SMS and MMS Marketing Notifications with Node.js and Express
Time to read: 7 minutes
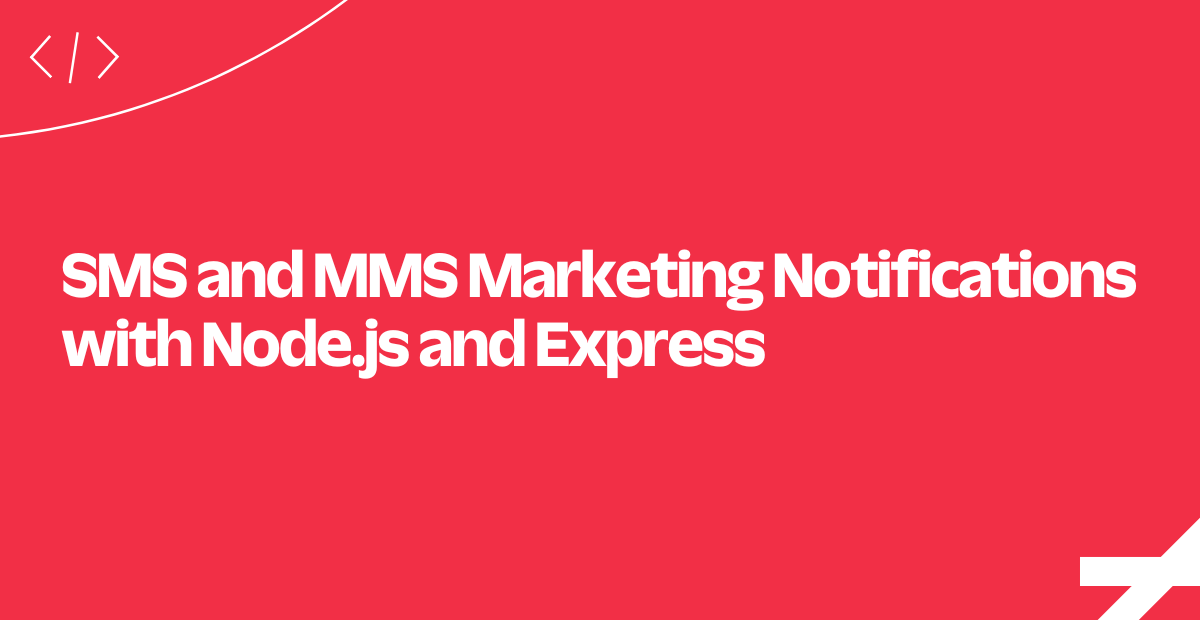
Ready to implement SMS and MMS marketing notifications? Today we'll look at adding them to your Node.js and Express application.
Here's how it will work at a high level:
- A possible customer sends an SMS to a Twilio phone number you advertise somewhere.
- Your application confirms that the user wants to opt into SMS and MMS notifications from your company.
- An administrator or marketing campaign manager crafts a message that will go out to all subscribers via SMS or MMS message.
Building Blocks
To get this done, you'll be working with the following tools:
- TwiML and the <Message> Verb: We'll use TwiML to manage interactions initiated by the user via SMS.
- Messages Resource: We will use the REST API to broadcast messages out to all subscribers.
Let's get started!
Subscriber Model
In order to track our subscribers, we need to start at the beginning and provide the right model.
To facilitate, we will need to implement a couple of things:
- a model object to save information about a
Subscriber
- an Express web application that can respond to Twilio webhook requests when our number gets an incoming text.
Let's start by looking at the model for a Subscriber
.
Creating Subscribers
We begin by adding a Mongoose model that will store information about a subscriber in a MongoDB database.
For our purposes we don't need to store very much information about the subscriber - just their phone number (so we can send them updates) and a boolean flag indicating whether or not they are opted-in to receive updates.
Now that we have a model object to save a subscriber, let's move up to the controller level.
Creating a Route for a Twilio Webhook
In an Express web application, we can configure JavaScript functions to handle incoming HTTP requests. When Twilio receives an incoming message, it will send an HTTP POST request to one of the routes we set up.
