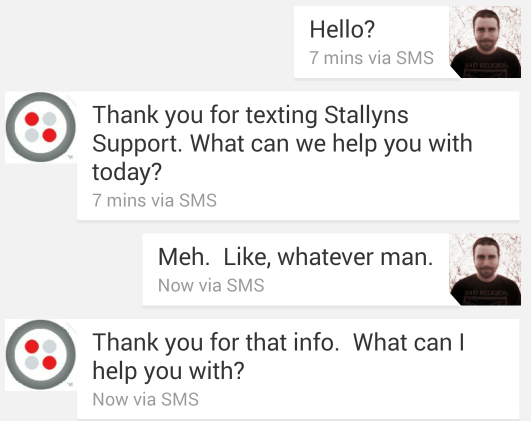
Party on – we can take wildly disparate input that we could not possibly anticipate and with just a few lines of code customize our responses for a more human experience. These kind of determinations can have wide implications beyond just customer support. Recording sentiment can improve SMS apps that collect survey data, handle logistics alerts like deliveries and even facilitate classroom instruction.
Forgiving Fat Fingers with Spelling Correction
One of the biggest issues with keyword SMS interfaces is the tendency for us humans to making spelling errors on the tiny keypads and touchscreens that power our texting. While parsing for a keyword in all capital letters like HELP is really easy for programmers, it’s not so easy for users.
Many keypads and keyboards have tiresome caps lock mechanisms and even four characters can be easy to miss when you’re typing with a single thumb. Let’s see if we can make a HELP menu for a Twilio SMS app more human by adding some NLP.
First, let’s build a HELP menu by looking for the HELP keyword and responding with a menu.
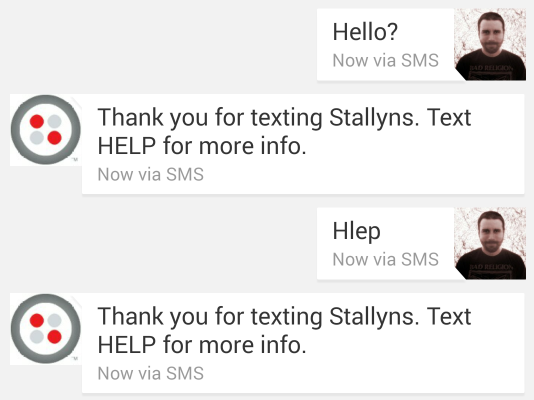
Let’s see if we can make this Twilio SMS app a little more forgiving of our human foibles with some NLP.
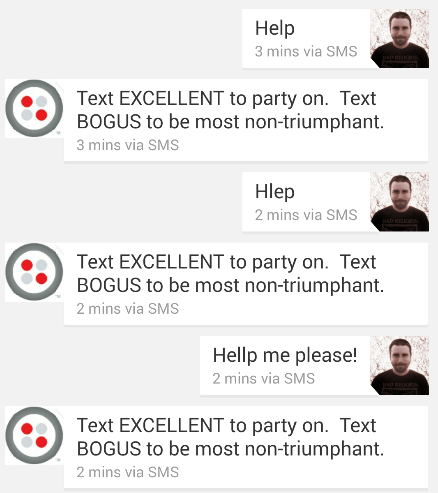
That’s a user experience that could cause even the most course button masher to break into air guitar spontaneously.
