Texting robots on Mars using Python, Flask, NASA APIs and Twilio MMS
Time to read: 3 minutes
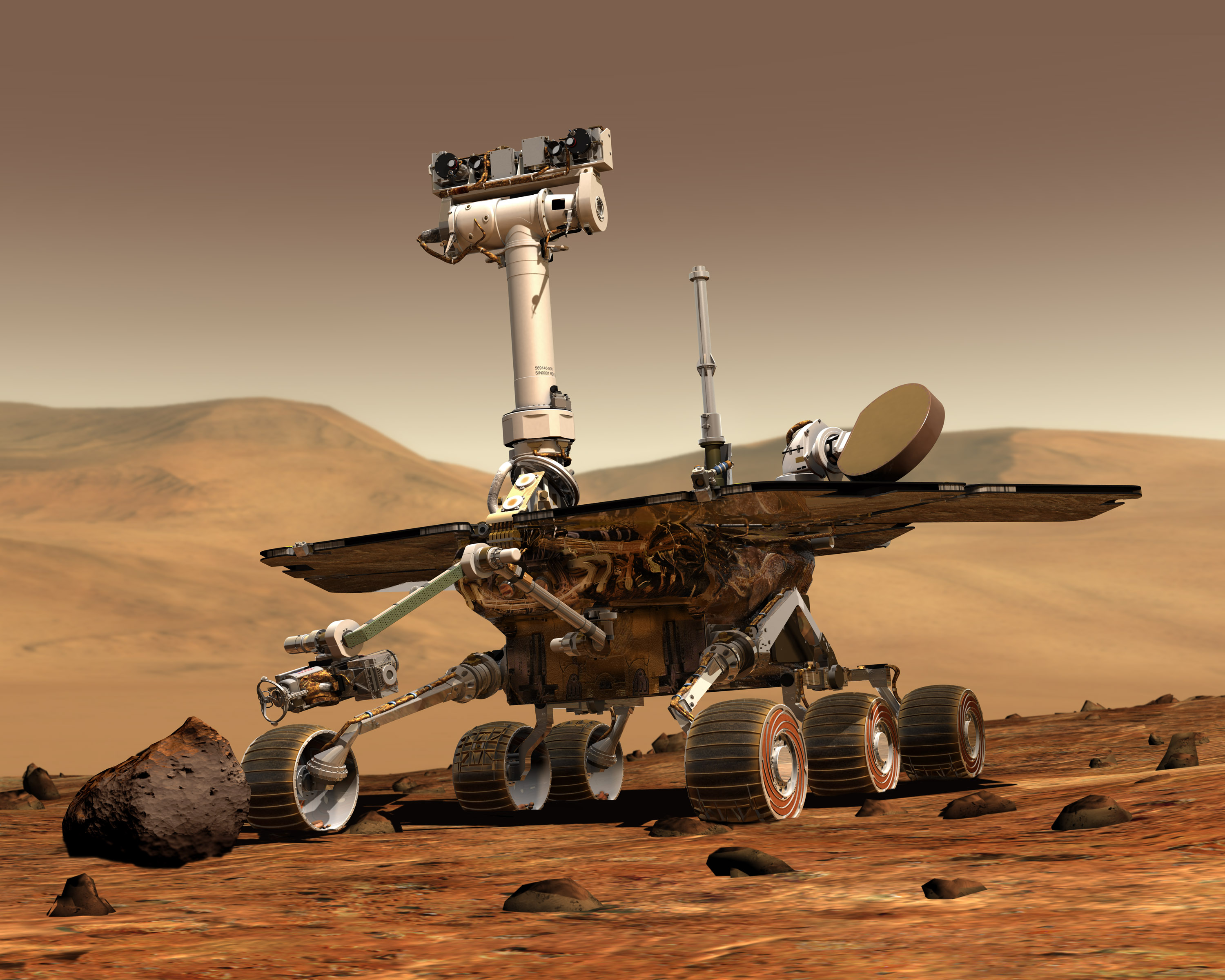
NASA has a bunch of awesome APIs which give you programmatic access to the wonders of space. I think the Mars Rover Photos API in particular is really amazing as you can use it to see what kind of pictures the Mars Curiosity rover has been taking.
