How to Automate Important Phone Calls Using Twilio Voice and C#
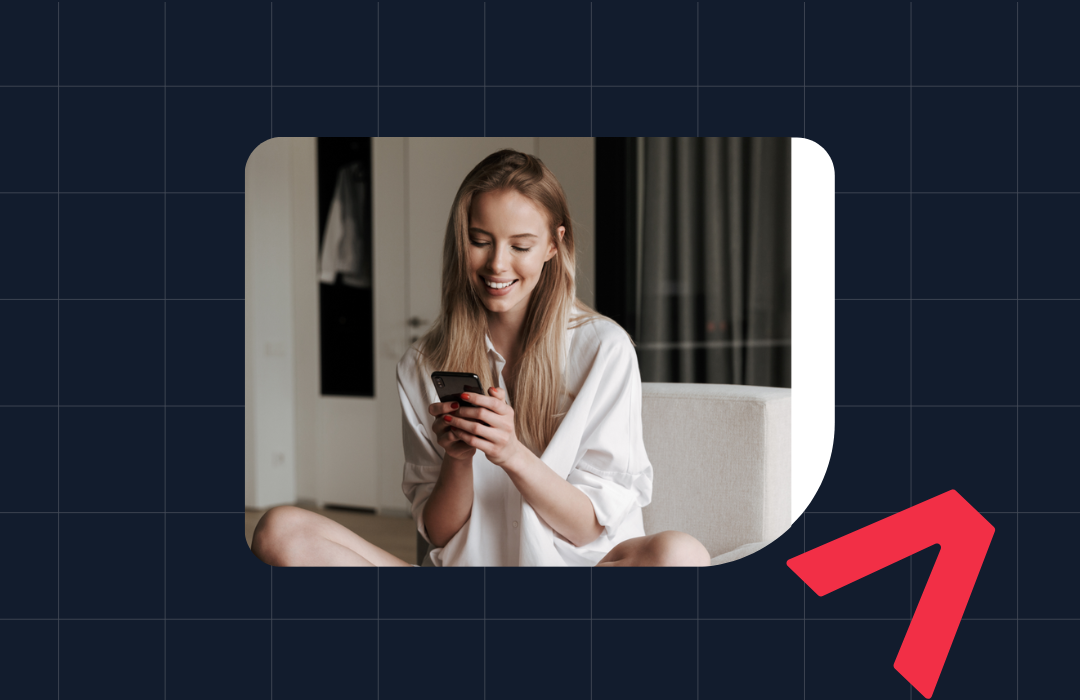
Time to read: 7 minutes
If you’re deep into technology, you can probably remember lots of trivia and several coding languages. But maybe you struggle with remembering the important dates where you’d need to call someone on the phone. You won’t ever forget to call your mom on Mother’s Day again - or call Dad on Father's Day coming up - if Twilio remembers to call for you!
Prerequisites
You’ll need a few things to follow along:
- Windows 10 or 11 OS, or Mac OSX.
- .NET 8 SDK.
- A code editor or IDE (I recommend VS Code with the C# plugin , or Visual Studio).
- A free or paid Twilio account.
- A Phone Number with Twilio.
Set Up the project environment
To do this tutorial, you’ll need a Twilio account and a phone number associated with that account. You can sign up for Twilio using this link. You will only need your email address.
From there, you will need to buy a phone number for automated calling. Access the Buy a Number page by going to Develop > Phone Numbers > Manage > Buy a Number. Be sure to leave the box checked for voice capabilities. You will see a list of available numbers that you can narrow down with search. You can choose any number that makes sense for you, whether it's a toll-free number, or a number that matches your region.
