An easy way to receive an SMS message with C# and Twilio
Time to read:
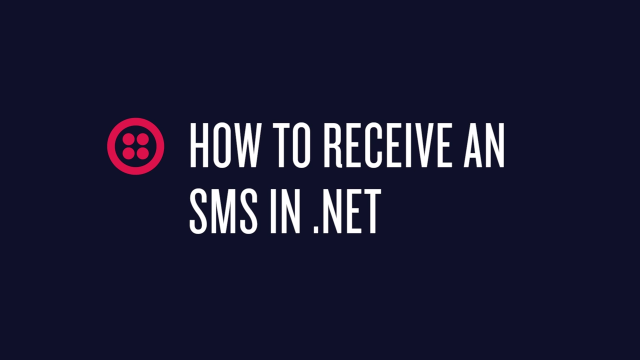
I already showed you how to send an SMS message with C# in 30 seconds. Today I’m going to show you how to receive an SMS message with C# and Twilio. Here’s all the code you’ll need.
If you’d like to see an explanation of how the code above works, watch this short video, or just keep reading.
When someone texts your Twilio number, Twilio makes an HTTP request to your app with details about the SMS passed in the request body. Let’s use use .NET MVC to handle Twilio’s request and send a response.
Let’s make sure we have the necessary libraries in our project by opening up the Package Manager Console in Visual Studio and installing the following Twilio .NET libraries.
With all our dependencies installed we need to make sure our Controller extends TwilioController
as this will make things easier when returning TwiML later.
We then create an endpoint called message
to accept a POST request with a From
telephone number and a message Body
.
When Twilio makes an HTTP request to your app, it expects a response in the form of TwiML, a simple set of XML tags that relay instructions back to Twilio. We will use the libraries we installed to build the response easily.
Run the project by clicking the Start
button with the green arrow at the top, and you will notice your browser opens up automatically. Make a note of the port the application is running on.
This app needs a publicly accessible URL, so you’ll either need to deploy it to the cloud or use a tool like ngrok to open a tunnel to your local development environment from your command line. We already wrote a blog post showing how to get started with ngrok.
Ngrok will provide you with a url that points to the application running on your localhost
.
Now that we’ve built our application we need to connect it up to a Twilio number so that it can receive SMS messages and make requests to our app.
Setup Twilio
Sign up for a free Twilio account if you don’t have one.
Buy a phone number, then click Setup Number. Scroll to the Messaging section and find the line that says “A Message Comes In.”
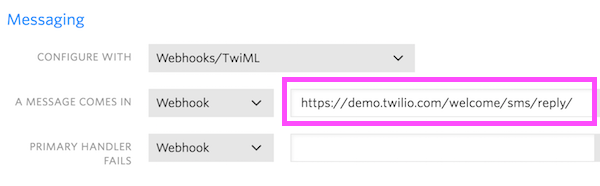
Fill in the full path to your endpoint (i.e., https://yourserver.com/sms/message) and click Save.
Now, send an SMS to your shiny new phone number number and get stoked when you receive the customized response you just wrote.
Next Steps
If you’d like to learn more about how to use Twilio and .NET together, check out :
And if you’d like to chat more about it, hit me up on Twitter @marcos_placona or by email on marcos@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.