How to Forward SMS to Your Telegram Account in PHP
Time to read: 3 minutes
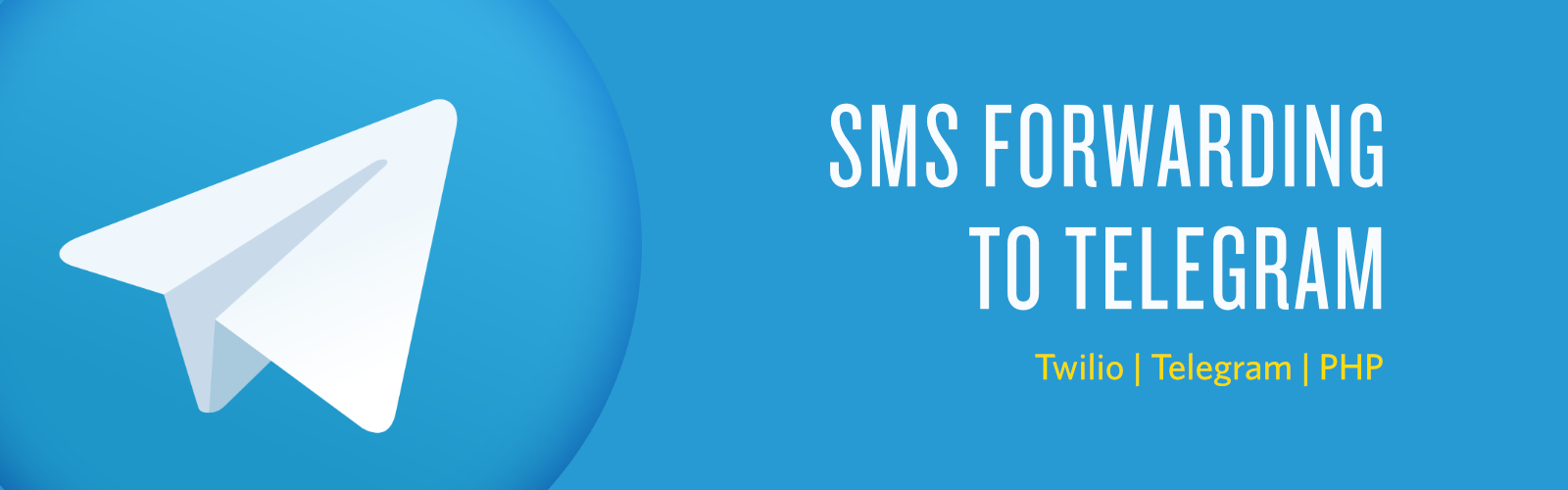
When working, I prefer to have my messages delivered on the computer to minimize distractions. With the switch of a tab, I am able to read my messages and quickly resume my work. On WhatsApp and Telegram, this is possible via WhatsApp Web and Telegram Web respectively. If you have business or personal messages coming in throughout the day via Twilio SMS, and you’d prefer to read them on your computer, I have good news for you. In this tutorial, we are going to learn how to receive Twilio SMS messages on Telegram.
Tutorial Requirements
For this tutorial, you will need a:
- PHP 7 development environment
- Global installation of Composer
- Global installation of ngrok
- Twilio Account
- Telegram Account
Setup our Development Environment
We need to start off by creating a project directory for our application. I have named mine Twilio-Telegram. Inside the directory let’s create two files:
- webhook.php
- .env
The next step is to install a dependency called Dotenv. It is good practice to store sensitive credentials where they can only be retrieved by the server and not the browser. This dependency helps us retrieve credentials stored in the .env file. From your terminal, in the project directory, run the command:
Create Telegram Bot To Capture Messages
In order to receive messages from Twilio on Telegram, we need to create a bot. Here’s the interesting part, we are going to use a bot to create our bot. BotFather, a bot from Telegram, helps us create new bots and make changes to existing bots. You can access BotFather by using this link or searching for it in the search bar within the Telegram app.
In your chat with BotFather, input the text /newbot
. You will be prompted to choose a name and a username for your bot. I have named mine TwilioTelegramBot and the username is Twilio_telegram_bot. BotFather will send you a message that contains a link to your newly created bot as well as a token.
