Add SMS to your Web App (with 4 lines of code)
Time to read: 4 minutes
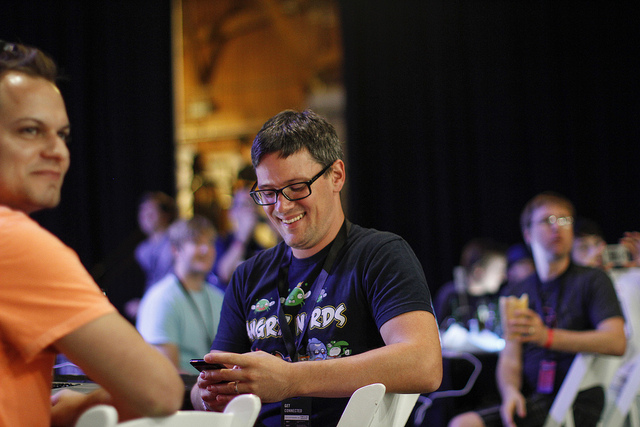
In this post I’d like to quickly show you how easy it is to add SMS to your web app then give you a more in-depth look at how Twilio works as well as some more robust use-cases for SMS.
This post was inspired by a conversation I had with a friend shortly after joining Twilio. We were talking about API’s when he nonchalantly explained that he himself used Twilio to send SMS notifications from his web app. As proof he then turned his laptop around to face me and pointed to the “four lines of code [he] added” to enable SMS.
Certainly it is not always that simple. Some of our biggest customers have built entire businesses around the Twilio Platform, but everyone has to start somewhere and in my opinion these four lines of code are the place to start.
So without further adieu, I present the four lines (okay, throw in a couple extra for proper formatting) needed to send an SMS from your web app:
Or how about Ruby:
To see more awesome examples including node.js, python, C# or java visit the Twilio docs.
So now that we’ve gotten that out of the way, let’s quickly discuss how Twilio accomplishes this task.
How Twilio Works
Outbound Messages
When you send an SMS/MMS from your web app using Twilio it works like this:
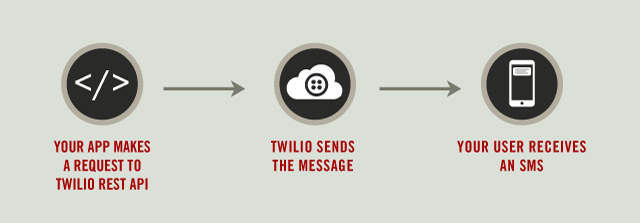
As you can see sending an SMS is pretty straight forward, in fact all of this interaction is triggered with just those four lines I showed you above. But what if you want that user to be able to respond to your outgoing message? Well then your Twilio Phone Number needs some extra instructions, specifically what to do when it receives an incoming SMS.
Inbound Messages
When an SMS is received by your Twilio Number it looks something like this:
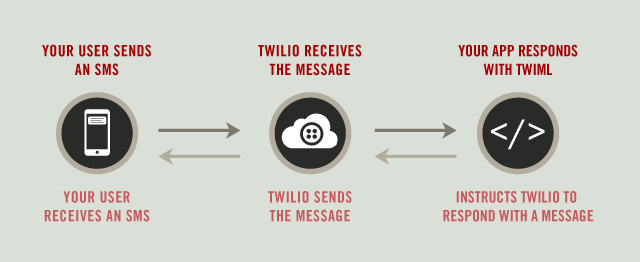
Now that we’ve looked at how Twilio interacts with your web application at a high level, it’s time to put our gloves on and dig in. We’ll start by doing some basic setup.
Setup: Get your free Twilio number and install a helper library
Sign up at Twilio to get your free Twilio number. Once you sign up click on Numbers to see your number.
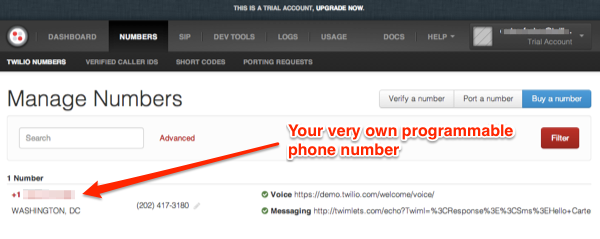
If you plan on receiving SMS messages with your web app you will also need somewhere to host your TwiML instructions. There are lots of web hosting services that will host your application for free or cheaply including Heroku and Googles AppEngine.
Installing the Helper Library
Twilio helper libraries give you the ability to interact with the Twilio REST API in the language of your choice. Installing the libraries is usually quite straightforward. For example, to install the Ruby helper library you could just install the gem like so:
Here are some of officially supported Twilio helper libraries:
Click to get installation instructions and further documentation
To view all of the available helper libraries visit the Twilio docs.
Once you’ve installed the helper library of your choice it’s time to use the REST API to send and receive SMS Messages.
Next Step: Read the docs
If you’ve worked with APIs in the past you might just want to dive in to the API docs. The Twilio REST API documentation is fully stocked with examples to help you dive in. However if you’re like me and you prefer a more tutorial approach check out the Twilio Quickstart, which will walk you through sending and receiving your first SMS.
API Reference:
Quickstart: Sending and Receiving an SMS (uses PHP)
Congratulations, now you’re able to add SMS capabilities to any of your web apps in minutes.
Once you’ve grasped these basic concepts consider upping the ante and adding even more sweetness to your application. Here are some popular ideas:
- Two-factor Authentication: Keep the SPAM out
- SMS Notifications
- Proactive Alerts
- Mobile App Distribution: Text to download – mobile solution
Well I hope this taste of the Twilio API has left you excited to learn even more. Many of my colleagues have written awesome tutorials on using SMS for even cooler purposes. Here are a few of my favorites. Happy hacking.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.