Make Phone Calls Using PHP and Twilio Programmable Voice
Time to read: 3 minutes
There are so many things that you can do in PHP, including creating websites, building command-line tools, generating images, encrypting and decrypting data, and scraping websites.
But did you know that PHP can also make phone calls? Okay, not on its own it can't, but with the help of Twilio Programmable Voice, it can! In this tutorial, you're going to learn how.
Prerequisites
To follow along with this tutorial you will need the following:
- PHP 8.1.
- Composer globally installed.
- A Twilio account. If you are new to Twilio click here to create a free account.
- A Twilio phone number.
- A phone that can make and receive phone calls.
Set up your environment
The first thing you need to do is to create a project directory and change into it. To do that, run the following commands.
Next, create a new file named .env to store the environment variables which the application requires. Then, in the new file paste the following code.
Install the required dependencies
Next, you need to install the dependencies which the code requires. There are just two:
The Twilio PHP Helper Library | Simplifies interacting with all of Twilio's APIs in PHP. |
---|---|
PHP Dotenv | Makes environment variables defined in `.env` available to PHP's `$_ENV` and `$_SERVER` superglobals. |
To install them, run the command below.
Set your Twilio credentials
The next thing you need to do is to retrieve your Twilio Auth Token, Account SID, and phone number. To do that login to the Twilio Console, copy the details from the "Account Info" panel and paste them in place of <TWILIO_ACCOUNT_SID>
, <TWILIO_AUTH_TOKEN>
, and <TWILIO_PHONE_NUMBER>
respectively in .env.
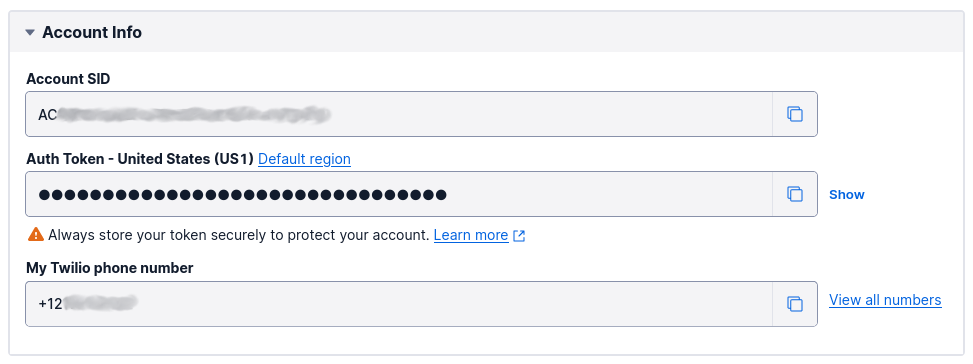
Create a TwiML Bin
Now, you need to create the phone call instructions. These must be in TwiML format and available via a publicly accessible URL.
If you're not familiar with it, TwiML (the Twilio Markup Language) is a subset of XML which tells Twilio what to do, such as when you make or receive a phone call or SMS.
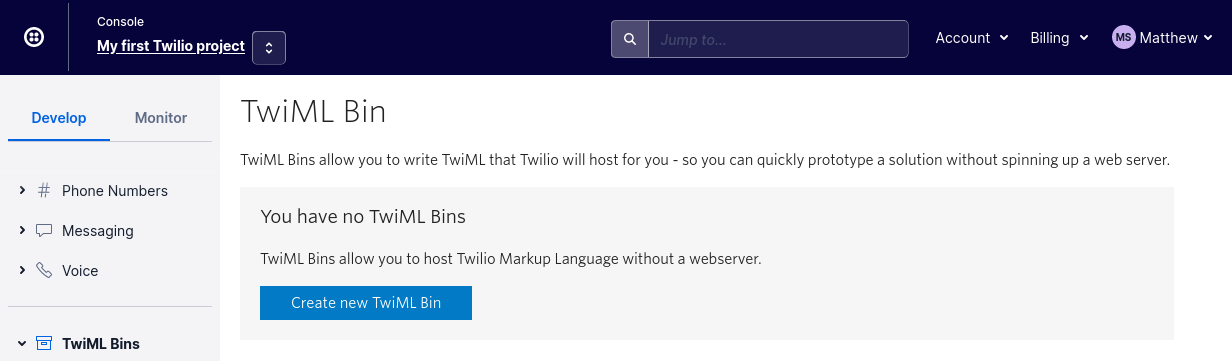
You don't need to host the file yourself, as you can host it for free in a TwiML Bin. To do that, navigate to the TwiML Bins section of the Twilio Console and click "Create new TwiML Bin".