Every user that interacts with the bot gets a unique chat ID. In order to receive messages via the API, we need to know our chat ID. To get it, start a conversation with your new bot. Type a random text in the chat. To get your chat ID, navigate to the following URL on your browser:
Remember to replace <TOKEN> with the token you received from BotFather. You should get a response similar to this:
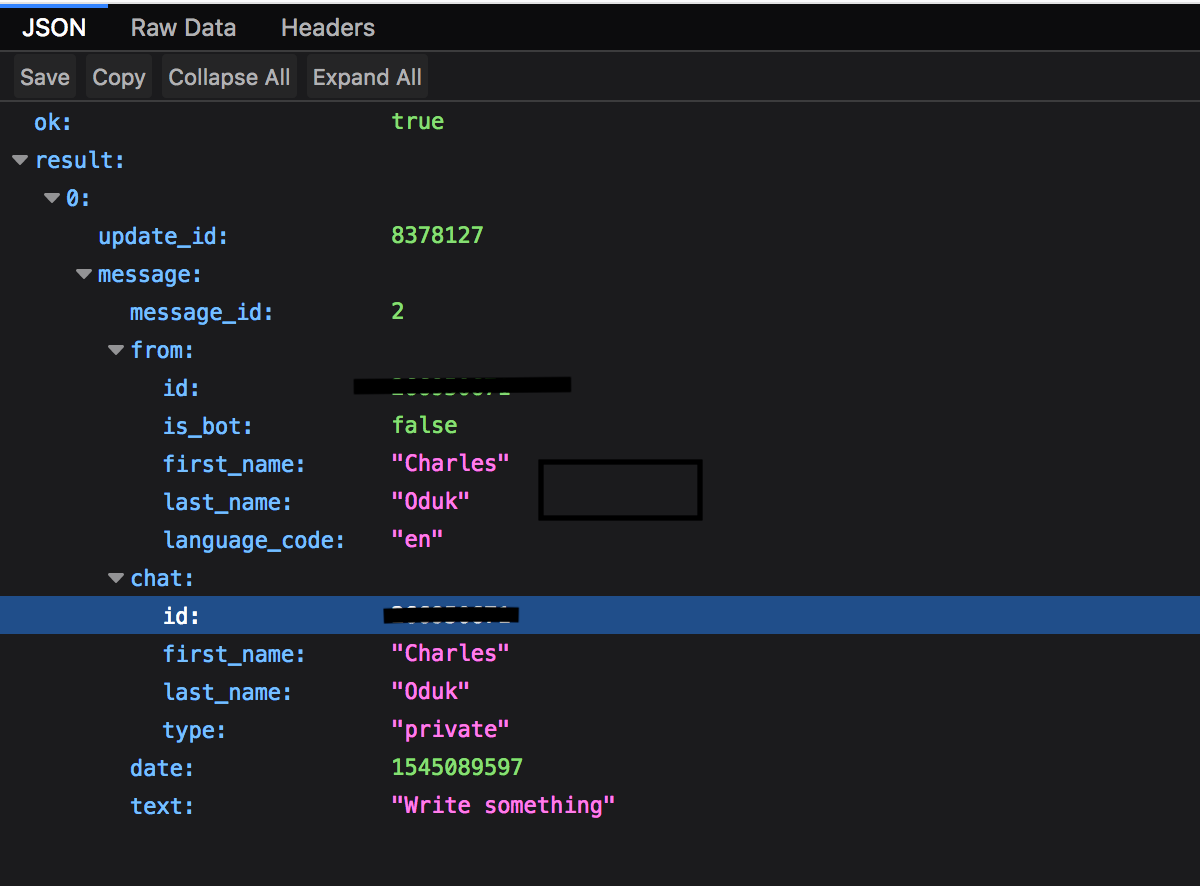
In your .env file, add the following environment variables and their corresponding values:
In the next section, we will update our webhook.php
file. The above chat ID will be required to receive notifications in our webhook.
Create Twilio Webhook to Forward Messages
By default, Twilio provides a webhook that sends a response when a message is sent to your number. In this section, we will work on building our own webhook that will handle the incoming messages. In the webhook.php file copy and paste this code:
This file listens for a POST request, retrieves the message and sends it to Telegram using the URL:
In our webhook, we pass the message retrieved from Twilio as well as the Chat ID in the body to Telegram. We will now use ngrok in order to make this webhook accessible through a public URL from our localhost.
