Using Twilio Functions with TypeScript
Time to read:
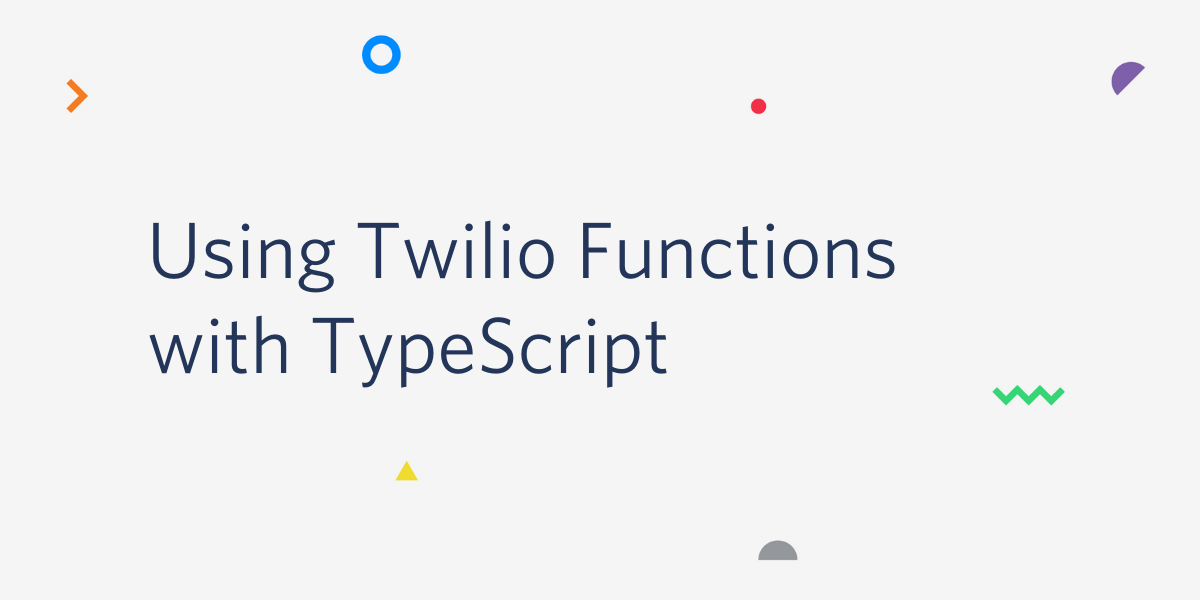
With the Serverless Toolkit we can include the development, debugging and deploying of Twilio Functions, Twilio's Serverless Runtime offering, more tightly into our existing development flows. For example we can add build tools such as TypeScript into our project to perform type checks on our Twilio Functions to catch more bugs during compilation time. In this post we'll look into how we can set up a Twilio Functions project using the Serverless Toolkit and TypeScript.
Requirements
Before we can get started, you'll need to make sure to have the following things:
- Node.js 8.10 (or newer)
- A Node.js package manager (the examples will be using npm)
- A Twilio account. Sign up for free
We'll be using the Serverless Toolkit via the Twilio CLI but you can also use it as a standalone. Check out the docs for more on how to use the Toolkit alone.
If you don't have the Twilio CLI and Serverless Toolkit installed run the following commands in your terminal:
Now that we have everything set up let's get started.
Creating a Project
We'll be working on a new project but you can also apply these steps to an existing project. To create a new project run the following:
This will create a new typescript-demo directory with a few default functions and assets in it.
If this is the first time working with a Twilio Functions project and the Serverless Toolkit, run the following command to start up your local development server:
Open your browser of choice and go to http://localhost:3000/index.html. You'll find a page that will walk you through the different files created in this project.