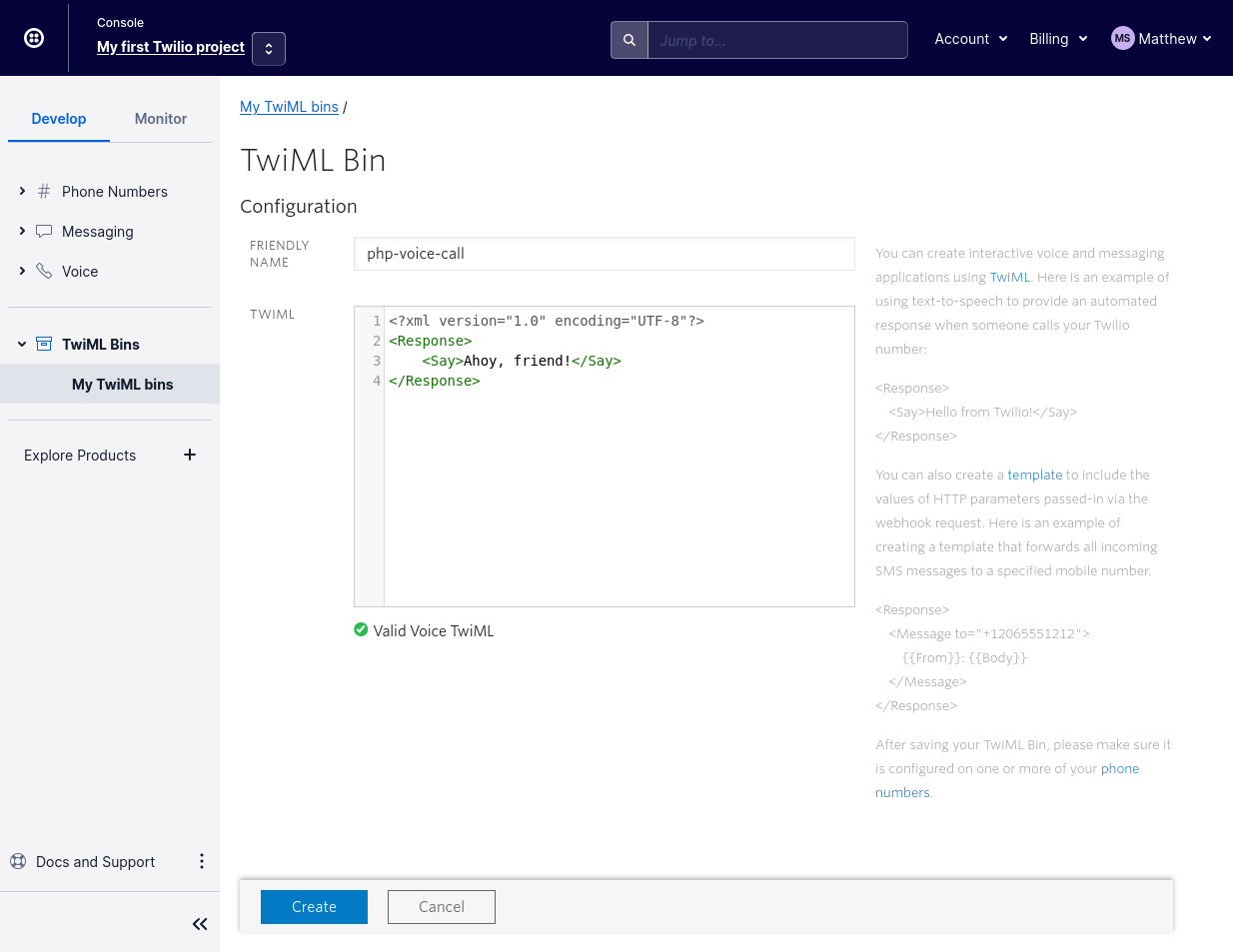
Then, in the "FRIENDLY NAME" field, add a human-friendly name, such as php-voice-call
, and in the TwiML field add the TwiML below.
