How to Verify Phone numbers in Python with the Twilio Lookup API
Time to read: 3 minutes
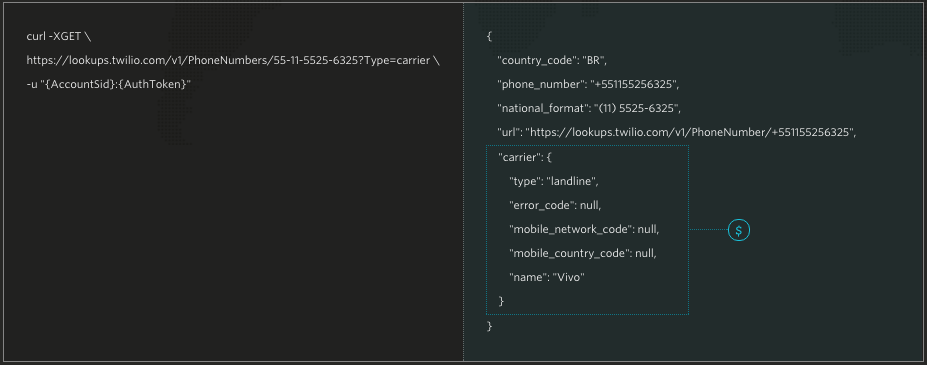
Twilio Lookup is a simple REST API with a ton of utility. You can use Lookup to check whether a number exists, format international numbers to local standards, determine whether a phone is a landline or can receive text messages, and even discover information about the carrier associated with that phone number.
In this post, we’re going to learn how to deal with valid and invalid numbers using the Twilio Python library. This code could work in any context whether you use it to look up customer numbers in your production Django app or just have a basic script you want to run to check numbers in a local database. This should also work regardless of whether you are using Python 2 or 3.
Before getting started you will need to have Python installed as well as pip, which should come with most versions of Python. You will also need to sign up for free Twilio account.
Getting started
First off you should run a virtualenv in order to cleanly install python libraries with pip. If you are unfamiliar with pip and virtualenv you can check out this guide.
Head over to your terminal and install the Twilio Python module:
You will also need to have your Account SID and Auth Token handy, which you can grab from your Twilio account dashboard.
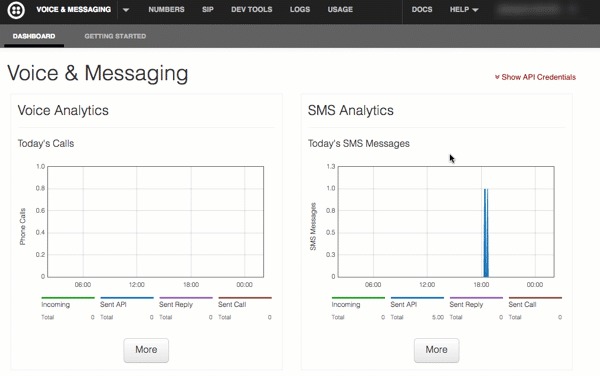
Now take those values and set them as environment variables:
With these set as environment variables, the Twilio Python module will be able to access them so you don’t have the problem of accidentally committing your account credentials to version control. You can also check out this guide on managing your environment variables.
Looking up valid phone numbers
You can check out the Lookup page if you want to play around with the API and see what type of data a request will return. Try entering a phone number and take a look at the JSON response object.
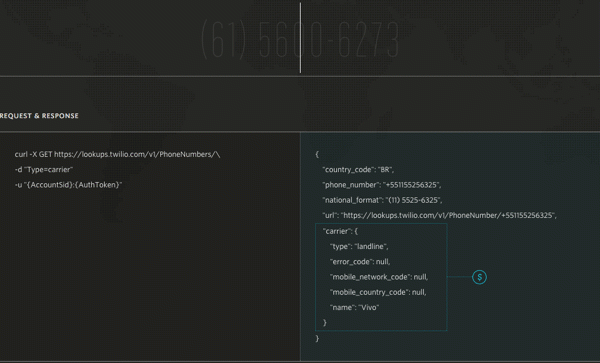
Here’s a quick code sample for that type of lookup without getting the carrier information:
This basic functionality is free, but you can get more information by doing a carrier lookup, which will not cost much. Carrier lookups are commonly used to determine if a number is capable of receiving SMS/MMS messages.
Carrier lookups contain much more useful information, but require an extra parameter:
Looking up invalid phone numbers
You can think about Twilio Lookup as a phonebook REST API. In this “online phonebook” the phone numbers serve as a unique ID. When you try to look up a phone number that does not exist you will get a 404 response.
Head over to the Lookup homepage again to see this in action when you check a number that doesn’t exist:
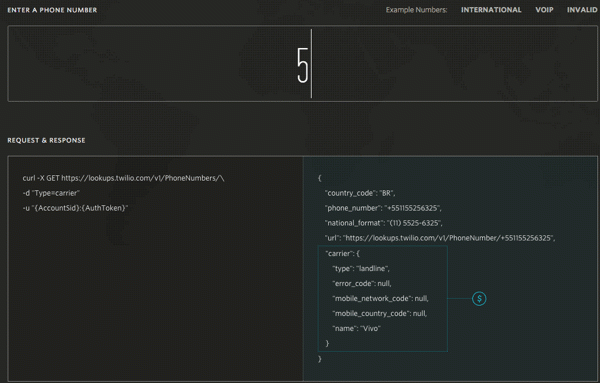
When the Twilio Python library encounters a 404 it will throw a “TwilioRestException”. If you change our current code to look up an incorrect phone number you will see this in action:
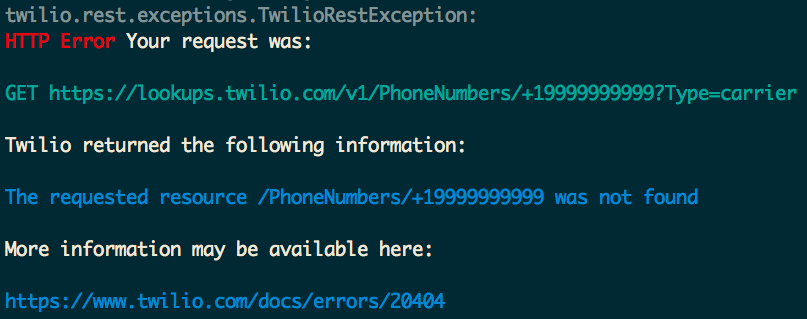
The error code for this is 20404, so if we want to write a function that validates phone numbers we can check to see if an exception was raised with code 20404.
Create a file called “lookup.py” and try this code out:
You now have a function you could use anywhere in your code for validating phone numbers.
Looking ahead
So now you know how to use the REST API phone book that is Twilio Lookup. You’ve used it for validating phone numbers, but you can also do cool things like phone number formatting and checking to see whether a number can receive text messages or not.
You might want to use one of these more common cases, but I’m always excited to see what other crazy ideas people think of with new APIs. I cannot wait to see what you build with Twilio Lookup.
If you have any questions or want to show off what you built, feel free to reach out:
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.