Monitor chess progress using Twilio Serverless and SMS
Time to read:
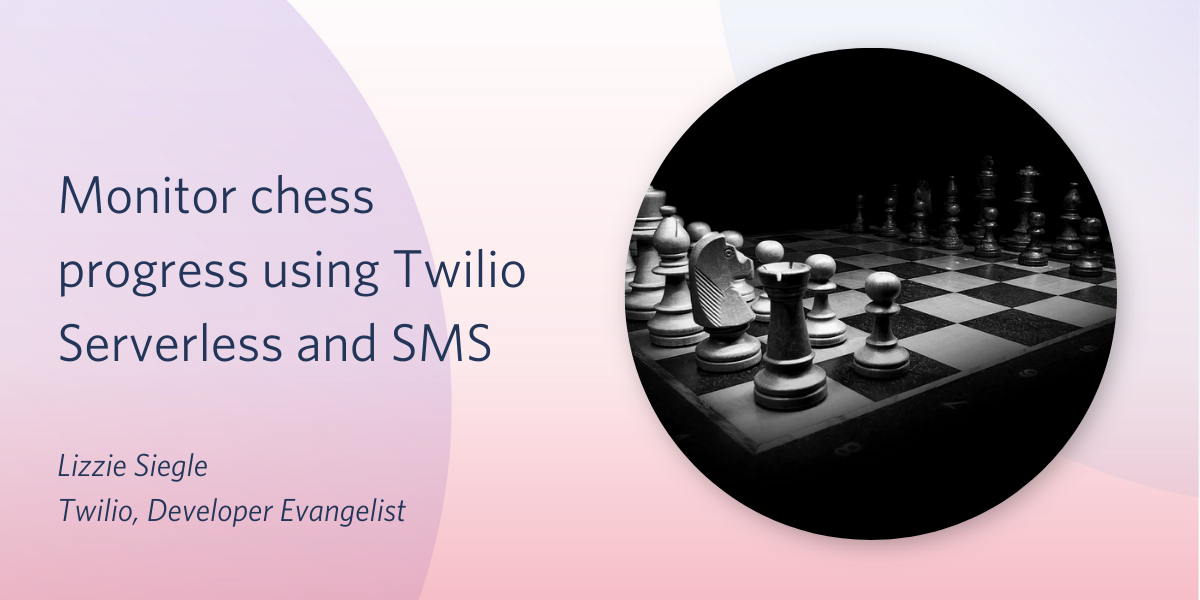
After the Queen's Gambit came out, I, alongside many, began playing a lot of chess and completing chess puzzles. This tutorial will go over how to build a chess progress monitor using the chess.com API, Twilio Functions, and the Twilio Serverless Toolkit. Text a chess.com username to +14243260196 (for example below, mine is lizziepika) to see it in action.
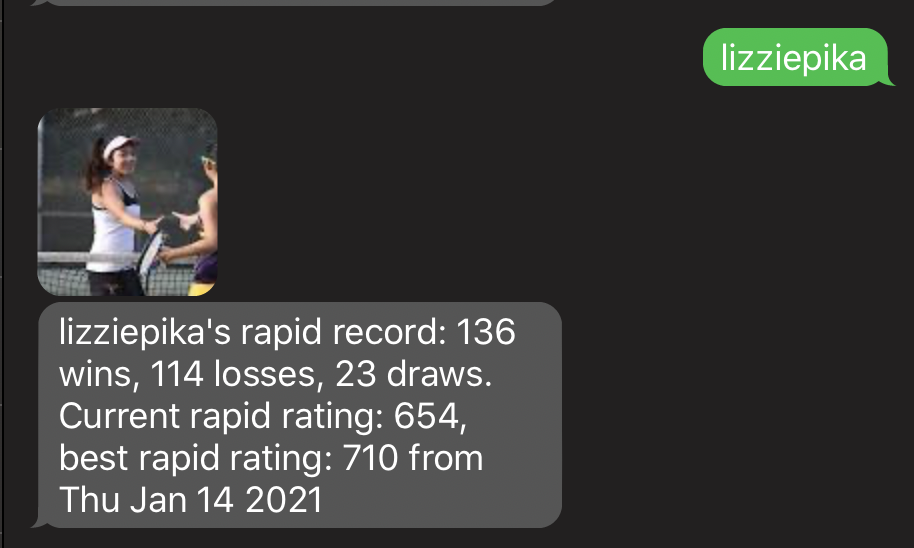
Prerequisites
- A Twilio account - sign up for a free one here and receive an extra $10 if you upgrade through this link
- A Twilio phone number with SMS capabilities - configure one here
- Postman (you could alternatively make cURL requests from the command line)
- Node.js installed - download it here
Make a chess.com API Request
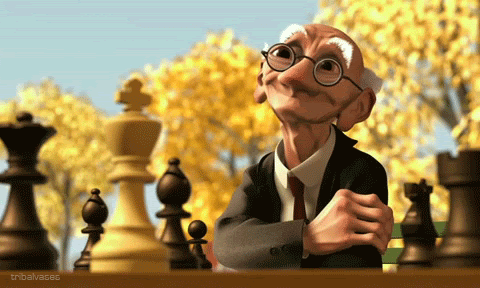
Chess.com provides a nice read-only published data API which makes available public data from the site, such as player data, game data, and club or tournament information.
