Create Your Own Conference Line in PHP using Twilio Programmable Voice
Time to read: 4 minutes
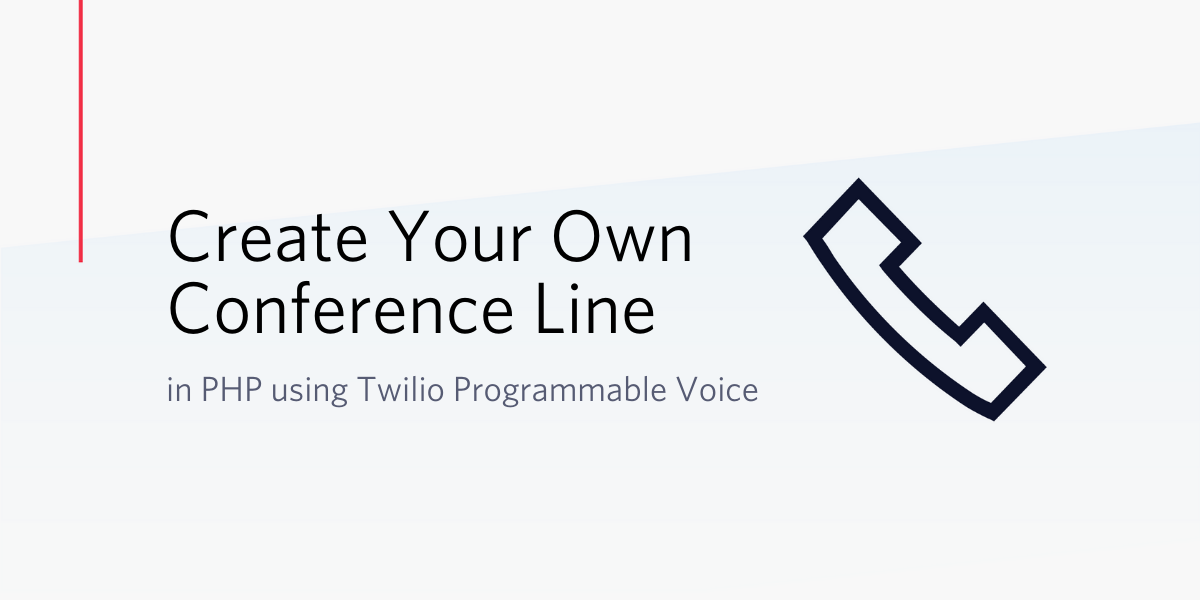
As much of the world is grappling with the overnight shift to work from home, the trusted conference call is a staple that is needed now more than ever. Teams that have only been separated by floors and walls are now teleworking from makeshift offices in kitchens and bedrooms.
While many software-as-a-service solutions exist, your company or organization may desire to roll out your own custom conference call solution or add it to an existing application. If PHP is your language of choice, this quick tutorial will teach you how to build your own conference line in PHP using Twilio Programmable Voice. Your team members will actually be able to call it directly and speak with one another from anywhere in the world.
If Node.js happens to be your preferred language, Sam Agnew just wrote an awesome version of this tutorial on the Twilio blog.
Prerequisites
This tutorial is a build-it-yourself solution, so you will need your own Twilio phone number to connect your team members. If you don’t have one or need an account, take a moment and sign up using the following link.
You will need the following dependencies to create this PHP conference line:
- PHP 5 or above (Version 7 is recommended)
- Composer globally installed
- Ngrok installed
- Twilio PHP helper library
- A Twilio account
Please make sure that you have them installed and configured before you begin.
Create a New Project Directory
For demonstration purposes, the application will be developed locally on your computer. Ideally, you would want to have this application hosted in the cloud for continuous connections and use, but for now, we’ll assume that all work will exist locally.
Select the most ideal place to keep projects on your computer and run the following command in your terminal:
The above commands simply created a project folder and set it as the current folder for us to work from.
Create a New Composer.json File to Install the Twilio SDK
Composer is a popular packager manager for PHP and we will follow that trend, using it to include the Twilio PHP SDK in the subsequent steps. Create the required composer.json
file in our project folder using the following command.
When prompted, confirm the Author, press enter for the remaining options, when presented with “Would you like to define your dev dependencies (require-dev) interactively?”, input n
, and press enter when asked “Would you like to install dependencies now?”
The output will look similar to that below:
The command created a new composer.json
file with the Twilio PHP SDK defined as a dependency and install it. The Twilio PHP SDK will not only give us access to the Twilio Voice API, it will also make it easy for us to write the code necessary to create the conference line.
NOTE: If you are unfamiliar with Composer, there are a lot of great articles such as this one to further your education on the subject.
Create an Application to Handle Calls via PHP
Believe it or not, we’re almost done with the application. All that’s left is the actual code that processes the incoming call via a webhook in the Twilio console. Create that file now in your terminal.
In your favorite IDE, add the following code to conference.php
:
This code is all we need! Isn’t it amazing what can be accomplished with just ten lines of PHP?
The code above imports and autoloads the Twilio SDK that we defined in the previous section. After it loads, we declare a new instance of the VoiceResponse
object from the SDK. This object gives us access to the TwiML verbs needed to define a conference line dial
and conference
.
If you would like to explore more TwiML verbs, this page is a great resource.
Make Your Application Accessible to the Internet
At this point, the internet has no idea that the conference.php
file even exists. In order to use it and connect to your phone number, you’ll need to make it accessible from your local computer. To do that we’ll first start your local webserver using PHP’s builtin server.
Now that your server is running, create a tunnel to expose it to the internet using ngrok.
When ngrok loads, your terminal will start a new session similar to the screen below. Take note of the Forwarding URL as you will use this to create your webhook URL.
