How to Send SMS Without a Phone Number using Alphanumeric Sender IDs and Node.js
Time to read: 5 minutes
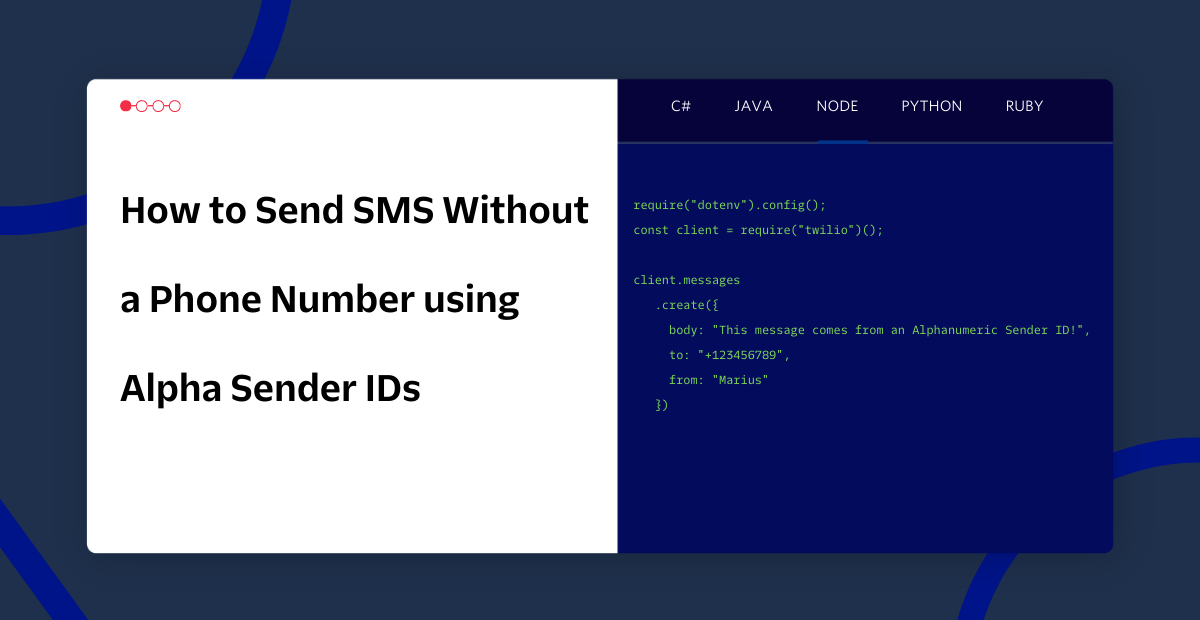
Sending SMS is a convenient way to notify your users about time-sensitive matters. These messages are often closely related to an action the user just triggered. For example, receiving a One-Time-Password (OTP) when transferring money from a banking app.
In these situations, the user probably doesn’t mind which number sent the message. However, in other cases — especially when you notify users "out of the blue" — you might prefer to display your company name as the sender. Alphanumeric Sender IDs allow you to do exactly that.
When a user receives a message from an Alphanumeric Sender ID, it looks like this.
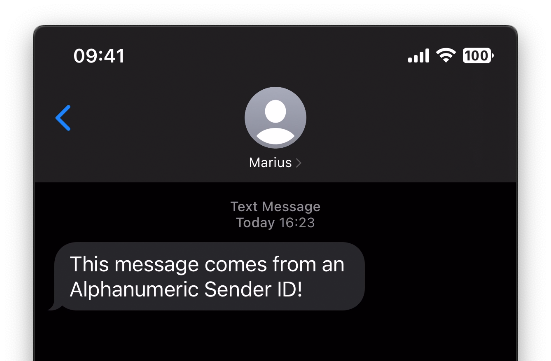
In this case, "Marius" sent the message. You can see that the ID takes the place of the phone number for the contact in the iOS messaging app.
What you'll need to send an SMS from an number with an Alphanumeric Sender ID
- You will need an upgraded Twilio account.
- The code at the end of this post uses JavaScript, so make sure your Node.js environment is set up.
What differentiates Alphanumeric Sender IDs
Alphanumeric Sender IDs behave differently from phone numbers and have some benefits:
- Your business is instantly identifiable. The recipient doesn't have to have your phone number in their contacts to recognize it, which can improve your open rate
- Alphanumeric Sender IDs can be used across many countries without requiring a dedicated phone number in each country. This might be especially helpful if you need a regulatory bundle to buy a number in the first place
- There is no charge to use an Alphanumeric Sender ID in most countries
- It is impossible to reply to messages sent from an Alphanumeric Sender ID. This means you don't need to handle inbound messages
Overall, however, Alphanumeric Sender IDs are handy for the right use cases. We'll spend the rest of this post setting up our Twilio account to send from an Alphanumeric Sender ID and showing you how to do so with Node.js.