You can look over different API endpoints offered. To get someone's profile information, the URL would look like https://api.chess.com/pub/player/{insert-chess.com-username}
. Open Postman and paste that URL (replacing it with a chess.com username--if you need one, you can use lizziepika) into the URL bar.
Click Send
to hit it with a GET request to see the following data returned:
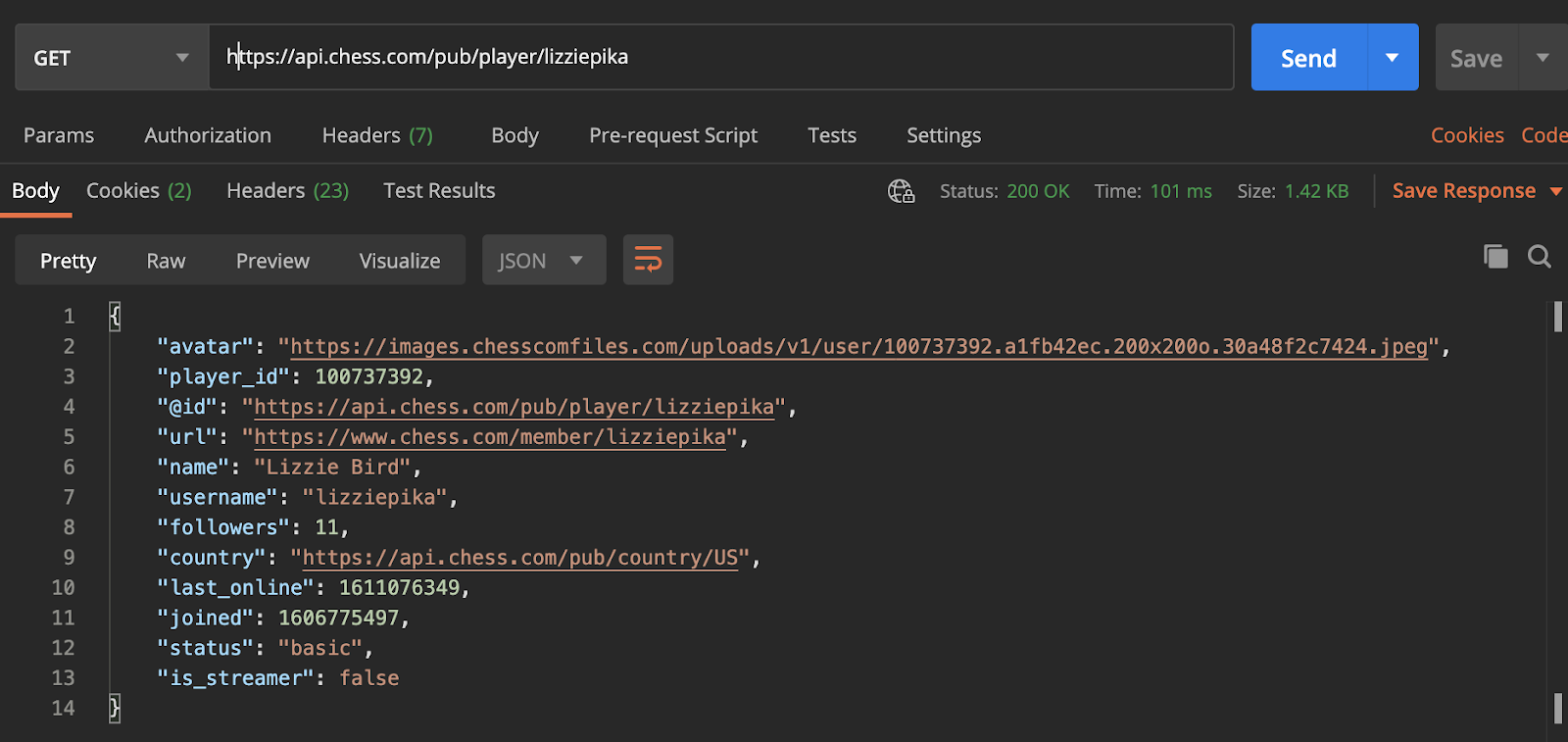