From the terminal, run the command:
On a new terminal window, run the command:
Your terminal window should look like this:
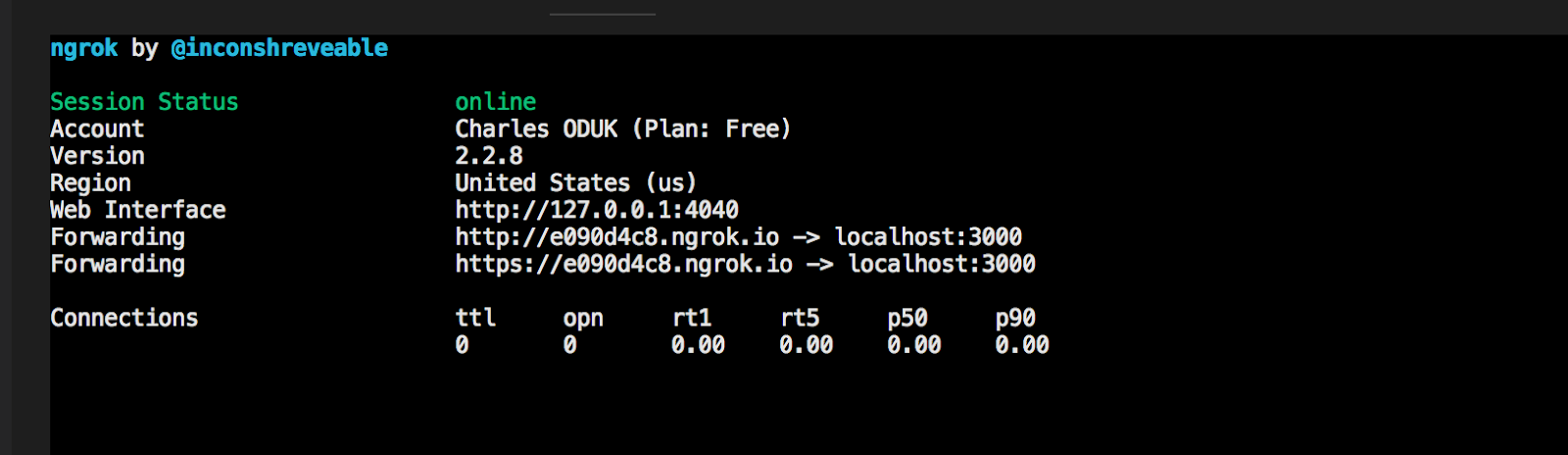
In our Twilio project, we will provide the webhook URL above followed by the path to our webhook file. For example, mine is:
The final step is hooking our Twilio number to the webhook we created. Navigate to the active numbers on your dashboard and click on the number you would like to use. In the messaging section, where there is an input box labeled A MESSAGE COMES IN, replace the URL with our webhook URL and click “Save”!
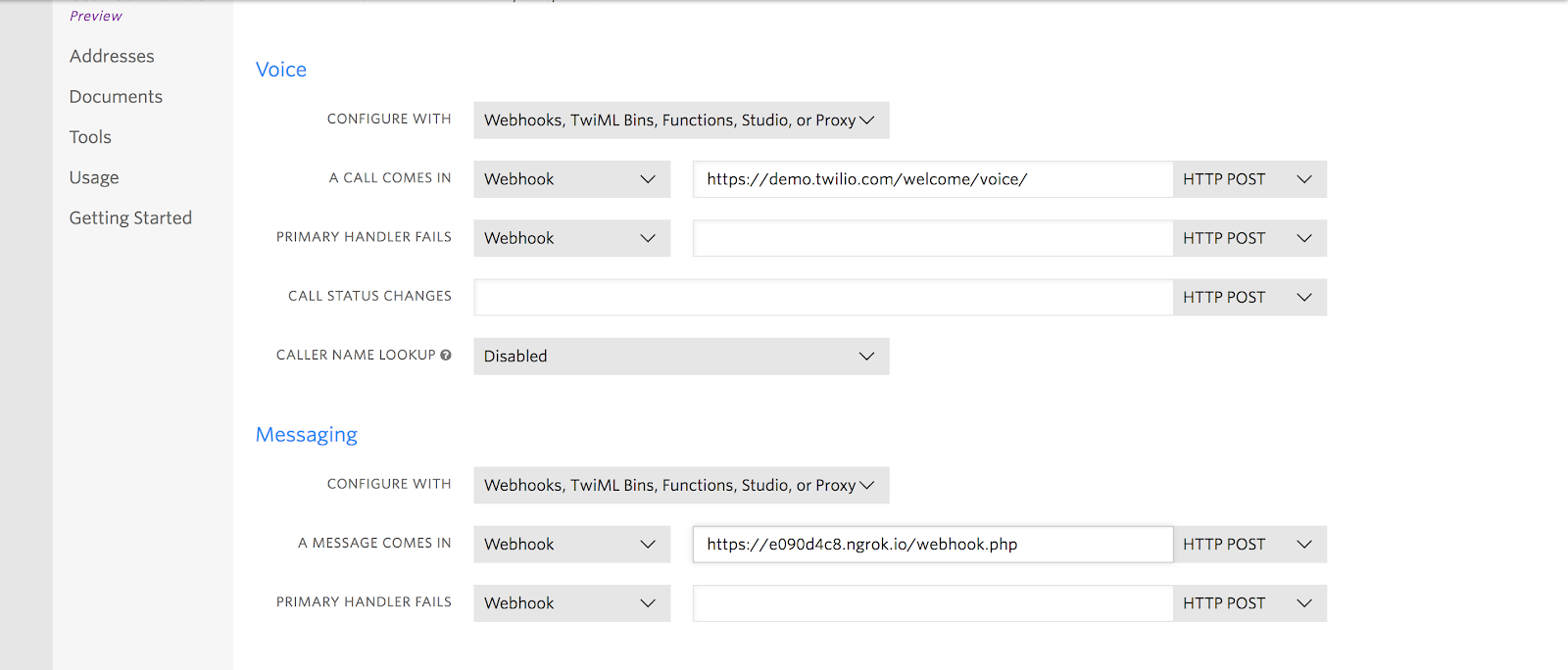
Testing Our Webhook
To test our logic, let’s go ahead and send an SMS to our Twilio number. The message you send should immediately show up in the Telegram bot we created.
