Build Chrome Desktop Notifications in PHP with Laravel 8, Pusher, and Twilio SMS
Time to read: 7 minutes
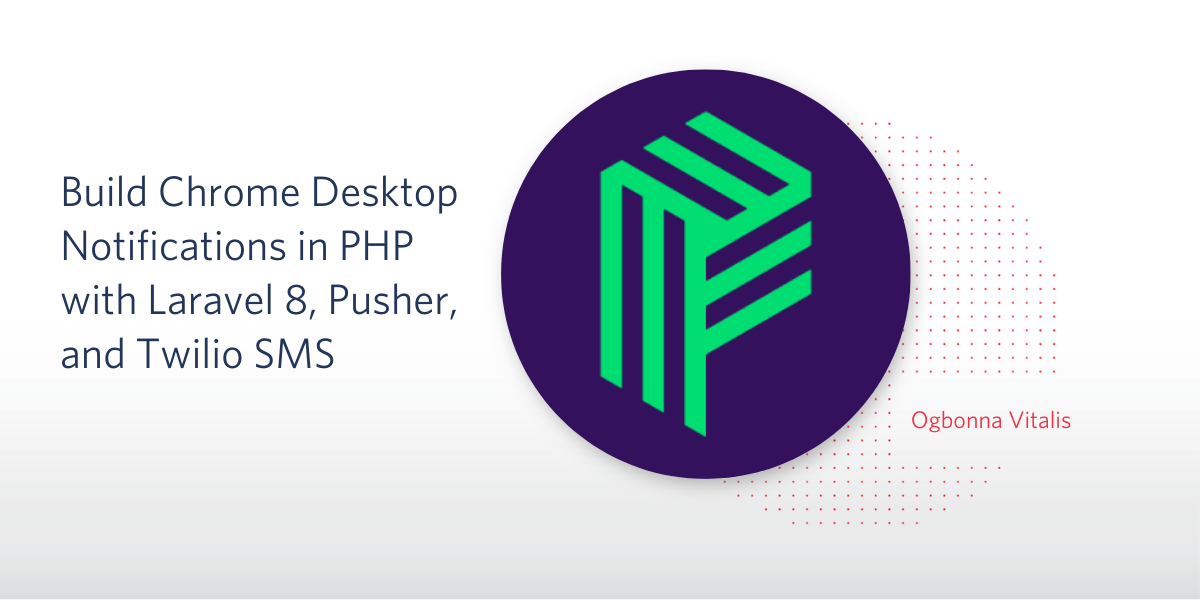
In modern day web applications, real-time notifications are very common as service providers want to keep users informed and engaged about events happening on their platforms. Notifications are a great way to build incentive for users to come back to the platform.
In this tutorial, we will be implementing a real-time application with desktop notifications by utilizing your browser’s Notification API, Laravel, Pusher, and notifications via Twilio Programmable Messaging.
Prerequisites
To follow along in this tutorial, here are the essentials to get started:
- PHP and Laravel 8 installed on your machine.
- Working knowledge of PHP and JavaScript.
- A Twilio Account
Set up a Laravel Application
This tutorial assumes that you are somewhat familiar with PHP and the Laravel Framework. Hence, we will quickly go through the installation process of Laravel and the procedures needed to create a brand new Laravel 8 Application.
Create a Laravel Application
First, download and install Composer, the PHP package manager, to your local machine.
