Automated Yugioh Deckbuilding in Python with OpenAI's GPT-3 and Twilio SMS
Time to read: 6 minutes
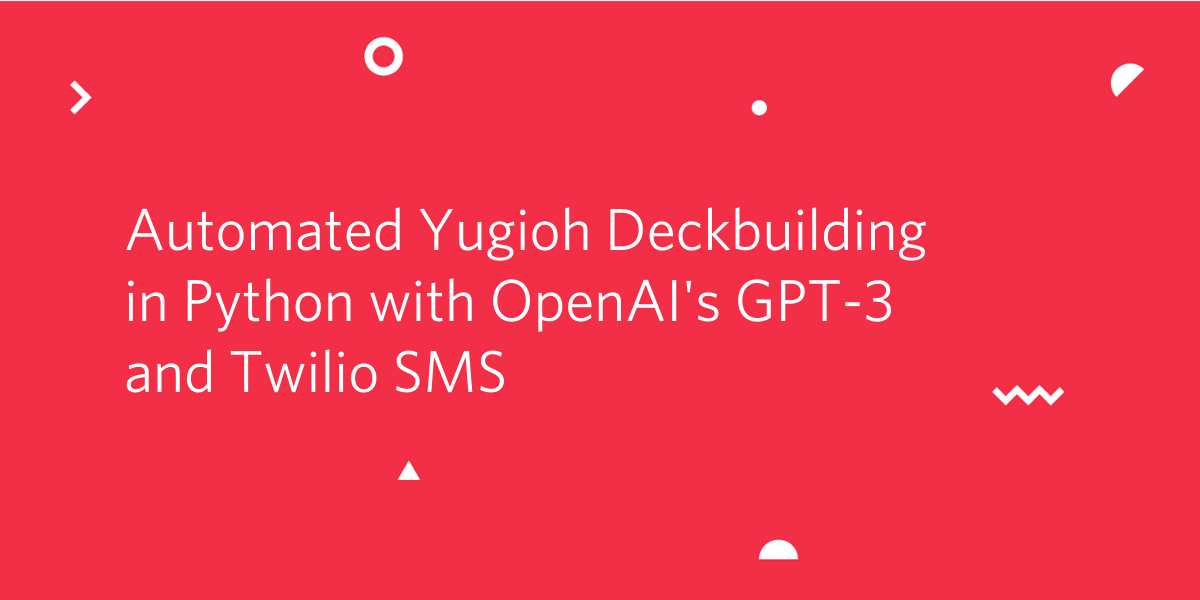
Coming up with a deck in any trading card game is often very difficult, and takes a lot of thought and experimentation. What if we could just have a computer do it for us?
OpenAI's new GPT-3 (Generative Pre-trained Transformer 3) model was trained on a massive corpus of text making it incredibly powerful. This can be used to generate something similar to pretty much any text found on the internet, including in our case Yugioh deck lists.
Let's walk through how to create a text-message powered bot to generate Yugioh deck lists in Python using Twilio Programmable Messaging and OpenAI's API for GPT-3.
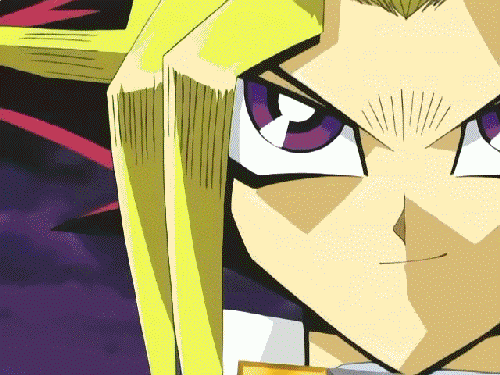
Before moving on, you'll need the following:
- Python 3.6 or newer. If your operating system does not provide a Python interpreter, you can go to python.org to download an installer.
- A virtual environment enabled before installing any Python libraries.
- A Twilio account and a Twilio phone number, which you can buy here.
- An OpenAI API key. Request beta access here.
- ngrok to give us a publicly accessible URL to our code
- Redis, which you can install with the following commands:
Introduction to GPT-3
GPT-3 is a highly advanced language model trained on a very large corpus of text. In spite of its internal complexity, it is surprisingly simple to operate: you feed it some text, and the model generates some more, following a similar style and structure.