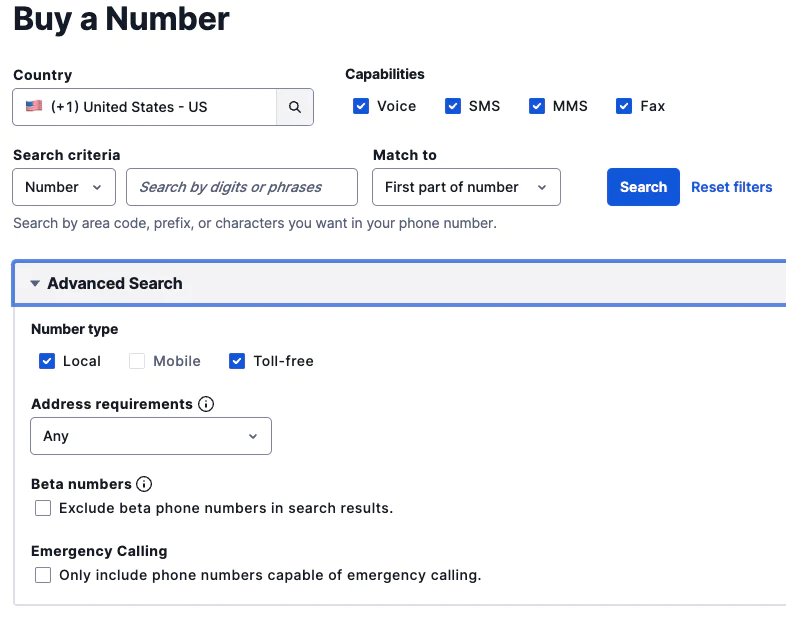
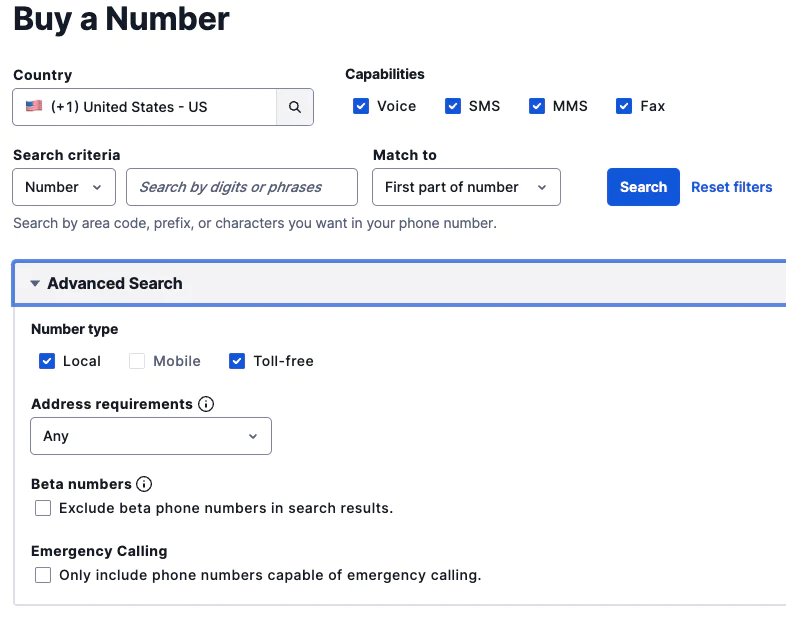
Click Buy to purchase the number you've chosen. You can find out more about phone number selection in Twilio's Documentation: How to Search and Buy a Twilio Phone Number.
You will also need to set up your IDE to work with C#, if you haven’t done so already.
If you have all the prerequisites, you can start building the application. Get started by creating a new folder, and then a new .NET project in your IDE of choice. For Visual Studio Code, go to the Command palette by hitting shift+command+p or going to View -> Command Palette, and select the options .NET New Project -> Console App. Accept the default folder choices if asked. In Visual Studio, you can go to New Project and create a .NET Console Application.
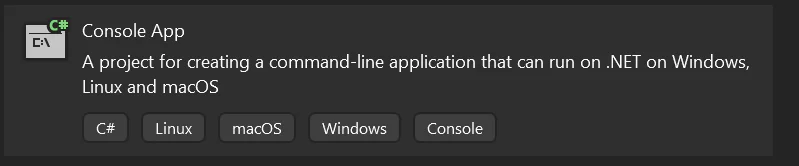
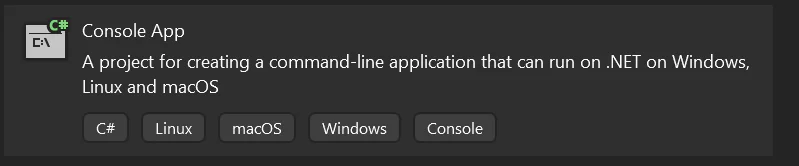
Give your project a friendly name. I called mine "TwilioCaller1". Hit OK, and this will create the project in your new folder and create a few files necessary for your project.
Once you have .NET installed and set up in your IDE of choice, you will need to add the Twilio CLI to your IDE.


If you’re using Visual Studio, you can use the nuget package manager that’s built in to install the Twilio CLI. If you are using Visual Studio Code, there is no built-in nuget package manager, so you need to install the CLI via the command line. Open up a Terminal in Visual Studio Code, and type in the following line. After 'add,' you’re using the name you gave your project. Since my project is TwilioCaller1, I used this line in the VS Code terminal:
Build the Base C# Application
A file named program.cs was automatically generated with your project. Open the program.cs file, and replace the code in that file with the following:
You might see some errors in this code, but we’ll fix those errors now. It’s bad coding practice to put your security keys directly into your code, so to secure your Auth Token and Account SID, you will use the Dotnet Secrets Manager.
