Creating a Smart Assistant using Twilio Voice, Amazon Alexa, and Laravel
Time to read:
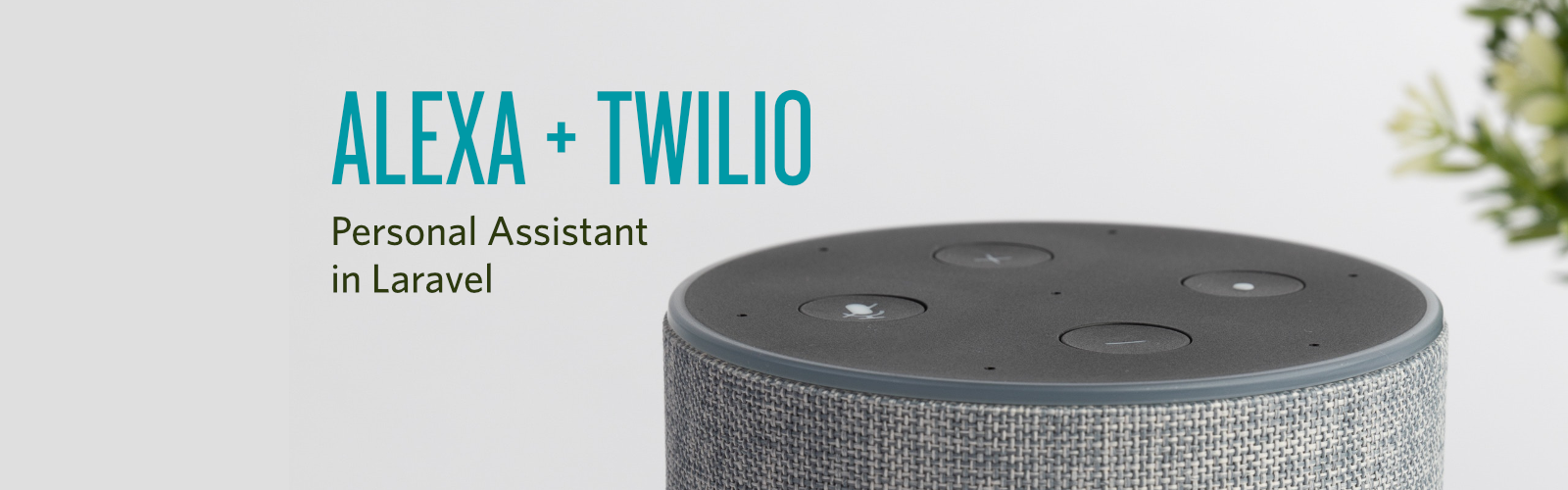
As a small business owner, I am always trying to increase my productivity and grow my business. Why have a meeting for what can be said in an email? Unfortunately, many of my clients don’t check their email regularly, so I have to call them. There’s no better way to decrease your productivity than a million simple phone calls every day. If you’re in the same boat as I am, Amazon Alexa + Twilio Voice is the perfect solution.
Technical Requirements
For this tutorial, we'll assume the following:
- You have PHP 7.2 installed
- You are familiar with Laravel
- You have Composer globally installed.
- You have an Amazon Developer account.
Let's get started!
Create a Laravel Project and Install the Twilio SDK
The first thing we need to do is create a fresh Laravel project. The simplest way to do this is by using Composer.
Once your project has been created cd
into the newly created alexa-twilio-voice
directory and install the Twilio PHP SDK.
Now that Twilio's fabulous PHP SDK has been installed, we need to set up your Twilio authentication credentials.
Set your Authentication Credentials
Open your project in a file browser and navigate to the root directory. Once in the directory, open .env
in your favorite text editor and add the following variables to it.
The values for your account SID and Auth Token come from the Twilio console:
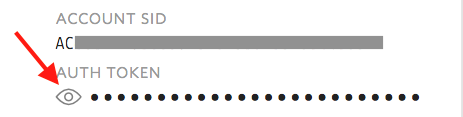
If you already have a Twilio Programmable Voice Number, you can add it to your environment file. If not you can create one on the Twilio Voice console:
Create Amazon Alexa Skill
Using the Amazon Developer portal is the quickest way to create an Alexa Skill. If you don't already have an Amazon Developer account, you can create one for free at developer.amazon.com. Once logged in navigate to the Alexa Console.
