Send Images and Other Media via WhatsApp Using Node.js
Time to read: 2 minutes
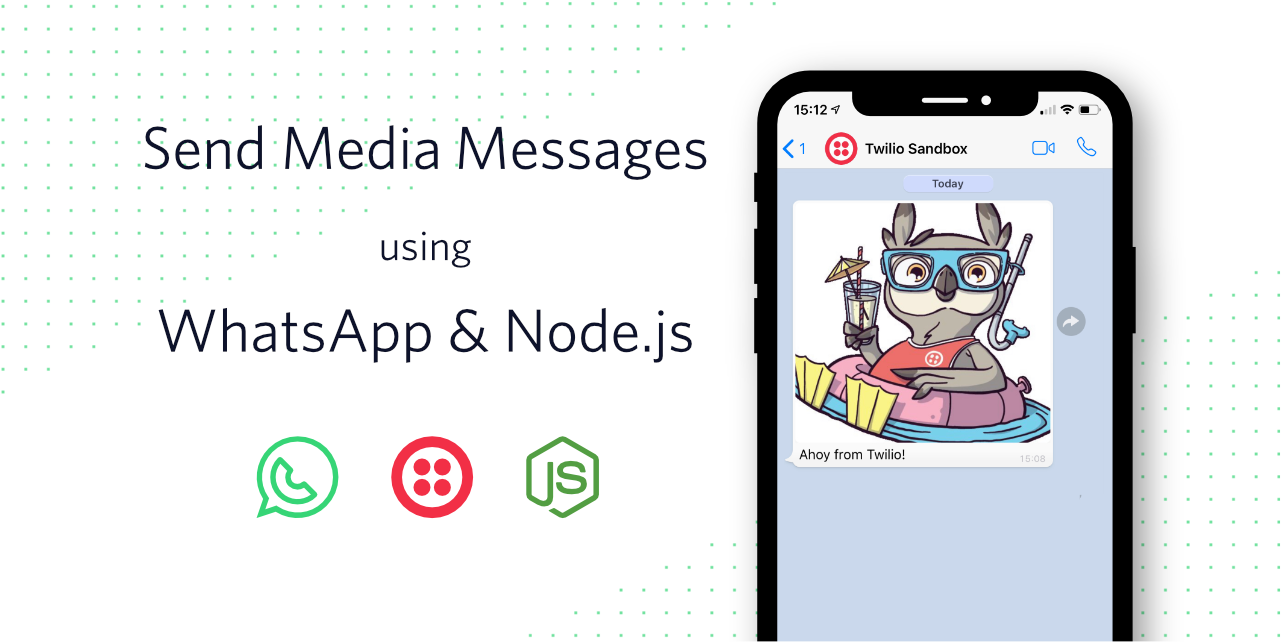
The Twilio API for WhatsApp allows you to not only send text-based messages to WhatsApp numbers but also send and receive media messages. This allows you to send images (JPG, JPEG, PNG), audio files and even PDFs up to 5MB. Let's see how we can do this using Node.js.
If you prefer watching a video tutorial instead, check out this video on our YouTube channel:
First Things First
Before we get started, make sure you have:
- A Twilio account (sign up for free)
- Node.js and a package manager like
npm
installed - The WhatsApp Sandbox Channel installed (learn how to activate your WhatsApp sandbox)
- Set your Account SID and Auth Token as environment variables
If you want to try this out make sure to set up a new project by running:
Sending Your First Media Message
Install the twilio
helper library by running:
Create a new file called index.js
and place the following code in it:
This will initialize a Twilio Client using the twilio
helper library and then create a new message to your WhatsApp number. Make sure to change the WHATSAPP_SANDBOX_NUMBER
and YOUR_NUMBER
with the respective numbers and feel free to change the value of mediaUrl
with a URL to any other image, sound snippet or PDF.
Afterwards run your code:
You should receive a message with your media and the text you sent.