How to set up your Alphanumeric Sender ID
We recommend sending from an Alphanumeric Sender ID via a Messaging Service. Messaging Services have a lot of benefits, including falling back to a long code number if you try to send to a country that doesn't support Alphanumeric Sender IDs. Setting up your Messaging Service only takes a couple of minutes, so let's configure one now.
Create and configure a Messaging Service
To create a Messaging Service, open the Twilio Console and, under the Explore Products > Messaging > Services. Click Create Messaging Service to start the wizard.
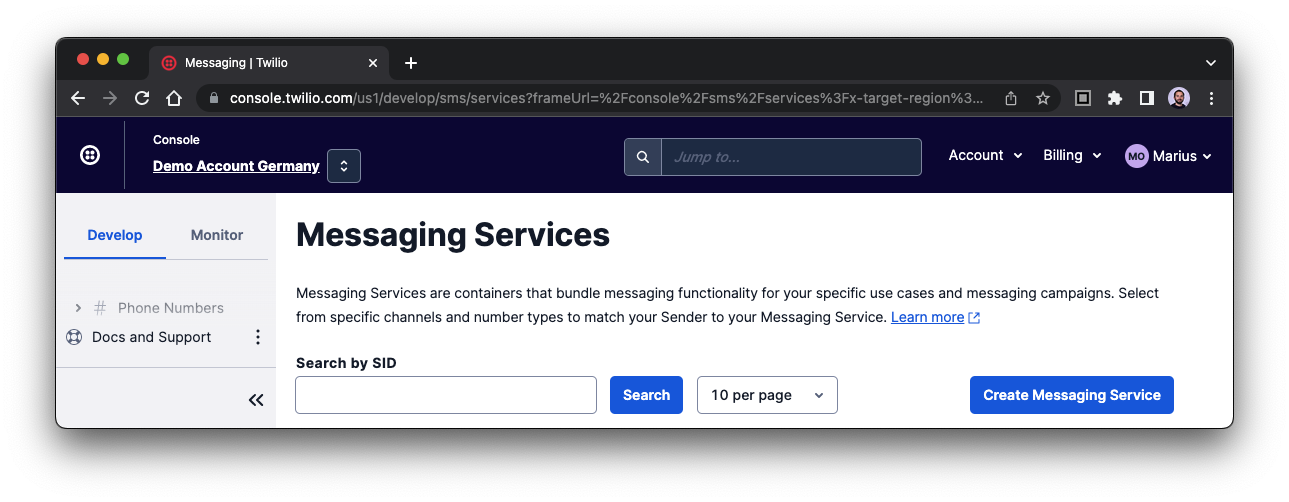
The first step is to give your new Messaging Service a name, and then confirm by clicking Create Messaging Service.
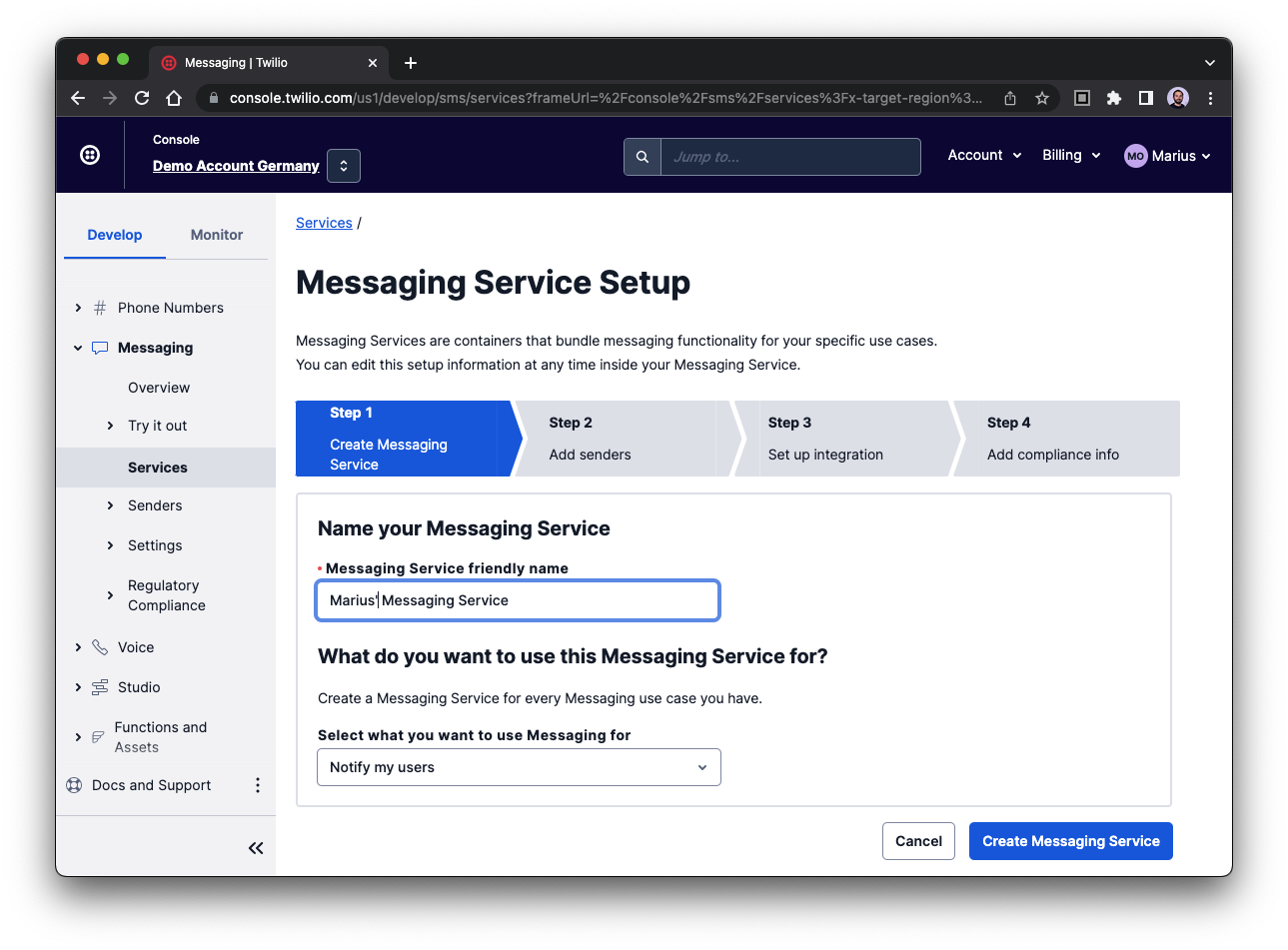
Next, add an Alphanumeric Sender ID to your Messaging Service by clicking Add Sender, setting the Sender Type to "Alpha Sender" in the Add Senders popup, and clicking Continue.
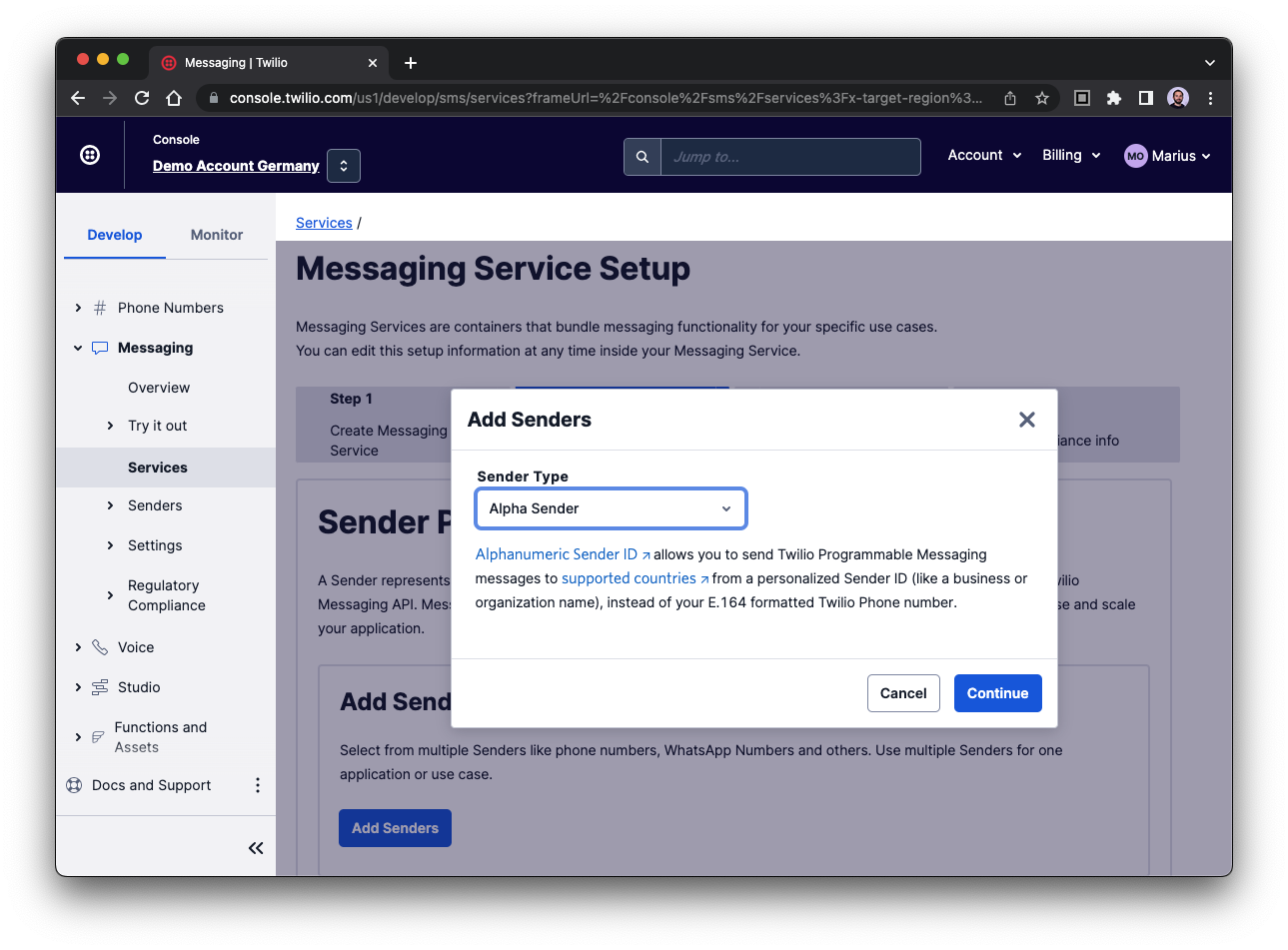
Then, enter an Alphanumeric Sender ID of your choosing. This will appear in recipients' messaging apps, as seen in the example at the beginning.