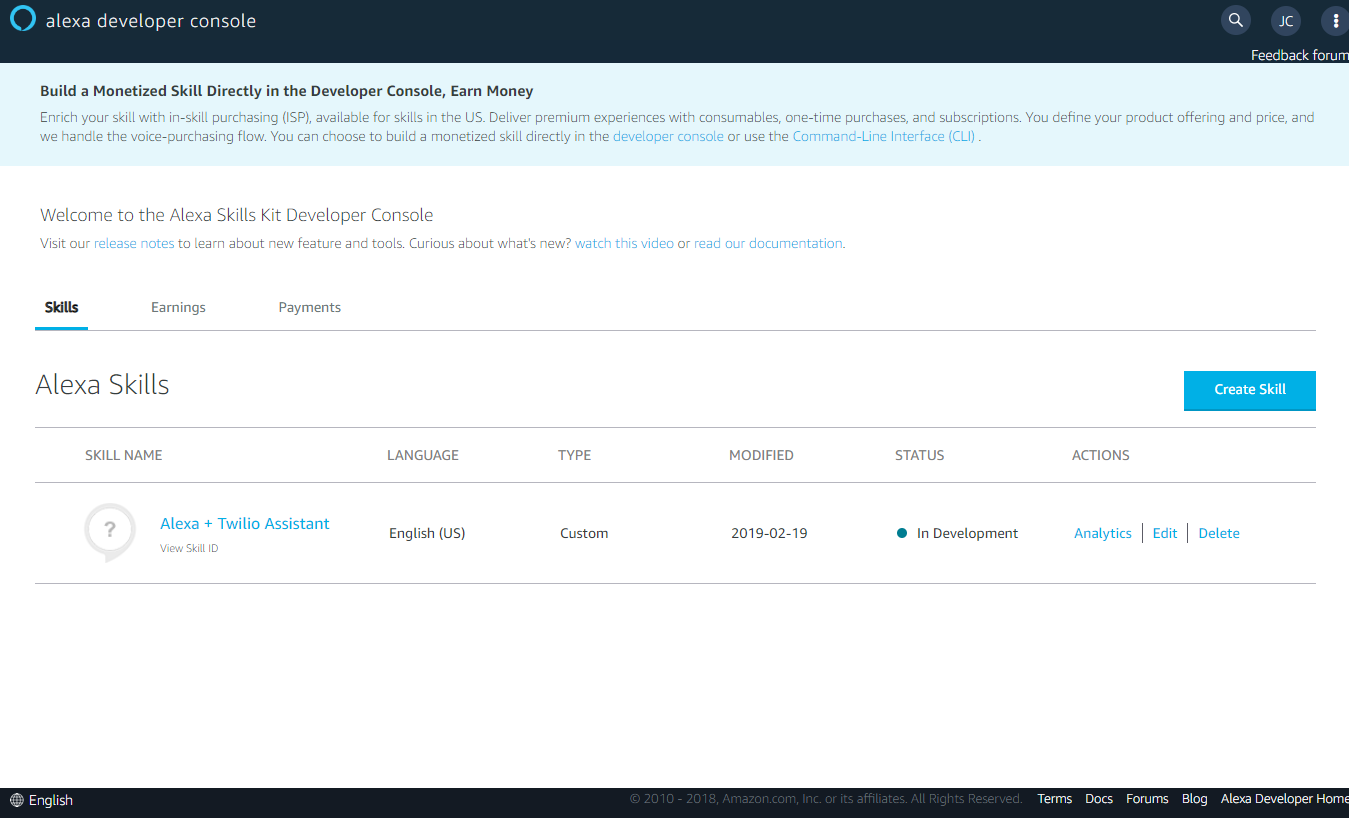
Click "Create Skill". We'll name this skill "Alexa + Twilio Assistant". Because our skill is going to be interactive, select the "Custom" skill model and "Provision your own” for backend resources and click "Create Skill." Now you should see a screen that looks similar to the image below.
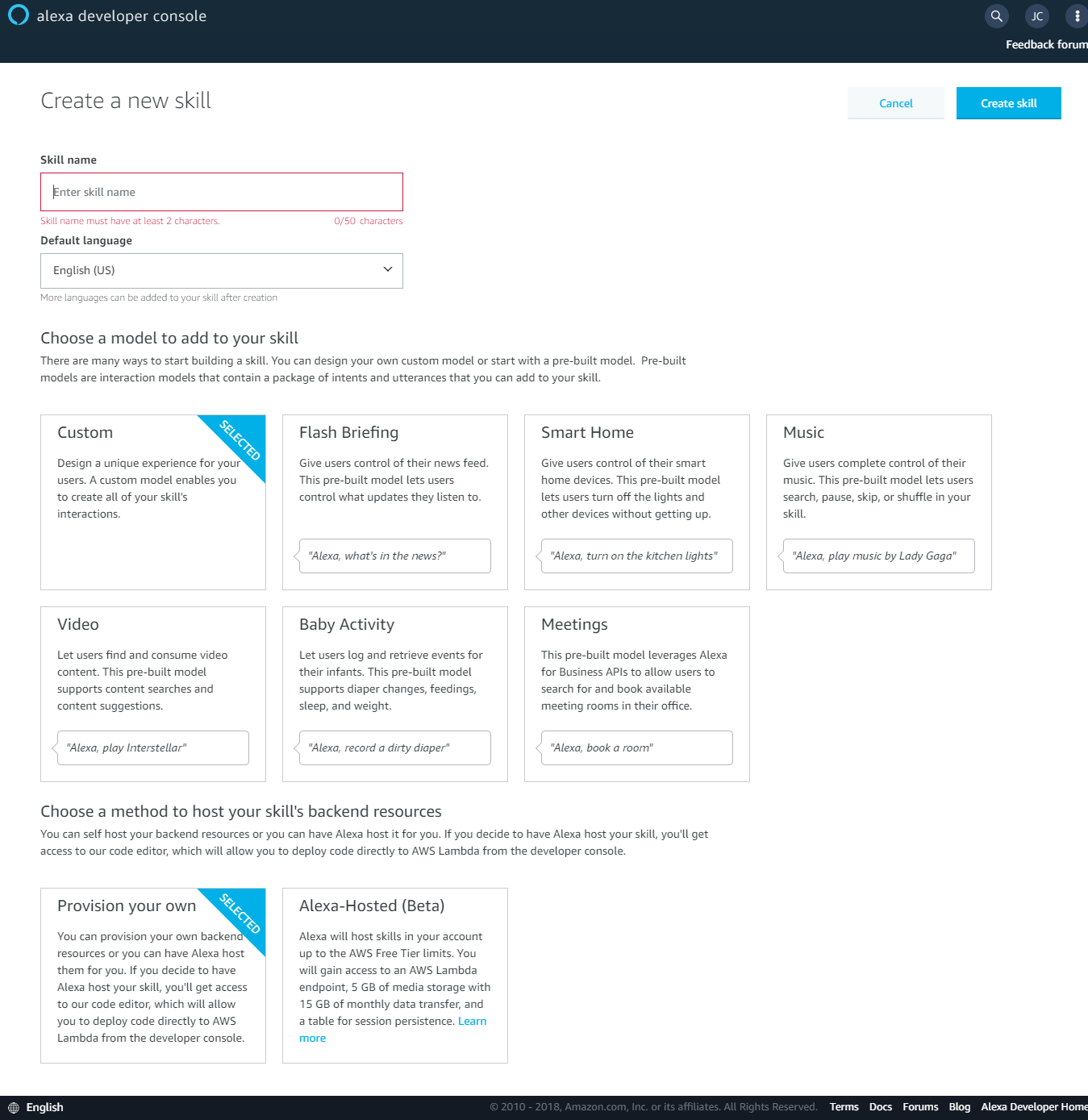
I've already created an Intent JSON that you can copy and paste into Alexa’s Intent Editor. Click on the “JSON Editor” link underneath the “Intents” menu to access it. Once you have pasted the JSON, click on “Save Model” to save your updates.
Building REST Endpoints for Alexa
Open your terminal again and cd into your projects directory. Using Laravel's Artisan commands create a new controller.
Navigate to the app/Http/Controllers
directory and you should see your controller IntentController.php
. Open it in your favorite IDE. Copy and paste the following code into IntentController.php
.
Let's look at our controller functions — the function processIntent
processes Intents from your Alexa Skill. It fetches the phone number from the SelectIntent
and the message from the MessageIntent
. Once the MessageIntent
has been called, it calls the Twilio API to create a call. Our other function, processCall
is our Twilio callback. It fetches the message from our cache and returns TwiML that Twilio Voice reads on our call.
Now we need to create our routes to direct requests to our IntentController
. Paste the following code into routes/web.php
.
Lastly we need to declare our routes exempt from Laravel’s CSRF Protection, this will allow Alexa to access our routes. Paste the following into app/Http/Middleware/VerifyCsrfToken.php
.
SSL Certificates