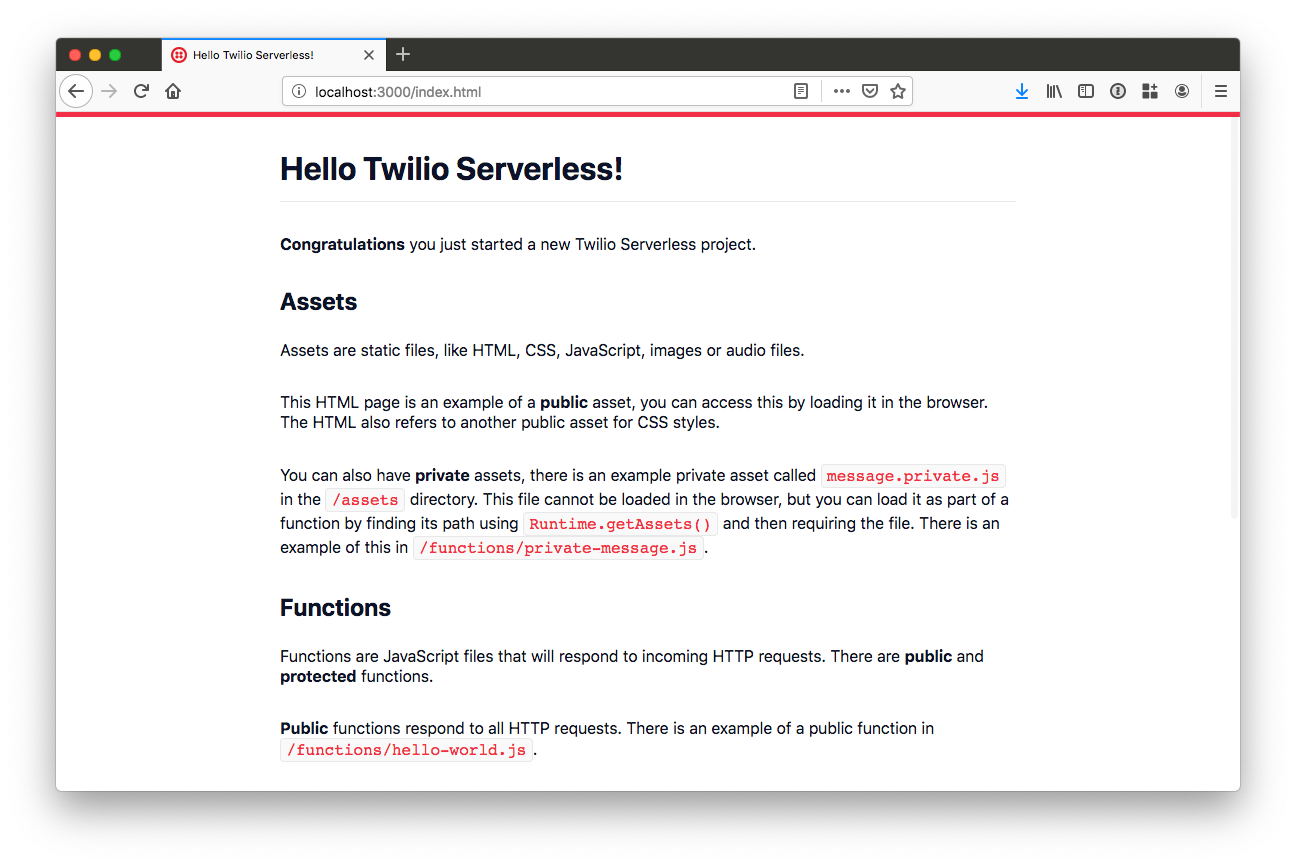
Now that we have the project up and running we have to add TypeScript to the party.
Setting Up TypeScript
The TypeScript compiler is available via npm registry. Since it's only needed as a build tool but not as a runtime dependency we'll install it as a devDependency
:
Now that we have our compiler installed, we have to set up our project configuration in a tsconfig.json
. This tells the compiler which options to use and where to place the output. In our case we'll use the existing functions/
directory as our output directory and a new src/
directory for input.
Create a new file called tsconfig.json
at the root of your project and place the following content into it:
This will set up everything necessary for the compiler to take any TypeScript files inside the src/
directory, compile them and place the output into the functions/
directory.
Since the functions/
directory will be our new output directory, go ahead and delete the current functions/
directory. The TypeScript compiler will create a new one once we run it.
We can run the TypeScript compiler manually for our changes but let's configure our npm scripts in a way that whenever we run npm start
or npm run deploy
we'll run the compiler. Update your package.json
accordingly:
Creating a Twilio Function in TypeScript
Now that we have the TypeScript aspect of our project all set-up, it's time to create our first Twilio Function using TypeScript. Create a new src/
directory inside your project and add a file called hello-world.ts
with the following content:
So far there's nothing TypeScript specific in this file except that we switched to an ES Module syntax for exporting the handler
function. This is okay because we are currently not running in strict
mode. We'll get to that in a minute.