After a successful installation, typing the word composer
in the command line will give an output as shown in the following screenshot:
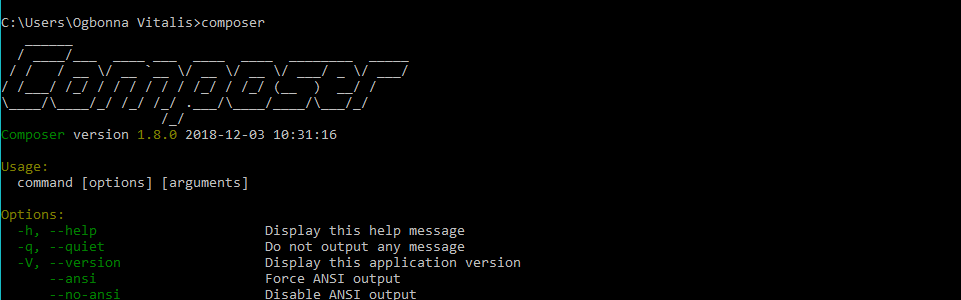
Now that Composer is installed, we can proceed to install Laravel on your local machine.
To download and install Laravel on your local machine, type the following command in your terminal.
Note that the installation may take some time to complete, depending on your connection speed.
Now that Laravel has been successfully installed, we can proceed with the creation of a brand new Laravel application for our project.
The name of our project in this tutorial will be called "desktop-notes-twilio". This application can be cloned from my GitHub repo here if you would like to take a look at the code directly, while following along.
Create this application by using the laravel new
command:
Once the installation is complete, navigate to this project folder from the command line and serve this application using the following command:
This will make our "desktop-notify-twilio" application live and accessible via the browser on port:8000
.
To access our newly created Laravel application, we navigate to any browser of choice and type in the address http://127.0.0.1:8000. Successful launch should display the Laravel welcome message in the browser as shown in the following image:
Hurray! The application is successfully initialized and is ready for development.
Set Up the Models, Migrations, and Controllers
After installing the frontend dependencies, we will need to set up our models, migrations, and controllers of our application.
Create a model and migration at the root directory of our project with the following command:
Paste the following schema inside of the database\migrations\2020_09_16_132505_create_posts_table file:
Migrate this schema after editing the .env file with your database details. Create the table by running the artisan migration command on your terminal:
After migration, edit the mass assignment properties of the model class that was created in app/Models/Post.php file:
Having completed the models and migrations setup, create the PostController
file using the make controller artisan command:
Save a Post
Add a route and controller method to save a new post. We will be making a call to the PostController
from the frontend to save our posts. Add the new route to the web routes at routes/web.php.
Having completed the route setup, we add the corresponding controller method as follows:
Work with Events and the Laravel Pusher Package
The importance of organization and separation of concerns cannot be overemphasized in the software development industry. Events in Laravel are a great way to decouple event login within your application.
We can define events to be triggered within our application when an action occurs, and we can define listeners to listen for such events and carry out other activities.
Laravel allows for easy definition of events and listeners out of the box. It also includes helper functions and classes to allow us to easily trigger and broadcast events.
Create and Configure Laravel Events
To create an event for each post created in our application, we use the make:event
command via our terminal, at the root directory of our project:
The PostCreated.php and PostLiked.php Event files are created inside the App\Events folder. Now, write the following code inside the PostCreated.php file:
Also inside PostLiked.php file write:
The Illuminate\Contracts\Broadcasting\ShouldBroadcast
interface on the event class is used to inform Laravel that this event should be broadcasted.
The broadcastOn
method returns the channel that we want to broadcast our event on. The Channel
class is used for broadcasting on public channels. PrivateChannel
and PresenceChannel
are for private channels. Public channels are channels made available for all users of the application. Here authorization is not required, whereas authorizations are required and only authorized users can listen to private channels.
By default, Laravel broadcasts all of an event class’ public properties as its payload. The broadcastWith
function helps us override that behavior and choose which specific class property to send.
Dispatch Events
In our app, we want to dispatch the PostPublished
event after a post has been saved or liked. In Laravel, we can dispatch events using the Event Facade, or the event()
helper function.
To dispatch our PostPublished
event, we can edit the store
method in the PostController
, and place the event call right after the post is saved:
Install and configure Pusher PHP server in Laravel
So far, we have configured and dispatched our events. It's now time to set up Pusher for Laravel.
