How to Hang Up Currently Active Twilio Phone Calls with Python
Time to read: 3 minutes
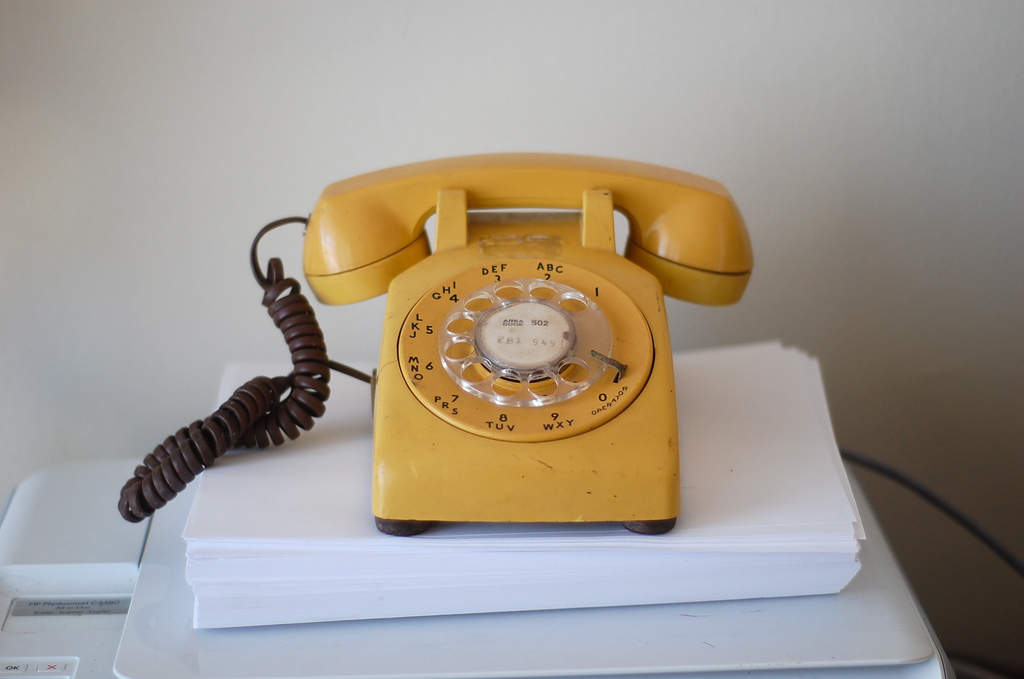
In case you found this in an emergency, here is all of the code you need to hang up all currently in progress, ringing or queued phone calls:
In order for this to work, make sure you grab your Account SID and Auth Token from your Twilio Console and set them as environment variables:
Why would I need to do this?
Sometimes when testing your code to make phone calls with Twilio, you can end up accidentally making too many calls. This situation has happened to me many times. As my friend Brodan said in a similar blog post: “Making too many calls too quickly is dangerous because it can use up a lot of Twilio credit very quickly or get your account suspended due to unintentional spam.”
Let’s take this code and turn it into a shell command that we can run in the terminal whenever too many phone calls are made unintentionally.
Setting up our environment
To separate dependencies from other Python apps running on your machine, we will need a virtual environment. I love using Virtualenvwrapper because it makes working with virtual environments simple.
Install it with the following terminal command:
Now create a directory to keep all of your virtual environments, and set this as an environment variable for Virtualenvwrapper to use. I created a directory called Envs
in my home directory:
Add that last line to your .bashrc
or other shell startup file, to make sure that virtualenvwrapper works every time you need to use it. Otherwise you will have to run it manually for each new terminal session.
With virtualenvwrapper
installed, create a virtual environment:
And install Twilio inside of that virtual environment:
Now add this code to a file called kill_calls.py
:
You can run this script when you need to hang up Twilio phone calls. Think of it as your panic button to avoid rickrolling 200 people while they are trying to sleep.
How do I know this works?
We can test this script out by writing some code that will make a bunch of phone calls. For this test you will need a Twilio phone number. You can buy a phone number here.
Create and open a file called make_calls.py
and add the following code:
Now run that code first (don’t forget to replace your phone number and Twilio number) and then the code you wrote to kill all of the calls:
You’ll never have to write this code again
Manually running this Python script every time you mistakenly make 500 phone calls to your users would be tedious and waste precious time. We can turn this program into a shell script that you can use at the drop of a hat.
Open your .bashrc
, or other shell startup file, again, and add the following line:
Don’t forget to use the correct path to kill_calls.py
. This will give you a shell command that you can run any time instead of scrambling to find the right code.
You’ll also want to make sure you have your Twilio authentication credentials set as environment variables permanently by adding the export lines we used before to your .bashrc
:
Hopefully, you won’t have any horror stories about accidentally calling your users in the middle of the night while testing code. Feel free to reach out for any questions or to share your own stories:
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.