There are multiple ways to access this depending on your IDE. If using VS Code you can install user secrets in the command line by typing this into the terminal:
If the command asks for you to enter a --project
, use whatever project name that you used when creating the application.
Microsoft.Extensions.Configuration
is necessary to configure the environment variables in this code. You can add this nuget package to your application in the package manager in Visual Studio. You can also add this via command line in VS Code by typing the following into your terminal:
Now find your Account SID and Auth Token in your Twilio account. You should see this information in the dashboard on the bottom left corner of the Welcome page. Look for the Account Info box as pictured below.
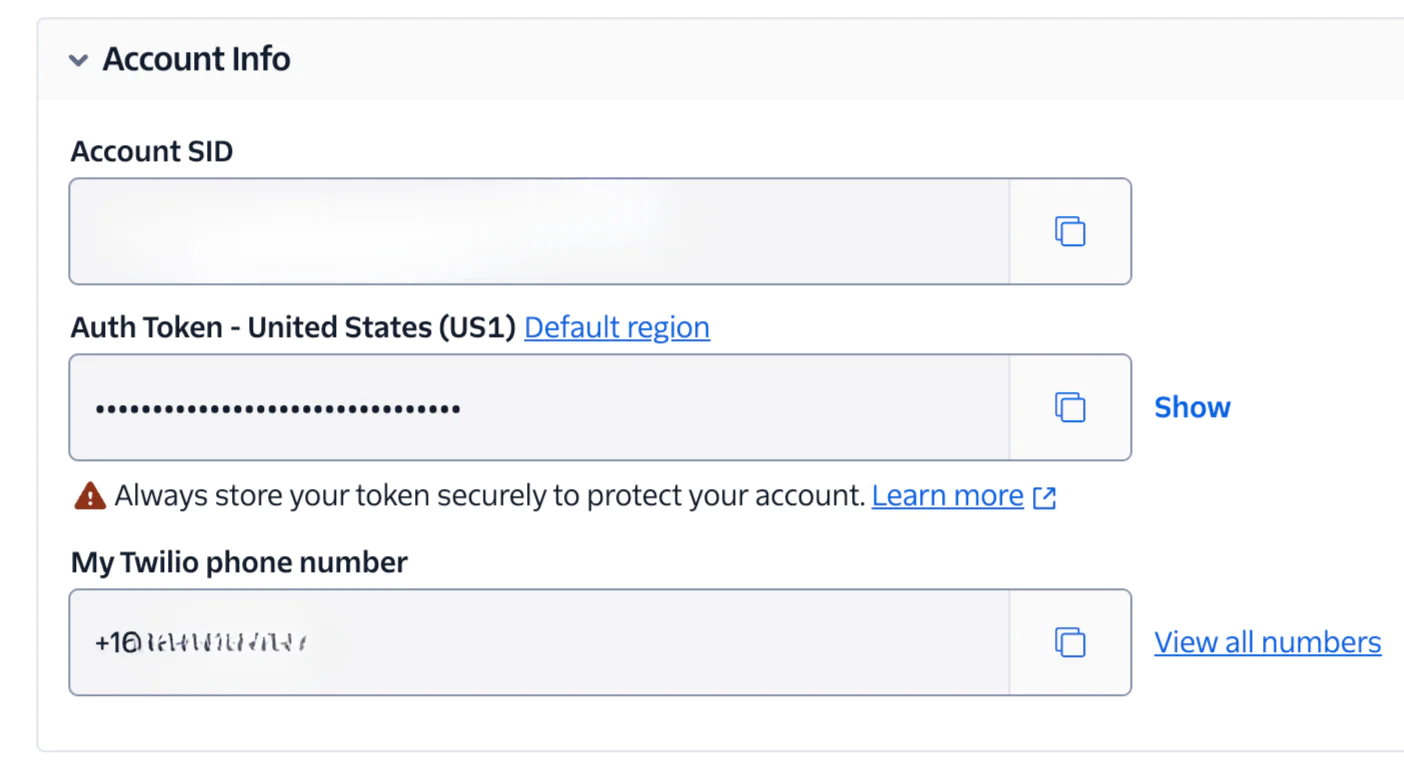
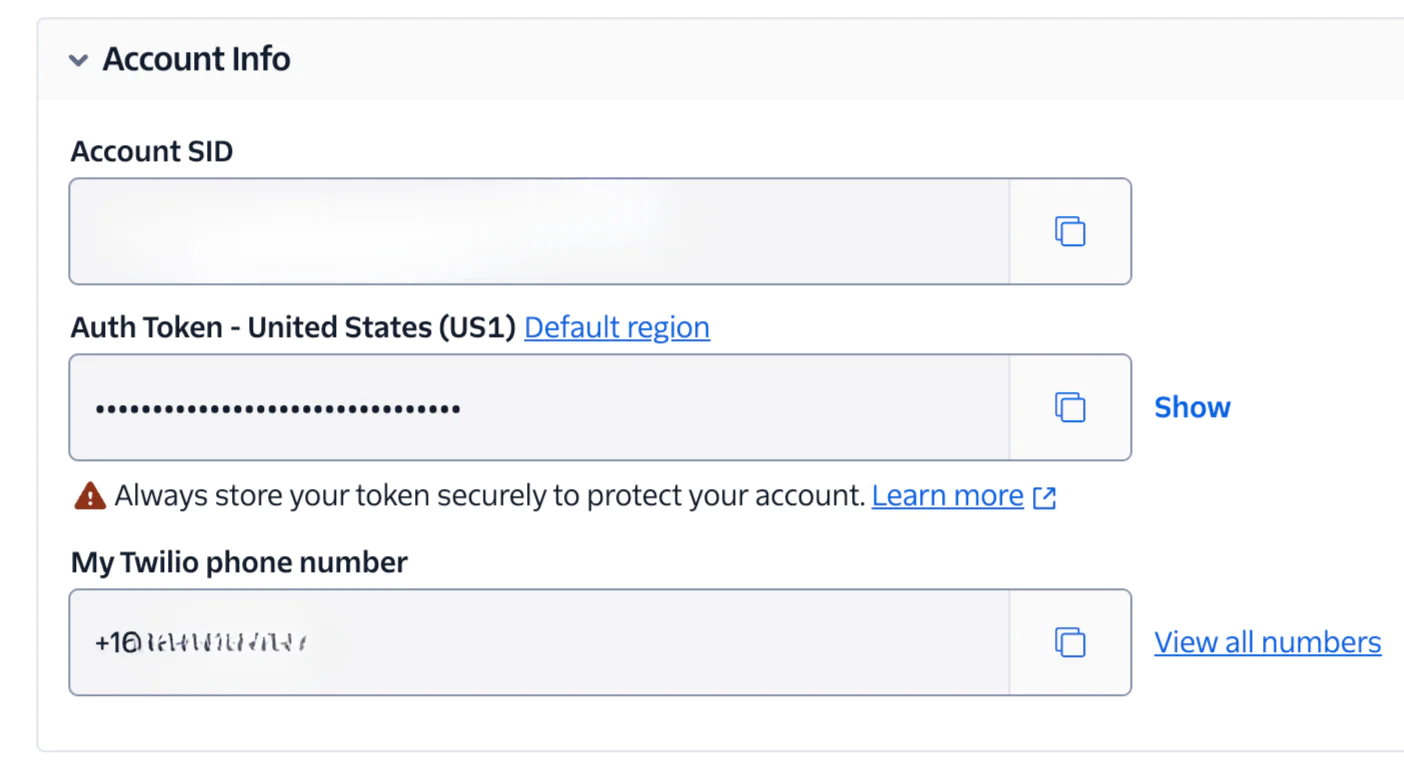
Type these statements into a command line. Replace the items in brackets with the appropriate AccountSID and Auth Token values from your account.
If you are using Visual Studio, you can also right click your project name and choose the “manage user secrets” option. This will create a secrets.json file. It starts out empty, but you can add in these lines to contain your secrets.
You can learn more about storing your Twilio credentials securely in the documentation . This article also goes into more detail about best security practices to keep your .NET application safe .
You’ll now need to add your Twilio phone number which you purchased as the "from" variable in the code. Make sure it matches a valid phone number from your Twilio account. For the "to" variable, it’s a good idea to add your own phone number here, for testing. Choose a number for a phone you have immediate access to. You will change this to your intended receiver’s number when testing is complete.
Replace and with your actual numbers, using the E.164 format .
Observe this line in the code:
This line sets a variable with inline TwiML. You can put any message you want here (and any voice type) for testing. To learn more about TwiML and the possible things you can do with it, check out the documentation .
That’s everything you need to make an automated voice call from Twilio. Run your application in debug mode, and you should get a call on the phone number you entered in the "to" line. It will say whatever you typed in the in-line TwiML. Change the phone number to the number for your final recipient and you are ready to make the automated call.
Time the phone call on a Windows machine
Now you have a quick .NET application that will call you on the phone. But there’s one more step: calling at the right time. To do this, we need to schedule the task.