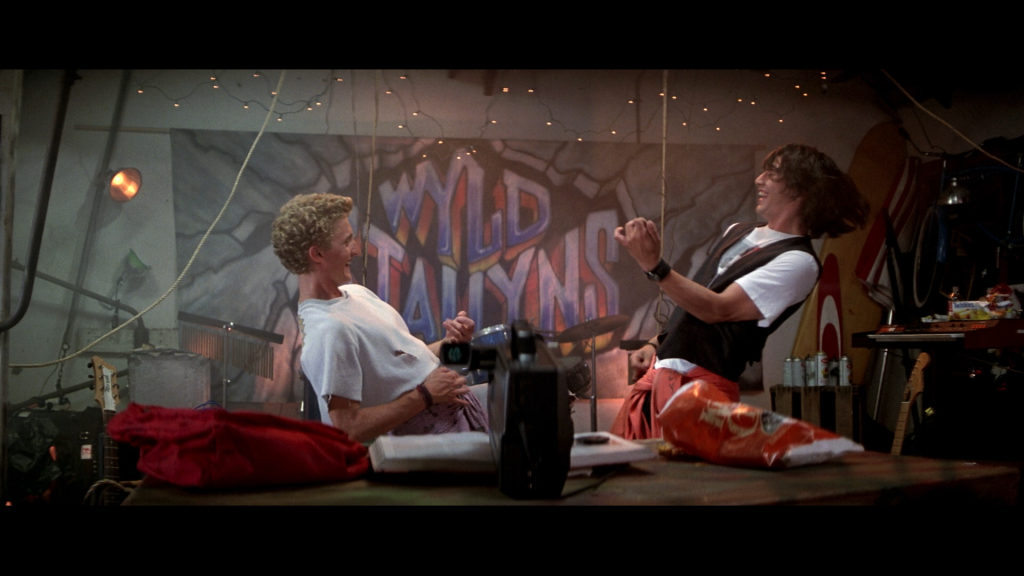
Picking Up What Your Customer Is Putting Down With Language Detection
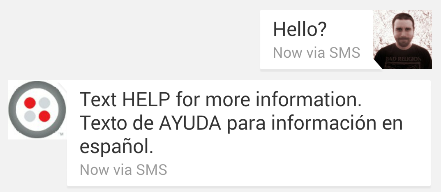
In SMS applications serving users of multiple languages, informational menus are often presented to users with language specific keywords.
For example, if we were serving customers through our Twilio SMS app in both English and Spanish, we might serve the HELP keyword for an English menu and the AYUDA keyword for a Spanish menu.
A stub for an app like this would like:
But that’s not a great experience for the user, right? In order to serve all the users, we have to make horrible UX decisions that bias user behavior, like what order we position the languages and what preference is given in the event of cognate collision.
As developers, we have all the information we need about the user’s language we need right in the text he/she is sending us. Using NLP to detect the language, we can create the best personalization of all with our application by conforming our application dynamically to the language of the user.
Let’s take a stab at what a language detecting help menu would like like using TextBlob’s built-in methods for the Google Translate API.
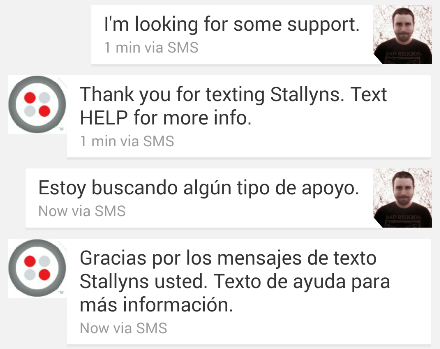
Most triumphant!
Playing More With NLP
Thanks for checking out how to use natural language processing to improve the SMS Interfaces of your Twilio apps. We prototyped some examples of how NLP can be used to create more human experiences for your users. We used sentiment analysis to customize our automated responses based on the user’s input. We leveraged spelling correction to forgive the occasional mistakes that happen with SMS keywords. And finally we used language to detection to ensure our app is literally speaking the same language as our customer.
Natural language processing is a way fun field of study and there are plenty more practical ways you can leverage it in your Twilio apps. Here are some other projects I found helpful when exploring NLP:
- Natural Language Toolkit – the ginormous gold standard of NLP open source libraries.
- Intro to NLP on Coursera – The free online introduction to the field from Stanford. Definitely brush up on your stats beforehand.
- LingPipe – A freemium NLP library for Java.
- Apache OpenNLP
I would love to see what you build with NLP and Twilio – you can find me on Twitter @dn0t or via email at rob@twilio.com. Be excellent to each other!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.