Putting the helmet on – Securing your Express app
Time to read: 15 minutes
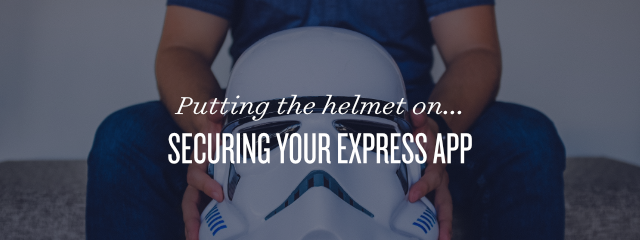
Express is a great way to build a web server using Node.js. It’s easy to get started with and allows you to configure and extend it easily thanks to its concept of middleware. While there are a variety of frameworks to create web applications in Node.js, my first choice is always Express. However, out of the box Express doesn’t adhere to all security best practices. Let’s look at how we can use modules like helmet
to improve the security of an application.
Set Up
Before we get started make sure you have Node.js and npm (or yarn) installed. You can find the download and installation instructions on the Node.js website.
We’ll work on a new project but you can also apply these features to your existing project.
Start a new project in your command line by running:
Install the Express module by running:
Create a new file called index.js
in the secure-express-demo
folder and add the following lines:
Save the file and let’s see if everything is working. Start the server by running:
Navigate to http://localhost:3000 and you should be greeted with Hello World
.
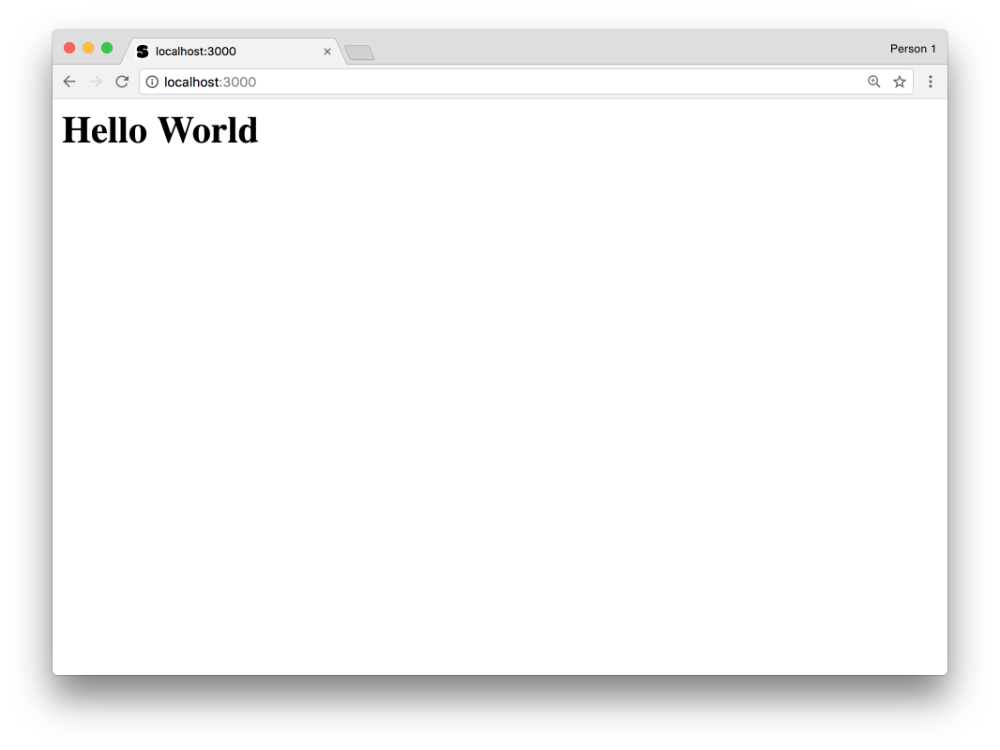
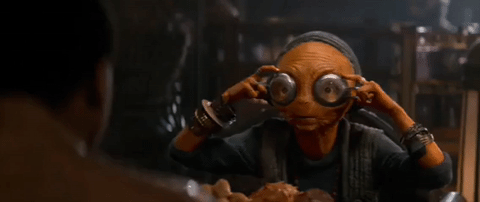
We’ll start by adding and removing a few HTTP headers that will help improve our security. To inspect these headers you can use a tool like curl
by running:
The --include
flag makes sure to print out the HTTP headers of the response. If you don’t have curl
installed you can also use your favorite browser developer tools and the networking pane.
At the moment you should see the following HTTP headers being transmitted in the response:
One header that you should keep an eye on is X-Powered-By
. Generally speaking headers that start with X-
are non-standard headers. This one gives away which framework you are using. For an attacker, this cuts the attack space down for them as they can concentrate on the known vulnerabilities in that framework.
Putting the helmet on
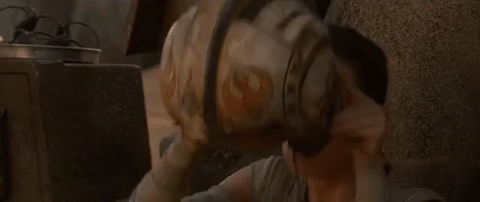
Let’s see what happens if we start using helmet
. Install helmet
by running:
Now add the helmet
middleware to your application. Modify the index.js
accordingly:
This is using the default configuration of helmet
. Let’s take a look at what it actually does. Restart the server and inspect the HTTP headers again by running:
The new headers should look something like this:
The X-Powered-By
header is gone, which is a good start, but now we have a bunch of new headers. What are they all doing?
X-DNS-Prefetch-Control
This header isn’t much of an added security benefit as much as an added privacy benefit. By setting the value to off
it turns off the DNS lookups that the browser does for URLs in the page. The DNS lookups are done for performance improvements and according to MDN they can improve the performance of loading images by 5% or more. However this look up can make it appear like the user visited things they never visited.
The default for this is off
but if you care about the added performance benefit you can enable it by passing { dnsPrefetchControl: { allow: true }}
to the helmet()
call.
X-Frame-Options
X-Frame-Options
allows you to control whether the page can be loaded in a frame like
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.