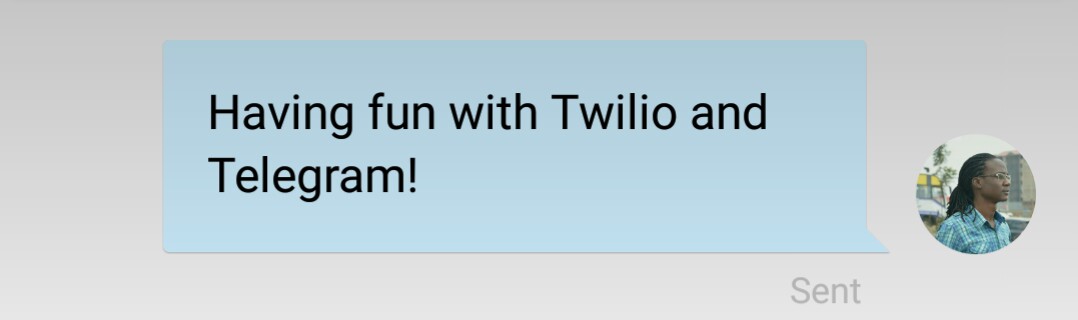
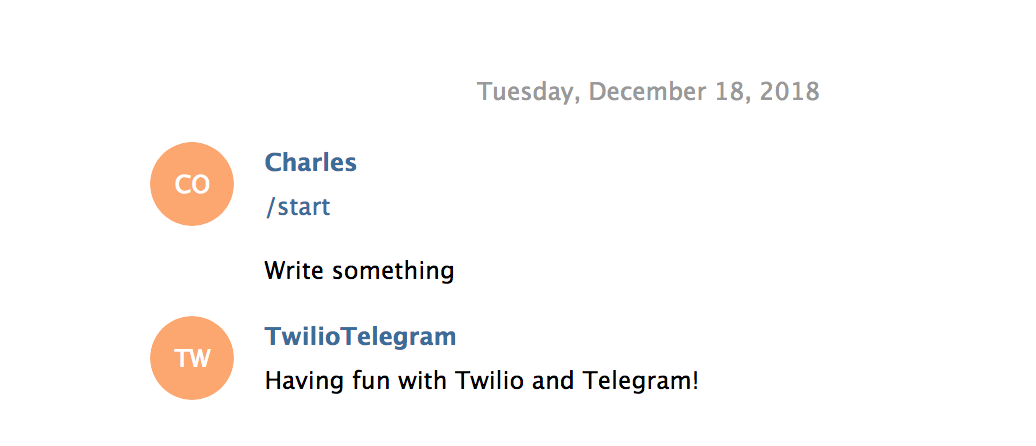
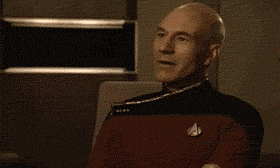
Congratulations! You have successfully received a Twilio SMS on Telegram. You could use this implementation as a customer service solution. You could have your customer service team as bot admins and have all customer SMS redirected to the bot for a prompt response. I look forward to hearing what you work on. You can reach me on:
Email: odukjr@gmail.com
Twitter: @charlieoduk
Github: charlieoduk
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.