How to Create an SMS Weather Forecast App using PHP & Twilio
Time to read: 3 minutes
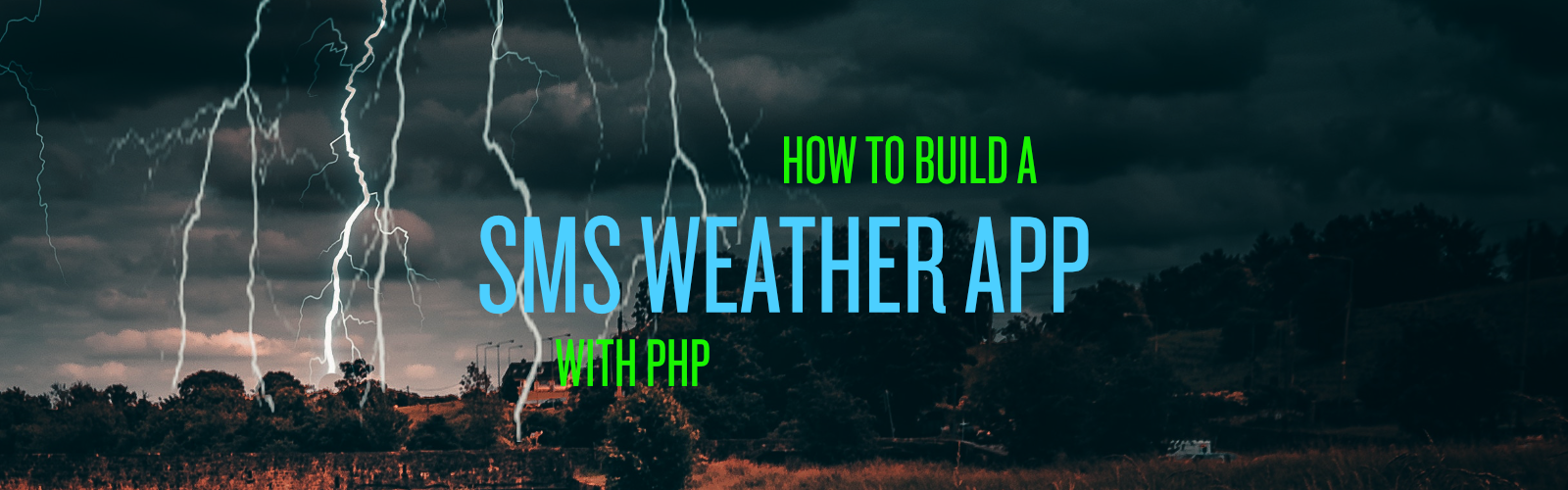
Imagine you’re on vacation and have limited or no access to the internet. Maybe you would like to go surfing or skiing and your access to a forecast is non-existent. This type of scenario is a very good use case of forecasting weather using SMS and a server-side language such as PHP. That's what we are going to learn how to build in this tutorial.
Technical Requirements
- PHP 7 development environment
- Global installation of Composer
- Twilio Account
- An OpenWeatherMap Account
- Global installation of ngrok
Set Up Our Development Environment
To kick start our project we will need to create a project directory for it. You may use Weather-App
as this is what I will be using too. In the folder create the following files.
- .env
- webhook.php
- functions.php
Next, we need to set up our .env file.
NOTE: The .env file is a hidden file we create on our servers to store secret and private keys it is not accessible by the browser we can store our API keys or credentials in it and access from anywhere in our app on the server side.
To allow the support of .env configs in our app we need to install a package using Composer. Run this command in your terminal in the project directory:
We also need to install one more package from Twilio. There are many libraries that enable us to access the Twilio API more efficiently than traditional cURL requests. For this project, we need to install the Twilio PHP SDK Run the following command in your terminal in the project directory.