Install pusher-php-server using the following command:
Next, we’ll set up some minor configuration to let Laravel know we will be using Pusher to manage our broadcasts.
Create a Pusher account here if you don't already have one, and then create a channel for your application from your Pusher dashboard as shown in the following screenshot:
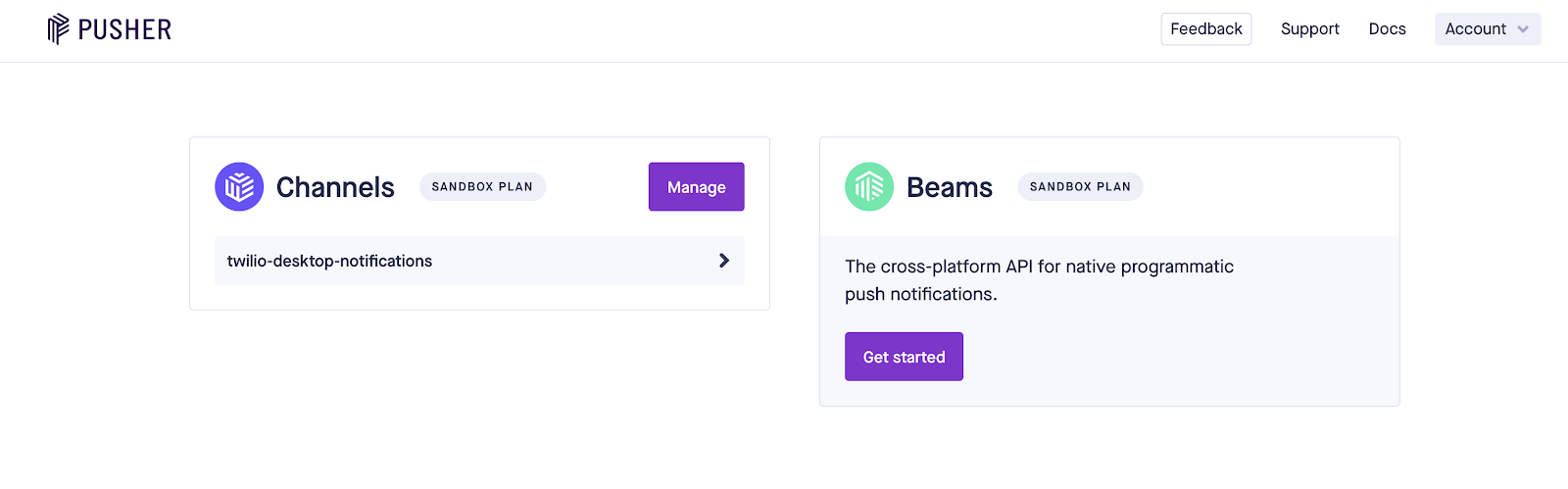
Add Pusher App Channel credentials to the .env file as follows in order to integrate the API:
Your Pusher credentials can be retrieved from the App Keys menu under your development channel as shown in the following screenshot:
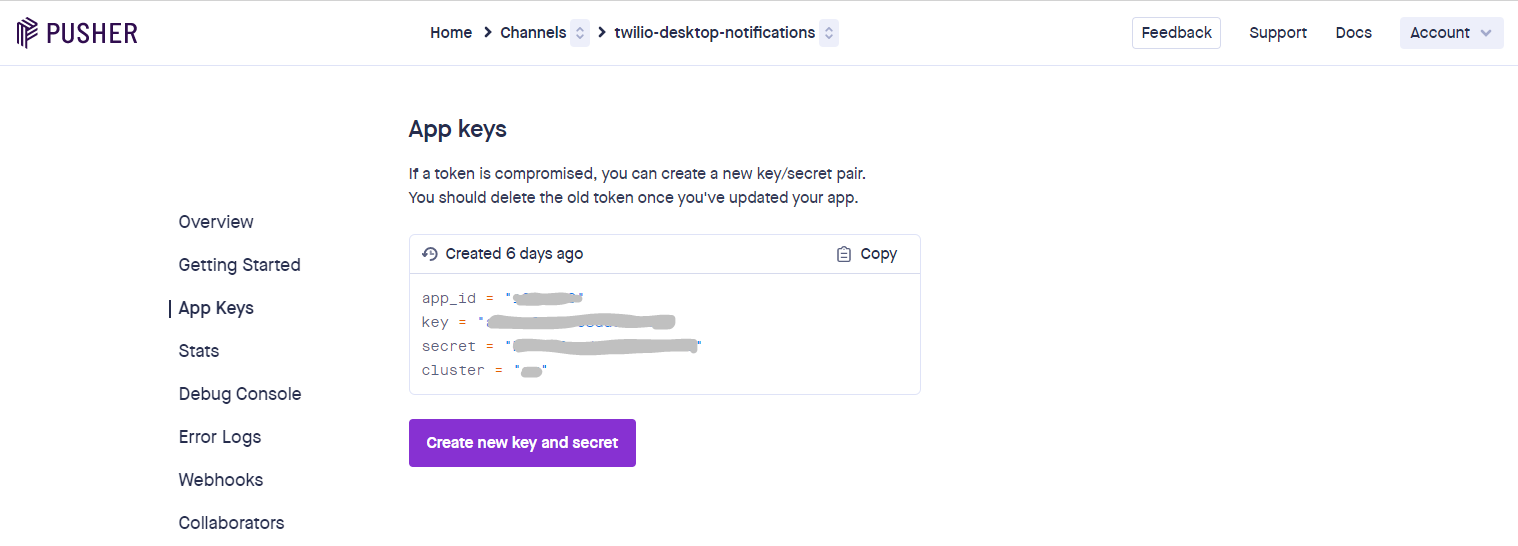
Build the Frontend
To create a basic page view for our app, we can edit the default resources/views/welcome.blade.php file created by Laravel. Replace its contents with the following:
Most of the code above is boilerplate Laravel HTML content with relevant scripts and CSS files attached.
Creating the Other Frontend Pages
Create two additional pages publish.blade.php and success.blade.php inside the resources/views folder. The publish.blade.php will have a form from which a post can be created while the success.blade.php will show success messages when a post is liked.
Add the following code to the publish.blade.php file:
Then in the success.blade.php file add:
From the code above, we created three files welcome.blade.php, publish.blade.php, and success.blade.php. The push notification in the welcome.blade.php is triggered whenever a user adds a new post or likes an existing post within the application.
To listen for events, we subscribed to the post-liked
and post-created
channels respectively. This allows us to watch the channel we are broadcasting to from our backend, listen for the PostPublished
and PostLiked
events, and define a callback that activates the desktop notification whenever any of these events are fired.
At this point, your routes file, routes/web.php should contain three routes as shown in the following code snippet:
Bring It All Together
Start the project using the command:
Navigate to the app's homepage to see it in action. If you use Laravel Valet, you can also share the app and visit it via another device to test the desktop notifications.
Thanks to Laravel and Pusher, we’ve built an event-driven, basic, real-time app enabled with desktop notifications. As a next step, we will be integrating Twilio SMS into this amazing application.
But before integrating Twilio SMS into this application, test the application and ensure the notifications are working. On Chrome browser, you can test the applications using incognito modes.
