Connect to Your Local Database Using Twilio Functions
Time to read:
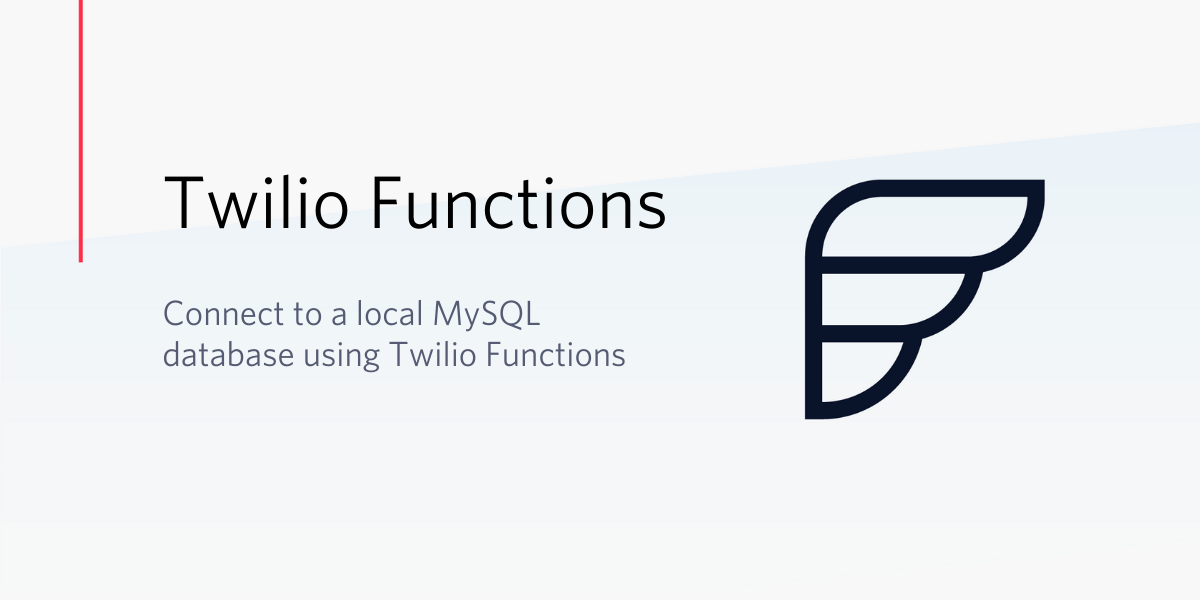
In this post, you’ll learn how to connect to a local or remote database such as MySQL and make queries using Twilio Functions.
Prerequisites and Items accomplished
- Sign up for a free Twilio Account
- Configure MySQL on a local machine
- Configure Ngrok on a local machine
Working with Twilio Functions
Twilio Functions (Beta) provides a complete runtime environment for executing your Node.js scripts. Functions integrates the popular package manager npm and provides a low latency Twilio-hosted environment for your application.
Building apps with Functions
Here's an example of what a Functions script looks like:
The handler method is the starting point of your function, and accepts the following arguments:
- The
context
object includes information about your runtime, such as configuration variables, environment variables. - The
event
object includes information about a specific invocation, including HTTP parameters from the HTTP request. - The
callback
function completes the execution of your code.
Configure a local MySQL instance
MySQL is an open-source relational database management system. MySQL is a very popular database, and we’re also using it today for its quick and easy installation. Please refer to the following for details on installing and configuring a local instance of MySQL.
Once your local instance is up and running, make sure it is accessible via the internet. Run the following queries to check if it's running on the appropriate port and networking is enabled:
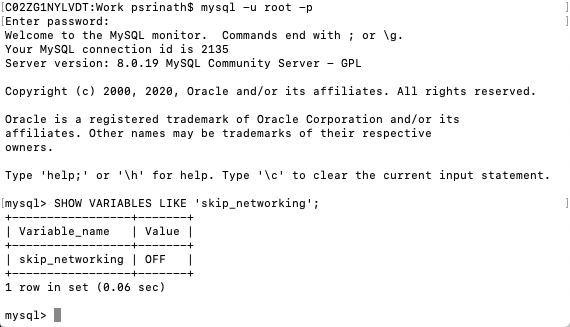
The above query should result in skip_networking value= off
by default.
The above query should result in a port value set to 3306 by default.
Let’s create and use a database by executing the following script:
Once the database is selected, create a user table and insert some values by executing the following script.
