Detecting Robocalls in Java with Twilio Lookup and Nomorobo
Time to read: 3 minutes
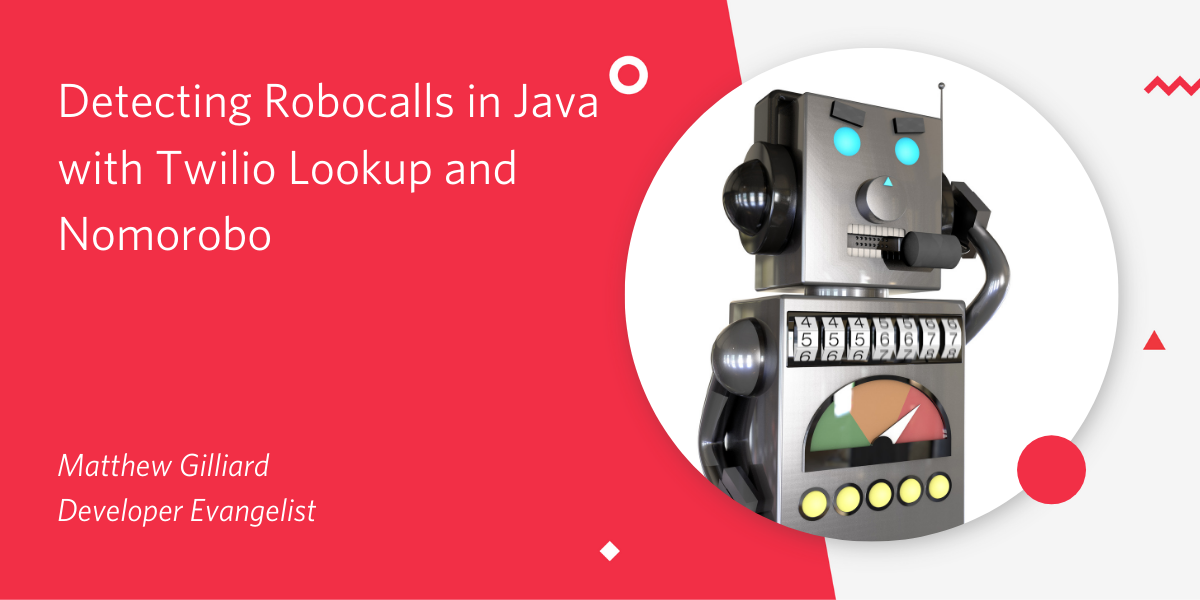
Twilio's CEO Jeff Lawson recently wrote about the history of robocalls and what we're doing to eliminate them.
Until that happens, we can build a tool in Java that will help us identify an unwanted call using the Twilio Lookup API with the Nomorobo Spam Score Add-on.
Set Up
In order to create this project you’ll need
Add the Nomorobo Add-on to your Twilio Account
Head to the Twilio Console and install the Nomorobo add-on. Look for the yellow logo and click through to "Install".
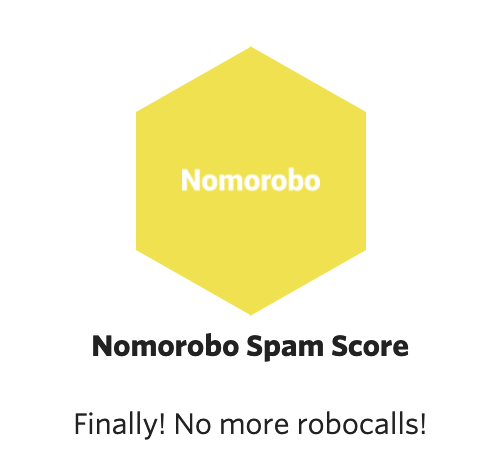
Leave the name as nomorobo_spamscore
and "Save" the Add-On.
Setting Up your Java Project
Create a new Maven project with the java8-quickstart-archetype:
This command will prompt for a groupId
and artifactId
. If you’re not sure what those are then check the Maven naming guide - I used lol.gilliard
and twilio-lookup-nomorobo
. You can accept the defaults for version and package. The project will be created in a subdirectory with the same name as the artifactId. Open the project in your favourite IDE.
In the pom.xml
file there will be an empty <dependencies>
section - we’ll be using the Twilio Java Helper Library, so add it here:
Next you’ll need to create a class to hold your code. Inside the src/main/java
directory add a new class - I called mine LookupNomorobo
. Then add the following code:
To avoid hard-coding your Twilio account credentials, this code looks them up in a couple of environment variables. You can find the values you need on https://www.twilio.com/console:

To make these available to your code you will need to set the ACCOUNT_SID
and AUTH_TOKEN
environment variables. I do this using the IntelliJ EnvFile plugin, you can also set them in Eclipse or system-wide.
If you run this code you should see output like this:
The output will all be on one line - I have reformatted it for clarity here. If you get a response that says AddOn not found
double check you have installed the Nomorobo AddOn in the Console.
The key section is score=1
. This means that Nomorobo has detected that this number is most likely a robocaller.
Making your Code Reusable
The possible values for score
are 1
(likely a robocall) and 0
(likely not). You can write your code to be more reusable by creating a method which checks that and returns a boolean
.
In order to do that we need to recognize that add-ons are able to put small amounts of data in Twilio’s API responses, and that the exact format of that JSON can be different for each add-on. This makes it difficult to provide Java types in the Helper library which represent the data that the add-on has provided. So, we will use the lower-level TwilioRestClient
API to be able to access the JSON response directly, and then use JsonPath to read the value out of the JSON.
First, add the JsonPath dependency to pom.xml
. The XML you need is this:
Then, change your source code to look like this:
The highlighted code uses JsonPath to retrieve the score
which is deeply nested in the JSON response.
If you run this code you should see true
as the output, and 🎉Ta da! Now we have a handy method we can use to identify robocalls.
What's next for using Lookup?
Maybe you want to filter calls in your call center or identify the line type (mobile vs. landline) of a phone number. With Lookup you can also prompt customers to add SMS (or voice!) based 2FA, clean up your database, or segment your users.
Have you built something that takes advantage of Twilio Lookup? Let me know in the comments or on Twitter @MaximumGilliard.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.