Setup Twilio Credentials & Phone Number
If you have not already done so, take a few minutes and head to your Twilio account to create a new number. We need to get the following information from Twilio to allow is send and receive SMS from our project
- Twilio Account SID
- Twilio Auth Token
- A Phone Number
Once we have these items, we can add them to our .env
file.
Create a Function to get Weather Updates
To get weather data we need to use the OpenWeatherMaps API services which we will integrate later into our app for SMS forecasting. In your browser, head to the OpenWeatherMaps website to create an account after creating an account, click on the “API keys” tab to see your API keys in your dashboard.
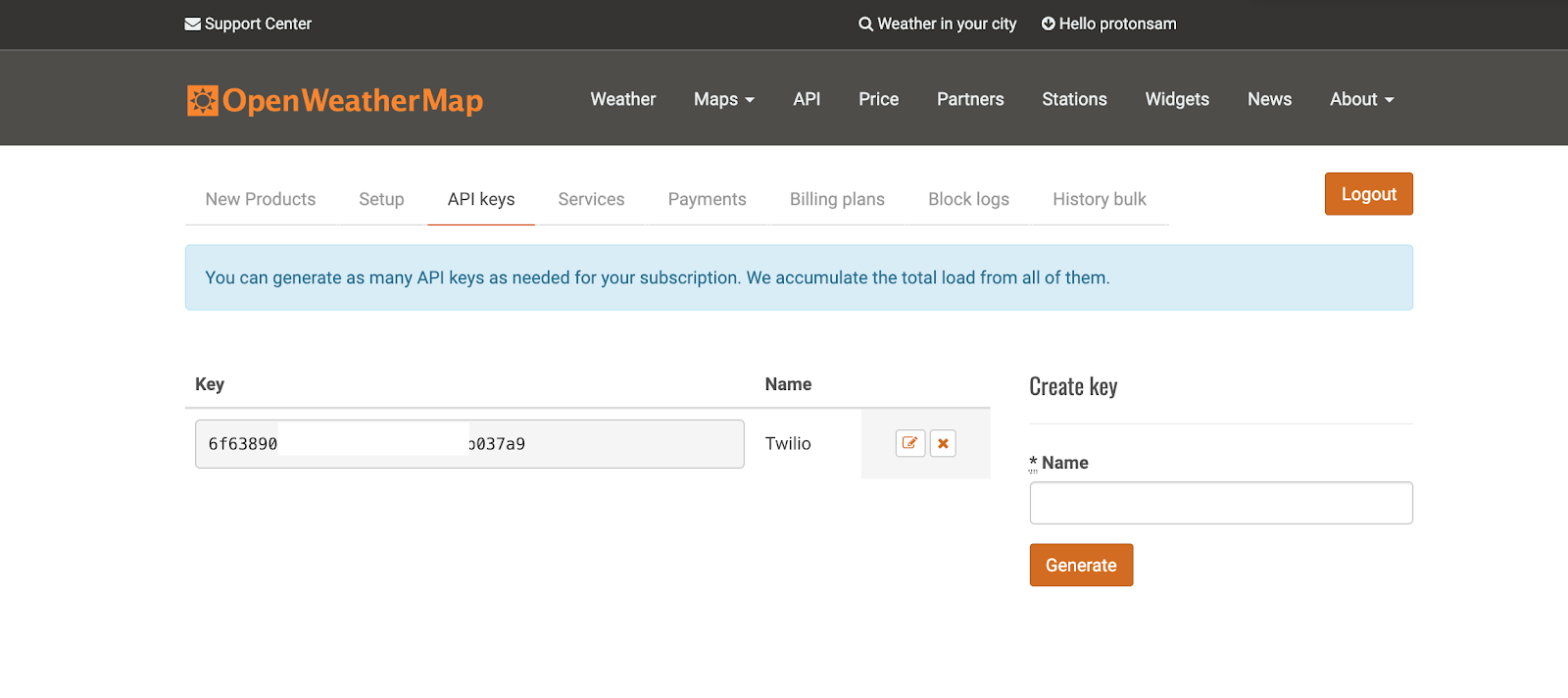
Copy the key you have generated or the default one, and put in your .env
file like so.