Let’s build an app using the Mars Rover API with Twilio MMS, Python and Flask to make it so that we can text a phone number and receive pictures from Mars.
Setting up your environment
Before moving on, make sure to have your environment setup. Getting everything working correctly, especially with respect to virtual environments is important for isolating your dependencies if you have multiple projects running on the same machine.
You can also run through this guide to make sure you’re good to go before moving on.
Installing dependencies
Now that your environment is set up, you’re going to need to install the libraries needed for this app. We’re going to use:
- Flask for our web framework
- Requests to grab data from the Mars Rover API
- Twilio’s Python library to interact with the Twilio API.
Navigate to the directory where you want this code to live and run the following command in your terminal with your virtual environment activated to install these dependencies:
Requesting data from other APIs
Let’s start by writing a module to interact with the Mars Rover API, which is a URL that returns some JSON.
Create a file called mars.py
and enter the following code:
What we’re doing here is sending a request using the requests
module to the URL corresponding to Mars Rover’s API, parsing the JSON response, and grabbing the URL associated with a random image for whatever Martian Solar day we provide.
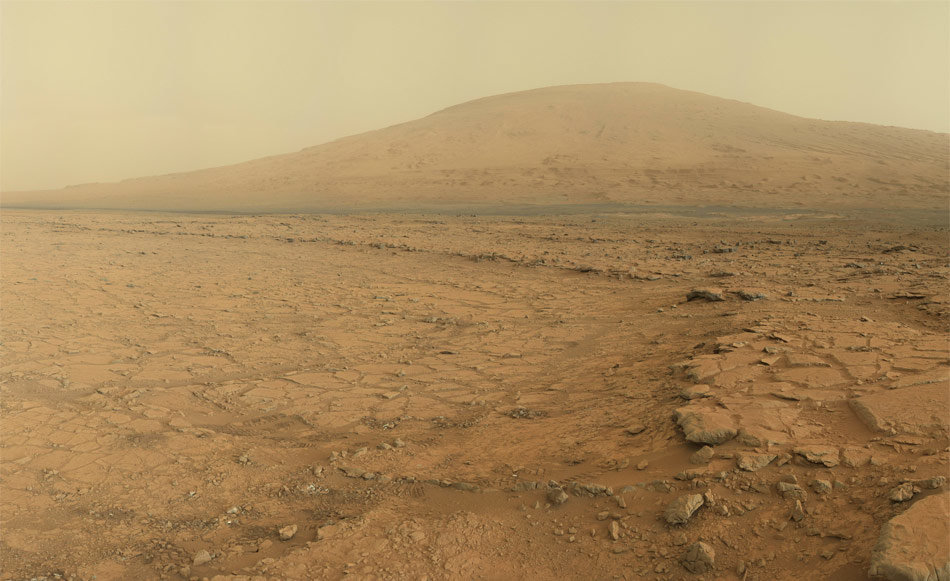
Setting up your Twilio account
Before being able to respond to messages, you’ll need a Twilio phone number. You can buy a phone number here.