To see if our changes worked, go into your terminal and run:
You should see the TypeScript compiler (tsc
) being executed and then it should print the same screen we've seen earlier when we ran npm start
.
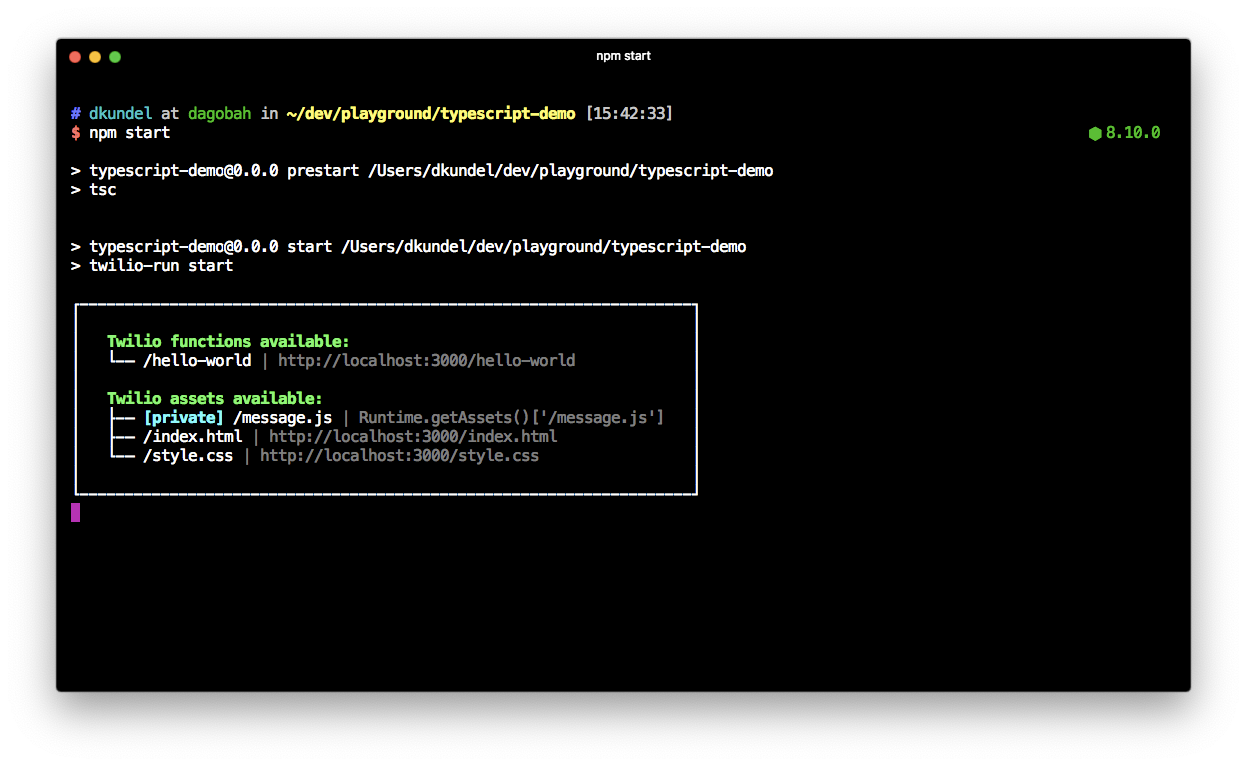
Open http://localhost:3000/hello-world and you should see Hello World from TypeScript!
printed on the screen.