Also check your Pusher dashboards for updates on each notification sent. Your dashboard should be similar to mine as seen in the following screenshot:
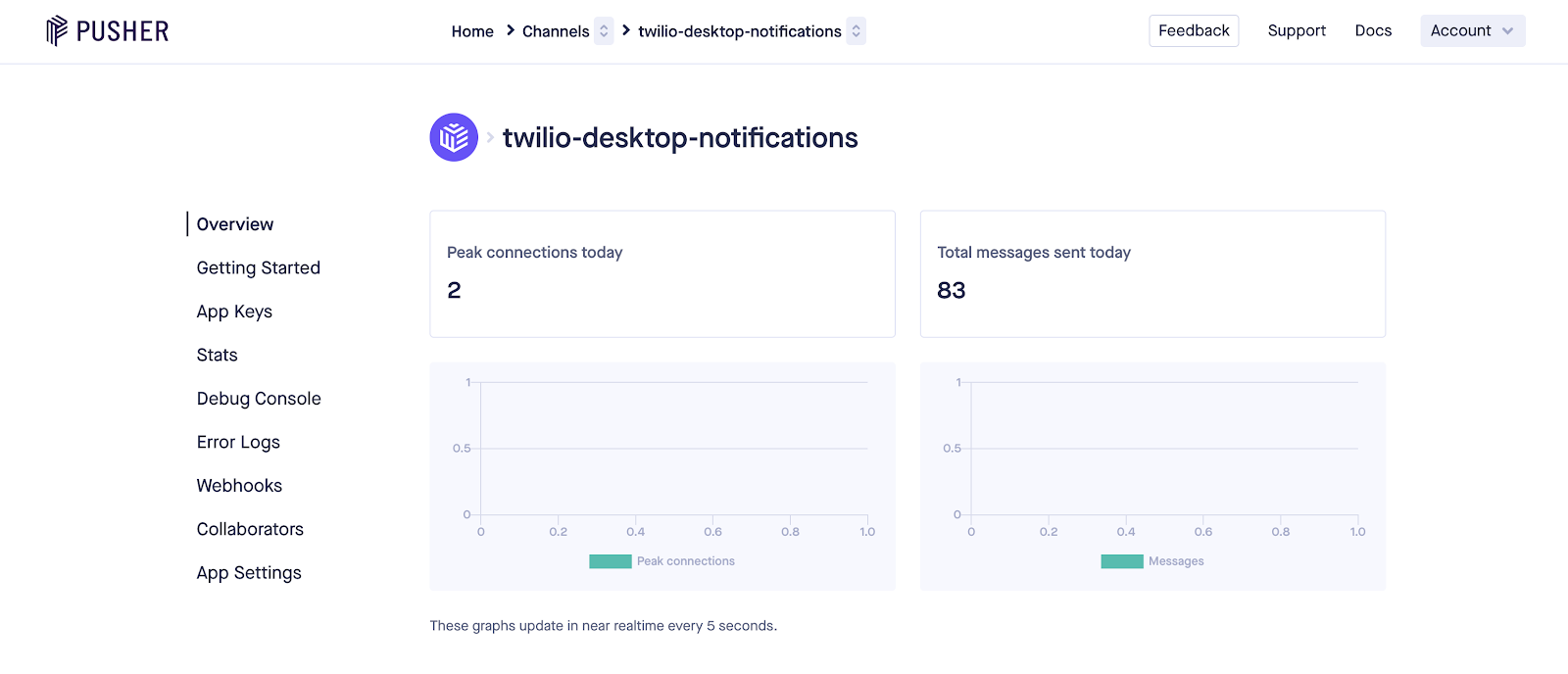
Set Up the Twilio PHP SDK
To connect the Twilio SMS API to our application, create a Twilio account and select a Twilio phone number for our application.
Locate the Twilio Account SIDand Auth Token from the Twilio Console in order to access Twilio's APIs. Be sure to store these credentials securely.
NOTE: You can only get a single Twilio phone number with your trial account. It is also required that the number to which you send the SMS be verified by Twilio and the country supported.