C# has various Timer classes that could help us construct code to run the call on Windows 10 and Windows 11 machines. But there are downsides. The timer can be imprecise, and you will have to keep your application running while the timer counts down to the special day.
Task Scheduler is a better alternative. On your Windows machine, go to your search bar and search for "Task Scheduler". This opens up the Windows Task Scheduler interface. Right click on the Task Scheduler Library folder on the left and create a new folder. Name this folder MyTasks to distinguish your personal tasks from other tasks being run by Windows.
Create a New Task. Right click on your new folder, and choose Create Basic Task. This will bring up a short wizard that allows you to schedule a task to run at a certain time. Give your task a friendly name, and then on the second page, choose `One time` as shown in the screenshot.
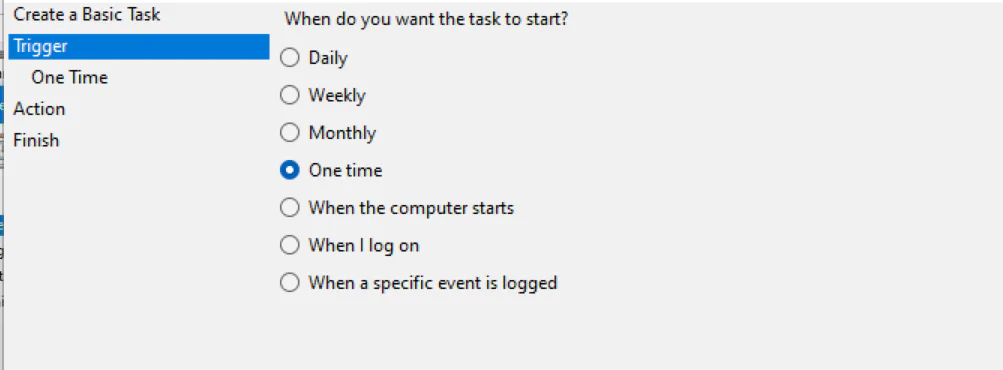
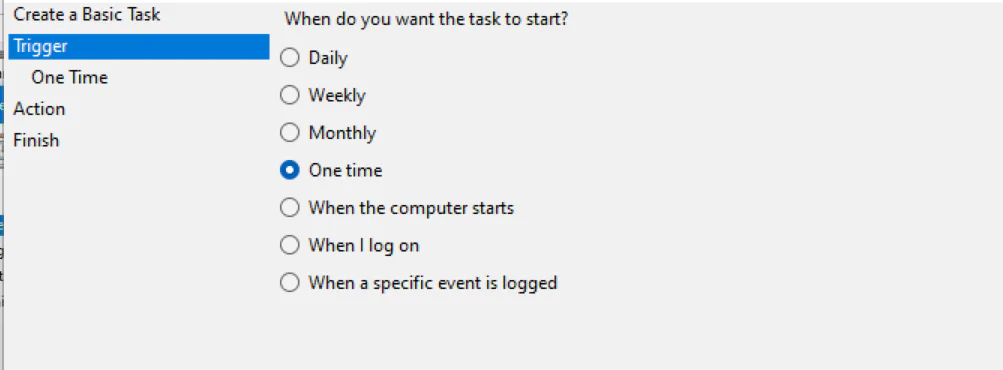
To find the action you need to execute, go into your build folder for your Console application and select the . exe file that was generated during your debugging session. Now complete the rest of the forms in the task Scheduler. The final scheduled task will look something like this:


You might want to test it by setting your scheduled task for a time sooner than your chosen date and checking to see if your phone rings. Once you’ve confirmed it works, set the date to that special day, and rest assured that call will be made on time!
Time the phone call on a MacOs machine
If you are on a Mac, you will have to use a different automation tool to make your code execute the call at the right time. Look through your Applications folder and find Automator. Automator can work with your Calendar application to execute an automated task at a time you set.
