Shell functions, git shortcuts and other magical scripts I use with the Twilio API
Time to read: 4 minutes
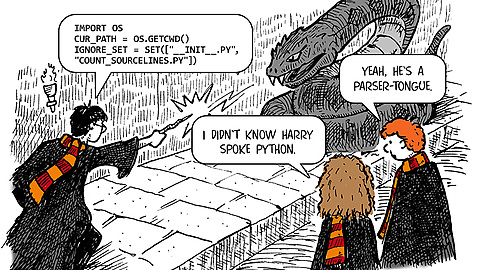
I love Harry Potter, and part of the reason I love the wizarding world so much is that those people know how to use shortcuts! I often look at the habitual tasks of my daily life and wish I had a magical umbrella that could clean up my messes and fold my clothes. But while I still longingly hope for real-world shortcuts, I at least have a good start with my developer shortcuts, and I’d like to share a few with y’all now.
The Gist
Today I will talk about a few local scripts and shortcuts that I rely on daily when working with Twilio. These fall into a few categories:
- Working with git
- Boilerplate stuff
- Twilio functions I use a lot
- Managing my local environment
- Commonly used commands
Let’s get out our time turners and start in the middle :)
Managing my local environment & commonly used commands
First off, there are a lot of people who have opinions about how best to manage your dotfiles— I am not one of them. I have used a lot of dotfile systems in the past, and they have all had their strengths and weaknesses. I currently run atomantic/dotfiles with Sublime Text as my IDE. I love that atomantic ships with a nifty setup script, and I also like that it keeps my “popular” dotfiles within the homedir
. Sorry about the acronyms and jargon there, let me break those down.
What are dotfiles?
Good question. Dotfiles are what we call configuration files for unix/linux systems, since config files on these systems usually begin with a .
. You might be familiar with .gitconfig
files or .gemrc
files. These are considered dotfiles, and knowing where to find them and how to work with them can be a little overwhelming, so developers like Mathias Bynens and Adam Eivy have created systems and boilerplates to help you out.
The short answer here is that dotfiles are where you manage your local development environment. So you might keep all of your ENV variables (always kept safe right?) in a .mischiefmanaged
dotfile. Regardless it pays to have some shortcuts to cut the cruft.
Some common shell shortcuts
If you are using atomantic/dotfiles some of these shortcuts will be familiar to you. But these are the ones I use the most:
One of my favorites however is my ngrok shortcuts. Once you get ngrok installed and setup your own custom domain, you can use this handy shortcut:
Now all we need to do to throw up a tunnel to my localhost:3000
at roomofrequirement.ngrok.io is run
grok 3000.
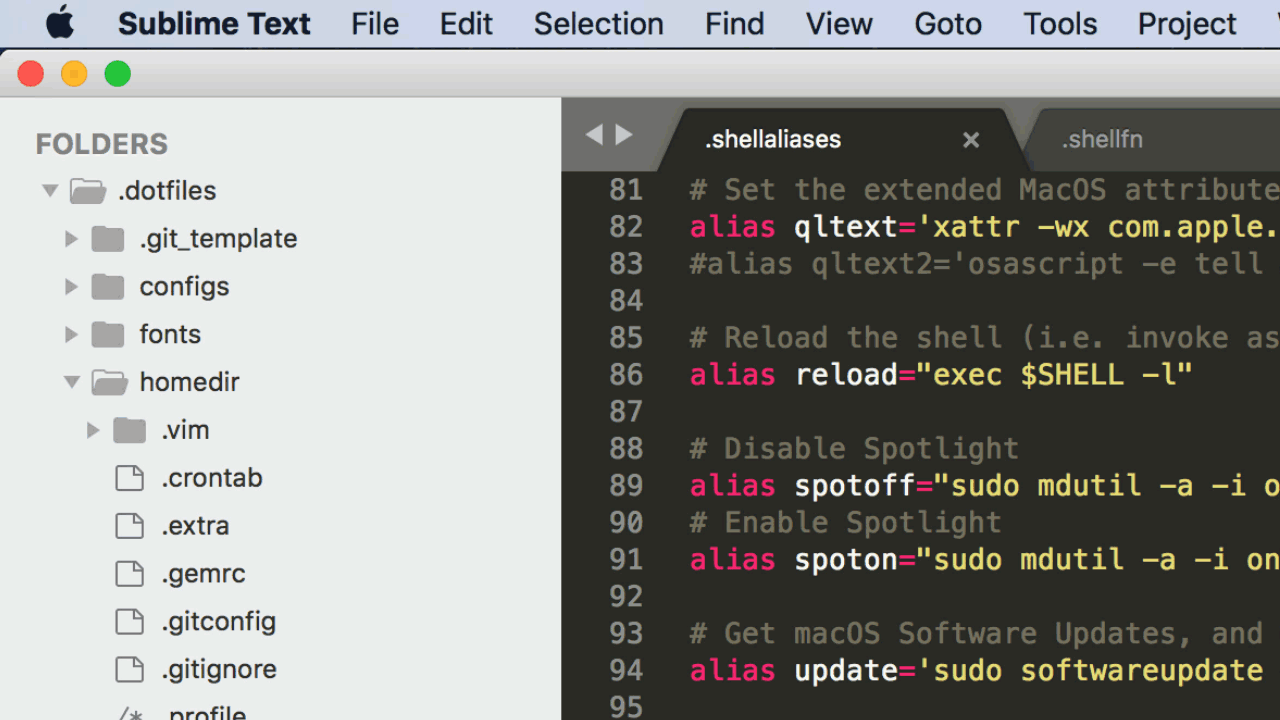
I also find myself working in node/Express apps often, and I have the default port set to 3000 for those apps. I also wrote a handy shortcut that starts up the node app and opens an ngrok tunnel to port 3000. If you want to do this you’ll need to install the tab() function by bobthecow on github.