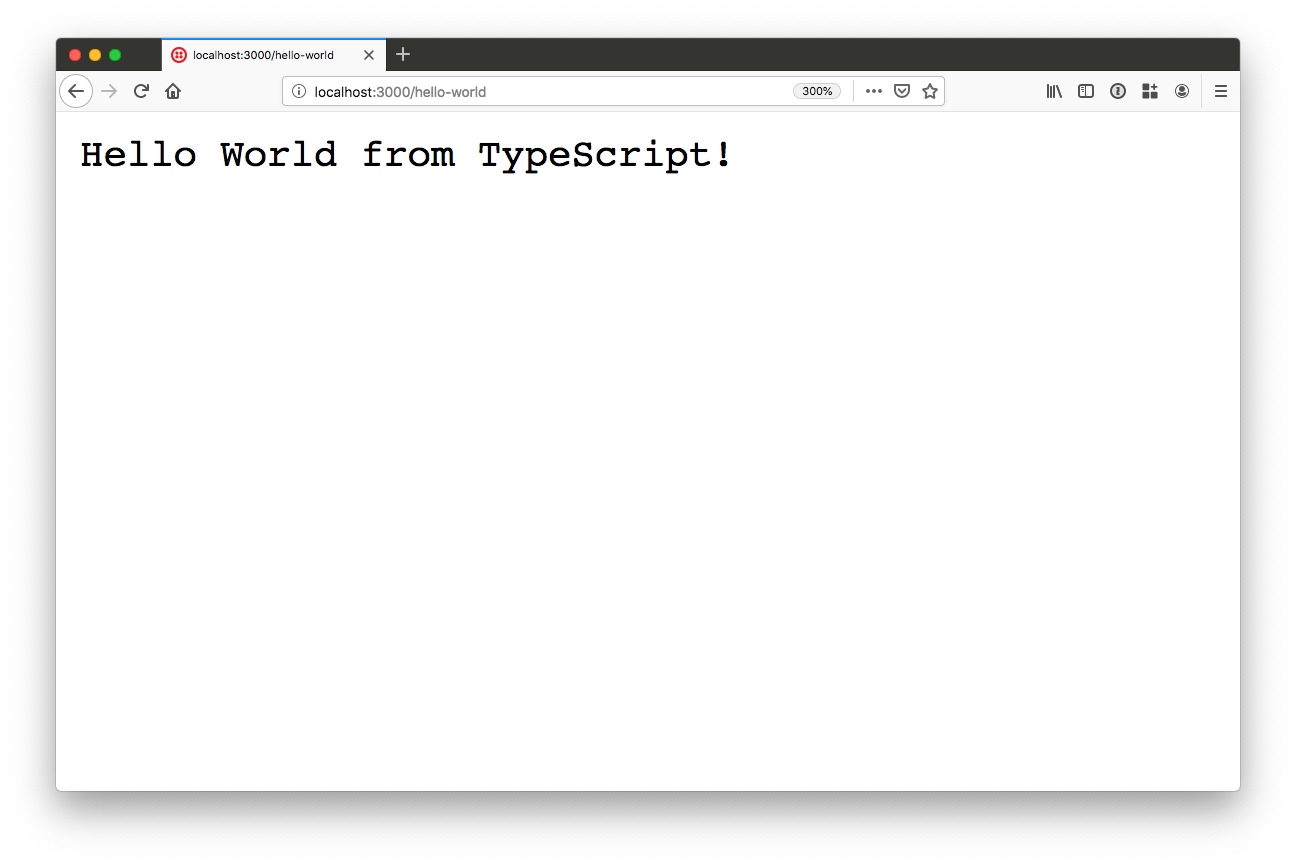
Getting Strict and Adding Types
Right now we have the TypeScript compiler set up but since we are not running in strict
mode we won't get all the benefits the TypeScript compiler can give us.
Start by enabling strict mode by changing the value of strict
in your tsconfig.json
to true
:
Try re-running npm start
and you'll see the TypeScript compiler complaining that we have three parameters that are implicitly of type any
. That's because so far we have omitted any time definitions.
We'll have to tell the TypeScript compiler what the types of the arguments are. We could create these types ourselves but to make this easier, we created a package containing all of these types under @twilio-labs/serverless-runtime-types
. Install the package by running:
Go back into your hello-world.ts
file and add the types for these arguments by adding the following lines:
Try re-running the TypeScript compiler by running npm start
again and you should see it's passing again but now with strict
mode enabled and http://localhost:3000/hello-world should work again.
Twilio Functions expose a few global objects like a global Twilio
instance of the Node.js helper library. Right now TypeScript is unaware of those. We can change that by importing the @twilio-labs/serverless-runtime-types
module directly. Let's try this by responding with TwiML instead of just a string in our hello-world.ts
:
Re-run npm start
and open http://localhost:3000/hello-world and you should now see TwiML with the same message being returned.
