Send SMS and MMS Messages In C++
Time to read:
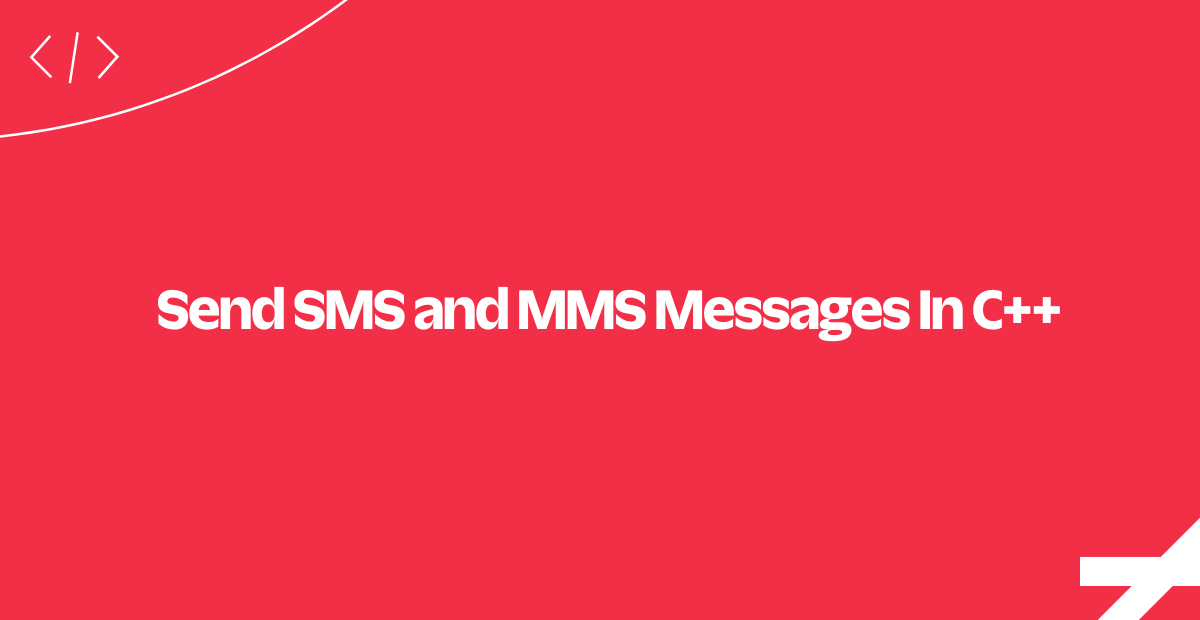
Today we'll use C++, some standard libraries, libcurl, and some POSIX APIs to send SMS and MMS messages using the Twilio REST API. You'll need a (mostly) POSIX-compliant build environment as well as libcurl to follow along with this guide.
Let's get started!
Sign Up for (or Sign In to) a Twilio Account
You'll need to login to your existing Twilio account, or create a new account.
Don't have an account yet? Sign up for a free Twilio trial, post-haste.
Purchase or Find a SMS-Capable Number
You'll need a SMS (or optionally, MMS) capable number to complete this demo. Either use a phone number that you already have, or purchase a new one with SMSes enabled.