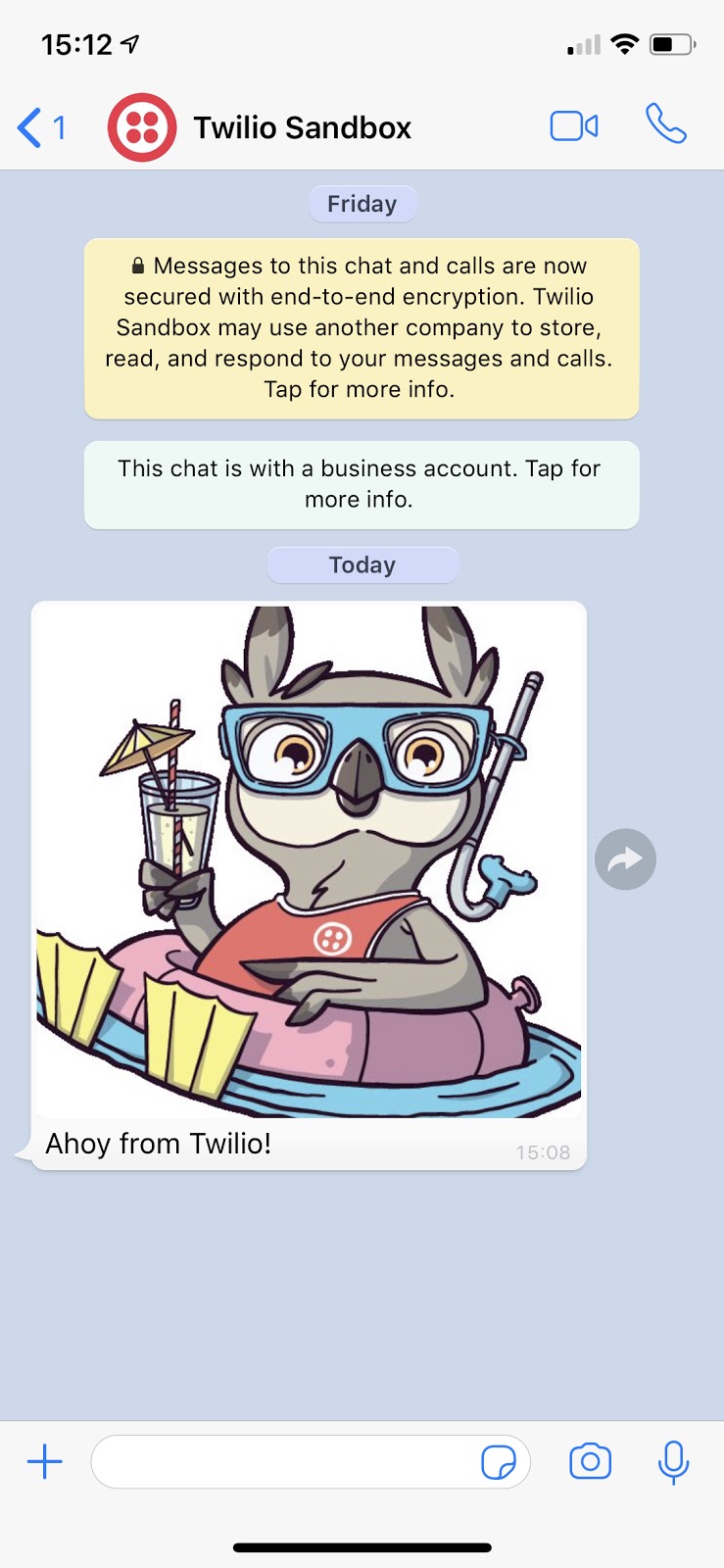
What's Next?
There are some differences between MMS and WhatsApp media messages. Media messages can only be sent to WhatsApp users if there is an active session
established. Sessions are created when a user responds to a template message or the user initiates the conversation by sending in a message. A session lasts for 24h since the last message from the user. WhatsApp media messages also do not support some of the file types that MMS does. For more information on file type support check out the FAQs.
If you want to check out other things that are possible with the Twilio for WhatsApp API, check out the following posts:
- How to Send a WhatsApp Message with JavaScript and Node.js
- Build WhatsApp Bots with Twilio Autopilot
- Twilio WhatsApp API and Flex
- Building an npm search bot with WhatsApp and Twilio
- Emoji translations with the Twilio API for WhatsApp and Node.js
I would love to see what you build. Feel free to ping me and tell me about it or let me know if you have any questions:
- Twitter: @dkundel
- Email: dkundel@twilio.com
- GitHub: dkundel
- dkundel.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.