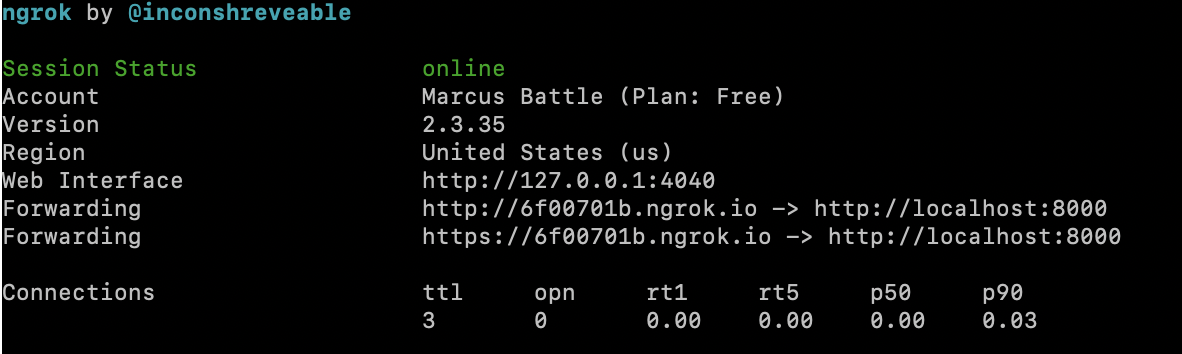
Connect the Twilio Webhook to Your Application
Navigate to your Twilio console and select the phone number that will work for your conference line. Under Voice & Fax there’s a section labeled “A call comes in”. In the field, input your Forwarding URL + /conference.php
. In my instance, that complete URL is https://6f00701b.ngrok.io/conference.php
.
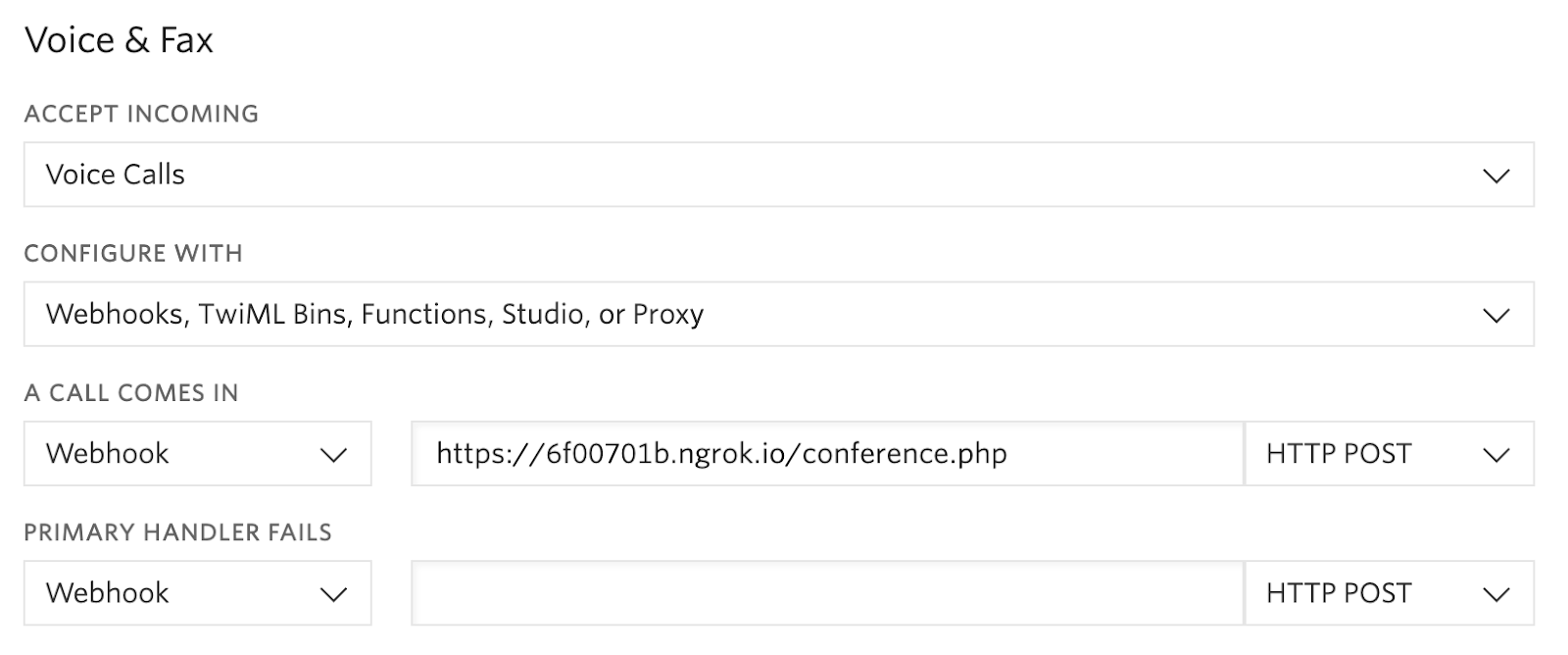
Testing Your Conference Line
You’re done! Your conference call is now complete. You will need two phones to actually test the application.
Simply call your Twilio phone number on two separate lines and start talking!
Conclusion
This tutorial has shown us how simple it is to:
- Create a new PHP project
- Install the Twilio PHP SDK
- Create a webhook
- Connect the webhook to a Twilio phone number
You could extend this application even further by modifying the experience of the call such as signaling a beep when a user joins the call. I look forward to seeing what you build!
Marcus Battle is Twilio’s PHP Developer of Technical content. If you have any questions, use the following channels to reach out:
Email: mbattle@twilio.com
Twitter: @themarcusbattle
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.