File Upload with Laravel and Google Cloud Storage
Time to read:
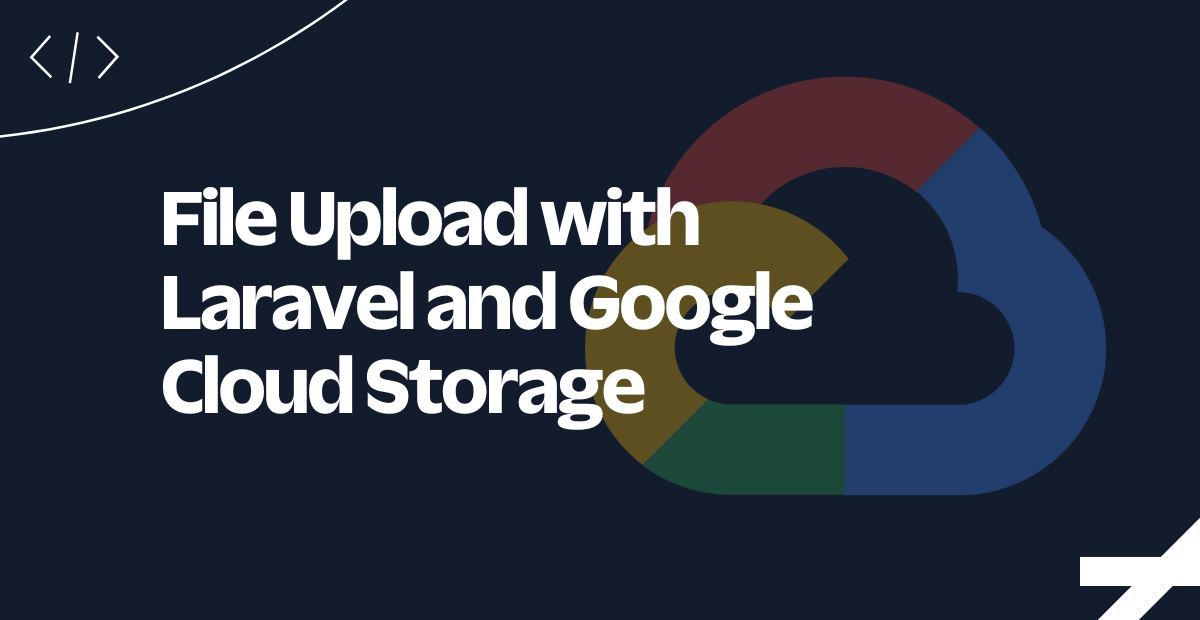
This tutorial will focus on three things:
- Integrating and using GCS in Laravel for file upload
- How users can access Google hardware to enhance their products and get secure databases
- The speed in response time cloud computing offers
We will begin by getting an introduction to Google Cloud Platform (GCP), and then expand into Google Cloud Storage (GCS) as a component of the platform.
What is Google Cloud Platform?
Google Cloud Platform (GCP) is a service provider built and maintained by Google for operating, maintaining, and deploying web applications. The services provided, such as a real-time database (firebase) and cloud storage, are essential for both Google's in-house teams and for external use by companies and developers to build better performant, resilient, and scalable applications.
Understanding GCP
On the GCP free tier, tons of tools can be accessed. These tools range from cloud computing to Google Kubernetes Engine, BigQuery, Pub/Sub system, machine learning tools, etc. We will be talking briefly about four of them.
Cloud Storage: Cloud storage stores and manages unstructured data on remote servers with options to backup on multiple data centres across the globe, get analytics on the data stored and queried, and also secure for active and cold data storage.
Pub/Sub system: This gives developers access to high availability, scalable pub/sub systems to allow easy sending and receiving of messages across multiple applications that are either hosted on Google or on other platforms. Furthermore, the filtering of messages based on attributes is included to reduce delivery volume.
Google Kubernetes Engine: Google gives access to a Kubernetes engine that puts containers on autopilot and manages the respective nodes.
FireStore: A NoSQL document-oriented database hosted on the cloud to store data then sync them across client platforms (web, mobile, etc) using a real-time listener. Firestore also syncs with other GCP services like cloud storage to read and write files into buckets.
What is Google Cloud Storage?
Google Cloud Storage is a cloud-based file storage system built on the Google Cloud Platform infrastructure. Files can be stored, read, encrypted, and deleted in a scalable manner. Google Cloud uses a “bucket” system to store a pile of files clustered together in a remote server with editable permissions for read and write access.
Why Cloud Storage is preferable
- Cost Efficient: A business can cut costs that would traditionally be incurred from buying and maintaining hardware. With cloud storage, applications storage consumption scales with the influx of usage on the platform hosted, and relieves companies of the need to set up hardware infrastructure, which is expensive.
