How To Create GIF Polls With Wedgies and Twilio MMS
Time to read: 1 minute
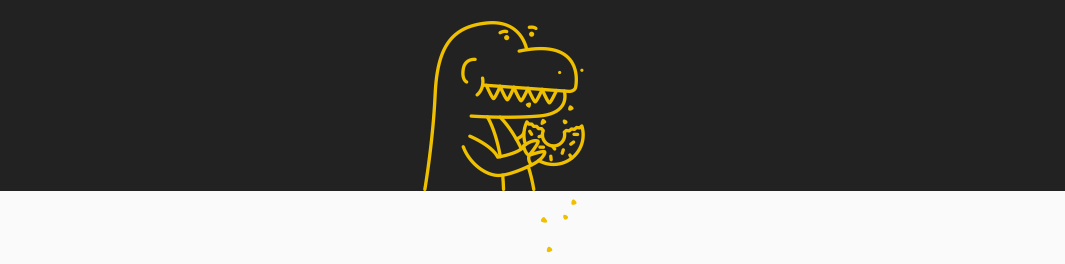
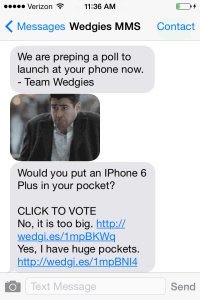
In this post, Wedgies all-star/co-founder Jimmy Jacobson will show you how to use Twilio MMS create your own poll that you can share and vote on, all with a simple text.
Check out Jimmy’s tutorial below and fork it here.
Creating a GIF Poll using Twilio MMS and Wedgies
Store your Wedgies API key in an environment variable and reference in a global variable.
This is the express route to handle the incoming Twilio SMS
This function is defined below, and returns a Wedgies Poll from the Wedgies API or an error object that can be passed to the Express function next.
Make sure your Twilio credentials are stored in environment variables.
Prepare the MMS message with a to, body and from fields.
If the Wedgies poll has an image, make it an MMS!
Send the MMS using Twilio’s awesome NodeJS client library
This is the function that hits the Wedgies API and returns a poll
Here is actual API call using Request
This is a pet peeve of mine. Use objects for errors, not strings. Here we handle the case where the request flat out fails. This could be due to a bad URL or similar.
Here we handle the case where the Wedgies API call is successful, but the response code is something other than 200
Finally, everything looks good, return the poll.
That’s it! Take a look a deeper dive into Wedgies’ platform here
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.