Writing a Node.js module in TypeScript
Time to read: 4 minutes
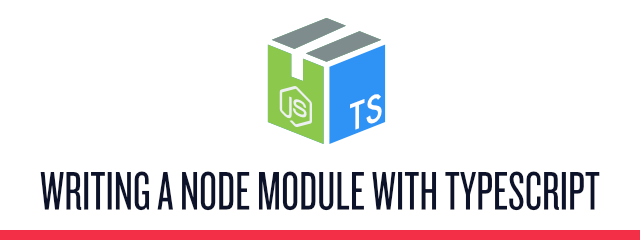
One of the best things about Node.js is its massive module ecosystem. With bundlers like webpack we can leverage these even in the browser outside of Node.js. Let’s look at how we can build a module with TypeScript usable by both JavaScript developers and TypeScript developers.
Before we get started make sure that you have Node.js installed – you should ideally have a version of 6.11 or higher. Additionally make sure that you have npm or a similar package manager installed.
Let’s build a module that exposes a function that filters out all emojis in a string and returns the list of emoji shortcodes. Because who doesn’t love emojis?
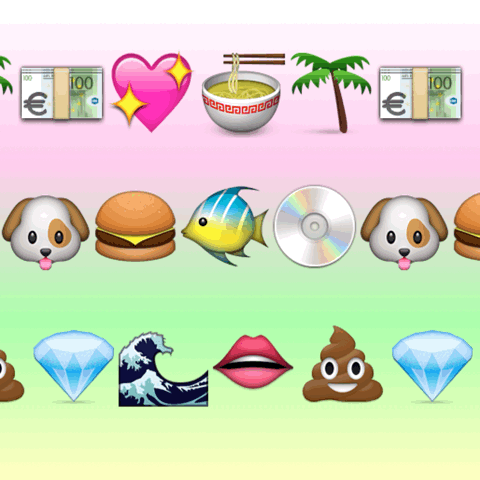
✨ Installing dependencies
First create a new directory for your module and initialize the package.json
by running in your command line:
The resulting package.json
looks like this:
Now let’s install some dependencies. First install the TypeScript compiler as a devDependency
by running:
Next install the emojione
module. We’ll use this to convert emojis to their shortcodes like 🐵 to :monkey_face:
. Because we will be using the module in TypeScript and the module doesn’t expose the types directly we also need to install the types for emojione
:
With the project dependencies installed we can move on to configuring our TypeScript project.
🔧 Configuring the TypeScript project
Start by creating a tsconfig.json
file which we’ll use to define our TypeScript compiler options. You can create this file manually and place the following lines into it:
Alternatively you can auto-generate the tsconfig.json
file with all available options by running:
If you decided for this approach just make sure to adjust the declaration
and outDir
options according to the JSON above.
Setting the declaration
attribute to true
ensures that the compiler generates the respective TypeScript definitions files aside of compiling the TypeScript files to JavaScript files. The outDir
parameter defines the output directory as the dist
folder.
Next modify the package.json
to have a build script that builds our code:
That’s all we have to do to configure the TypeScript project. Let’s move on to writing some module code!
💻 Create the module code
Create a lib
folder where we can place all of our TypeScript files and in it create a create a file called index.ts
. Place the following TypeScript into it:
Compile the code by running:
You should see a new dist
directory that has two files, index.js
and index.d.ts
. The index.js
contains all the logic that we coded compiled to JavaScript and index.d.ts
is the file that describes the types of our module for use in TypeScript.
Congratulations on creating your first module accessible to both TypeScript and Javascript! Lets prep the module for publishing.
🔖 Prepare for publishing
Now that we have our module, we have to make three easy changes to the package.json
to get ready to publish the module.
- Change the
main
attribute to point at our generated JavaScript file - Add the new
types
parameter and point it to the generated TypeScript types file - Add a
prepublish
script to make sure that the code will be compiled before we publish the project.
We should also make sure to exclude unnecessary files from the installation of our module. In our case the lib/
folder is unnecessary because we only need the built files in the dist/
directory. Create a new file called .npmignore
and place the following content into it:
That’s it! 🎉 You are ready now to publish your module using npm publish
. Unfortunately someone already built a module called emoji-search
😕 so if you want to publish this module, just change the name in the package.json
to another name.
🍽 Consume the module
The great thing with our module is that this can now be seamlessly used in JavaScript or TypeScript projects. Simply install it via npm
or yarn
:
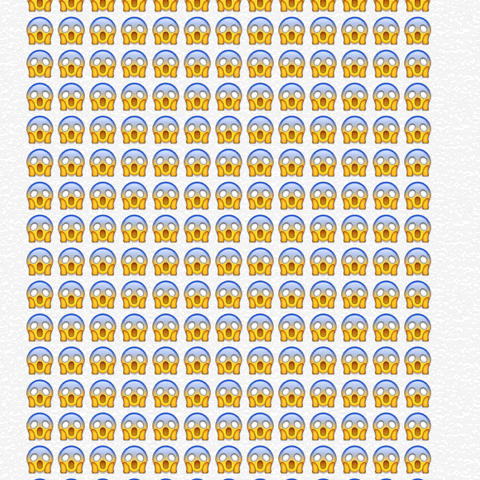
If you want to try this out without publishing the module yourself you can also install the demo-emoji-search
module. It is the same code published on npm. Afterwards we can use the module in JavaScript:
Or in TypeScript with full type support:
🎊 Conclusion
Now this was obviously just a very simple module to show you how easy it is to publish a module usable in both Javascript and TypeScript.
There are a boatload of other benefits provided by TypeScript to the author of the module such as:
- Better authoring experience through better autocomplete
- Type safety to catch bugs especially in edge cases early
- Down-transpilation of cutting-edge and experimental features such as decorators
As you’ve seen it’s very easy to build a module in TypeScript to provide a kickass experience with our module to both JavaScript and TypeScript developers. If you would like to have a more comprehensive starter template to work off that includes a set of best practices and tools, check out Martin Hochel’s typescript-lib-starter on GitHub.
✌️ I would love to hear about your experience with TypeScript and feel free to reach out if you have any problems:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.