Expose network services with ngrok
TCP tunnels allow you to expose any networked service that runs over TCP. ngrok offers secure tunneling capabilities to expose your local development environment. If you haven’t yet, visit ngrok’s download page and download and install the tool.
Once you have the tool installed, here is the script to run:.
The output of the script returns the following details in the terminal:
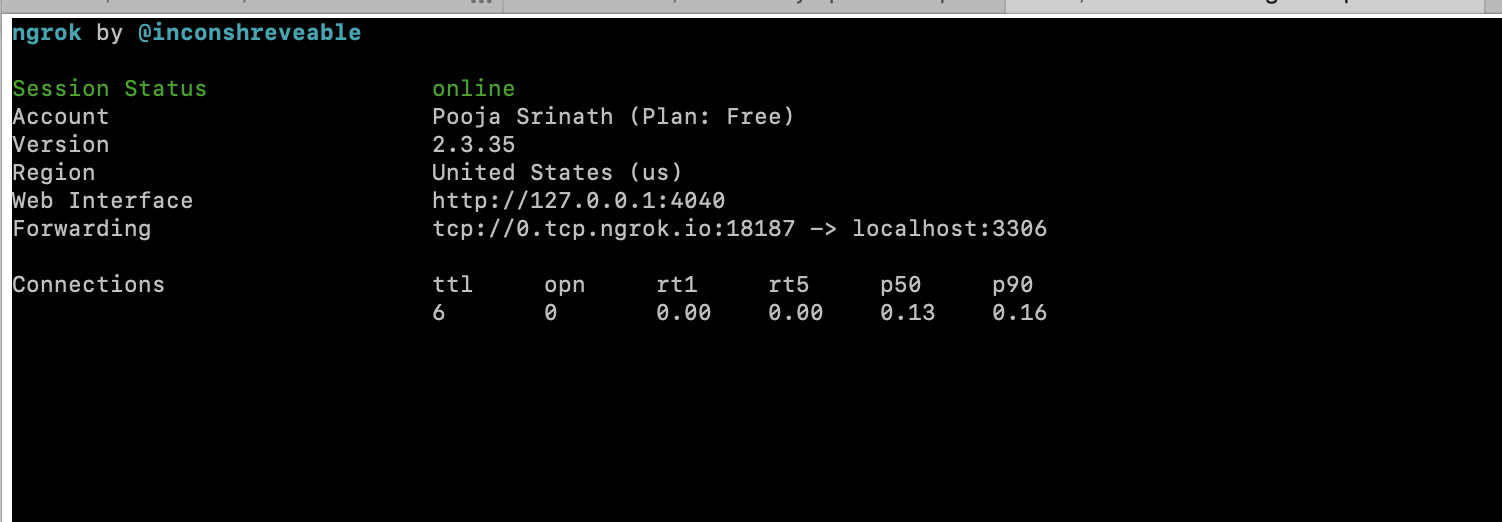
To validate the exposed service is available from the broader internet, run the following script to affirm that MySQL is accessible:
Create Your Twilio Functions
In the following sections, we’re going to be building Twilio Functions. If you’d like more context on Twilio and our serverless tools, please refer to the following articles:
Configure the Function environment variables
The environmental variables for a Twilio Function are configured in the configure section of the Twilio functions portal, as shown in the image below.