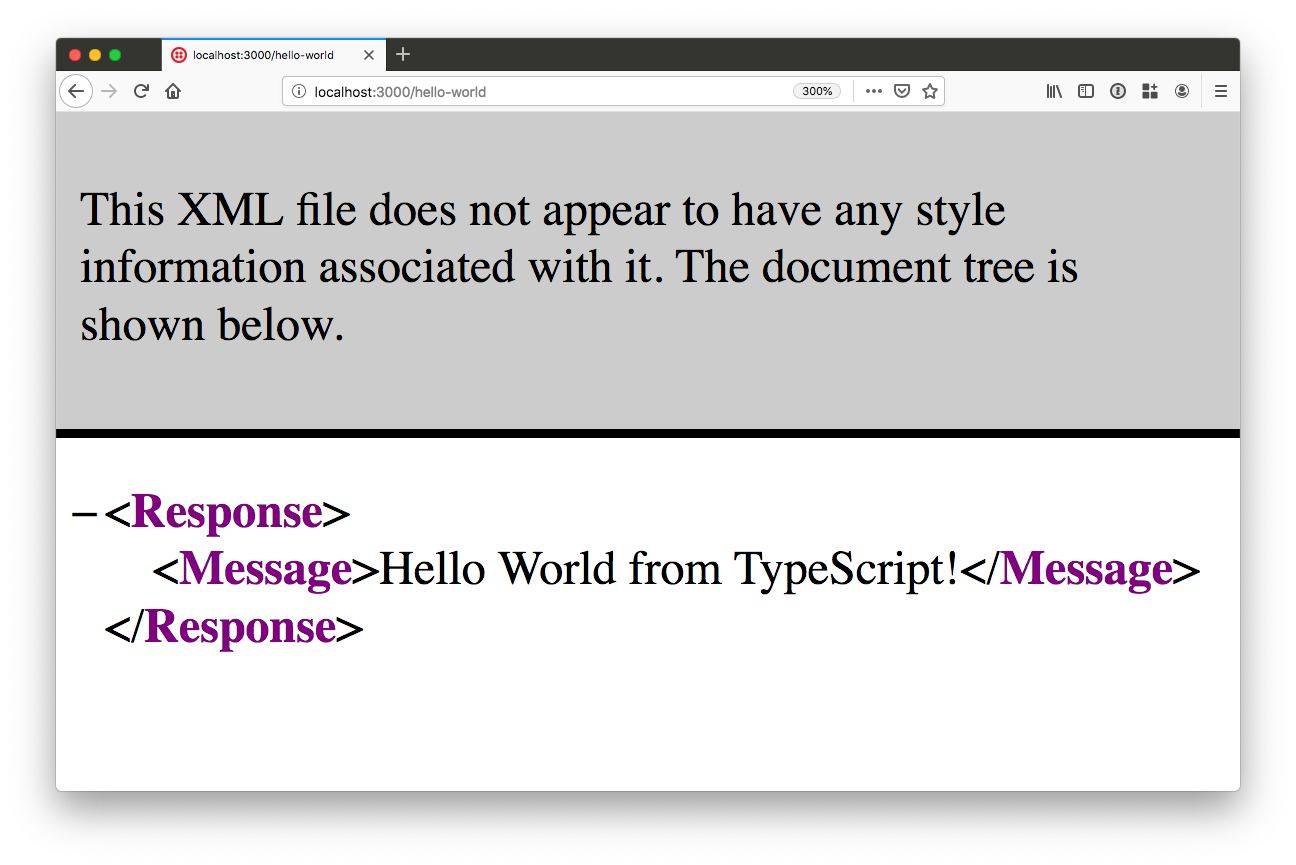
Since Twilio Functions are running Node.js you might want to use some Node.js specific functionality at one point. If you want to do that, you can install the Node.js types by running:
This will make any built-in Node.js module and globals available for you.