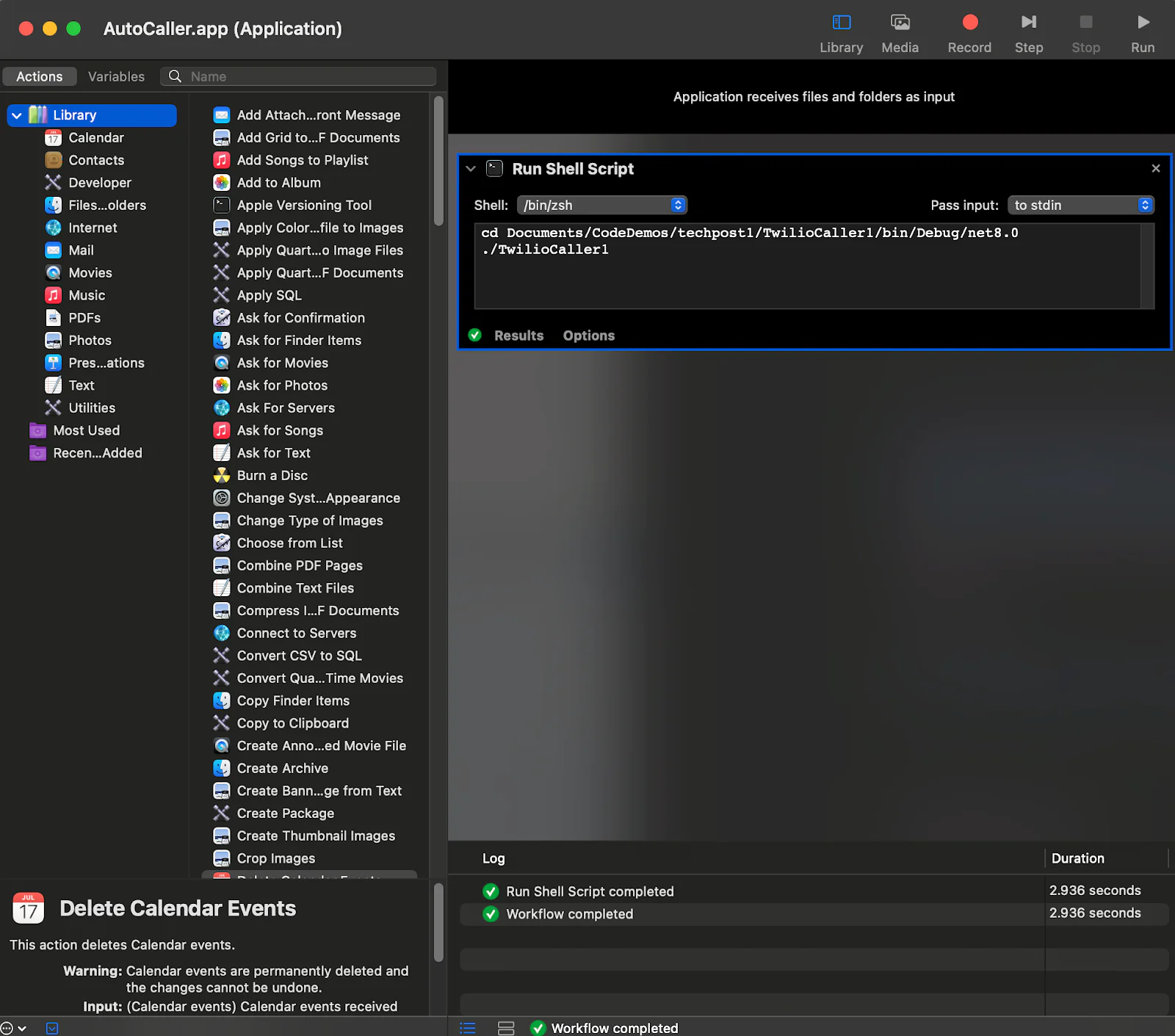
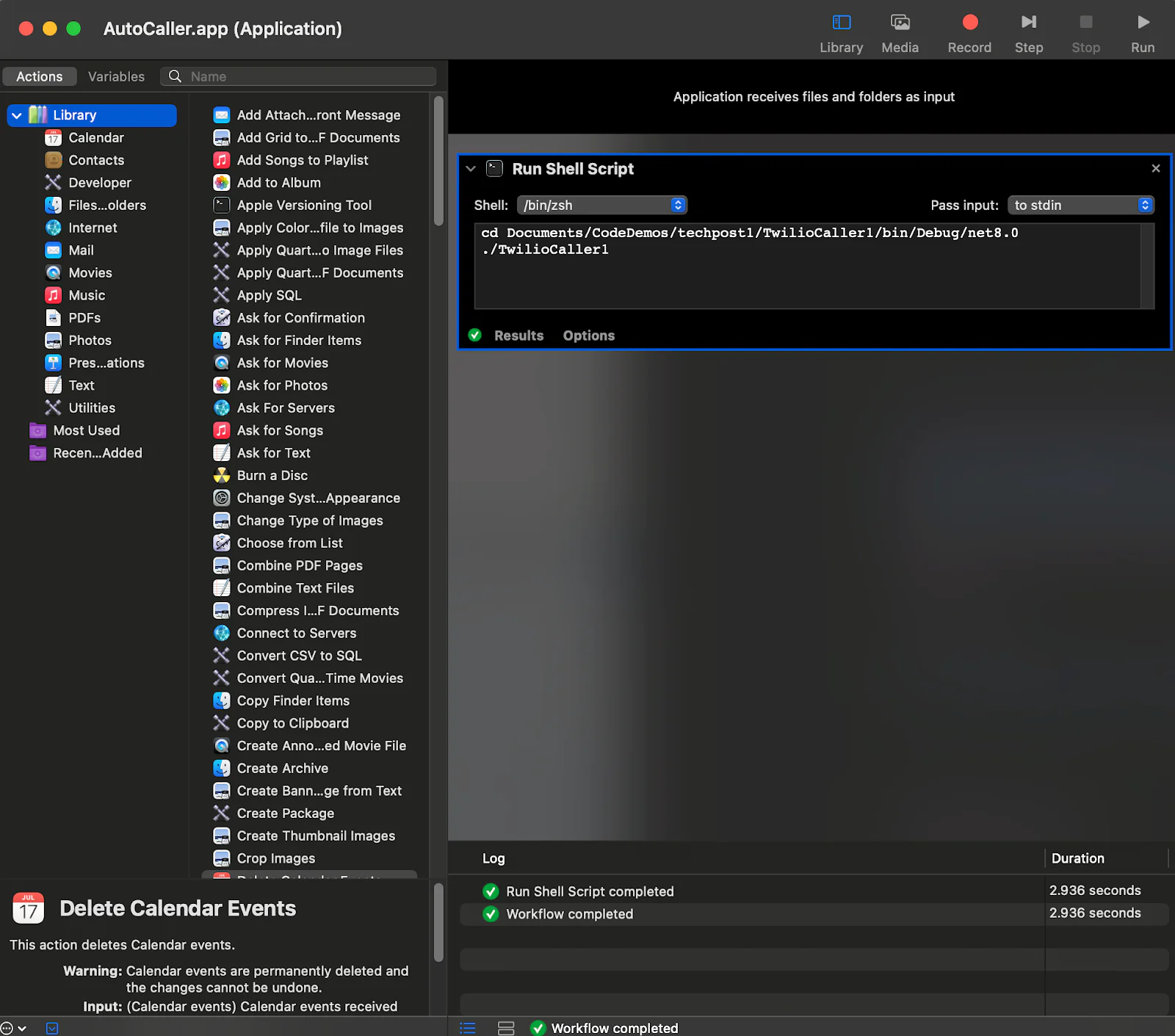
Give your automated task a friendly name such as "TwilioCaller1", and choose the Run Shell Script option from the list of possible actions. Write a shell script that goes into the directory where your script is stored, and then run the script with the following command:
Automator will save your task as an application. You can save that application to your Documents folder on your Mac, or another specified location.
Use Mac’s built-in calendar to make sure your code runs on the right day and time.
Open up the Calendar app, and click on the date that you want to add the event. The date highlighted in the screenshot is Mother’s Day, but you’ll choose the day that works for you. Now you want this event to fire a custom alert. Click on the event time, and you’ll see the Alert options. Choose Alert, scroll down to Custom, and then click Open File.
From here, select the autocaller automated application that was created. You can set the exact time for your Alert to run your Twilio script. Be sure to pick the right time, taking into account time zone differences between yourself and your intended recipient.