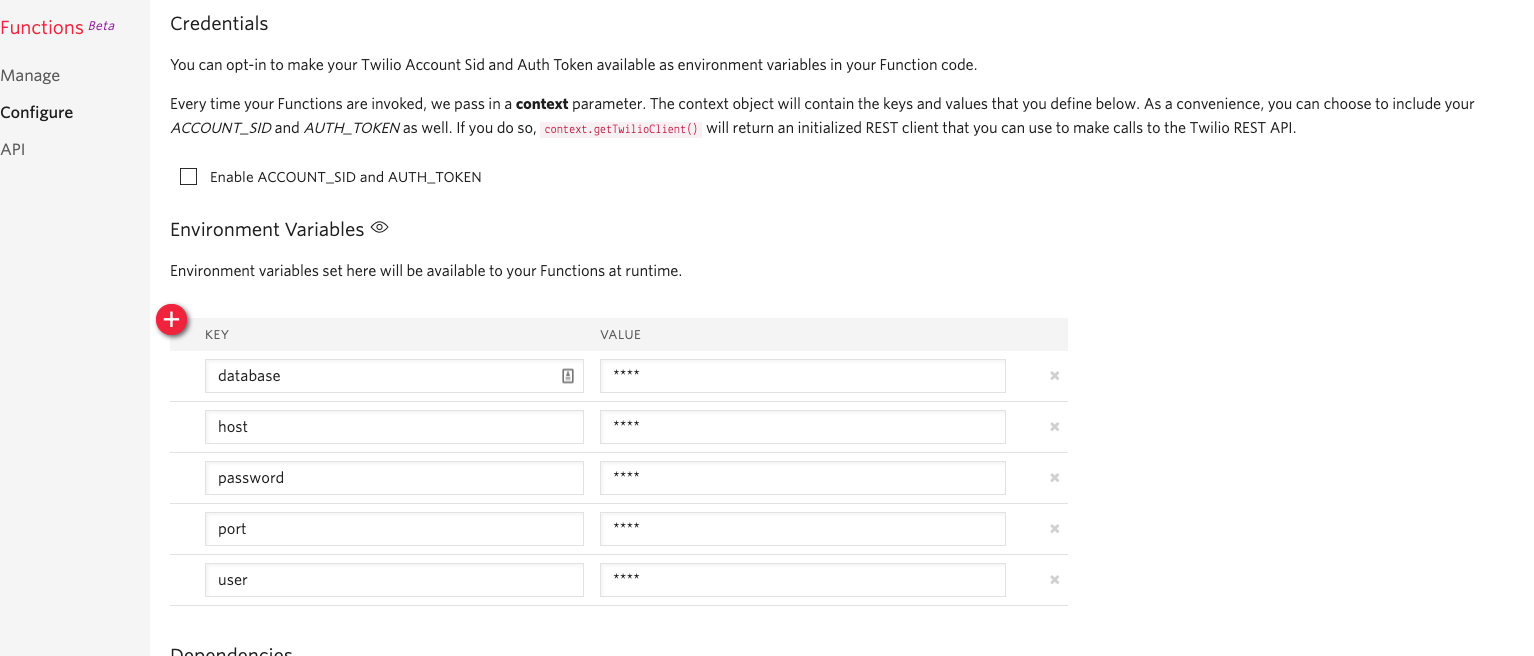
The key-value pairs are nothing but the details of your DB configurations obtained from validating the exposed services. (“Configure a local MySQL instance” above)
The entries of the configure section are provided in the table below:
Key |
Value |
database |
User |
host |
0.tcp.ngrok.io |
password |
password |
port |
18187 |
user |
root |
Configure the Function dependencies
The node dependencies are added through the configure section of the Twilio Functions console, as shown below.
We haven't specified the version – Twilio’s console picks the latest versions available by default. However, I recommend you use mysql > 2.18.1
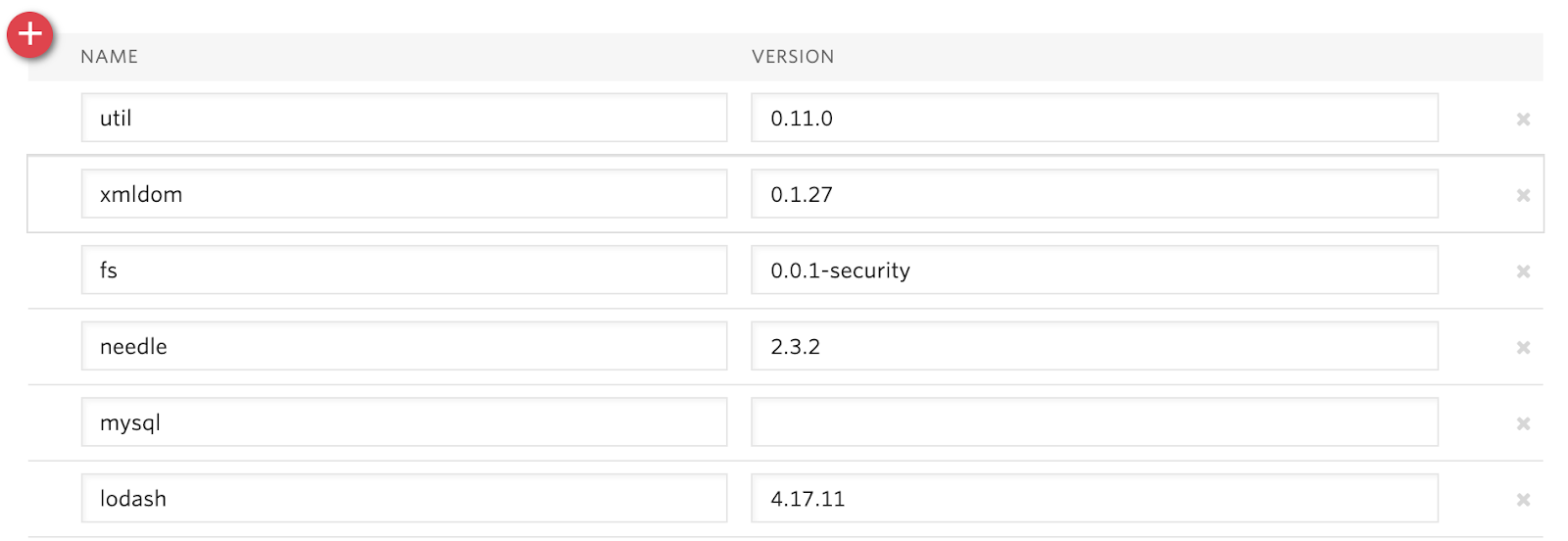
Create a new Function in the Twilio Console
Upon configuring the above values, create a Twilio Function to connect to a local DB and also perform the necessary actions.