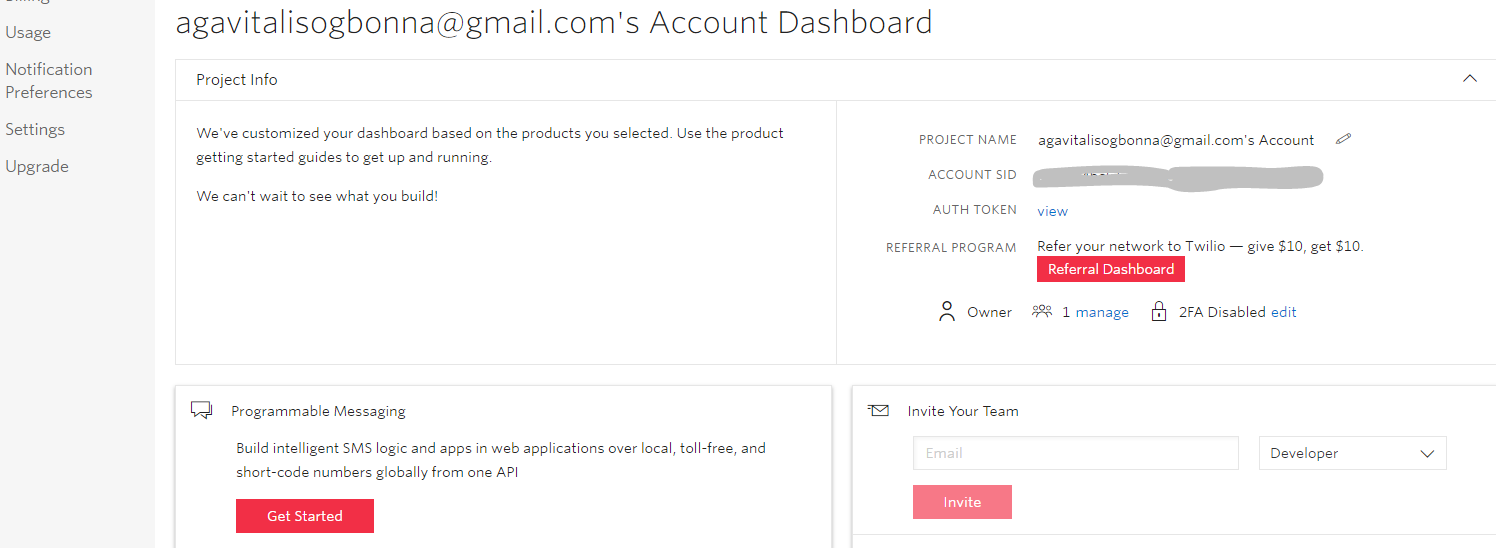
Install Twilio PHP SDK to the project
The installation process of Twilio PHP SDK is similar to the Laravel Bouncer package which we installed earlier. To install the Twilio SDK, type the following Composer command in the terminal to bundle the Twilio SDK:
Configure Twilio SDK to work with the application
Add the following credentials in the .env file:
In order to make the Twilio configuration variables available globally in our application, we will add the following lines of code in our config/services.php file.
Edit the PpostController
as shown below to enable SMS notification after post creation. Don’t forget to change the Twilio phone number used in this sample tutorial to yours.
Test the Laravel Desktop Notification App
To test the application, serve this application using the PHP artisan server command:
This command will make the application accessible via the browser using the address http://localhost:8000 where we can create and like posts, receive a push notification on the desktop, and receive a Twilio confirmation SMS on the posts created.