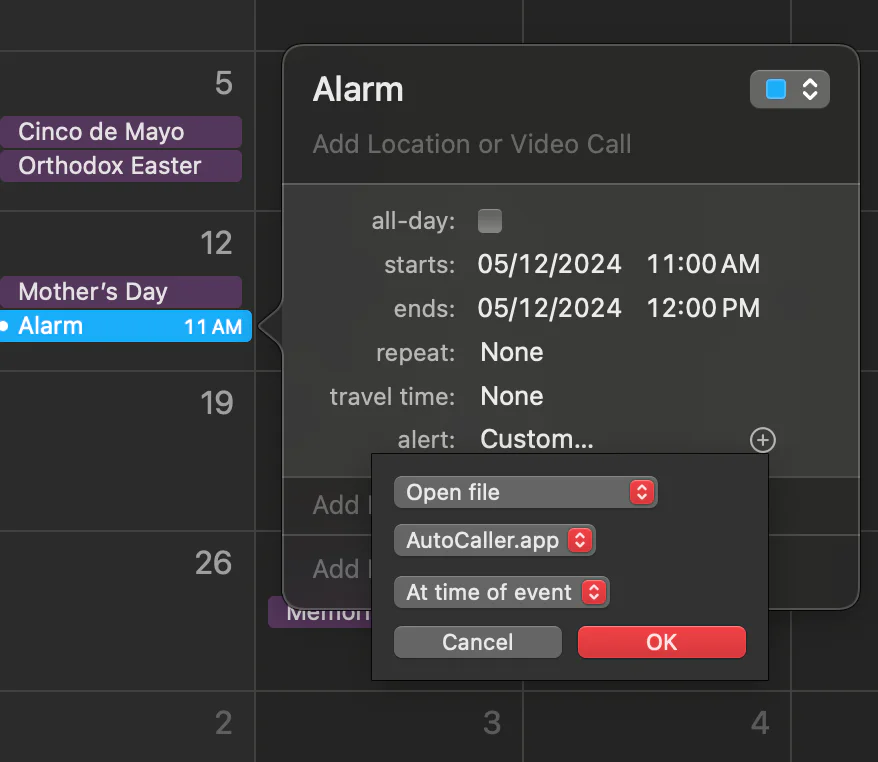
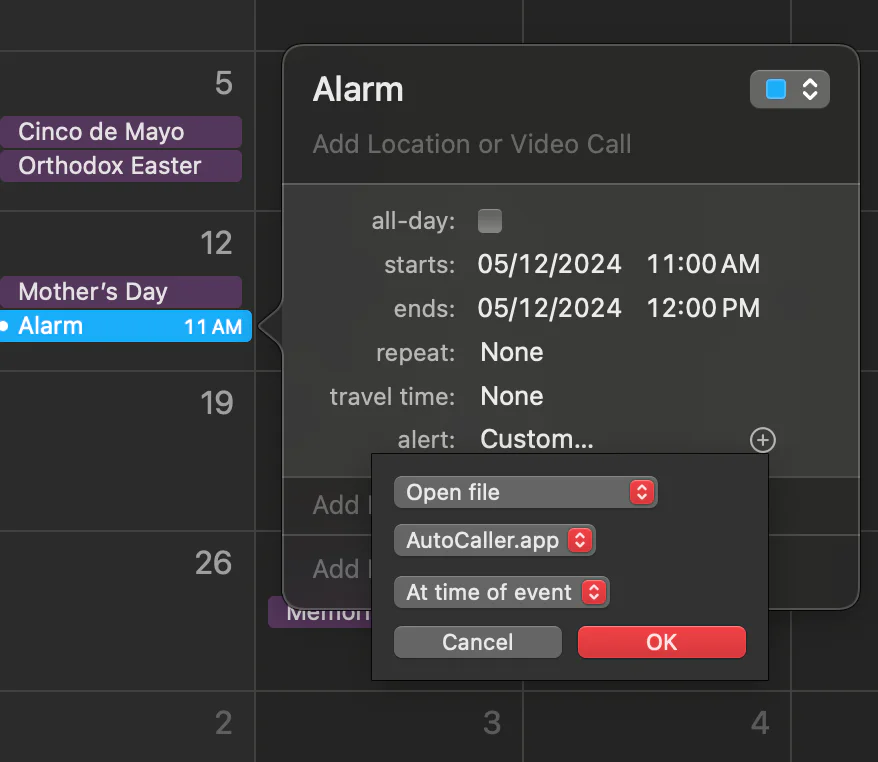
Now your calendar app on Mac will remember to make your phone call automatically!
Test Your Automated Phone Call Application
You need to make sure that the intended recipient of your call knows that this call is coming from a new Twilio phone number, so that they'll be sure to answer the phone when it rings on time. You wouldn’t want that call to be ignored as spam!
But after all that, you might be thinking: “This is actually impractical. My mom isn’t super tech-savvy and I think she’d find an automated robo call a little impersonal, rather than fun.”
To prevent your mom from feeling confused, try this test. Replace var to = new PhoneNumber("[your_recipients_number]");
with your own personal phone number instead of your mother's.
Replace the following line:
With this:
Now the application reminds YOU to make the personal phone call, and all your problems are solved!
What's next for automating phone calls with Twilio Voice?
You might consider some ways to improve this application. You should, for example, try hiding the phone numbers you’re calling to and from in the secrets store to prevent anyone seeing your phone number. You could also experiment with making your phone call more fun by adding an .MP3 file to play music on your Twilio call or going deeper into TwiML.
You can also consider sending automated texts instead of an automated phone call. Twilio Messaging Service will automate texting no matter what OS you are using. Try out this C# Scheduled Messaging tutorial next if you want to send an automated text message at any time.
Amanda Lange is a .NET Engineer of Technical Content. She is here to teach how to create great things using C# and .NET programming. She can be reached at amlange [ at] twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.