Async and await act as syntactic sugar on top of promises, making asynchronous code easier to write and to read afterward.
Create a function named WriteToMySQL and also specify the path, as mentioned in the screenshot.
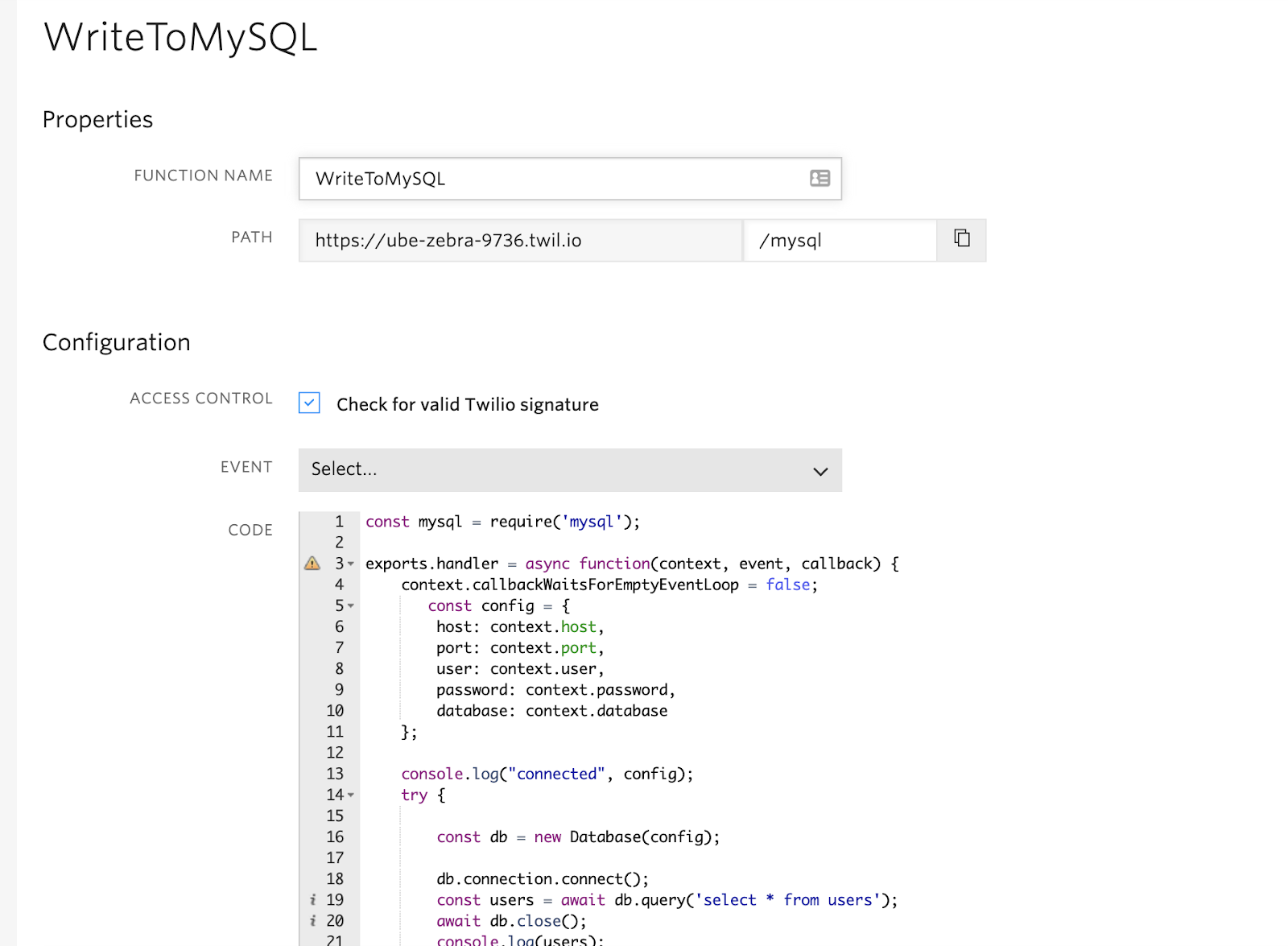
Paste the below code in the code console
From the above code, we can read the values from a database.