From the Twilio Console, here's where you can determine the capabilities of your purchased number:
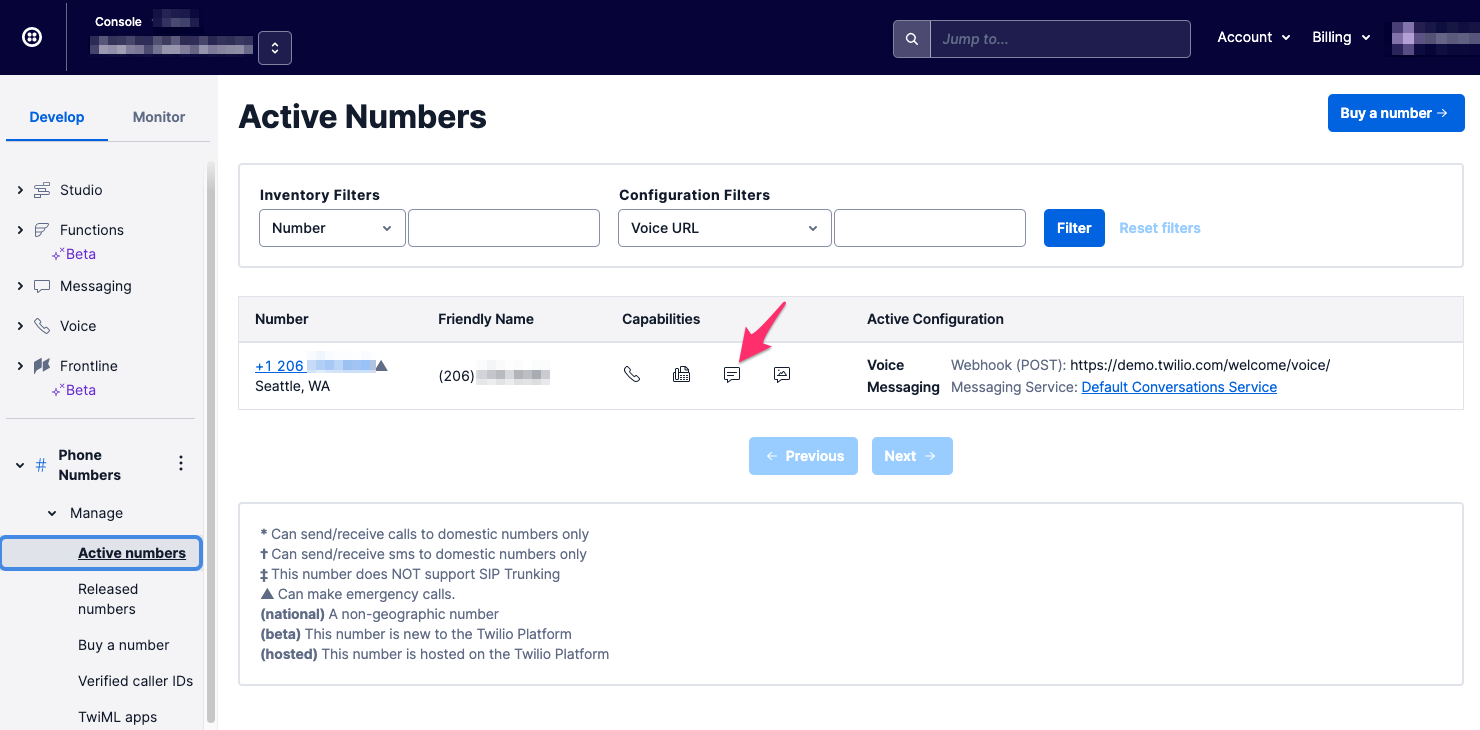
If you need to purchase a number, after navigating to the 'Buy a Number' link click the SMS checkbox (and the MMS checkbox, if you wish to send media messages).
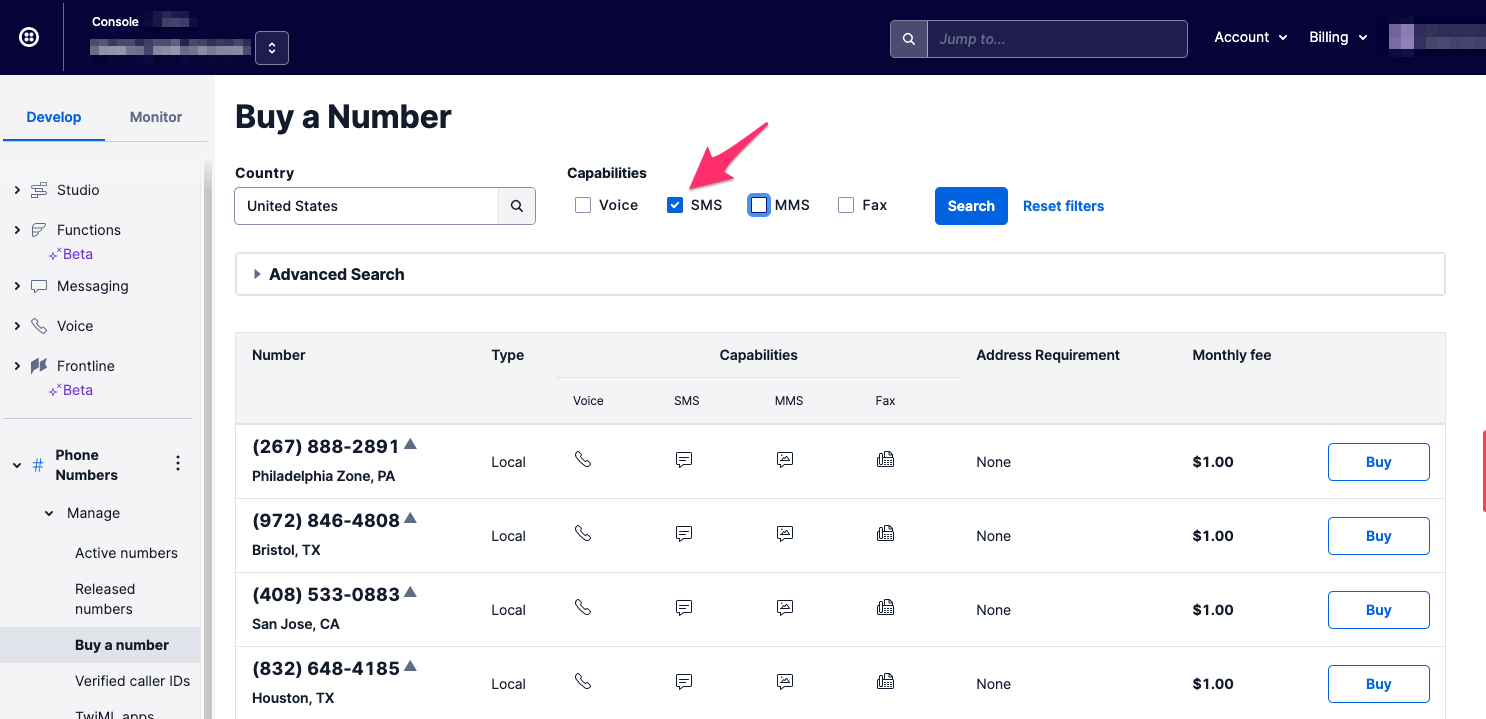
Prerequisites for This C++ MMS and SMS Guide
We're targeting POSIX Compliant environments with this guide, and tested our code with clang-800.0.42.1 on Mac OSX 10.11, gcc version 4.9.2 on Raspbian 8.0 (kernel 4.4.38-v7+) and gcc 5.4.0 in Cygwin 2.877 on Windows 7 64-Bit.
