How to play music over phone calls with Twilio Voice and JavaScript
Time to read:
This post is part of Twilio’s archive and may contain outdated information. We’re always building something new, so be sure to check out our latest posts for the most up-to-date insights.
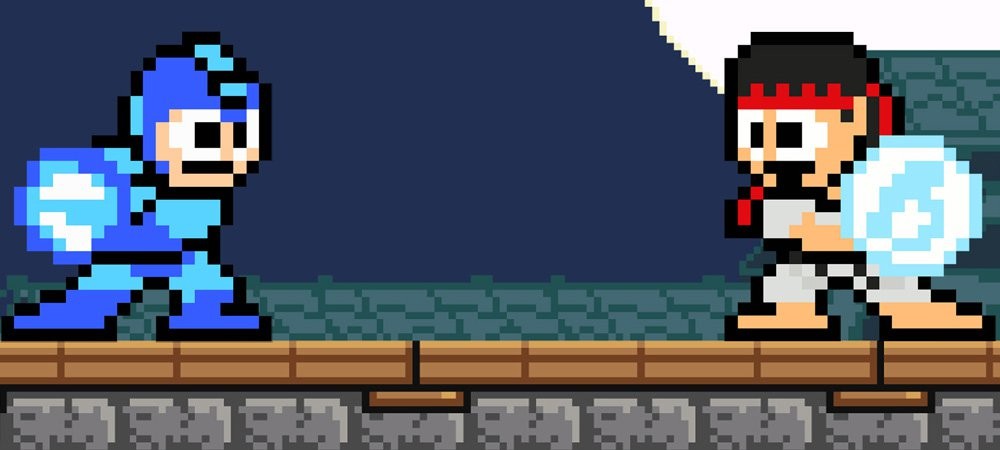
When handling phone calls with TwiML, you have many options for controlling the caller’s experience. You can something in a robot voice, other numbers into the call and even the call. My personal favorite is the ability to play audio files over the phone using the TwiML verb. You can even create your own version of Dial-A-Song, a hotline run by the band They Might Be Giants that allows you to listen to their music if you call the number (844) 387-6962.
Today I’m going to show you how to make and receive phone calls using the Node.js Twilio library. We will also be using some ES6 features to “spice things up a notch.” What better way to show what we can do with Twilio voice than to allow the callers to rock out over the phone?
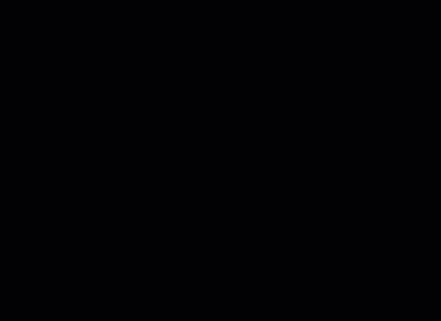
Here is our code in full on GitHub if you want to skip straight to having a working app.
Gearing up
We are going to need to grab some gear and tune up first. For starters, you’ll need to have have Node.js and NPM installed. You will also need to create a Twilio account and purchase a phone number with Voice capabilities.
Now let’s initialize a new app. Open your terminal, navigate to the directory you want to start this project in and run:
This will generate a package.json file that will contain all of the information about our project. Feel free to breeze through the prompts if you are not interested in customizing the default options.
After you have that taken care of we can install our dependencies.
We will need the Twilio Node module so let’s grab that:
When our phone number receives a call, Twilio will make an HTTP request to the URL that we provide. We will have to create a web app to handle these incoming calls. Let’s use the Express web framework to do this:
We will also need to install Babel in order to run our ES6 code. Babel is a compiler for JavaScript that can turn your ES6 code into regular ES5 JavaScript that will run everywhere.
Installing Babel globally will allow you to use the babel-node
command line tool which can directly run your ES6 code. You may need to use sudo to run this command:
Now that we have all of our dependencies installed we still need one important thing: a song to play over the phone. I was having trouble figuring out which tune would be appropriate until I realized there exists a song that goes with everything.
For those of you who don’t know, this is the infamous Guile’s Theme from Street Fighter 2. Luckily for us there exists even better versions of this tune provided by the wonderfully talented community of musicians at OverClocked ReMix. A band called Shinray did a metal remix of Guile’s Theme that manages to surpass the original in terms of epicness. This is freely hosted online making it a perfect candidate for our phone call’s soundtrack.
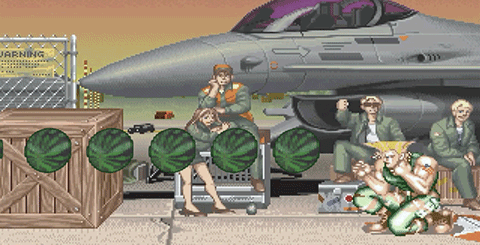
Try calling (347) 826-3536 to hear “Guile’s Theme Goes with Metal” over the phone for a sneak peek at what we are building.
