SMS and MMS Notifications with Node.js and Express
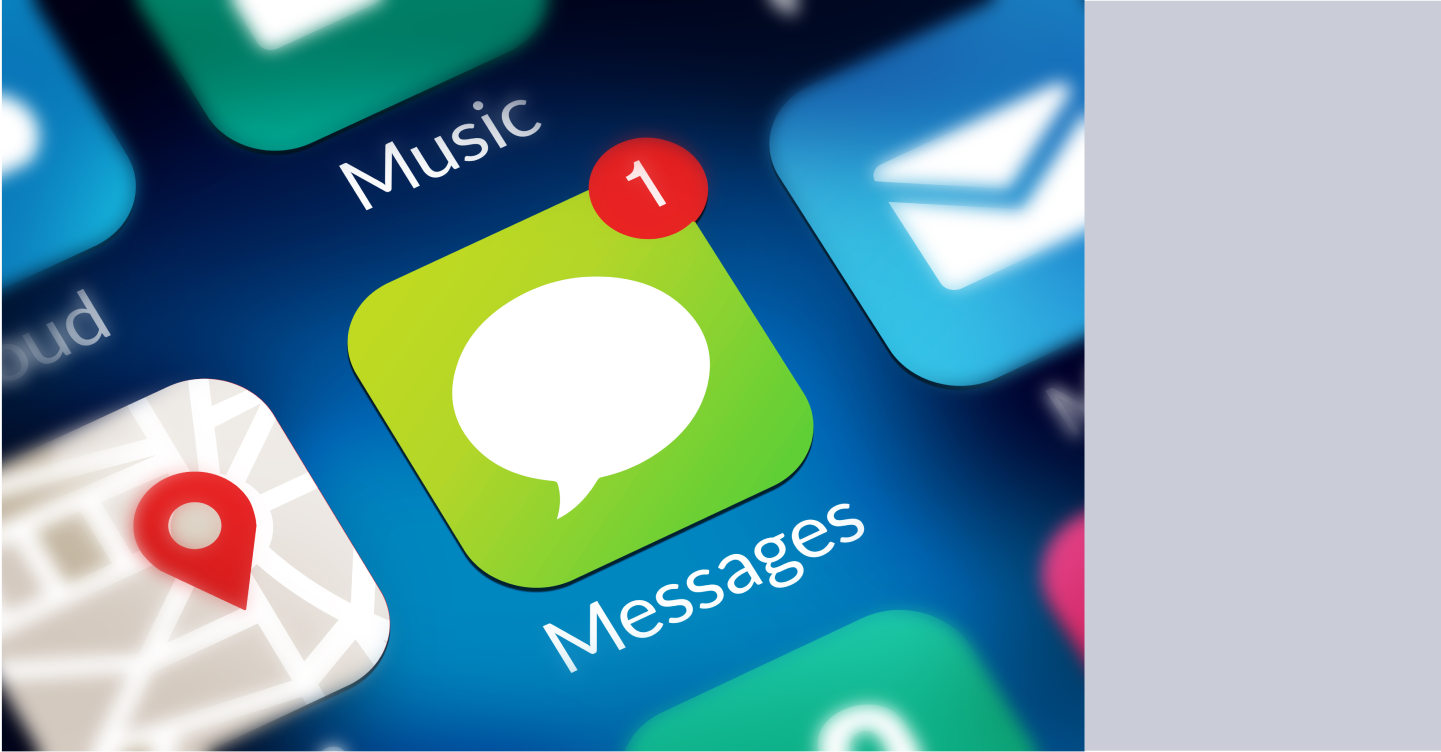
Time to read:
Today we're going to get your server to automatically sound the (textual) alarm when something goes wrong. Using Node.js and the Express framework, we'll light up the phones of all of your server administrators when there's an exception. Read on to send automatic server notifications through SMS and MMS when your code throws an exception.
Start by cloning the sample application from Github, here. Then head to the application's README.md to see how to run it locally.
Configure a Twilio REST client
In order to send messages we'll need to create a Twilio REST client, which requires first reading a TWILIO_ACCOUNT_SID
, TWILIO_NUMBER,
and TWILIO_AUTH_TOKEN
from environmental variables.
We also make the variables available through the config
module.
The values for your account SID and Auth Token come from the Twilio console:
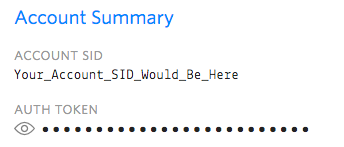
Click the eyeball icon to expose your Auth Token to copy/paste.
You will have to use a purchased number for the TWILIO_NUMBER
variable. Phone numbers can be found and added in the Twilio console.
The link above will explain how to set environmental variables on Mac OSX, Windows, and *NIX (although may vary based on choice of shell). On other platforms it might be in a console or set in some other way. You probably have to read platform specific documentation to see where to set these. Read it, though - best practice dictates you keep them out of the code itself.
Let's get started!
You found what you needed from the console - next, let's get your server administrators in a list.
List Your Server Admins and Well-Wishers
Here we create a JSON formatted list of administrators who should be notified if a server error occurs. The only essential piece of data we'll need is a phoneNumber
for each administrator.
Next, let's have a look at how we're going to capture application exceptions.
Handle All The Unexpected Application Exceptions
We will implement error handling and message delivery as a piece of Express.js middleware.
We'll make all our Twilio API calls from inside our custom middleware.
Now you've seen the main server application - let's look in detail at how we'll send SMS notifications to the administrators in case of emergency.
Trigger Notifications for Everyone on the Administrator List
In our Express middleware module, we read all the soon-to-be-awoken administrators from our JSON file and send alert messages to each of them. We do that using the sendSms
method in twilioClient
. We also call next
with the error object, which will allow other configured middleware to execute after us.
And that's how our notifications will be prepared - let's take a look now at how the server will send the message(s).
Send SMS or MMS Messages from the Server
There are three parameters needed to send an SMS using the Twilio REST API: from
, to
, and body
. US and Canadian phone numbers can also send an image with the message (other countries will have an automatic shortened URL). Just uncomment mediaUrl
and insert your choice of image, serious or funny.
And with that, you've seen how easy it is to add powerful administrative features with Twilio! Let's explore where you might want to look next...
Where to Next?
At Twilio, we love Node.js - and we have plenty of Node content for you to keep those browser tabs full.
Two-Factor Authentication with Authy and Node.js
Increase the security of your login system by verifying a user's identity using Twilio's Authy.
SMS and MMS Marketing Notifications
SMS and MMS messages are a personal way to engage with users. There are many benefits of marketing directly to phones, but perhaps most of all - SMSes and MMSes are opened at a rate far greater than email.
Did this help?
What are you going to build next? Hit up the Twilio account on Twitter and let us know!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.