We can see how versatile it is even by giving it a small amount of input text. Just from a short sentence followed by "[deck]", it's able to determine that this text is structured as a deck list similar to something you'd see on an online forum, and generate a somewhat coherent example based on that. Here's an example using the input text "Here is my Yugioh deck: \n\n[deck]":
I don't think that deck would be great for tournament play, but it looks like those are all cards that actually exist. It even has over 40 cards so it seems to be legal with some small exceptions like having two copies of Raigeki, although as someone who hasn't seriously played this game in over 10 years it blows my mind to see that having 3 copies of Sinister Serpent is actually allowed nowadays.
As mentioned above, this project requires an API key from OpenAI. At the time I’m writing this, the only way to obtain one is by being accepted into their private beta program. You can apply on their site.
Once you have an OpenAI account, you can use the Playground to play around with GPT-3 by typing in text and having it generate more text. The Playground also has a cool feature that allows you to grab some Python code you can run, using OpenAI's Python library, for whatever you used the Playground for.
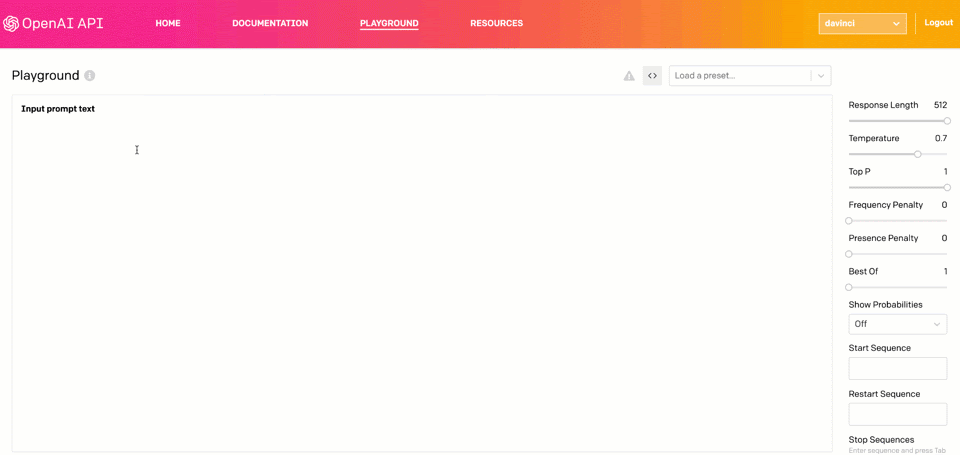
Working with GPT-3 in Python using the OpenAI helper library
To test this out yourself, you'll have to install the OpenAI Python module. You can do this with the following command, using pip:
Now create an environment variable for your OpenAI API key, which you can find when you try to export code from the Playground. Actually, while you're at it you might as well grab your account credentials from your Twilio Console and make environment variables for those as well:
We will use these in our code throughout the post. To try generating a deck list, open a Python shell and run the following code, most of which I took directly from the Playground:
You should see something like this printed to your terminal (I took empty lines out in the deck list to reduce the amount of scrolling you need to do):
OpenAI has a variety of different models to choose from, and for this one we are using davinci
which is the most advanced but also the slowest. I haven't been able to get good results for building decks with any of the other models. So one concern I have at this point is that it will take too long to generate this text, resulting in an HTTP timeout when we try to respond to a text message. We'll handle this later by using a tool called Redis Queue, which we also have a tutorial for.
Sending your generated decklist via SMS with Twilio
We want to make it so a person can text a phone number and receive a Yugioh deck list. Before being able to respond to messages, you’ll need a Twilio phone number. You can buy a phone number here.
As I mentioned before, generating the text takes too long to simply respond to Twilio's requests to our app for incoming text messages, so we will have to break this up into two parts. A Flask app which will handle messages from the user will begin generating a deck list asynchronously using Redis Queue, and when that task is finished a text message will be sent with the deck list.
Let's write the code for sending a text message once the deck list is generated. This will be called from our eventual Flask app. Create a file called deck_generator.py
and add the following code to it:
The twilio_client
object implicitly accesses the account credentials you set as environment variables, and sends the text message at the end. There is also some new logic for handling cases where the script either doesn't finish generating a deck, determined by the presence of a closing "[/deck]" string in the text, or where it generates multiple (which sometimes happens). In the latter case, because the deck list begins immediately after the prompt text, we cut the string off at the beginning of the first "[/deck]" tag and it just sends the list of cards.
You can test this code out by calling the generate_decklist
function in a Python shell and passing your personal phone number and your Twilio phone number to the function.
