Start a new Twilio Functions project the easy way
Time to read:
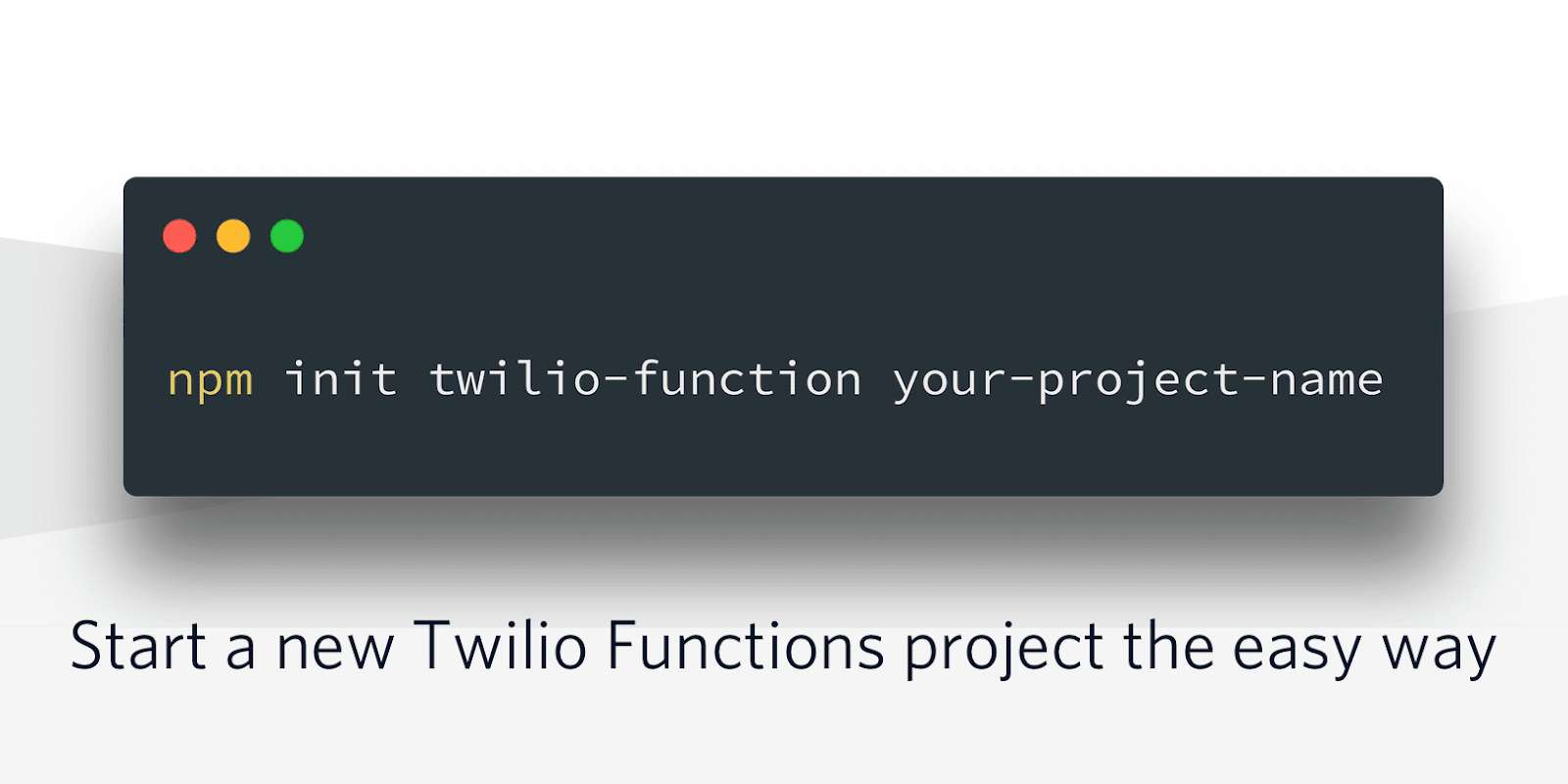
If you're building a Twilio project you will inevitably need to run some code in response to an incoming webhook request. One of the easiest ways to do this is with Twilio Functions, our serverless platform for running Node.js. Recently my colleague Dominik released the twilio-run
package that makes it easier to develop, test and debug Twilio Functions locally.
I wanted to make it even easier to get started with a Twilio Functions project, so I built a project generator called create-twilio-function
.
Let's take a look at how you can easily start and develop a Twilio Functions project using create-twilio-function
and twilio-run
.
Getting started
There are a few ways you can use create-twilio-function
. The easiest is if you have npm
version 6 or higher. You can check this out on the command line with:
If you don't have an up to date version of npm
you can update with:
Take a look at this article if you have permissions errors when you try to install global modules.
If you're on Windows you have a bit more work to do and I recommend you read the instructions in the npm documentation.
Once you have npm
up to date you can use create-twilio-function
by running:
Alternatives
npm init <initializer>
actually calls out to the npx
tool. If you have npx
installed you can call:
The last option is installing create-twilio-function
globally:
create-twilio-function
then generates a new project structure for you and installs the required dependencies.