Generating some TwiML
Now we are ready to build our application. The first step is to create a web app that will respond to incoming HTTP requests with TwiML – a set of XML tags that will tell Twilio what to do when you receive an incoming call. This web app will generate TwiML instructions to play the mp3 file of the Guile’s Theme remix that we are using.
Let’s start working on our Express app to take care of this. You may not be familiar with some of the new features of ES6 so let’s walk through the code that our app will contain step by step. Fire up your text editor and create a file called “index.js” where we will write our app.
First we need to import the modules we will be using. You may be used to something like
but we can use new ES6 import statements instead:
Next we need to create an app object and add a route to it that will accept HTTP requests from Twilio.
Notice that we used the new arrow syntax in the anonymous function that we passed to app.post()
rather than using the traditional function keyword. Arrow functions lexically bind the outside scope’s this
variable to the function that you’re writing.
Now let’s fill out the function that we are giving to app.post
:
Here we are using the let
keyword to declare our variables. It allows us to use block scoping instead of JavaScript’s traditional functional scoping with the var
keyword. This allows us to avoid adding variables to the function scope that are only relevant to a particular block.
All we have left to do is to declare the port our server will listen on:
We are using another anonymous arrow function, but this time it will be a one liner withno arguments. It may look strange at first, but this syntax allows us to keep things concise.
Here is the entire code for our Express server in case you missed anything:
Now let’s run our server:
Open up a new terminal window and try hitting it with curl to make sure everything is working and we receive XML as a response:
Our response should look like this:
In order for Twilio to see our application and for us to be able to receive phone calls, we need to expose our server to the Internet. We can do this using ngrok. If you want to learn how to set up ngrok, you can follow my buddy Kevin’s tutorial.
Once you have that taken care of, have ngrok listen on port 3000:
You should see a screen that looks like this with a generated link that we can visit to access our Express app.