Configuring a Twilio phone number
We're going to create a web application using the Flask framework that will need to be visible from the Internet in order for Twilio to send requests to it. We will use ngrok for this, which you’ll need to install if you don’t have it. In a new terminal window, run the following command:
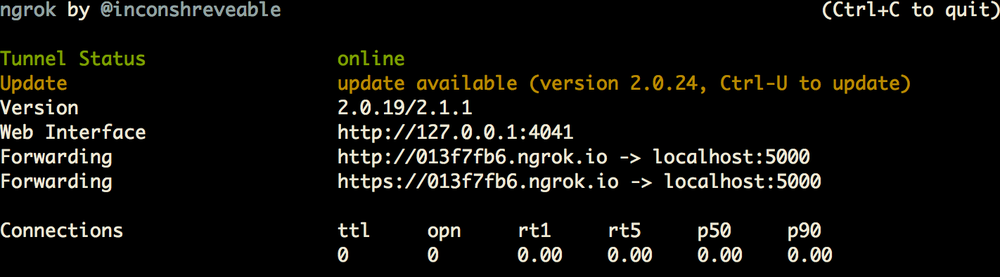
This provides us with a publicly accessible URL to the Flask app. In your Twilio Console, configure your phone number as seen in this image so that when a text message is received, Twilio will send a POST request to the /sms
route on the app we are going to build, which will sit behind your ngrok URL:
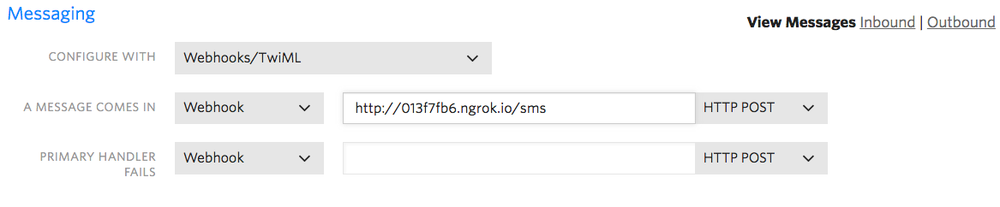
With this taken care of, we can move onto building the Flask app to respond to text messages.
Responding to text messages in Python with Flask
So you have a Twilio number and are able to have OpenAI generate a deck list. It's time to link the two together. Before moving on, install the Twilio, Flask, and Redis Queue Python libraries with the following command:
Now create a file called app.py
and add the following code to it for our Flask application:
This Flask app contains one route, /sms
, which will receive a POST
request from Twilio whenever a text message is sent to our phone number, as configured before with the ngrok URL. In this route, a task is sent to Redis Queue to generate a deck list and send a text message when it's finished. The HTTP response will contain TwiML that has Twilio send a message to the user telling them a deck list is being made for them.
Save your code, and run it with the following command:
You'll have to run a Redis server as well as an RQ worker before your Flask code will work. Open another terminal window, and run the following command from the directory where Redis is installed:
The &
means that it will run in the background, but if you want to open up yet another terminal window you can take that out of the command. Now for the RQ worker, make sure your virtual environment is activated and run this command from the same directory where your Python code is, same rules apply with the &
:
Because we have ngrok running on port 5000, the default port for Flask, you can now text your Twilio number and receive the list of your new Yugioh deck! Don't worry if you have to try a few times to get a good one.
It's Time to D-D-D-D-D-D-D-D-D-D-DUEL!!!
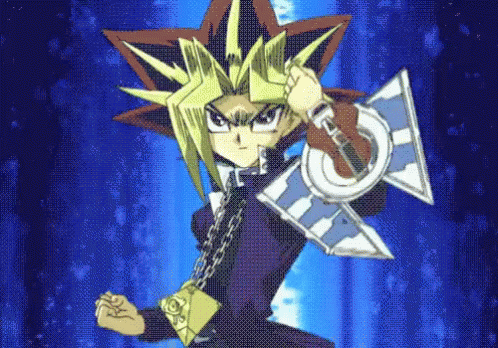
Now that you and your friends can text your Twilio number to receive new deck lists whenever you want, try throwing these cards into a deck on Dueling Book and see what kind of ridiculous matchups you can get. Maybe you can even have a tournament where every duel consists of AI-generated decks!
You can also try modifying the code a bit. Maybe you can get better results by changing the number of tokens generated or using more specific prompt text such as "The following is a Yugioh deck list for the Goat Format: [deck]" or "This is my Blue Eyes White Dragon Yugioh deck: [deck]".
If you want to do more with Twilio and GPT-3, check out this tutorial on how to build a text message based chatbot and this one on how to generate Yugioh fan fiction.
Feel free to reach out if you have any questions or comments or just want to show off any stories you generate.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.