Now that we have generated our API Keys, let use them to connect to the OpenWeatherMap service. The functions.php
file we created earlier will contain the function that will take the user’s command, process it and send the location name to OpenWeatherMap’s API. Add the following code to the functions.php
file.
Create a Twilio Webhook
To receive SMS weather forecast requests from users we will need to create a webhook. Twilio has a webhook feature that sends a response to an endpoint whenever your phone number receives a message.
We will need to create our own webhook to receive forecasts from users. In the webhook.php
file, copy and paste the following code into it.
We will now use Ngrok in order to make this webhook accessible through a public URL from our localhost.
From the terminal, run the command:
On a new terminal window, run the command:
Your terminal window should look like this:
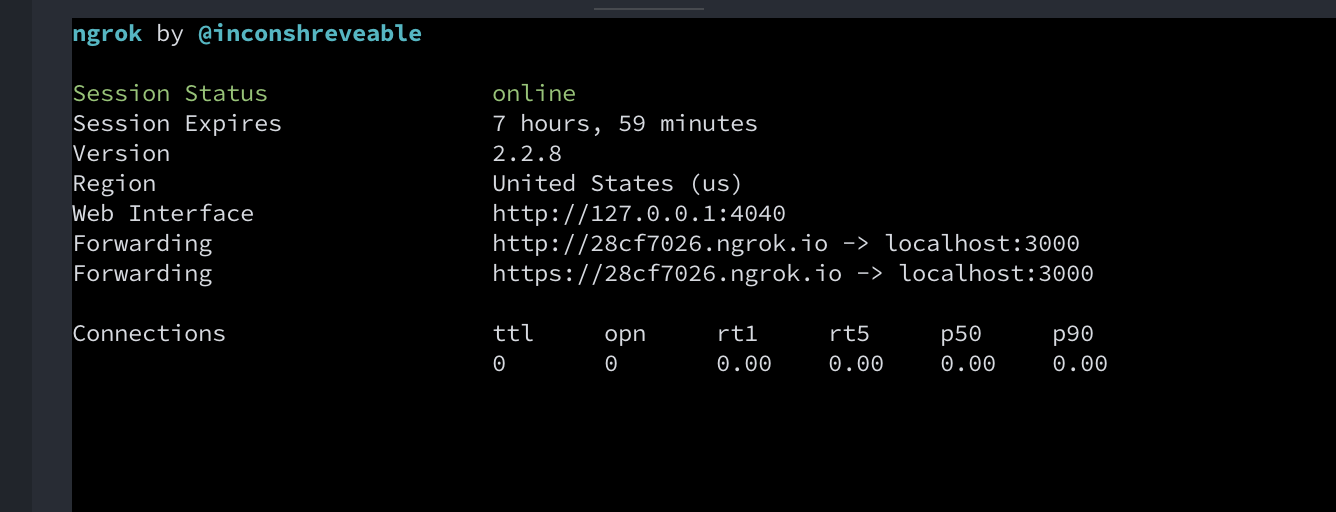
In our Twilio project, we will provide the webhook URL above, followed by the path to our webhook file. For example, mine is:
The final step is hooking our Twilio number to the webhook we created. Navigate to the active numbers on your dashboard and click on the number you would like to use. In the messaging section, where there is an input box labeled “A MESSAGE COMES IN”, replace the URL with our webhook URL and click “Save”!