Be aware that some naming rules apply
- Names are limited to 11 characters
- At least one character must be a letter
Now, click Add Alpha Sender, and you will see that the Sender Pool contains your new Alphanumeric Sender ID. Now you can confirm by clicking Step 3. Set up integration.
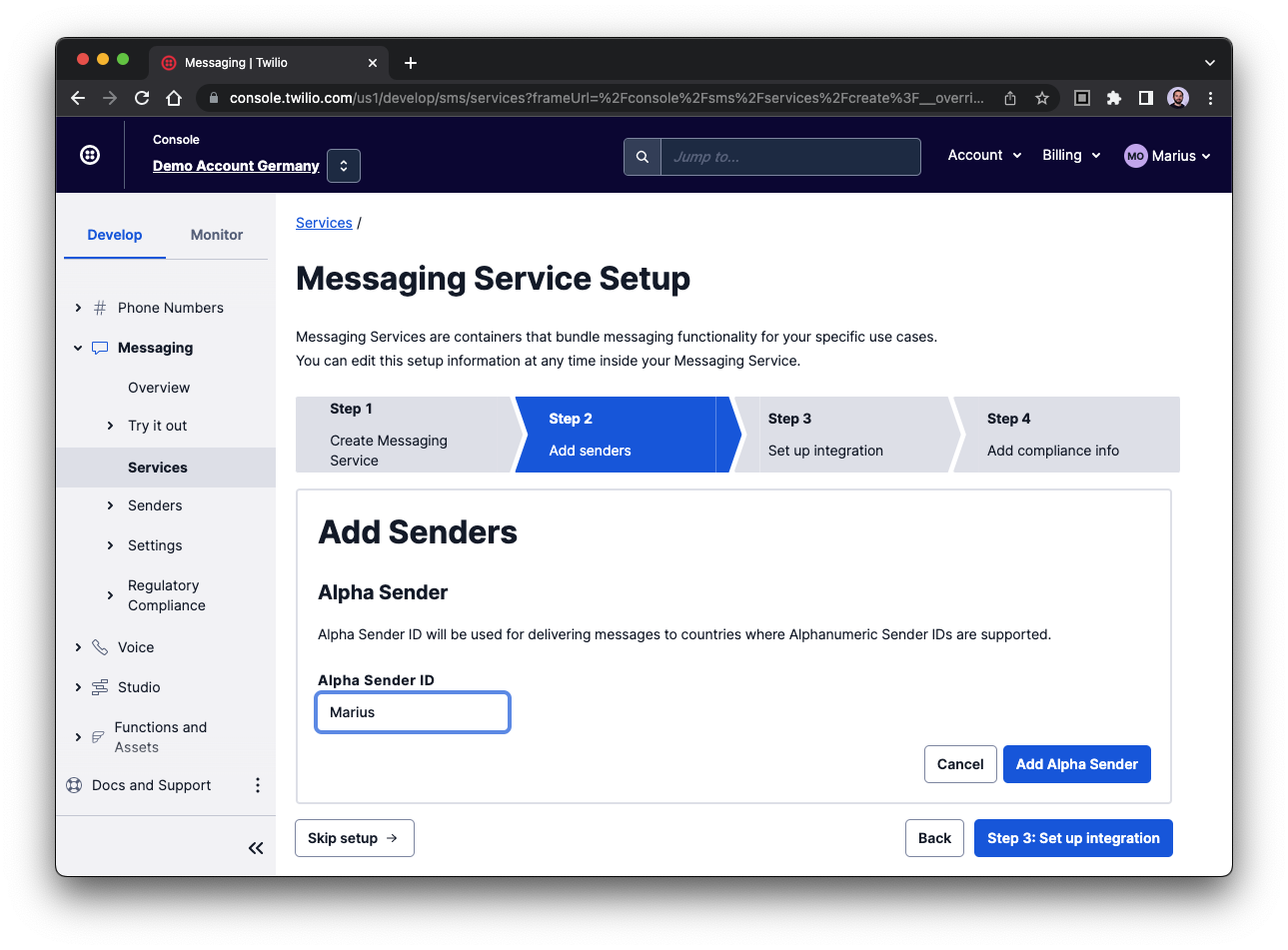
The next step is to set up how your Messaging Service handles incoming messages. Since you are only dealing with Alphanumeric Sender IDs that can't receive incoming messages, you can move straight on to the next step, by clicking Step 4. Add compliance info.