You can install your Laravel project on a web server. I suggest using Laravel Forge for one-click deployments. Unfortunately, Amazon doesn't recognize any CA approved SSL certificate providers other than their own. You'll have to generate a self-signed certificate, luckily Amazon has a guide for that here.
Because you're using a self-signed certificate, you'll need to disable SSL verification in your Twilio console. Navigate to your Twilio Project Settings. Scroll down to SSL Certificate Validation. Change the validation option from Enabled to Disabled and save your project settings.
Lastly let’s set up our endpoint on the Alexa Console. Within your skill, scroll down to Endpoint. Select "HTTPS" as your endpoint type. Upload your certificate we generated in the previous step and set the default region to https://your.url.here/alexa/intent
. Then save your endpoints and build your skill.
Testing
Connect your Alexa powered device to your Amazon Developer account. Below is a sample conversation with our skill.
- User: Alexa, open personal assistant
- Alexa: Welcome to the Twilio Alexa assistant
- User: I’d like to send a voice message to +11111111111
- Alexa: What message would you like to say?
- User: Say Hey jake are we still on for lunch tomorrow?
- Alexa: Sending "hey jake are we still on for lunch tomorrow" to +11111111111
Conclusion
Now that you've completed this tutorial you know how to:
- Make calls using the Twilio PHP SDK
- Create Amazon Alexa Skill Intents
- Integrate Twilio Voice with a Smart Assistant
Next, you should try building custom interactions into your Twilio Voice assistant.
You can find me on Github, Twitter, or reach me via email at jake@lynndigital.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.