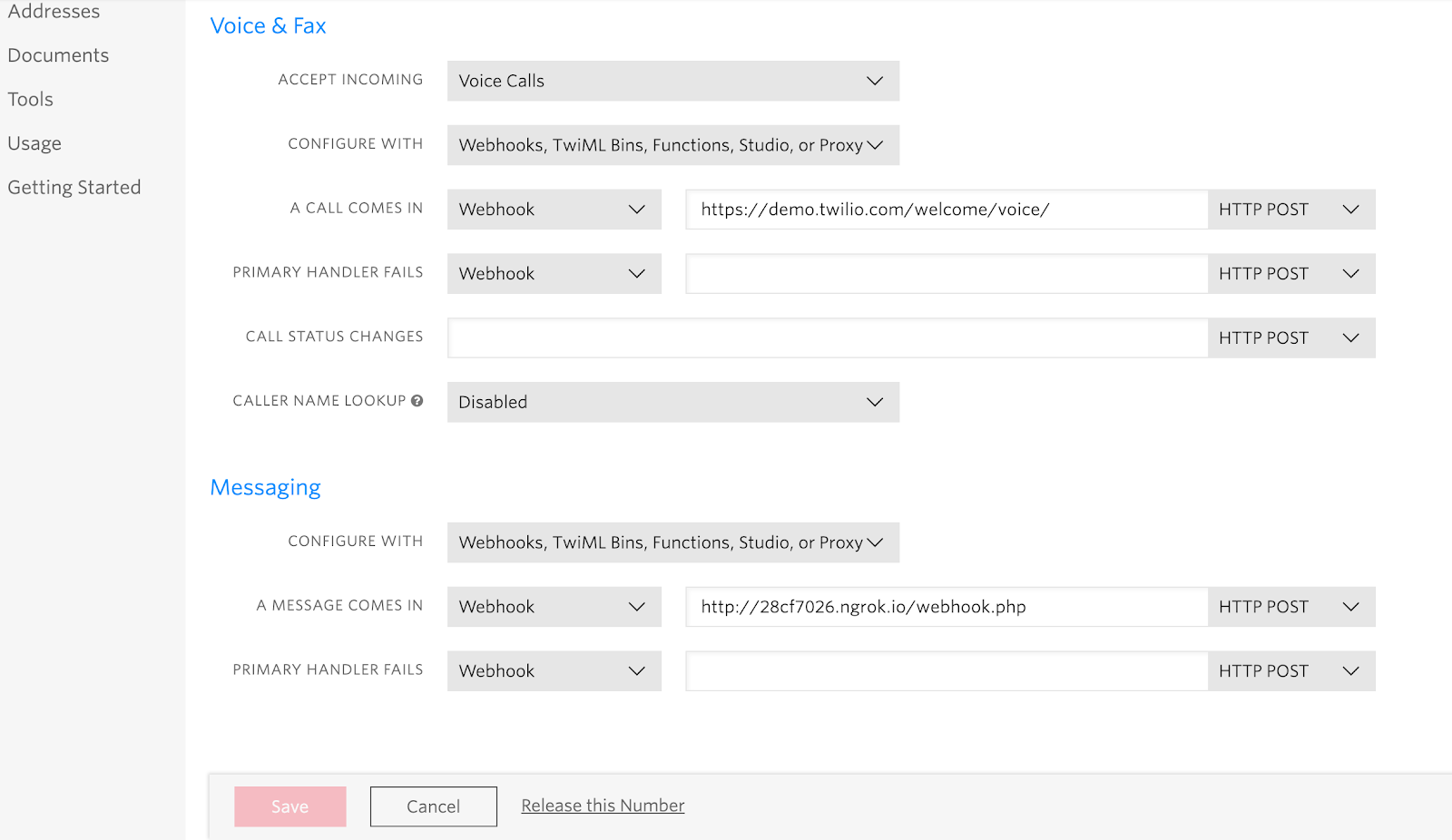
Testing Our App
Send WN-Lagos
to your TWILIO phone number to get the current weather status of Lagos City. You can try your own city by using WN-City Name
