VOIP Apps for iOS with Twilio Client and Azure Mobile Services
Time to read:
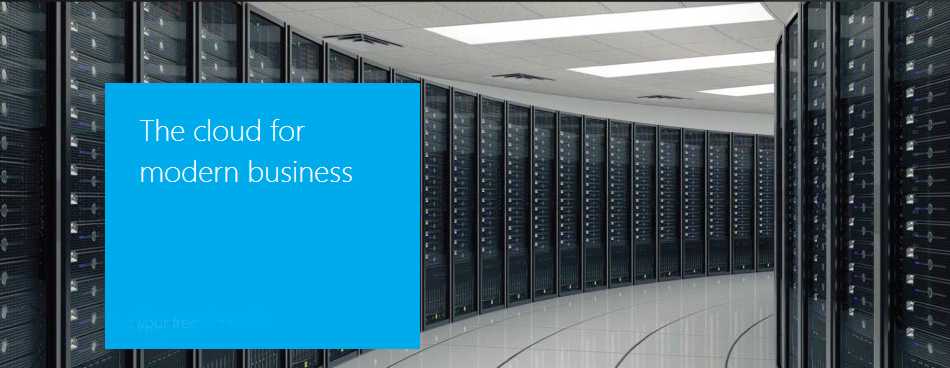

In this tutorial, we will focus on how to integrate with these services on iOS as we customize a simple todo list application. We will also be integrating Twilio Client, which will enable us to dial phone number links directly from our iOS app. We’ve got a lot to do, so let’s get started!
First Steps
If you haven’t already, sign up for a Windows Azure account. A free trial is available for new users so you should be able to get started right away. To build a Windows Azure Mobile Services application, you first need to create an application in the Windows Azure management portal. On the dashboard, click the “NEW” link on the bottom of the screen. Choose to create a new mobile service, which is under the “COMPUTE” sub menu:


Next, you will create a new database server, which will contain your application’s data. If you’re creating a database for the first time, a configuration that looks like this should suffice – make a note of the database admin username and password, should you need to manually access the database later:


On your first AMS application, you will be presented with a Quick Start dashboard for your mobile app. You’ll see options to get started with many different client-side platforms, but today, we’ll be focusing on iOS. Please note that in order to use Xcode 4 and the iOS development tools, you will need to be running a Mac running a supported version of OS 10 (10.6 Snow Leopard as of this writing). Choose the quick start guide for iOS:




Using Windows Azure Mobile Services for Data Persistence
We won’t walk through all the code for the generated sample app, but let’s look at some of the highlights. Right now, our application only has a single view controller, which will be created by the app’s default storyboard. In the generated project, this file is called “QSTodoListViewController.m”. The first lifecycle event we care about is “viewDidLoad”, which will be run once the view controller has been initialized from the storyboard/xib file:
The Windows Azure Mobile Services service is initialized on line 6 of the snippet above. This class method will instantiate a new singleton object, which will access your todo list item data store in the cloud. This requires a special back end key which is embedded in your application, as in this code below, taken from “QSTodoService.m”:
This client will be used to read, create, and update todo items from your database. To create a todo item, you will access the Windows Azure Mobile Services client to save an NSDictionary of properties in a database table. Note that properties of back end tables do not need to be defined ahead of time (by default) in the data store – Windows Azure Mobile Services will dynamically add columns for any properties added to the dictionary object we pass in! You’ll also notice that Objective-C blocks are used for any operations that require network access, and will be executed asynchronously:
This code is used to update a record in a data store table:
To read items from the data store, we create a query (using NSPredicate) to fetch all todo list items that are not completed:
Just like that, we have a native iOS application integrated with our Windows Azure Mobile Services back end. Now, wouldn’t it be cool if our todo list application were smarter with links in the text content? What if when a phone number was detected in the todo item, we could click it and immediately place a call to that number, right in our app?
The Twilio Client SDK for iOS lets us do just that, but let’s start by modifying our table view to display phone numbers as clickable links using a third-party component designed to do that intelligently.
Integrating Third-Party Components with CocoaPods
CocoaPods is a community-driven repository for Objective-C libraries. Many library authors make their software available as “pods”, so users can quickly install these modules (and their dependencies). If you don’t already have Cocoapods installed, get it set up by following the instructions on the home page. CocoaPods is distributed as a Ruby gem, which should be easy to install from the Terminal in OS X. For most systems, the following two commands are sufficient to install and setup CocoaPods:
[sudo] gem install cocoapodspod setup
CocoaPods will help you install components your project depends on using a configuration file called a Podfile. To edit this file, navigate to the same directory as the “supertodos.xcodeproj” directory in the Terminal (replace “supertodos” with your app’s name). At the same directory level as the Xcode project directory, create a file named “Podfile”. Open it in your favorite text editor, and enter in the following contents (it’s actually a Ruby file):
Install these pods (and their dependencies) by running “pod install” in the Terminal. NOTE: By using Cocoapods, you will now need to launch your project via the .xcworkspace file associated with your xcode project! Let’s do this now – quit any running simulators and quit out of Xcode (not strictly necessary, but let’s avoid the confusion of multiple windows). From the terminal, run the command “open supertodos.xcworkspace”, replacing “supertodos” with the name of your application. When Xcode launches, you should see a workspace that looks similar to this:

TTTAtributedLabel will help us to display links via a drop-in replacement for UILabel. In our case, we want to automatically link any phone numbers inside our todo item labels, and respond when they are clicked. Since we’re going to be programmatically creating labels, go into “MainStoryboard_iPhone.storyboard” and “MainStoryboard_iPad.storyboard” in Interface Builder, select the Labels that say “Label”, and hit delete on your keyboard. After you have removed those labels, we need to write some code to display touchable links.
To start customizing the table cells, we will need to edit “QSTodoListViewController.m” and “QSTodoListViewController.h”. In “QSTodoListViewController.h”, we’ll import the TTTAttributedLabel header, and indicate that we intend to implement parts of the TTTAttributedLabelDelegate protocol:
In “QSTodoListViewController.m”, we’ll begin by importing the necessary header at the top of the file:
Now, when we generate table view cells for the main table view control, we will use these attributed labels rather than the built-in UI Label. Replace the current version of “tableView:cellForRowAtIndexPath:” with the following implementation:
To actually handle the phone number clicks, we will need to implement part of the TTTAttributedLabelDelegate protocol. Anywhere in your controller file, add the following code, which for now will just print out the phone number value when a click happens:
Run the code in the simulator. Enter a todo item that contains a telephone number. Todo items with telephone numbers will be displayed like this:
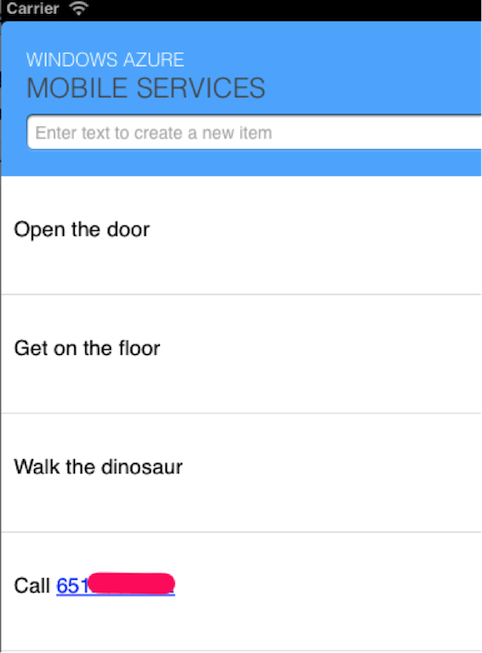
When you tap the underlined phone number, you should notice a logging message in Xcode which contains the text of the phone number. Now it’s time to use Twilio to initiate a VoIP call to that number!
Using Twilio Client for VoIP Calling
Previously, when we installed the Cocoapod for TTTAttributedLabel, we also installed an in-development pod containing the Twilio Client SDK for iOS. We’re going to put it to use now by making an outbound call directly from our iOS app.
To use Twilio Client, your application needs to have code running on a server to generate what’s called a capability token. This is a long string which is signed with your Twilio Account SID and auth token, which you received when signing up for an account.
In the interest of time, we won’t dive into setting up a server. For the next week or so after this blog post, I will make a URL available that can generate tokens, which will be embedded in the code below. This server-side application is written in node.js, and is deployed as a Windows Azure Web Site! You can check out the source here.
To start making some phones ring, include the Twilio Client libraries at the top of your view controller file:
Before making an outbound call, we need to fetch a capability token from a server. When our view is initialized, we’ll make an HTTP request to grab a capability token, and create a Twilio Device (which acts as a soft telephone in your mobile app). In the UIView section, add this method to initialize the device:
Now, when the phone number is tapped, let’s make a call! To enable this, replace the TTTAttributedLabelDelegate method with the following:
In a few moments, you should have an outbound call connecting from your iPad or iPhone to any phone on the PSTN network!
Conclusion
In this example, we created a Windows Azure Mobile Services application, integrated it into a native iOS project, and added in some basic voice calling functionality. Obviously, this is just scratching the surface of what’s possible both with Windows Azure Mobile Services and Twilio Client. Windows Azure Mobile Services provides a slick back end for mobile apps, eliminating tons of server side code. Twilio Client eliminates the headaches of VoIP infrastructure and adds voice collaboration to any application. Together, they’re excellent additions to a mobile developer’s tool belt that will slash effort and lines of code from their projects. Hope you enjoy using both!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.