Sanic Getting Started: asynchronous, uvloop based web framework for Python 3.5+
Time to read:
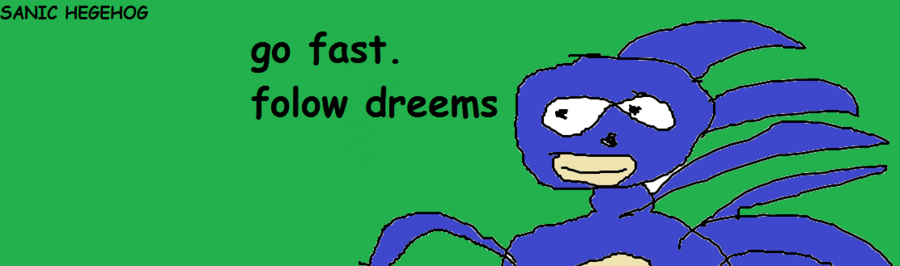
What Is the Sanic Python Framework?
uvloop has been making waves in the Python world lately as a blazingly fast drop-in for asyncio’s default event loop. Sanic is a uvloop-based web framework that’s written to go fast. It's similar to Flask in that it's easy to use, but its enhanced speed is what sets it apart. It is also named after the popular Sanic Internet meme, a poorly drawn version of Sonic the Hedgehog.
Sanic is made for Python 3.5. The framework allows you to take advantage of non-blocking code via async/await syntax for defining asynchronous functions. With this, you can write async applications in Python similar to how you would write them in Node.js.
Sanic Python Web Framework: Getting Started
The “Hello World” example with Sanic looks like this:
Create a new directory for this project, and paste that code into a new file called app.py
. In order to run this code, you’ll also want to create a virtual environment (make sure to use Python 3 when creating the environment) and run the following command to install Sanic:
And run the application:
And visit http://localhost:8000 to see “Hello World!” on the page.
“Hello World” is nice, but let’s see what else we can do. In this next example, we’ll switch things up a bit to figure out how to work with query arguments in the request data. Change the code in app.py
to the following:
Run the code again and this time visit http://localhost:8000/?name=Sam. Feel free to replace “Sam” with your own name. When you visit the web page, it should be greeting you by name.
Responding to text messages
Next we can use Sanic to do something useful. We’ll write code that uses Twilio to respond to a text message. You’ll need a Twilio account for this, but don’t worry you can sign up for free.
When someone texts your Twilio number, Twilio makes an HTTP request to your app. Details about that SMS are passed via the request parameters. Twilio expects an HTTP response from your web app in the form of TwiML, which is a set of simple XML tags used to tell Twilio what to do next.
Replace the code in app.py
again with the following code to quickly respond to a text message:
All you need to do is grab a Twilio number or use a phone number that comes with your trial account and configure it to send POST
requests to your app whenever you receive a text message.
Our app needs a publicly accessible URL. To avoid having to deploy every time we make a change, we’ll use a nifty tool called ngrok to open a tunnel to our local machine.
Ngrok generates a custom forwarding URL that we will use to tell Twilio where to find our application. Download ngrok and run it in your terminal on port 8000
Next we need to point a phone number at our app. Open the phone number configuration screen in your Twilio console. Scroll down to the “a message comes in” field. Before entering your URL you should see:
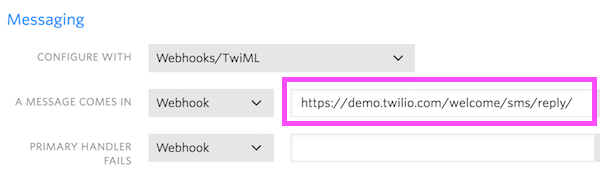
Punch in the URL for our message route that was generated by ngrok. It should look like http://your-ngrok-url.ngrok.io/sms
.
Click save, make sure your application is running and text your number to get a response.
Rollin’ around at the speed of sound
That should be all you need to get started building apps with the Sanic Python framework. You are officially part of the revolution of meme-driven development.
Of course, Sanic is a brand new framework so expect improvements and changes in the near future. You can even contribute to its open source repository and help build it yourself.
Feel free to drop me a line if you have any question or just want to show off what you built:
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.