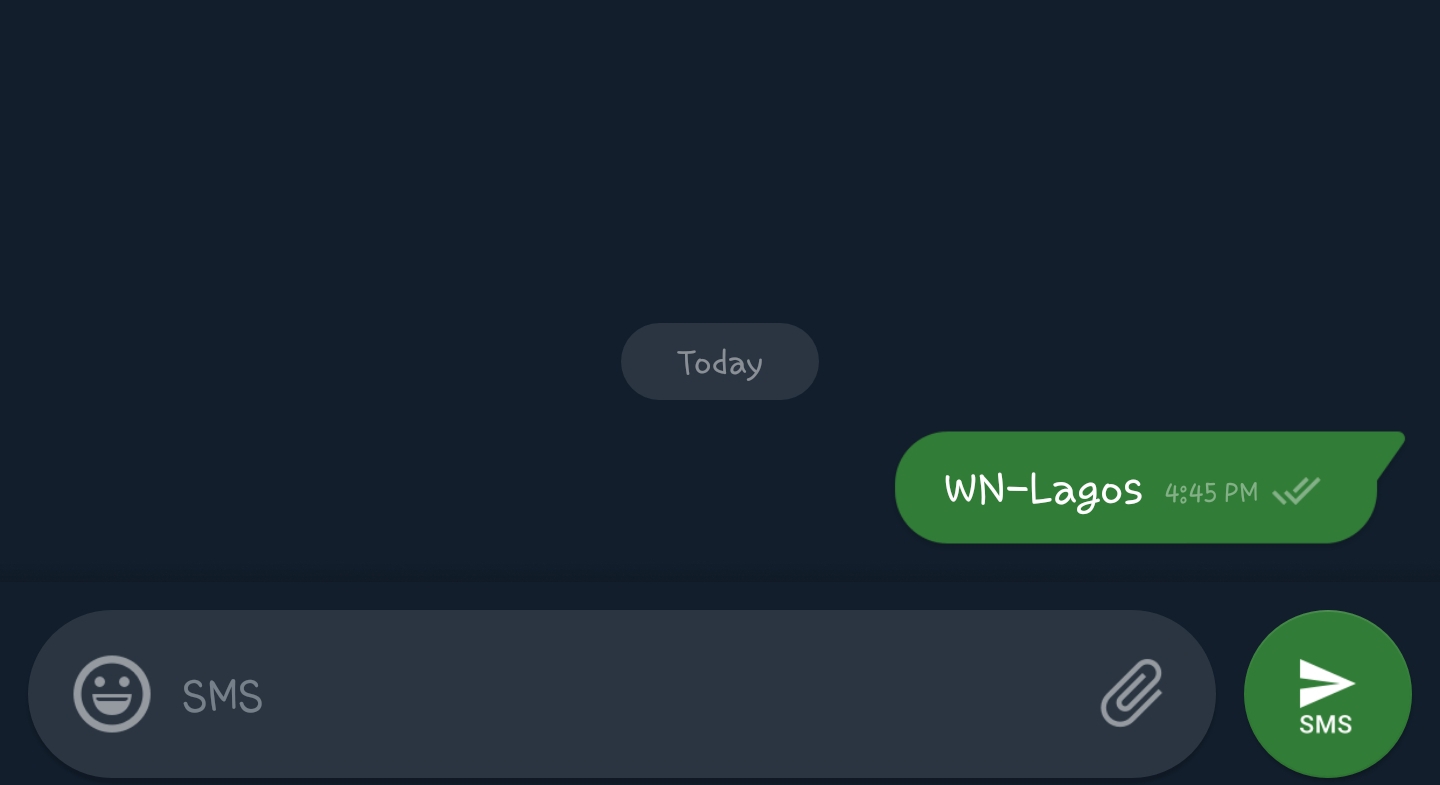
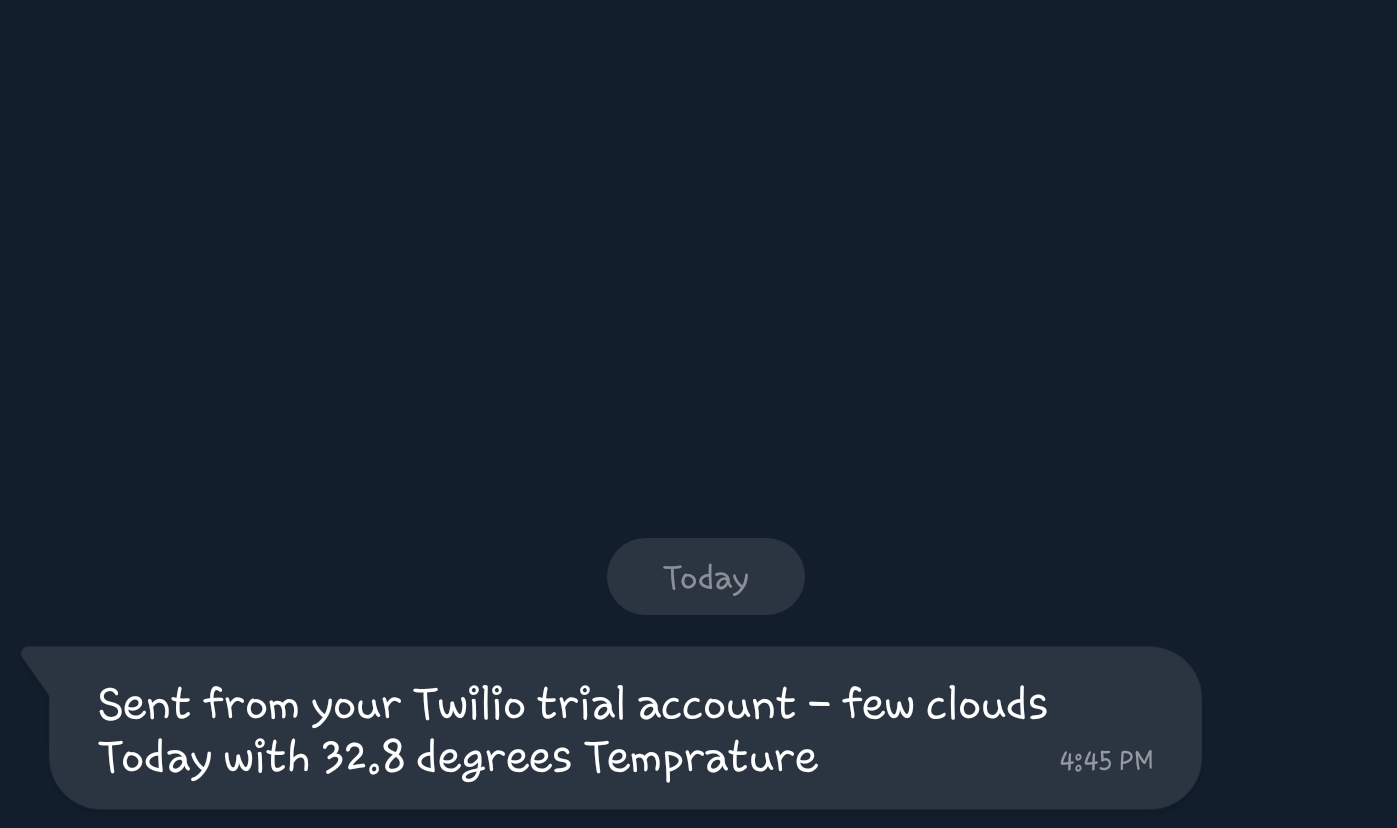
Conclusion
Good job! Now we can receive weather updates using SMS. You can implement other features like making users send more commands to get more weather data.
Bonus
Github Repo: https://github.com/ladaposamuel/sms-weather-php
- Email: ladaposamuel@gmail.com
- Github: ladaposamuel
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.