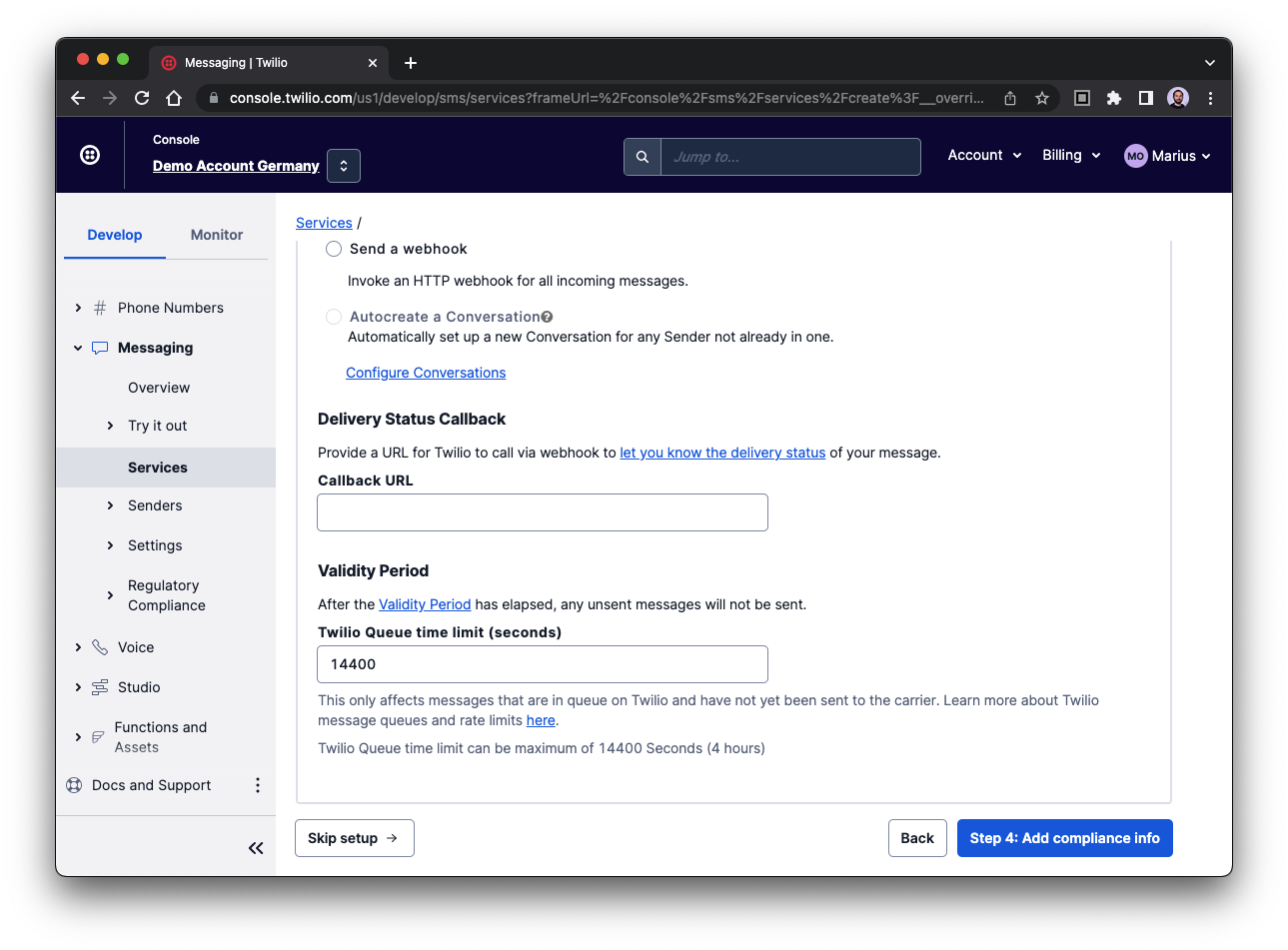
If you send application-to-person SMS in the USA, you will be subject to A2P10DLC rules. I won't cover that here, but if that applies to you, you should learn more about and register to use A2P 10DLC messaging. Click Complete Messaging Service Setup, then View my new Messaging Service.