The TwiML instructions you used tell Twilio to say "Ahoy, friend" when the call is answered.
With that done, click "Create". With the TwiML Bin created, copy the URL and paste it into .env in place of <TWIML_BIN_URL>
.
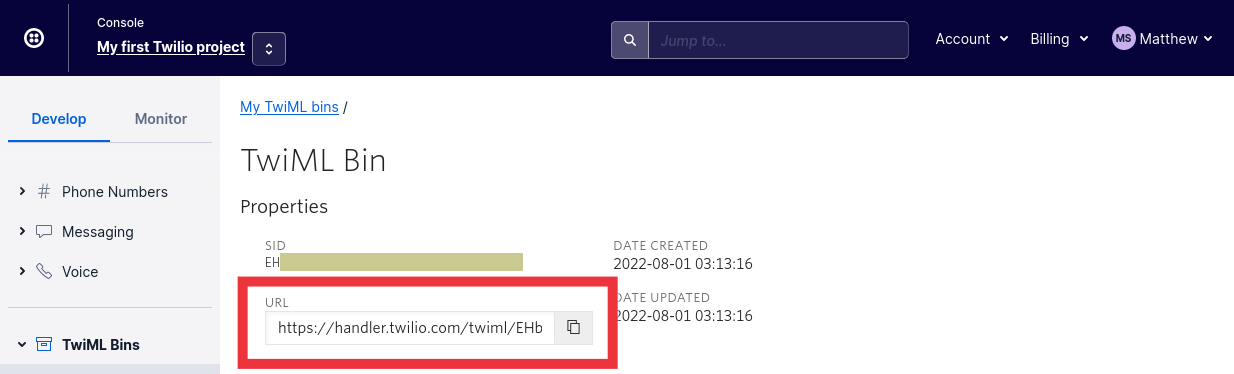
Make a Phone call in PHP
Now, it's time to write the code that will make the phone call. To do that, create a new PHP file named voice-call.php, and in the new file paste the following code.
The code starts off by importing all the required libraries before using PHP Dotenv to load the variables defined in .env as variables in PHP's $_ENV
and $_SERVER
superglobals.
After that, it initialises a new Twilio Client
object, which provides the functionality for interacting with Twilio's REST APIs. Then, via$twilio->calls->create()
, it makes a request to the Programmable Voice API to make a phone call, passing to the request:
- The recipient's phone number.
- The Twilio phone number to call from.
- The publicly accessible URL with the TwiML call instructions.
Assuming that the call was successful, the call's SID will be printed to the terminal and the phone call will be made.
Test the code
Now, it's time to test the code. To do that, run the command below.
Within a few seconds, you should receive a phone call on your mobile phone and hear "Ahoy, friend".
That's how you make phone calls using PHP and Twilio Programmable Voice
There's not a whole lot to the essentials of making a phone call in PHP Twilio Programmable Voice. To be fair, there is a lot more functionality that you can draw upon thanks to the incredible power of TwiML, but this is all you need to get started.
Do you prefer Python over PHP? Then, check out this post from Miguel Grinberg.
Otherwise, check out the TwiML documentation to learn more. I can't wait to see what you build!
Matthew Setter is a PHP and Go editor in the Twilio Voices team, and a PHP and Go developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. You can find him at msetter@twilio.com. He's also on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.