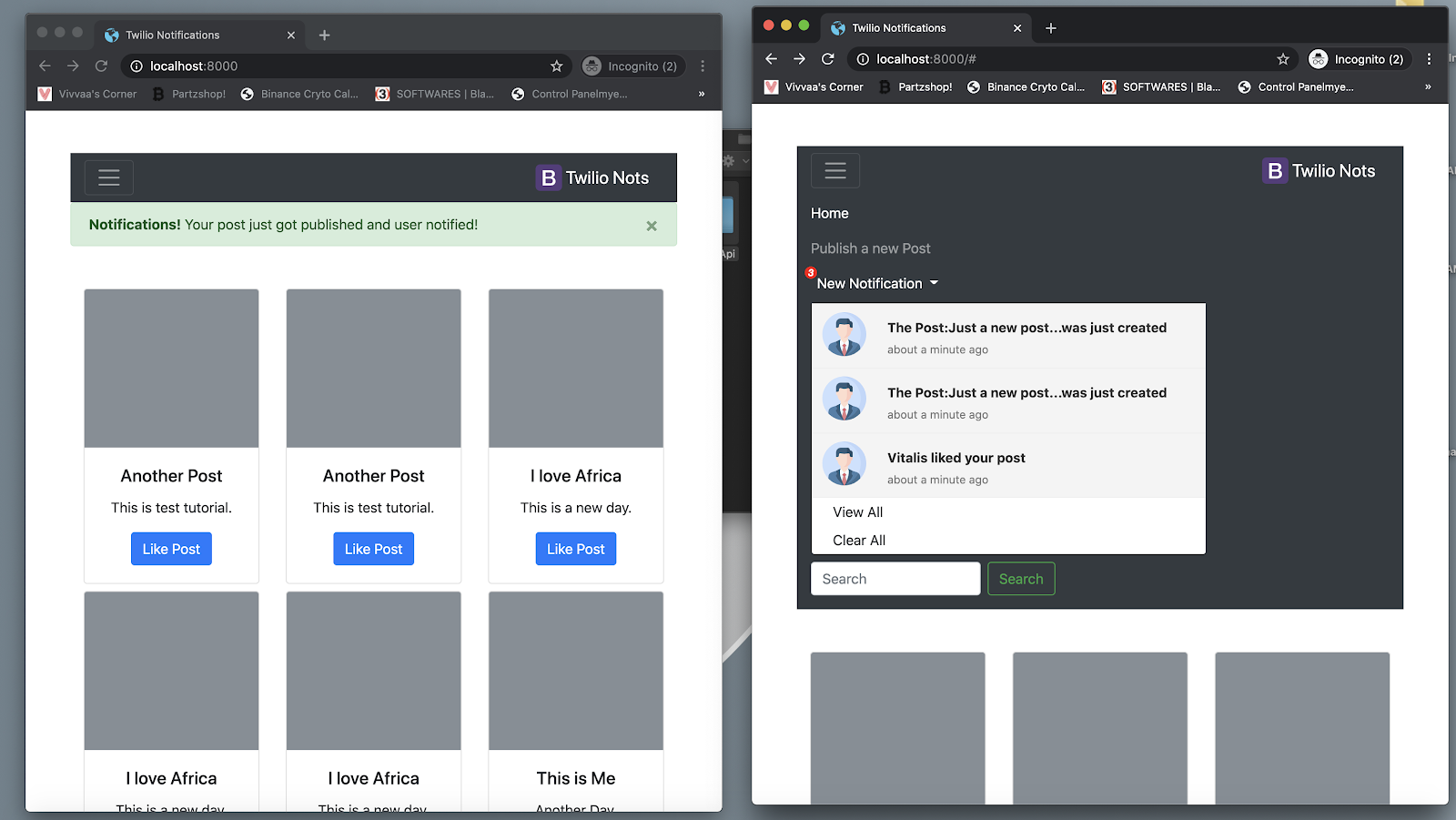
Conclusions and Recommendations
We have successfully created a Laravel application that integrates Pusher and events for notifications. We also sent an SMS using the Twilio SDK in a Laravel application. The source code of this application can be found on my Github repo here.
This application however is limited in functionality as it should have authentication, the post liked should be stored in the database, and the creation of new posts restricted to authenticated users only.
As a recommendation, try adding these extra features while you explore the amazing functionalities of Twilio Programmable Messaging, Laravel, and Pusher.
Vitalis is a software engineer who loves technology and finds hubbies in teaching and learning. He loves comedy, quality discussions and Football. You can find Vitalis on Twitter via @agavitalis, GitHub via @agavitalis, Linkedin, and his blog, Vivvaa’s Corner.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.