In the line const users = await db.query('select * from users');
, we wait for the connection to be established to query the entries of the table users
. Below is the code to write to the database.
In both the codes provided above, we have used the object-oriented programming approach in Javascript.
Debugging your Functions
In-order test these functions quickly, you can spin up a studio project to receive an incoming call to the Twilio number. Say a friendly message and then connect the say widget
to the functions widget
. This will make a call to the above serverless Twilio function. Once the values are returned from the function, pass those responses as an SMS to your phone number.
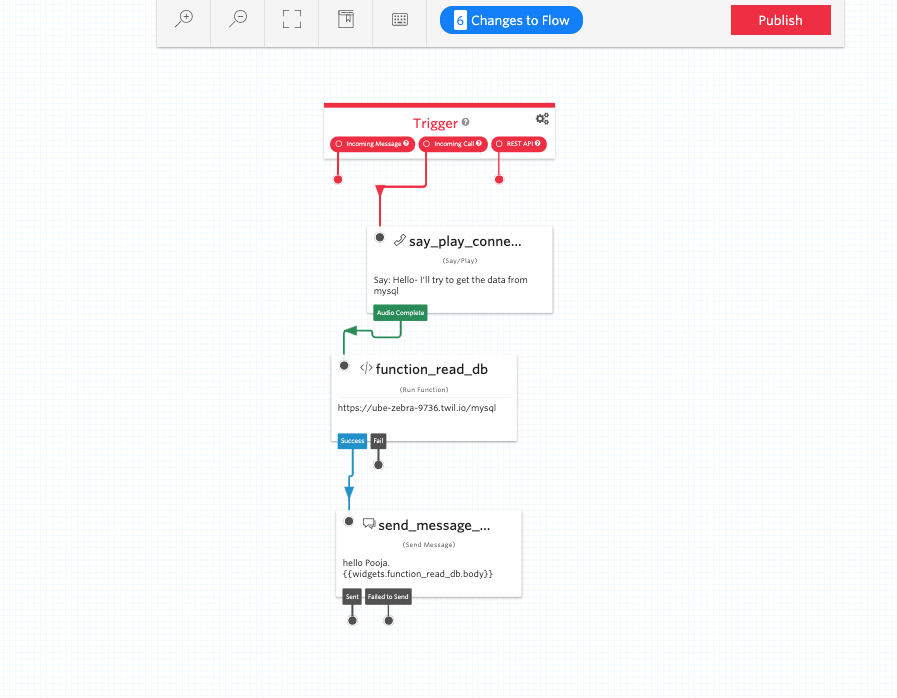
If you're unfamiliar with Twilio Studio or with how to buy a number, please refer to the following articles:
Conclusion:
Reading and writing to a database is simple with Twilio Functions. And better – you don't have to worry about complex server setup as the runtime environment is provided by our Twilio Functions console.
Pooja Srinath is a Senior Solutions Engineer at Twilio. She's focused on learning new things and building cool apps to help budding developers. She can be found at psrinath [at] twilio.com.
Source for the Two Functions:
Further References:
- https://www.twilio.com/docs/runtime/functions#getting-started-with-serverless-and-twilio-functions
- https://www.twilio.com/docs/runtime/functions/debugging
- https://www.twilio.com/docs/runtime/functions/invocation
- https://support.twilio.com/hc/en-us/articles/115007737928-Getting-Started-with-Twilio-Functions-Beta-
- https://serverpilot.io/docs/connect-to-mysql-remotely/
- https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Asynchronous/Async_await
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.