- Collaboration: users and teams can share files and collaborate on files owned by other users. Changes to files shared by the owner are synced remotely to all users who have access.
- Security: To prioritise data security, data is encrypted before being written to a user’s designated storage. To control who has access to information and read/write data, Google also uses layers of authentication and IAM (identification and access management) systems.
Installation
We will create a small application to demonstrate how Laravel can use Google Cloud to create and fetch a file. First, we will set up a Laravel development environment and then set up Google Cloud Storage in the Laravel boilerplate.
Prerequisites
- Knowledge of PHP
- Composer installed globally
- A Google Cloud account with a Cloud Storage Bucket that has Fine-grained access control enabled
- Postman
- Basic knowledge of Google Cloud
- Prior knowledge of Laravel
- Some command-line experience
We will begin by scaffolding a Laravel application called laravel_gcs. To do that, open the terminal and run the command below:
Next, step into the created directory and start the application using the commands below.
Once the application is running, install the Google Cloud Storage package for Laravel, using the command below in a new terminal window or tab.
Configure GCS
Google Cloud uses service account keys, in the form of a JSON file, to store sensitive information about a user’s bucket. If you are unfamiliar with this, refer to this guide for clarification on how to retrieve a service account JSON file.
Once the service account JSON file has been obtained, move it to the root directory of the laravel_gcs project and name it laravel-gcs.json.
Next, open config/filesystems.php and add the gcs
disk configuration array below to the existing ones in the disks
array.
In the project's .env file, add the keys GOOGLE_CLOUD_PROJECT_ID
and GOOGLE_CLOUD_STORAGE_BUCKET
. These are required for connecting to, and reading and writing data from the storage bucket. These keys can be retrieved from the Google Cloud Console for the project.
Also, update the .env to include Google Cloud Storage as the default driver.
Create a controller
After the configuration parameters have been retrieved from the Google Cloud Console, we can then create a new controller to allow file uploads to the bucket. To do that, generate a new controller using the command below:
The controller can be found in app/Http/Controllers/FileUploadController.php. We will add a function containing the functionality for fetching and uploading a file by updating the file to match the following code.
In the above code, we created a function and called it uploadFile()
. Inside the function, we fetched the requested file and stored it in a folder named test in the Google Cloud Storage, using the storeAs()
function that comes by default with Laravel.
Create a route
To test out the implementation in the upload controller, add the code below at the end of routes/api.php.
Testing
For the purpose of using form data to implement the file upload to Google Cloud, we will be using Postman to test out our API.
In Postman, create a new request like the example below and include the file
parameter in the form data. Then send the request as a POST
request and the file will be uploaded.
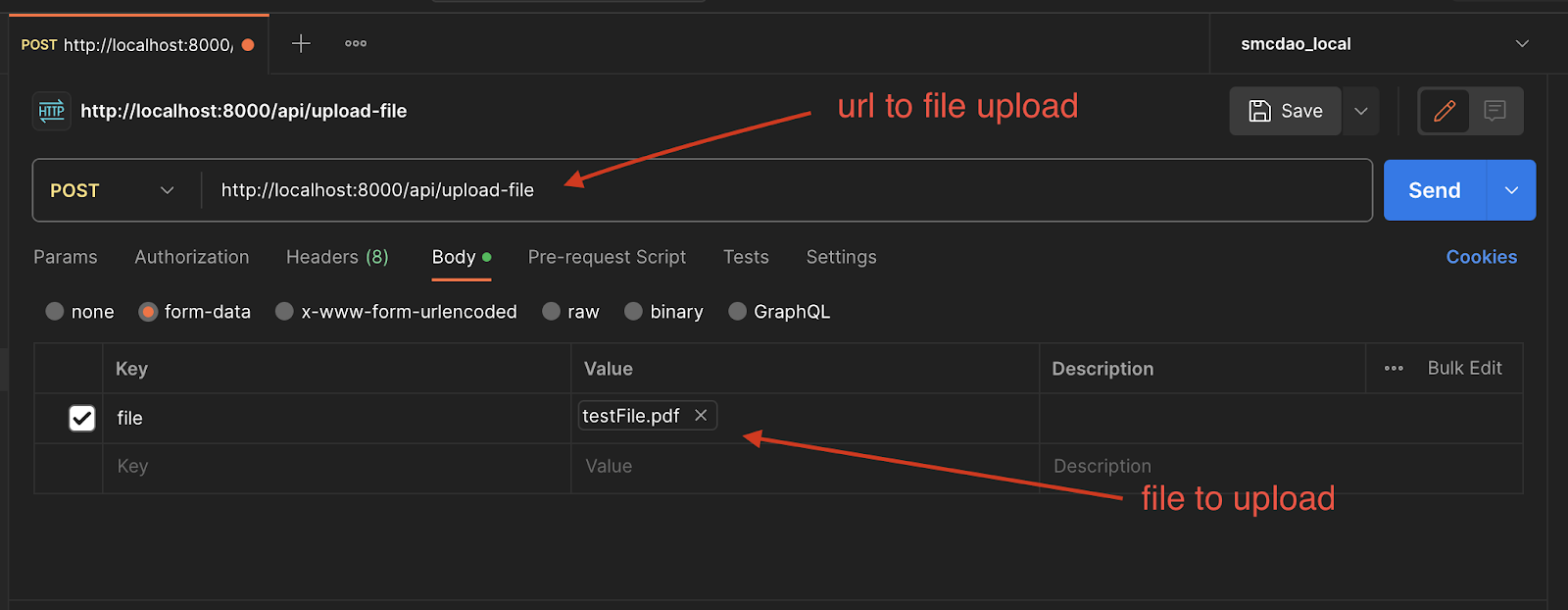
Once the request has been completed, you can check the cloud bucket for the file, as in the screenshot below.