To get a player's current games, the URL would be: https://api.chess.com/pub/player/{insert-chess.com-username}/games
. As a big sports fan, I personally am a sucker for statistics. The URL to retrieve the stats would look like https://api.chess.com/pub/player/${insert-username}/stats
and return data as shown below:
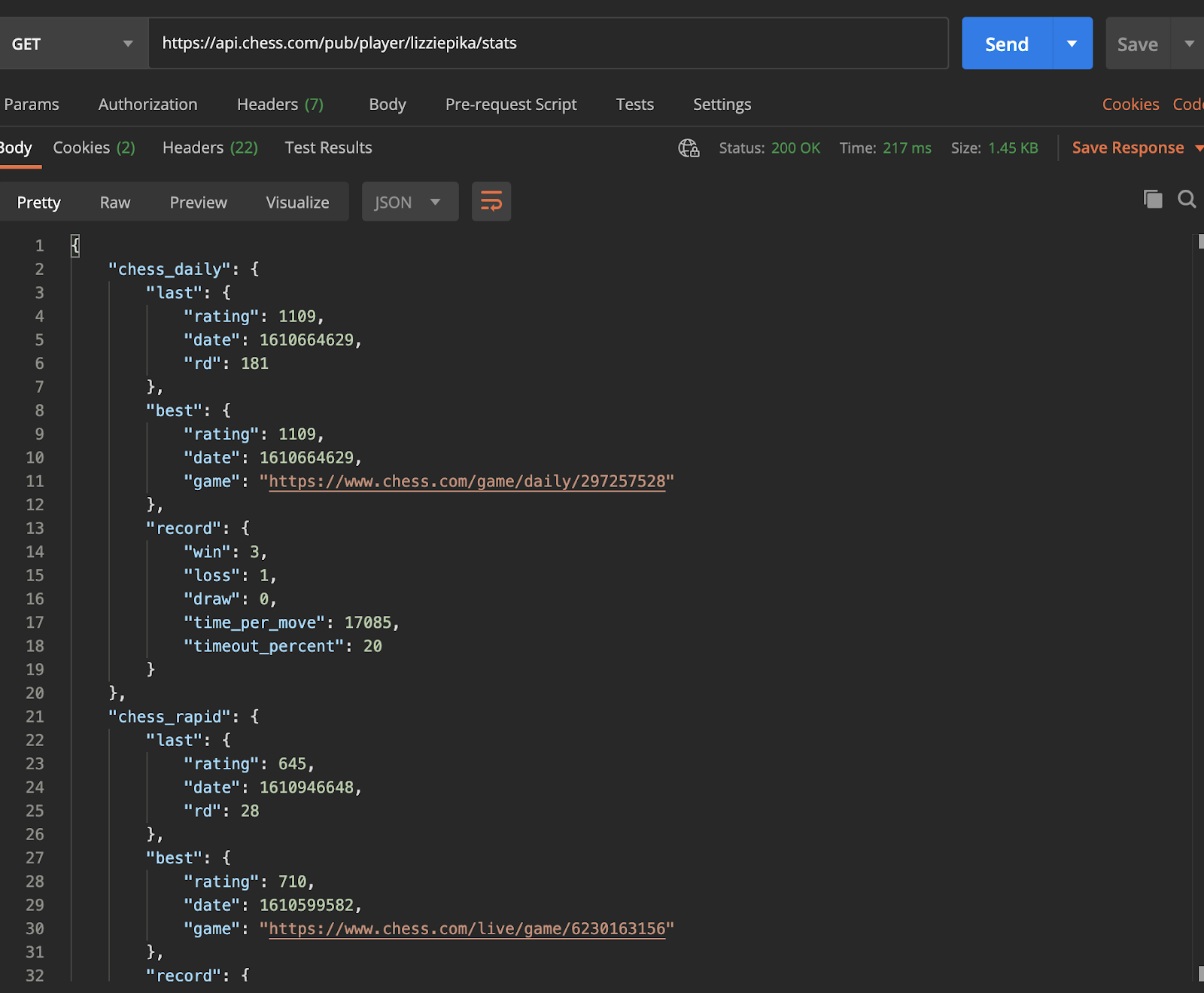
You can see more chess.com API endpoints here.