Deploying your Functions
Now that we have locally developed our Twilio Functions, all we have to do is, deploy our Functions to Twilio. Deploy your Functions by running:
Once your project has successfully been deployed you should see a list of the Functions and Assets that have been deployed. Your URL should look something like this: https://typescript-demo-1234-dev.twil.io/hello-world where 1234 will be some other number.
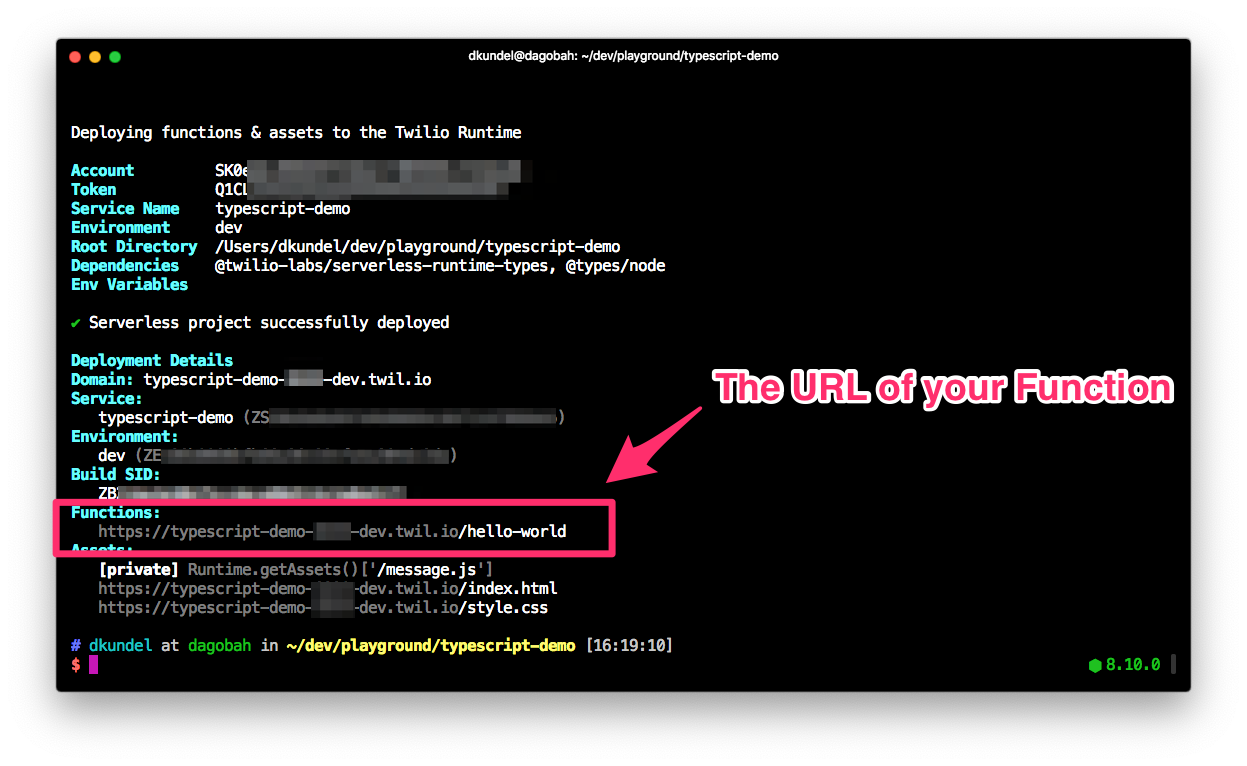
Wrapping It Up
Congratulations! You built your first Twilio Functions project with TypeScript and successfully deployed it to Twilio. Why not check out how you can link your new Twilio Function to a phone number using the Twilio CLI?
Before you leave, here are a few more tips of things you might want to do:
- Add the
functions/
directory to your.gitignore
file to not track the compiled output. This avoids confusion - If you don't want to constantly re-run
npm start
while developing, run in a separate terminalnpx tsc --watch
, this will kickoff TypeScript in "watch mode" and triggers a re-compiling whenever your Functions change. - Check out the Serverless Toolkit documentation to learn how you can locally debug your Functions or run ngrok to test them with Twilio
If you have any questions, feedback or just want to show me what you build, feel free to reach out to me:
- Twitter: @dkundel
- GitHub: dkundel
- Email: dkundel@twilio.com
- dkundel.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.