In this code, we configure all the routes
our application will handle, and map those routes to controller
functions.
And how do we tell Twilio to call a route on an incoming event? We'll look at that logic, next.
Mapping the Webhook to a Route
Click on one of your Twilio numbers on the Manage Phone Numbers screen in the account portal. For this number, you will need to configure a public server address for your application, as well as a route which Twilio will POST
to when your number gets any incoming messages:
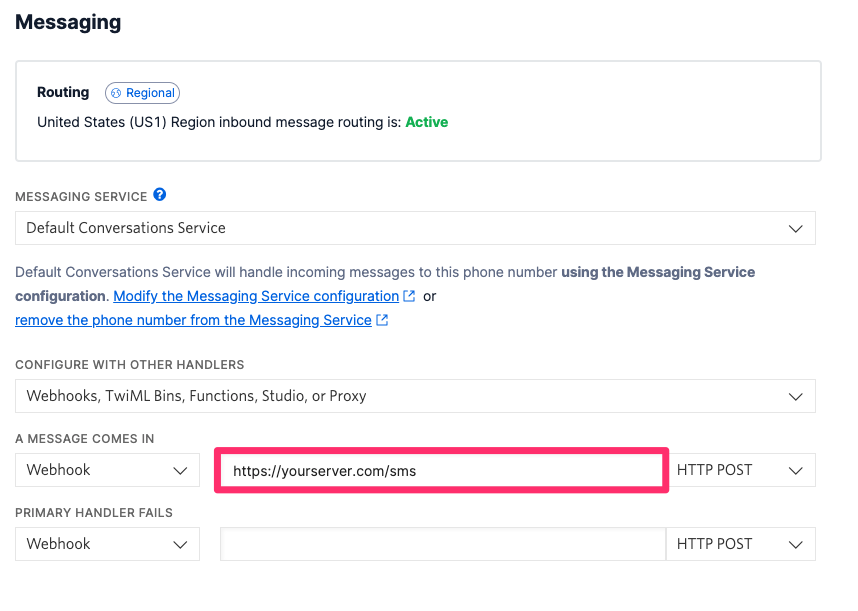
The route has to be publicly exposed. In the above screenshot you can see a route exposed by ngrok. Our colleague Kevin has written about testing with ngrok in the past.
Let's dive into the controller function that will handle incoming message logic next.
Handling An Incoming Message
Since the webhook
function will be called every time our application receives a message, it will eventually need quite a bit of business logic. We'll look at how this function works piece by piece as the tutorial continues, but let's focus on the first message the user sends for now.
Creating a New Subscriber
We begin by getting the user's phone number from the incoming Twilio request. Now, we need to find a Subscriber
with a matching phone number (a phone number is a unique property on the Subscriber
).
If there's no subscriber with this phone number, we create one, save it, and respond with a friendly message asking them to text "subscribe". We are very careful to have potential customers confirm that they would like to receive marketing messages from us.
And that's all we want at this step! We've created a Subscriber
model to keep track of the people that want our messages, and shown how to add people to the database when they text us for the first time.
Next, let's look at the logic we need to put in place to allow them to manage their subscription status.
Managing User Subscriptions
We want to provide the user with two SMS commands to manage their subscription status: subscribe
and unsubscribe
.
These commands will toggle a boolean on a Subscriber
record in the database, and only opted-in customers will receive our marketing messages. Because we want to respect our users' preferences, we don't opt them in automatically - rather, we have them confirm that they want to receive messages from us first.
To make this happen, we will need to update the controller logic which handles the incoming text message to do a couple things:
- If the user is already in the database, parse the message they sent to see if it's a command we recognize
- If it is a
subscribe
orunsubscribe
command, update their subscription status in the database - If it is a command we don't recognize, send them a message explaining available commands
Let's go back into our controller function to see how this works.
Process an Incoming Message
This internal function handles parsing the incoming message from the user and executing conditional logic to see if they have issued us a command we recognize. It's executed after we have already hit the database once to retrieve the current Subscriber
model.