Get Started with the Twilio Serverless Toolkit
The Serverless Toolkit is CLI tooling that helps you develop locally and deploy to Twilio Runtime. The best way to work with the Serverless Toolkit is through the Twilio CLI. If you don't have the Twilio CLI installed yet, run the following commands on the command line to install it and the Serverless Toolkit:
Afterwards, create your new project and install our lone requirement got
to make HTTP requests in Node.js by running:
Make a Twilio Function with JavaScript
Use cd
to change into the functions
directory and make a new file called chess-open-to-other-usernames.js containing the following code:
This code imports got
, makes a Twilio Messaging Response object, creates a variable username
from the inbound text message users will text in and variables for the stats
and profile
endpoints of the chess.com API, parses the responses from the endpoints (like we saw using Postman above), and finally parses the responses to handle data from rapid chess games.
We use a try..catch
block to check that the user (and link we are making a HTTP request to) exist, and if not, a message is returned saying so. Else, we parse the rapid chess record for information such as wins, losses, draws, best rating, and the time of the best rating which is formatted with toDateString()
. We return a text message containing that data as well as the account's avatar image.
You can view the complete app on GitHub here.
Configure the Function with a Twilio Phone Number
To open up our app to the web with a public-facing URL, go back to the chess-api root directory and run twilio serverless:deploy
. You should see this at the bottom of your terminal:
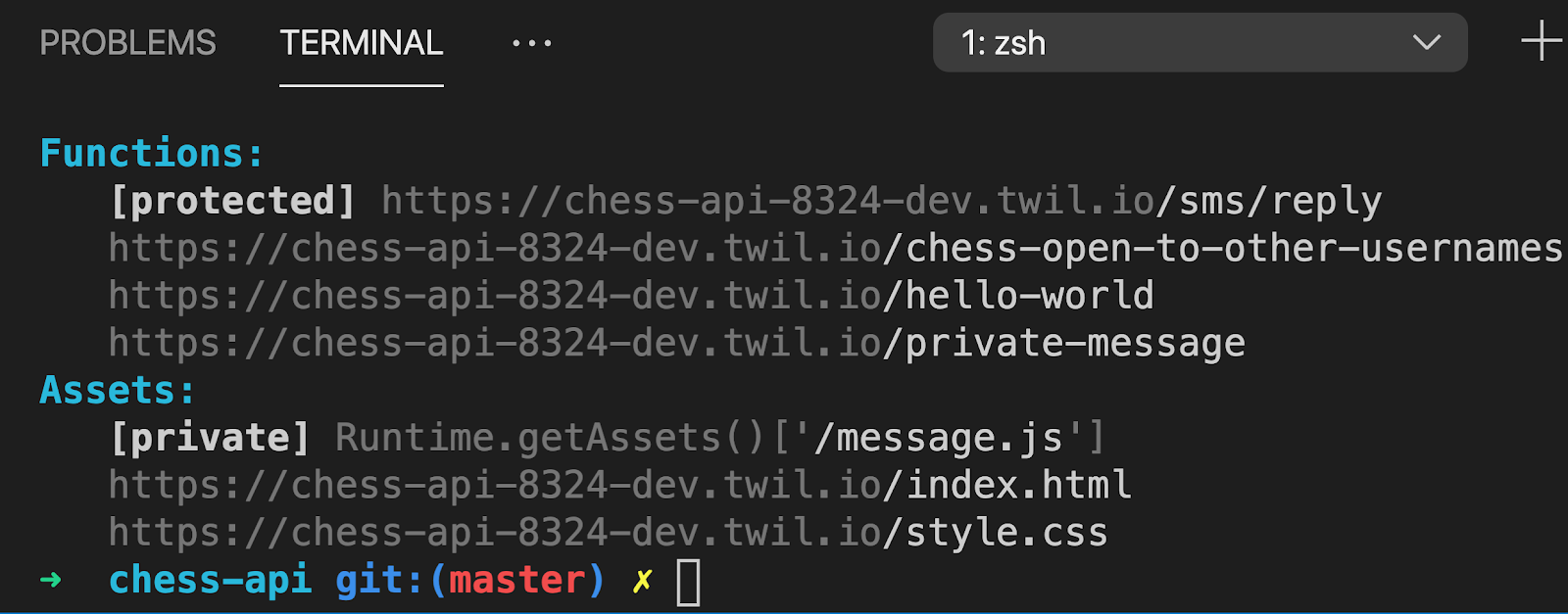
Grab the Function URL corresponding to your app (the one that ends with /chess-open-to-other-usernames) and configure a Twilio phone number with it as shown below: select the Twilio number you just purchased in your Twilio phone numbers console and scroll down to the Messaging section. Paste the link in the text field for A MESSAGE COMES IN webhook making sure that it's set to HTTP POST.