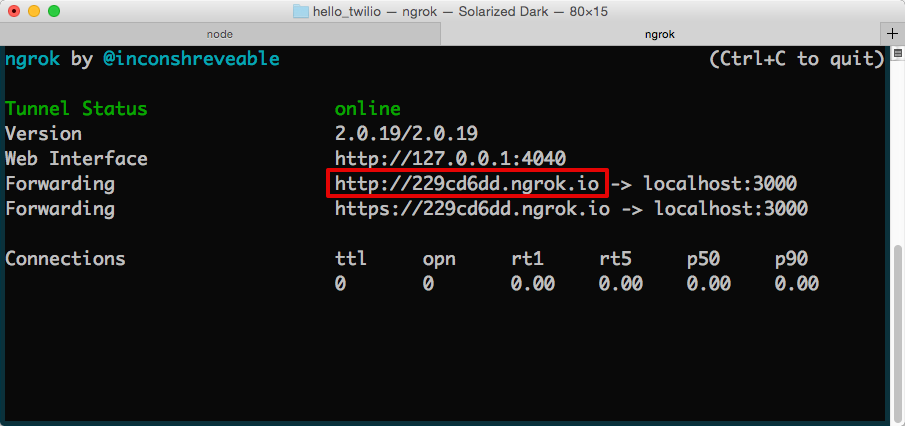
We need to add this URL to our Twilio phone number in the account phone numbers dashboard so Twilio knows to send us an HTTP request when a call is received.
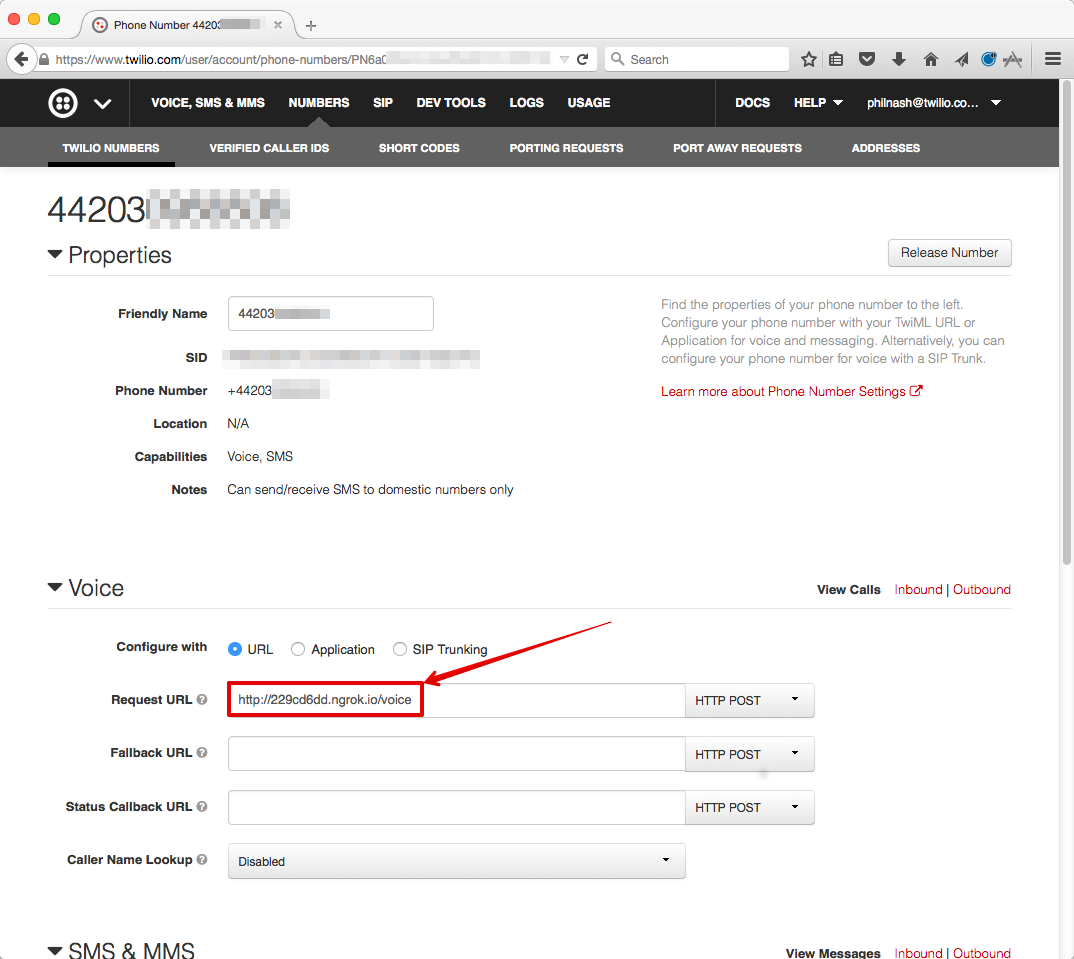
It’s time to win round one of our battle. Whip out your phone and give your Twilio number a dial to have quite possibly the most epic phone call of your life.
Initiating phone calls with NodeJS
Being able to call a phone number to hear a rockin’ version of Guile’s theme is a ton of fun. But we can also start the call ourselves with a little bit of code. This involves using the Twilio REST API.
We are going to write another quick Node script that will send a phone call to a number of your choice using the TwiML we generated earlier to play the same song.
Create a file called “makeCall.js” in your text editor and add this code:
Don’t forget to replace the variables with their appropriate values (a phone number to call for testing, your Twilio number, and the URL to the TwiML that your Express app is generating).
This is all the code we need to make phone calls, but we still need to authenticate with Twilio. Head over to your Twilio account dashboard and grab your Account SID and Auth Token. Store them in environment variables like so:
No need to add these to your code, because upon initialization of the REST client object, the Twilio Node module checks to see if these environment variables exist.
You should now be able to make a phone call by entering the following in your terminal:
Now listen in amazement as you once again experience the epicness of Guile’s Theme, this time initiated by your code.
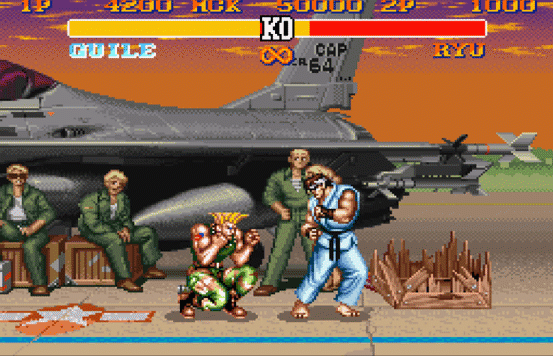
You Win
You’ve won round 2 by implementing an app that can make and receive phone calls using the Twilio Node.js module, and even got it running with newer ES6 features. You are now ready to change your app to play different music files or utilize some of the other TwiML verbs that you haven’t messed around with yet. I am excited for you to move on to the next round, and look forward to seeing what you build with Twilio Voice.
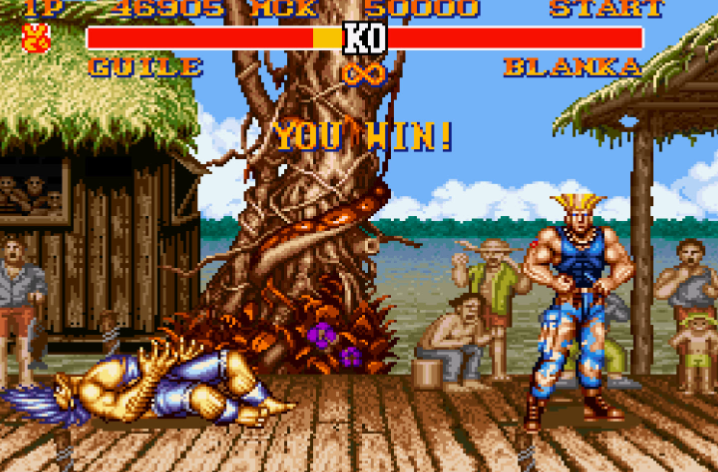
Feel free to reach out if you have any more questions or want to show off your hacks.
Email: sagnew@twilio.com
Twitter: @Sagnewshreds
Github: Sagnew
Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.