12 Hacks of Christmas – Day 7: Spread Christmas Cheer With littleBits, Laravel and Twilio
Time to read: 6 minutes
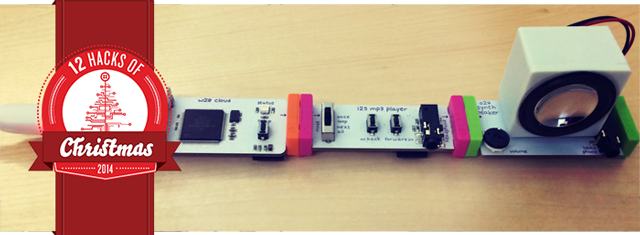
My mom’s favorite Christmas song is “I’ll be home for Christmas” which means I’m in major trouble since I won’t be making the trip to KC to visit her this year. I imagine many of you, like me, may not be able to spend the holidays with your families. I wanted to build a hack that helps people feel connected to the ones they love no matter how many miles separate them. My creation is a hardware device that will play a clip of a Christmas song whenever someone sends a text to a Twilio number. I can share this number with my family, they can text whenever they want and I’ll have a festive way of knowing they’re thinking of me. Check out this video to see it in action:
You can deploy this web part of this application to heroku with one click by clicking here. But you’ll have a lot more fun if you read this whole post, I promise. Let me show you how to build your very own version this hack..
Our Tools
I opted to build this hack using littleBits which are a collection of electronic modules that snap together with magnets. They’re super easy to get started with and approved for ages 8+. That means this hack is great for the whole family!
We’ll be building our device using the following littleBits modules:
- cloudBit – This will allow us to interact with our with our littleBits via the cloud using the cloudBit API.
- USB Power – Power for our device is necessity!
- mp3 Player – This module will let us load mp3s onto an SD card and play them back on demand.
- Synth Speaker – This module will actually let us hear the mp3s as they play.
For our web server, we’ll be creating a very basic Laravel application that receives data from Twilio. Make sure you have:
- A Twilio account – sign up for free
- PHP 5.4+
- Laravel installed on your server
Just a Little Bit of Hardware
As I mentioned, littleBits are great pieces of hardware for hackers of all ages. They magnetically snap together so there’s no wiring required. If you want – you can use them without any coding required but this a Twilio blog post so you know we’ll be writing some code.
First let’s get our littleBits connected to the internet. For this task we’ll be using our cloudBit. If you haven’t set up a cloudBit before it’s super easy. Connect your cloudBit to the USB power module and then head over to littlebits.cc/cloudsetup. The instructions there will walk you through getting connected to your local network and making sure everything works as expected.
Now that we’re connected to the internet, we can start adding modules to play our holiday mp3s. But before we connect our mp3 module let’s add some Christmas tunes to the SD card. On the bottom of your mp3 module you’ll find a memory card:
Put this card in an SD card adapter:
Now you can access this data with a memory card reader. Some computers have a card reader built-in which makes it even easier. On this card you’ll see a handful of sample audio files. The mp3 module loops through each any mp3s stored on the card. Since we’re only using one song we can delete all the mp3s and just include the Christmas song we want to play. I felt there was nothing more fitting for my hack than Death Cab for Cutie’s rendition of Christmas (Please Come Home).
After you’ve loaded up some tunes, connect your mp3 player module to your cloudBit. To make sure our hack works continuously toggle the switch on the mp3 player to loop:
Then connect your synth speaker to your mp3 player. The end result should look like this:
Before we go any further we need to test out our device to make sure it’s working as expected. Let’s use the littleBits cloud control to send a signal to our device and make sure a song starts playing. In the cloud control select your cloudBit and make sure you’re on the send screen. Here you’ll see a giant purple button:
Clicking this button will send a signal to your cloudBit and you’ll hear beautiful music. Victory! I’m hope you’re getting as excited as I am about this hack.
Thinking of You
Now that we’ve built and tested our littleBits device we need to build a web server in order to communicate between Twilio and the device. In this case, we’ll be building a small Laravel app but if you’d prefer you should be able to apply these concepts to the littleBits API and language or framework of your choice.
Let’s start a new Laravel application:
Now we can add a new /message
route in our apps/routes.php file:
We need to make a request to the littleBits API but first let’s setup some environment variables we’ll be using in our code. Create a new file called .env.php:
Here we’ll be storing our cloudBit device ID and access token. You can find this data in the settings section of your littleBits cloud control dashboard:
In case we have any issues in our code during this process let’s turn on debugging. Open app/config/app.php and look for the debug value. Make sure it’s value is set to true:
Now we can add the code to our /message route that will make a request to the littleBits API to trigger our device to play music:
We’re making a request to the /output endpoint which will output voltage to our device (triggering our mp3 to play). We want to have the music play for 30 seconds so we’re setting the duration_ms to 30,000. We’re using the token we got earlier to properly authenticate our request.
Start up your server so we can test this out:
Now we can make a curl request to make sure everything is working as we expect:
Hearing beautiful music? Perfect. Let’s add the code we need to have this work with Twilio. When a SMS messages comes into our application we want to respond to whoever sent that message. We can use TwiML to accomplish this. Let’s add the code to our /message route that will take care of this for us:
If you’re running your Laravel application locally you need to make sure Twilio can access your web server at a publicly accessible URL. Ngrok is a great tool for accomplishing this. You can find a great tutorial for setting up ngrok here. Or you can deploy this code to heroku by clicking here.
Once you have your server exposed to the outside world, head into your Twilio Dashboard and set up our Messaging Request URL for your number to point at your /message route
Now test it out by sending an SMS message to your Twilio phone number and hearing the beautiful music come from your littleBits device! Once you’re sure it’s working, share the # with your friends and family so you can know when they’re thinking of you. You can find the full code for this project on github.
I (won’t) Be Home For Christmas
Nothing beats being home for Christmas but when we can’t make the trip technology can help us have a unique connection to those we love. What improvements could you make to this hack to make it even better? Maybe you could forward the messages people send to your phone so you hear the song and get the message. Are you doing any hacking for the holidays? I’d love to see it: @rickyrobinett or ricky@twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.