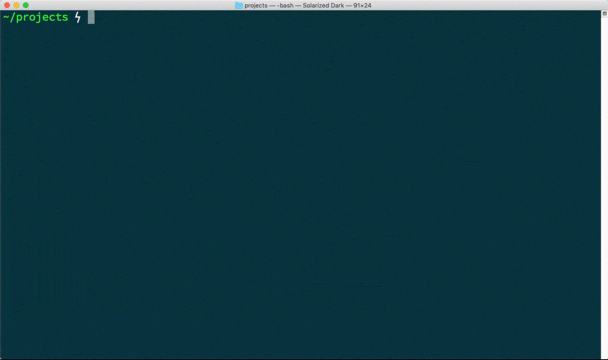
Building with Twilio Functions
Once the script has run to completion you will have a brand new project setup for local Twilio Functions development. Change into the new directory and take a look at the file structure. It should look like this:
In order, the files and directories we see are:
.env
: which will contain your account sid and auth token if you supplied it. You can use this to add more environment variables for your functions.gitignore
: The Node.js.gitignore
file from GitHub's gitignore project.nvmrc
: Twilio Functions currently supports Node.js version 8.10.0. If you are using nvm you can runnvm use
and get the right version.assets
: the directory you can store assets like images, HTML, CSS and JavaScriptfunctions
: the directory in which you write your Twilio Functionsnode_modules
andpackage-lock.json
: all your dependenciespackage.json
: your dependencies, scripts and other metadata about the project
If you take a look in package.json
you will see a start
script is defined to run twilio-run --env
. Call it with:
Your Functions project will start up showing which functions and assets you are hosting in this local environment. create-twilio-function
has one example function to get you started.
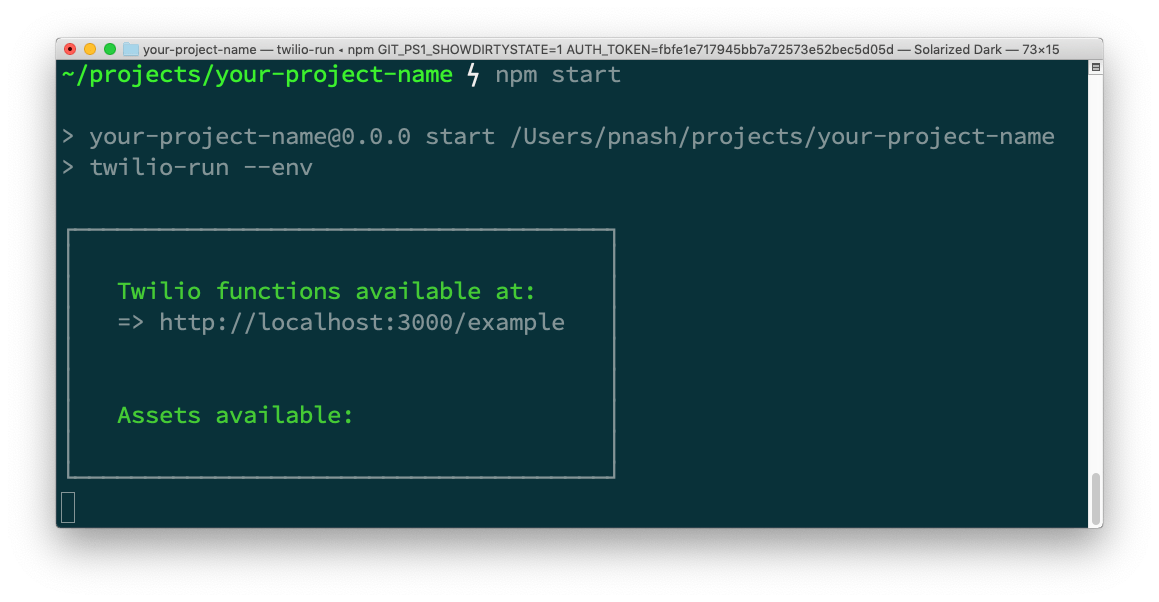
Open up the example from functions/example.js
to see how it's built. Currently, it is a "Hello World!" application for a voice webhook response using the Twilio Node.js library (which is provided in the Twilio Functions environment) to build the TwiML response.
If you make a request to http://localhost:3000/example using curl
you'll see the TwiML output:
With one command to create-twilio-function
and another to npm start
we've gone from zero to a full local development environment for Twilio Functions.
If you want to learn more about the advanced features of twilio-run
, from serving your functions publicly using ngrok to hooking in with a debugger, check out Dominik's post.
What about deploying my functions and assets to run on Twilio?
Right now, you'll have to copy and paste Functions code and/or drag and drop asset files in the Twilio console to deploy them live. We're working hard on an API for deployment. Look out for that soon, and get in touch with me if you'd like to be one of the first to try it.
What's next?
create-twilio-function
is a good way to get started building your own Twilio Functions and twilio-run
makes it easy to run and test your functions locally.
Now it's your turn to write your own functions. If you're looking for some inspiration, check out my posts on forwarding SMS messages or faxes as emails using the SendGrid API, or translating WhatsApp messages into emoji all using Functions.
We're always interested in how we can help you build Twilio applications faster and better. If you have ideas for how create-twilio-function
could help more, please let me know in the comments or open an issue on the GitHub repo.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.