Capturing Twilio Video JS SDK Logs
Time to read: 3 minutes
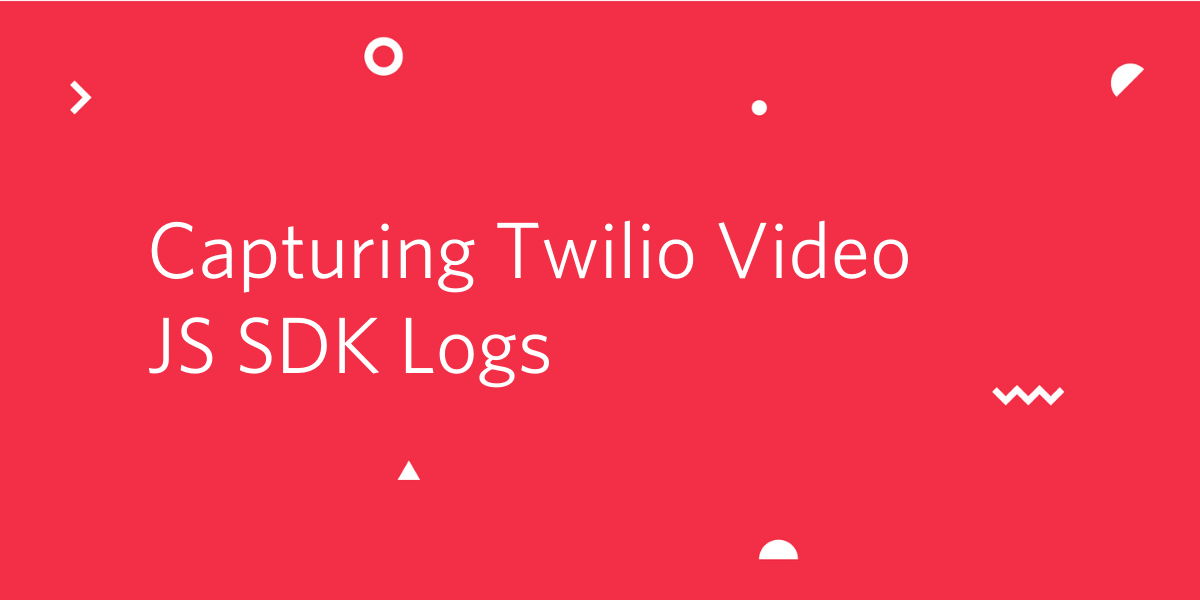
Logging provides visibility into your application's behavior. This information is essential when troubleshooting operational issues. Traditionally, however, logging is only performed on the backend in a production build. Frontend applications usually hide client side debug logs and only expose warnings and errors by default. Even with debug logging enabled, there is no easy way to send logs to a remote server, making it hard to debug issues in production.
While application developers can build logging mechanisms to capture client side logs and send them to a remote server, the growing number of third party libraries and SDKs makes this harder to do. In most cases, third party libraries and SDKs have their own logging mechanisms and do not expose a way for developers to capture their specific logs.
Now with Twilio Video JS Logger, developers can intercept logs generated by the Twilio Video JS SDK. This allows for real-time log processing to easily monitor your frontend applications and see how they behave in production.
Getting Started with Twilio Video JS Logger
In this section, I’ll show you how to add the Twilio Video JS Logger to your existing Twilio Video JS application. If you are new to Twilio Video JS, check out our example application and get started in minutes. Let’s dive into the code!
Setup
The SDK uses the loglevel module and is exposed via Video.Logger API. Use this API to access internal loggers and perform actions as defined by the loglevel APIs.
Intercepting Logs
You will need to override the logger's original method factory to begin intercepting logs. With this approach, you have the option to process each log before it is printed out to the console. The following example shows how to add the prefix `[My Application]` to each log prior to connecting to a room.
Example log output:
Callback Parameters
The Logger callback returned in the method factory contains parameters provided by the SDK. You can use these parameters to gather more information about the log – and potentially act on it. For example, you may want to filter logs for a specific component and send them to a different server.
Here's a list of the callback parameters along with a brief description.
- datetime - The current date and time in simplified extended ISO format.
- logLevel - The current logging level. Possible values include debug, info, warn, and error.
- component - The component where the log originated using `
[name #count]
` format, where name is the component name, and count is the instance count. For example, `[createLocalTracks #1]
`. - message - The message that is being logged.
- data - An optional data object which can be inspected for more information about the log. The example below shows how to use the data object when checking for signaling events. In the future, any new type of data will be published in the SDK changelog.
Plugins
The loglevel module used by the SDK is a lightweight logging library that works in all modern browsers and NodeJS environments.
While loglevel
isn't useful in all instances, its plugin system allows you to add features specific to your applications, such as formatting a log message. For example, in the getting started section, we are able to prefix each log with [My Application]
by overriding the logger’s original method factory. This approach is basically how the plugin system works when using loglevel
.
One common use case in client side logging is the ability to forward logs to a remote server to monitor your frontend applications. Using the plugin system, you can intercept the logs, transform them into your own format, and forward them to your server. Fortunately, there is a plugin that supports this use case. The loglevel-plugin-remote module allows loglevel
to send logs to a server with very minimal configuration.
The example above demonstrates how to use loglevel-plugin-remote
where the plugin is applied to the root Logger
object before accessing the SDK’s internal logger. With this configuration, logs are automatically sent to /logger
endpoint. You can update this endpoint and other configurations by following the loglevel-plugin-remote
‘s APIs.
Start Collecting Logs!
By enriching your logs and centralizing them with a logging server, you can easily monitor your frontend applications and see how they behave in production. This is just one of the many use cases we’ve heard from our customers and can’t wait to see what comes next.
Please feel free to head over our GitHub repo and leave feedback.
Charlie Santos is a Software Engineer on the Programmable Voice and Video team at Twilio. He builds real-time communication web applications and libraries that help customers hit the ground running with Twilio and WebRTC. He can be reached at csantos@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.