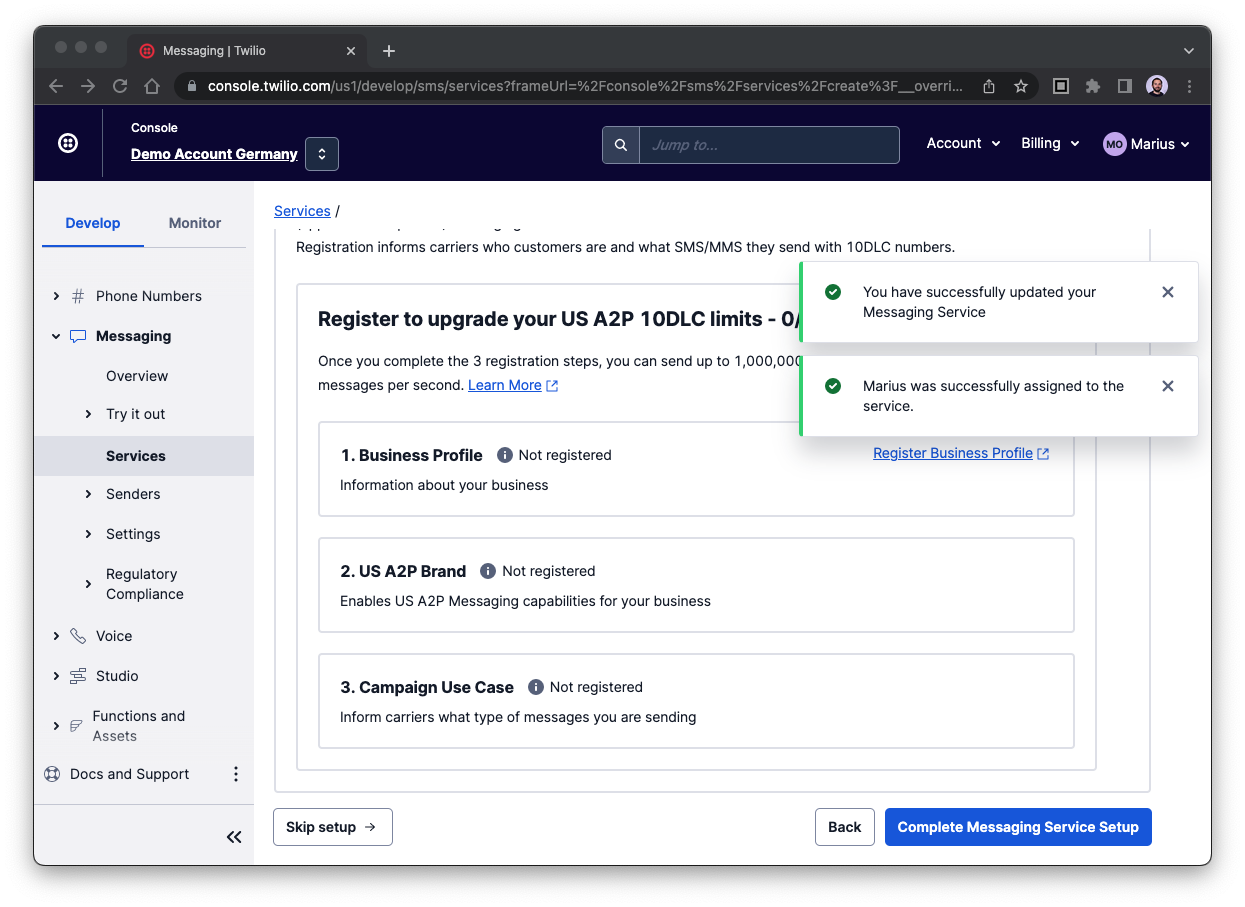
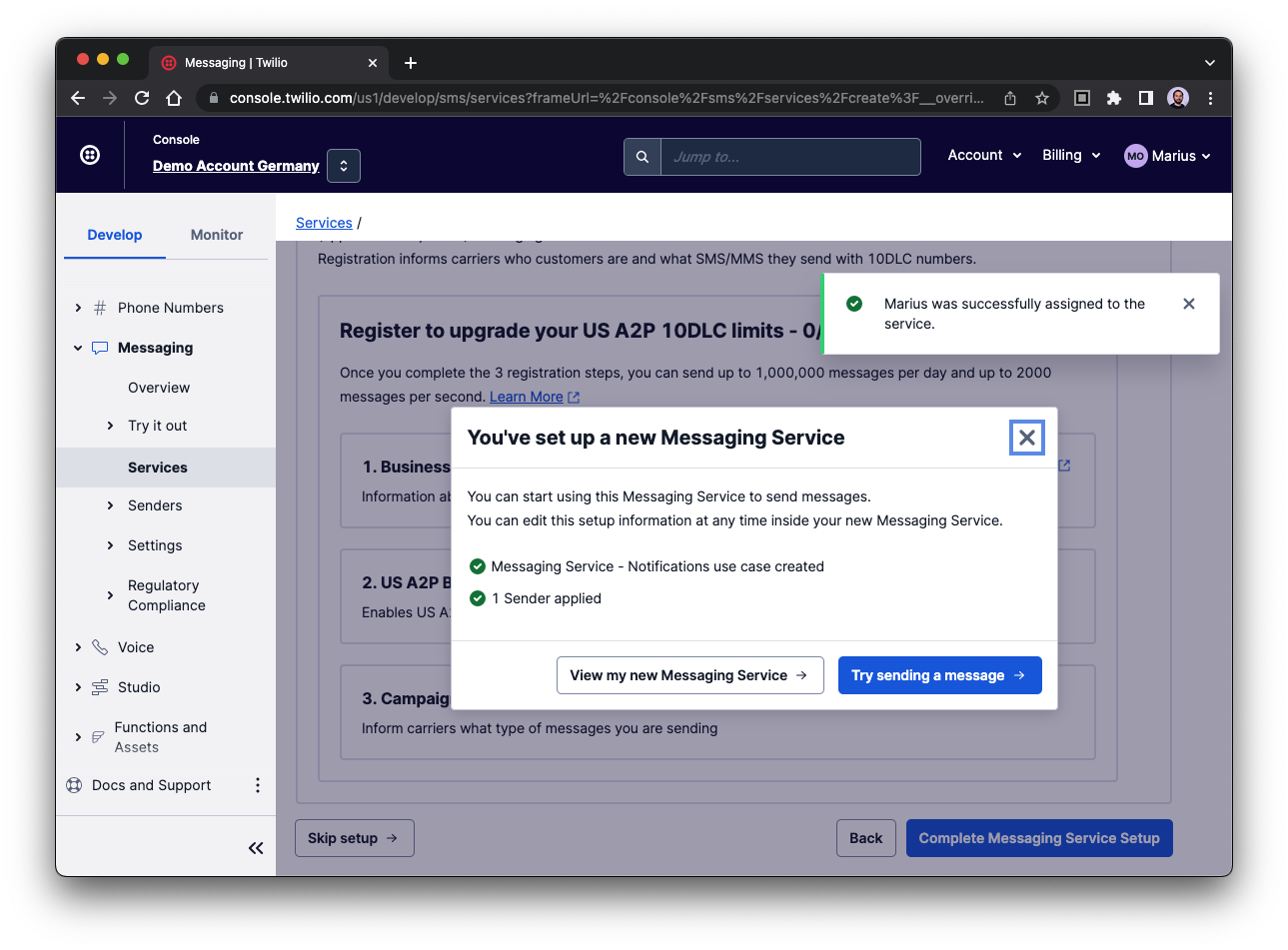
This page will reveal the SID of the service. Make sure you save it for future use.
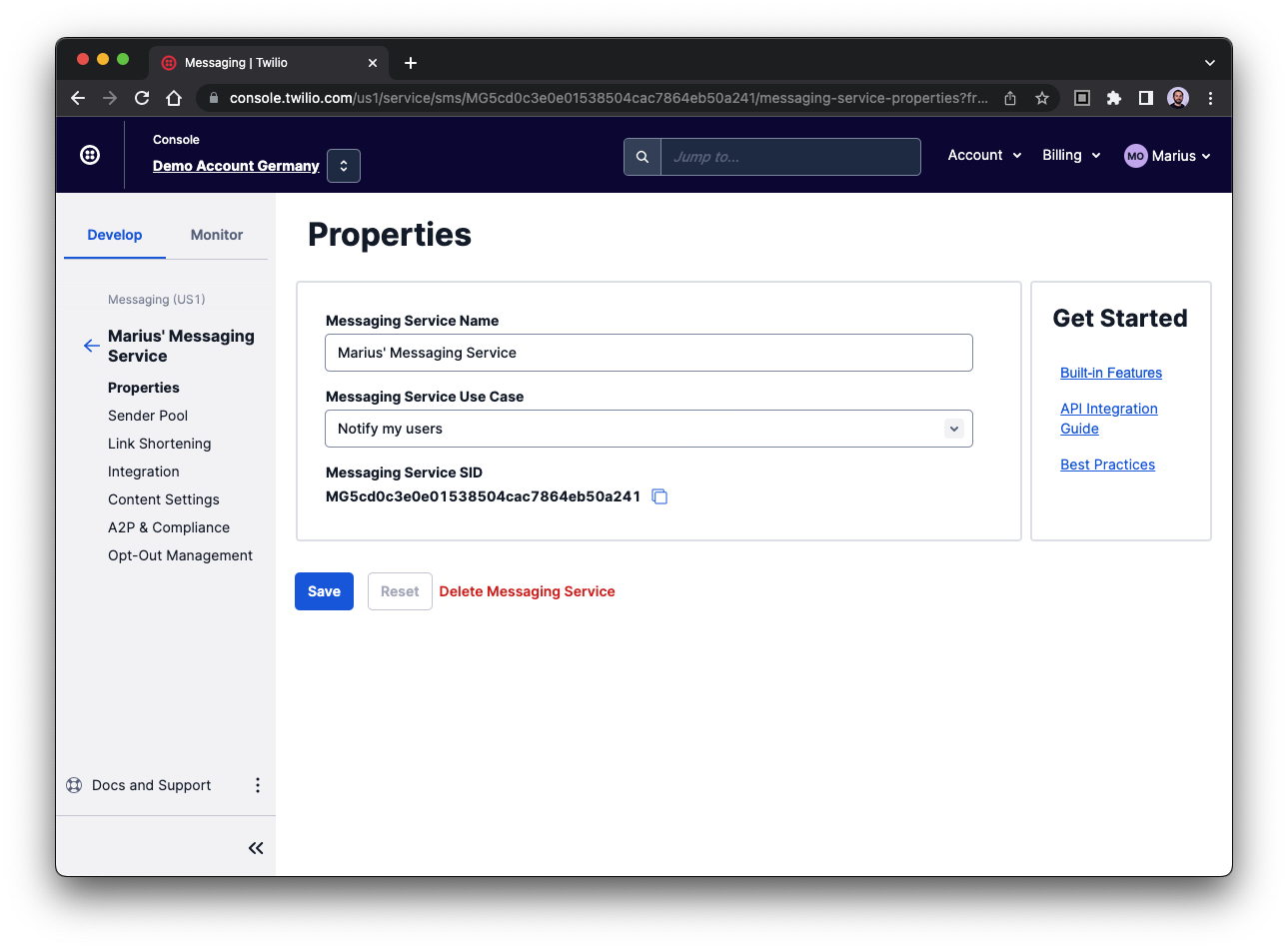
Send an SMS message from an Alphanumeric Sender ID with Node.js
Now that your Twilio account is set up to send messages from your Alphanumeric Sender ID, it's time to write some code that does this. If you just want to see the final result, the code is also available on GitHub.
Create a new Node.js project
Create a new directory for this project and then change into that directory by running the commands below:
Inside the directory run the following command and accept all the default values that the wizard suggests:
With those two dependencies in place, you need a file for the code and a file for your Twilio credentials. Create a new file called send-alpha.js
and another called .env
by running the following Unix-command or create them manually in your favorite editor.