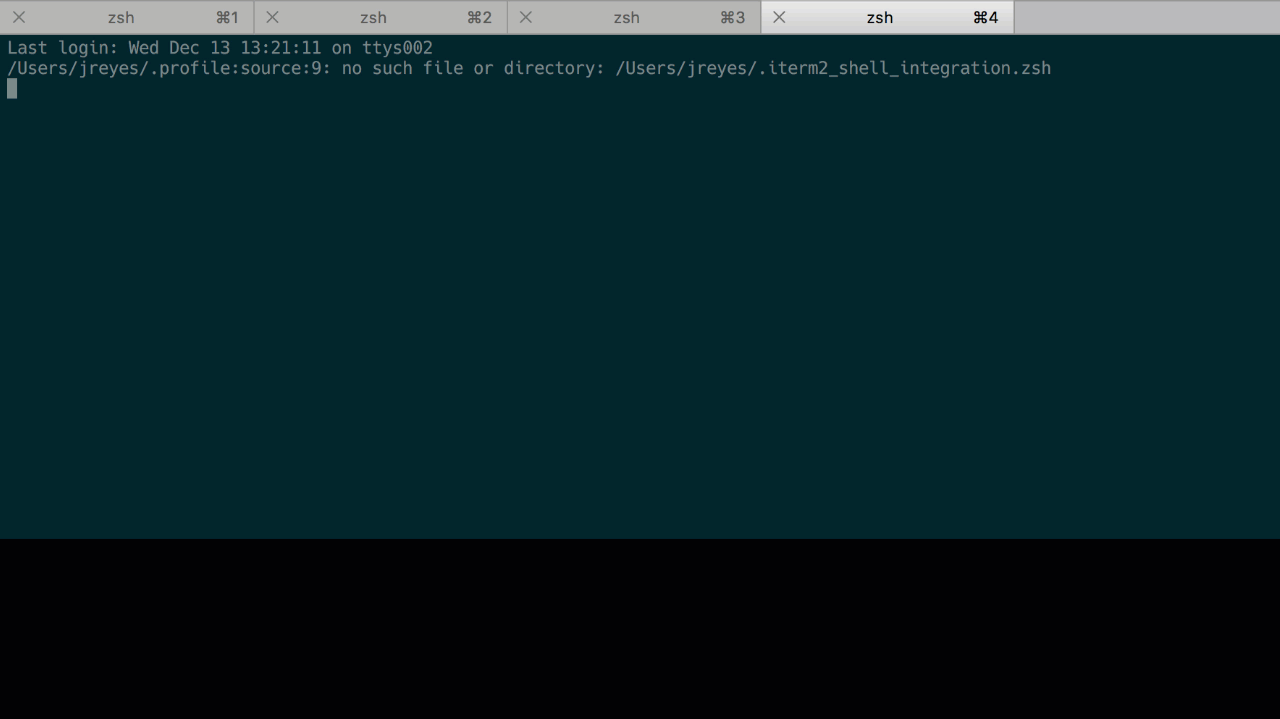
Git tricks Hermione would be proud of
A lot of developers spend a ton of their time gitting around their code, me included. You can learn more about git here. Here are some git shortcuts I use in order of frequency used:
And of course my favorite, from Kelley Robinson, a colleague:
If you’d like to use these just add them as is to your global .gitconfig file by running
git config —global —edit
Lastly, let’s take a look at some useful Twilio tools I have in my local-directory.
Useful Twilio scripts to run from CLI
So one of the most common use cases I have is looking up a phone number and getting country code. For this I use a handy little lookup script. This uses Twilio’s lookup feature which can grab basic information about any phone number in the world.
This next script I use a ton is one that quickly buys a random phone number from a country for hacking. Comes in very useful when I’m spending most of my day in code land :)
To wrap it all up, once I’ve bought a Twilio number, I copy the sid that was just entered to me, and I set up the url handlers for voice and sms with this little snippet.
I’d love to see some folks simplify some of these tasks even more into a rad little github repo. For now you can visit my github profile to see my twilio-functions and dotfiles repo. Remember to keep your .env variables safe, to never ever commit .env files to a publicly accessible repo, and to always git ca at the end of the day. Well that about does it for my tool-time update, feel free to ping me on twitter and share your favorite tools. Look forward to seeing what you $bash.
Jarod Reyes is in no way an expert on developer tools, nor has he ever been accepted to Hogwarts School of Witchcraft and Wizardry. However, you can reach him at any time, butter beer in hand, at jarod@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.