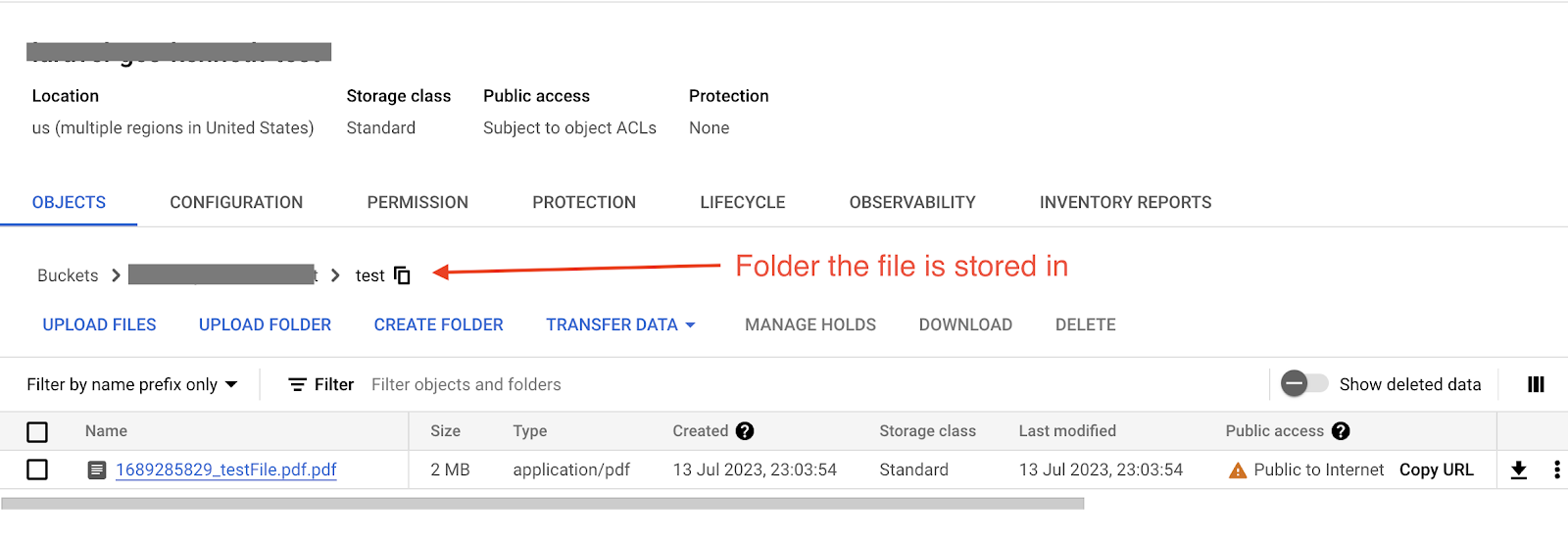
Conclusion
In this article, we went through Google Cloud Platform and expanded into Google Cloud Storage, a service under GCP. Taking it a step further, we set up Google Cloud Storage on a Laravel boilerplate and sent files to a cloud bucket. If you need to set up an external storage system that is secure and scalable for your project, you should consider using the Google Cloud platform to kick start.
Kenneth Ekandem is a full-stack developer from Nigeria currently in the blockchain space, but interested in learning everything computer science has to offer. He'd love to go to space one day and own his own vlogging channel to teach the next generation of programmers. You can reach out to him on Twitter, LinkedIn, and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.