How to Validate Phone numbers in Node/JavaScript with the Twilio Lookup API
Time to read:
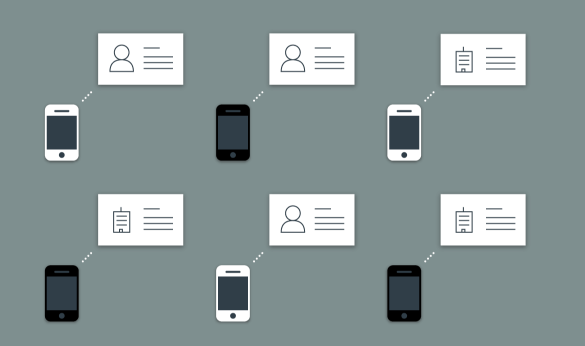
Twilio Lookup is a phonebook REST API that you can use to check whether a number exists, determine whether a phone can receive text messages, and retrieve carrier data associated with a number.
Let’s write some code to validates phone numbers using the Twilio Node module.
Getting started
Before we dive into the code you’ll need to make sure you have:
- Node.js and npm installed
- A Twilio Account – Sign up for free
- Your Account SID and Auth Token handy from the Twilio console
- The Twilio Node module. You can install this by opening your terminal, navigating to where your code lives and entering the following command:
Looking up valid phone numbers
Check out the Lookup page if you want to play around with the API and see the type of data a request will return. Try entering your own phone number and take a look at the JSON response object.
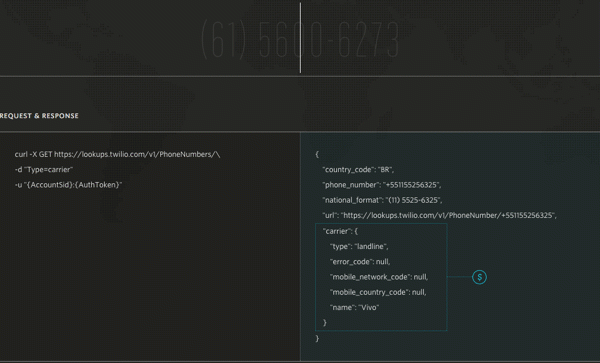
Here’s a quick code sample to do a basic phone number lookup. Create a file named
lookup.js
and add this code to it:
In your terminal navigate to the directory containing lookup.js and run this command:
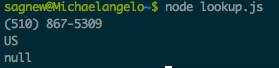
This basic functionality is free, but you can get more information by doing a carrier lookup. Carrier lookups are commonly used to determine if a number is capable of receiving SMS/MMS messages. These cost $0.005 per request but a free account has some trial credit to play with.
Carrier lookups contain a ton of useful information, but require an extra parameter.
Replace the code in lookup.js
with the following:
And run it again:
Looking up invalid phone numbers
In this “online phonebook” the phone numbers serve as a unique ID. When you try to look up a phone number that does not exist you will get a 404 response.
Head over to the Lookup homepage again to see this in action when you check a number that doesn’t exist:
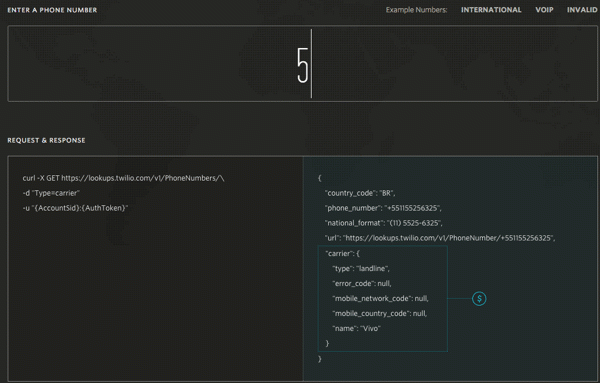
Let’s write some code that looks up a phone number and determines whether it exists or not. We’ll do this by trying to do a carrier lookup and checking to see if an error object is passed to your callback function with a 404 error code.
Replace the code in lookup.js
with the following:
Run this code in your terminal with the following command, and don’t forget to insert your Account SID and Auth Token:
You can now use this whenever you need to determine if a phone number is valid.
Looking Ahead
Now you know how to use the REST API phone book that is Twilio Lookup. You can also check out other resources to learn how to use Lookup in other languages:
- Lookup phone numbers in Python, PHP or Ruby.
- Check out the Lookups API documentation to see what else you can do.
Lookups also supports Twilio Add-ons, enabling you to retrieve information from a multitude of 3rd party data sources, available via the Twilio Marketplace. There are some awesome features that work specifically with Lookup.
Feel free to reach out if you have any questions or comments or just want to show off the cool stuff you’ve built.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.