To build, you will need to ensure you have:
- stdio.h, stdlib.h, unistd.h - C POSIX libraries
- libcurl - Client-side transfer library
- iostream, sstream, string - C++ Standard Libraries
libcurl was already on the Mac. On the Raspberry Pi, you can install a suitable version with:
sudo apt-get install libcurl4-openssl-dev
In Windows 7 and Cygwin, you can install libcurl with Cygwin Setup:
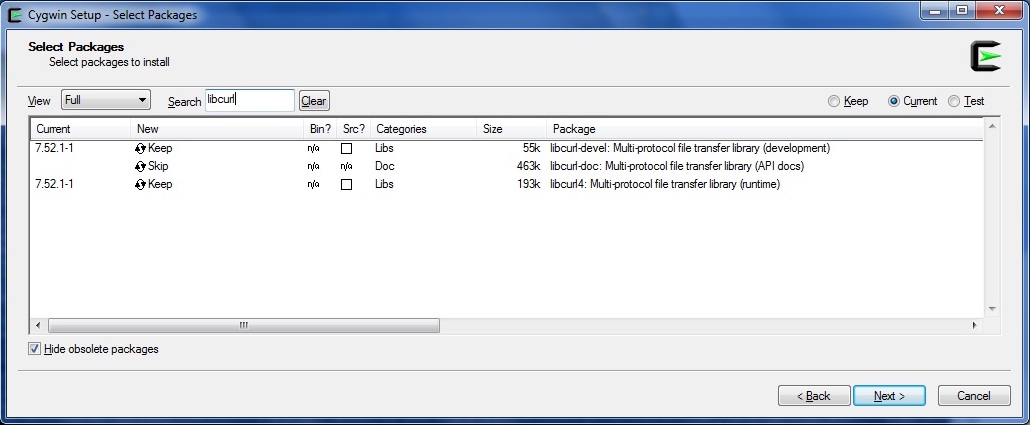
Building cpp_demo
All three environments worked with this example Makefile. You may need to make minor edits - usually to the library search paths - to build in your environment.
On almost all *NIX-type systems (including Windows/Cygwin), building should be very similar to the following lines (you might even be able to copy, paste and execute):
git clone https://github.com/TwilioDevEd/twilio_cpp_demo.git
cd twilio_cpp_demo
make
Send an SMS or MMS Message with C++
We've just built cpp_demo, which attempts to send an SMS or MMS based on your inputs from the command line. src/twilio.cc
and include/twilio.hh
demonstrate a class, Twilio, which should be easy to integrate into your own codebase. (Note that before putting it into production, you need to add input validation and safety. The first order of concern: the code in its current form can be passed additional HTTP parameters through the command line inputs.)
For C++ developers, our C SMS and MMS guide might give some key insights into the following code - but let's take a closer look to see what's happening under the hood.
- First, we instantiate a
Twilio
object with our account credentials (find them on the Twilio Console). - Second, we call the method
send_message
with the to/from numbers, the message, and optionally a URL to a picture and a boolean of whether we want verbose output.
- Third, once inside the code we first do some exception checking (making sure the message is the proper size), followed by setting up libcurl with our eventual HTTP POST
- Fourth, curl makes the HTTP POST and we clean up with
curl_easy_cleanup
. - Finally, we check the response for success or failure, and return the result to the calling function.
Running cpp_demo
Running cpp_demo from the command line is easy once you have it built. Merely set your account credentials and call the code as follows:
account_sid=ACXXXXXXXXXXXXXXXXXXXXXX
auth_token=your_auth_token
bin/cpp_demo -a $account_sid -s $auth_token -t "+18005551212" -f "+18005551213" -m "Hello, World!"
# Optionally, use '-p http://some/path/to/img.jpg' to send an MMS.
Getting C-Rious With Twilio and C++
After this demo, you now have the means to add communications to your new or existing C++ applications. Add monitoring or help to your C++ application, or bring your own use case.
Whether you're a C++ pro or closer to a practitioner of "C with classes" please do let us know on Twitter when you've got something built!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.