Your Flask app will need to be visible from the Internet in order for Twilio to send requests to it. We will use ngrok for this, which you’ll need to install if you don’t have it. In your terminal run the following command:
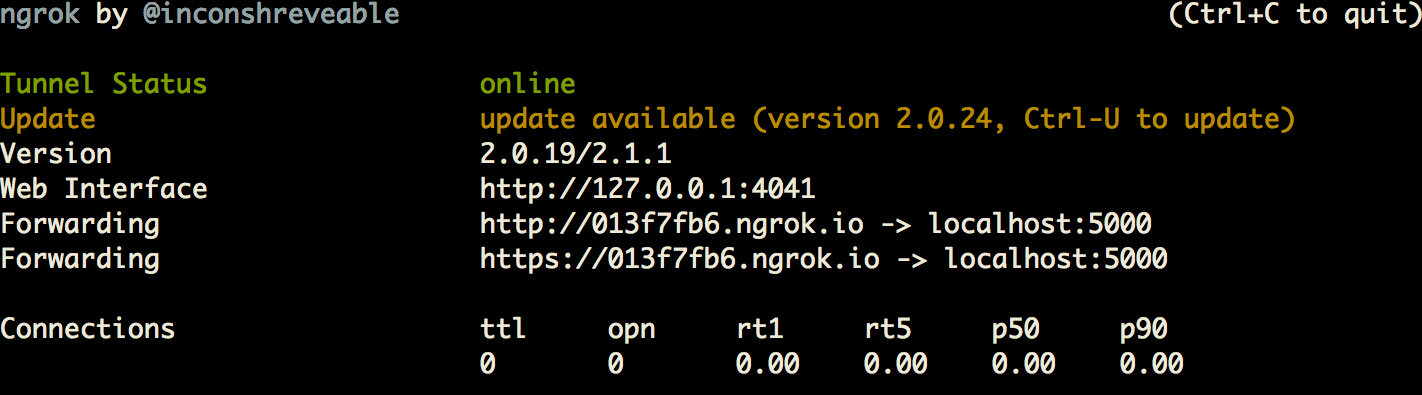
This provides us with a publicly accessible URL to the Flask app. Configure your phone number as seen in this image:
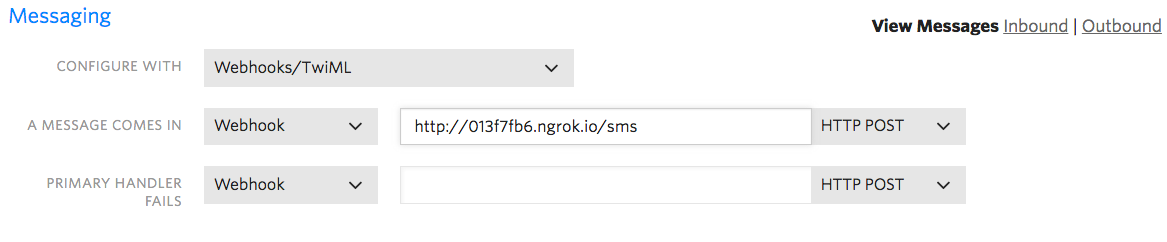
You are now ready to send a text message to your new Twilio number.
Building the Flask app
Now that you have a Twilio number and are able to grab a URL corresponding to an image taken on Mars, you want to allow users to text a phone number to view these images.
Let’s create our Flask app. Open a new file called app.py
and add the following code:
We only need one route on this app: /sms
to handle incoming text messages.
Run your code with the following terminal command:
Now text your Twilio number to literally communicate with a robot on Mars!
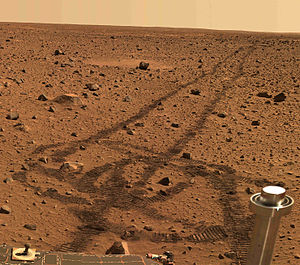
What just happened?
With this app running on port 5000, sitting behind our public ngrok URL, Twilio can see your application. Upon receiving a text message:
- Twilio will send a
POST
request to/sms
. - The
inbound_sms
function will be called. - A request to the Mars Rover API will be made, receiving a JSON response that then gets parsed to acquire an “img_src” URL to a photo from Mars.
- Your
/sms
route responds to Twilio’s request telling Twilio to send a message back with the picture we retrieved from the Mars Rover API.
Feel free to reach out if you have any questions or comments or just want to show off the cool stuff you’ve built.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.