Open the .env file. Here you're going to store the credentials you need to make calls to the Twilio API; your Account SID and Auth Token. You can find them in the Twilio Console. Copy the lines below into .env and then paste the values of the two Twilio credentials in place of the two placeholders.
Next, open the send-alpha.js file and add the following lines to import and initialize the dependencies.
Then, at the end of the file, add the client.messages.create()
function below to send an SMS from an Alphanumeric Sender ID, substituting the two placeholders parameters with their respective values.
Save the changes and run the command below to receive a message from your Alphanumeric Sender ID.
Congratulations, you've successfully sent yourself a message from your Alphanumeric Sender ID!
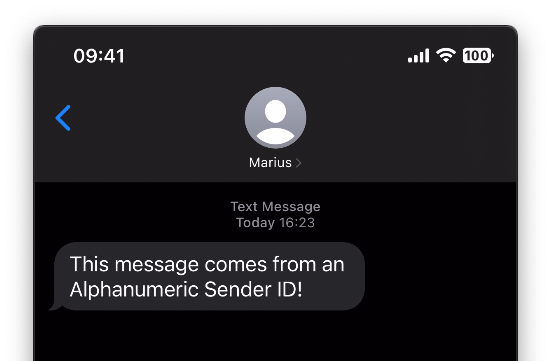
Get to know Messaging Services
In this post, you've seen how to set up a Messaging Service with an Alphanumeric Sender ID, and sent a message using that service with Node.js. This repository also contains all the code.
Sending a message without a phone number is just one of the many things you can do with a Messaging Service. They can also:
- Fallback to a long code for countries that don't support Alphanumeric Sender IDs
- Use Geomatching to select the best number from a number pool
- Scale up to handle sending higher volumes of messages
- Apply Sticky Senders to use the same number when communicating with a particular end-user
- And more!
I can't wait to see what you build!
Marius is a Developer Evangelist at Twilio who's a regular at various developer events in the DACH-Region. When not on the road, he works on innovative demo use cases that he shares on this blog and GitHub. If you have any open questions, contact him via the following channels.
- Email: mobert@twilio.com
- Twitter: @IObert_
- Github: IObert
- LinkedIn: Marius Obert
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.