Next: how to handle user commands.
Handling a Subscription Command
If the user has texted subscribe
or unsubscribe
, we will update their subscription status in the database. We will then respond to them via SMS with the opposite command to either opt in to updates or opt out.
List Available Commands
If a user texted in something we don't recognize, we helpfully respond with a listing of all known commands.
You could take this further and implement "help" text for each command you offer in your application, but in this simple use case the commands should be self-explanatory.
Now we'll show how to return a response to Twilio in a form it expects.
Responding with TwiML
This respond
helper function handles generating a TwiML (XML) response using a Jade template.
The TwiML response is reasonably simple, but for the sake of completeness let's peek in to see what it does.
Responding with TwiML: Jade Template
Jade is a popular template engine for Node.js that can be used to generate HTML or XML markup in a web application. We use Jade here to generate a TwiML Response
containing a Message
tag with the text we defined in the controller.
This will command Twilio to respond with a text message to the incoming message we just parsed.
That's it for the user-facing commands! Now, we need to provide our marketing team with an interface to send messages out to all subscribers. Let's take a look at that next.
Sending SMS or MMS Notifications
Now that we have a list of subscribers for our awesome SMS and MMS content, we need to provide our marketing team some kind of interface to send out messages.
To make this happen, we will need to update our application to do a few things:
- Create a route to render a web form that an administrator canuse to craft the campaign
- Create a controller function to handle the form's submissions
- Use the Twilio API to send out messages to all current subscribers
Let's begin at the front end with the web form our administrators will interact with.
Creating the Web Form
Here we use Jade to render an HTML document containing a web form to be used by our marketing campaign administrators.
It just has a couple of fields - one to specify a text message, and another to specify an optional URL to an image on the public Internet that we could send via MMS.
Let's go to the controller next to see what happens when the form is submitted.
Handling the Form's Submission
On the server, we grab the message text and image URL from the POST body, and use a function on our Subscriber
model to send text messages to all current subscribers.
When the messages are on their way, we redirect back to the same web form with a flash message containing feedback about the messaging attempt.
Let's jump into the model now to see how these messages are sent out.
Configuring a Twilio REST Client
When the model object is loaded, it creates a Twilio REST API client that can be used to send SMS and MMS messages. The client requires your Twilio account credentials (an account SID and auth token), which can be found in the Twilio Console:
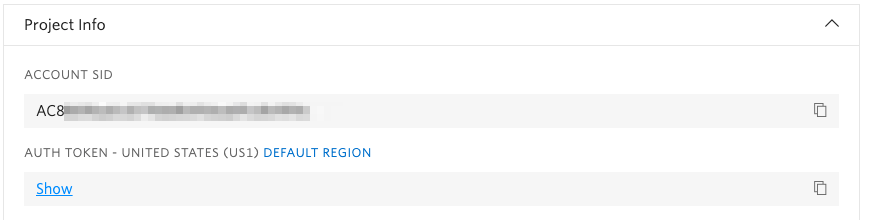
Let's check out the static function that sends the messages next.
Sending Messages from Node.js
Here we define a static function on the model which will query that database for all Subscribers
that have opted in to receive notifications.
Once the list of active subscribers has been found, we loop through and send each of them a message based on the parameters sent in from the controller. If there's no image URL associated with the message, we omit that field from the Twilio API request.
And with that, you've seen all the wiring necessary to add marketing notifications for your own use case! Next we'll look at some other features you might enjoy.
Where to Next?
That's it! We've just implemented a an opt-in process and an administrative interface to run an SMS and MMS marketing campaign. Now all you need is killer content to share with your users via text or MMS (you'll have to craft that on your own...).
Here's a couple of our favorite Node posts on adding features:
Automate the process of reaching out to your customers in advance of an upcoming appointment.
Convert your company web traffic into phone calls with the click of a button.
Did this help?
Thanks for checking this tutorial out! Whatever you're building and whatever you've built - we'd love to hear about it. Drop us a line on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.