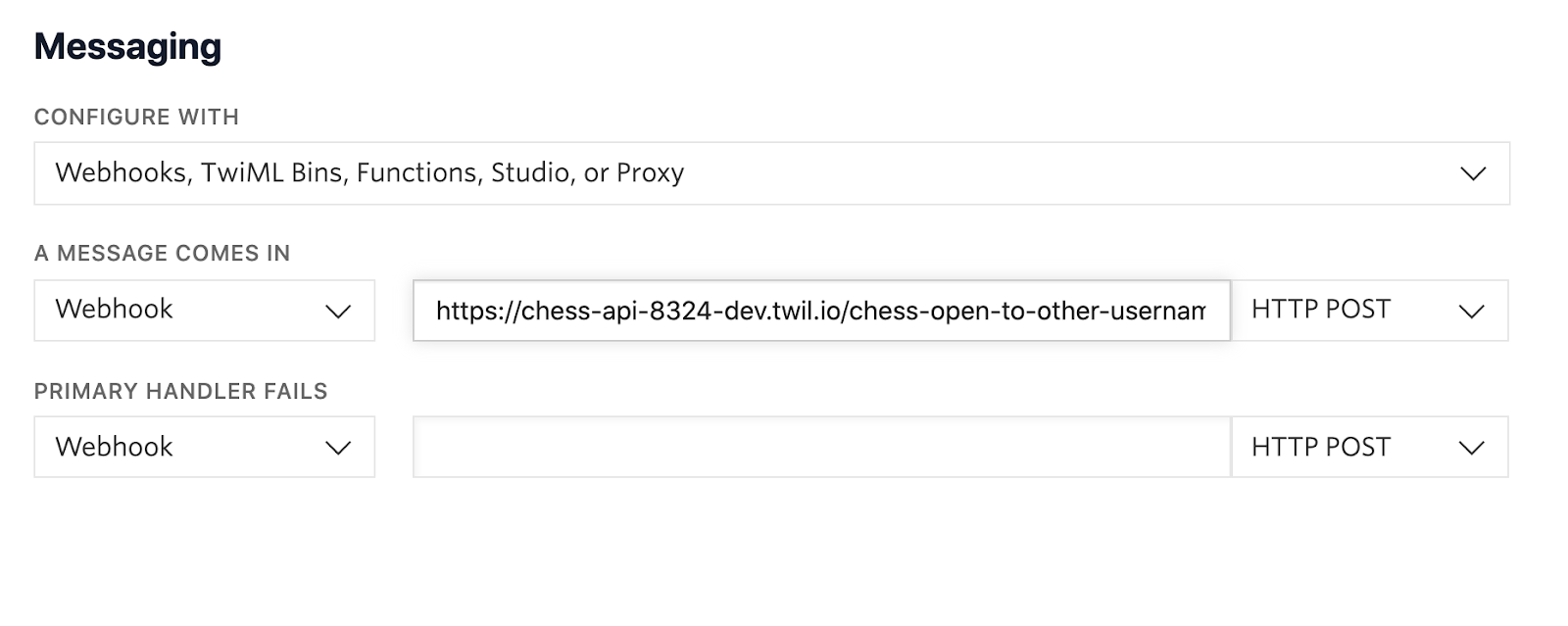
When you click Save at the bottom, the new Twilio Functions UI will update the Webhook to the Function like so:
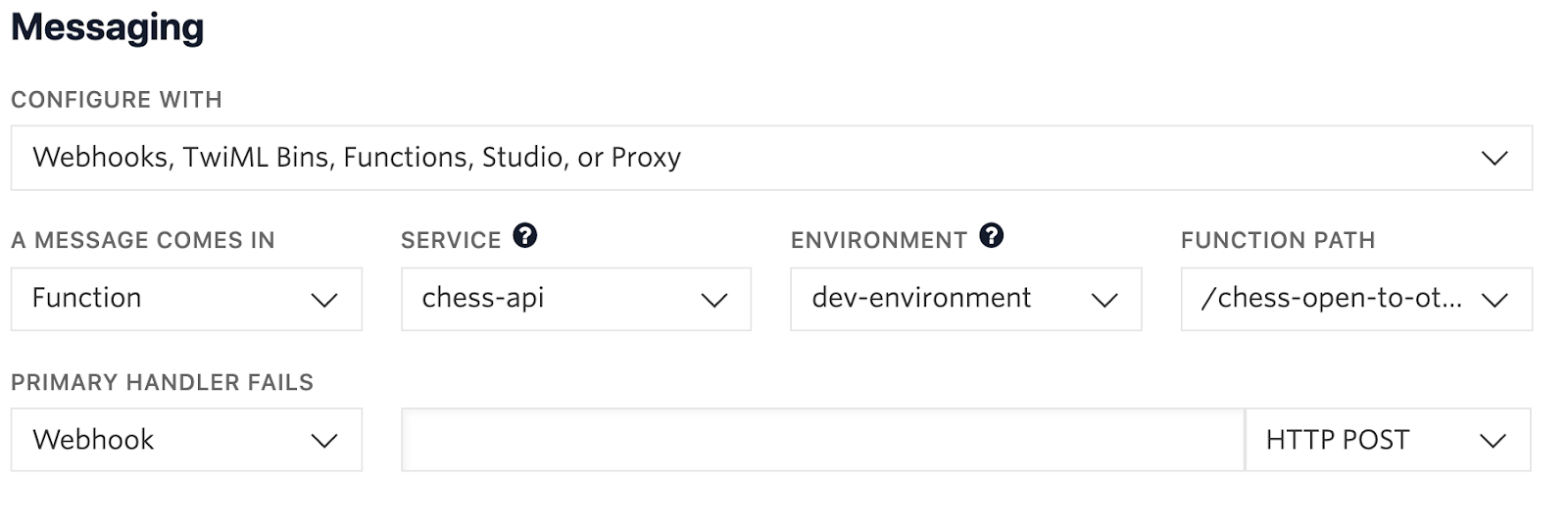
The Service is the Serverless project name, environment provides no other options, and Function Path is the file name. Now take out your phone and text a chess.com username to your Twilio number.Share the Twilio number and instructions with friends so they can stay up-to-date on your progress!
What's Next for Twilio Serverless, APIs, and Chess?
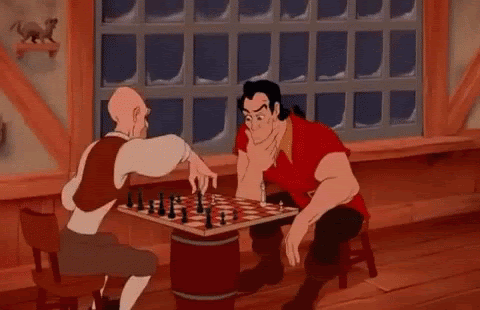
Twilio's Serverless Toolkit makes it possible to deploy web apps quickly, and Runtime seamlessly handles servers for you. Let me know online what you're building with Serverless, or if you want to start a chess game!
- Twitter: @lizziepika
- GitHub: elizabethsiegle
- Email: lsiegle@twilio.com
- Chess + code streams: twitch.tv/lizziepikachu
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.