How to Create A Mock Twilio Lookup API
Time to read: 3 minutes
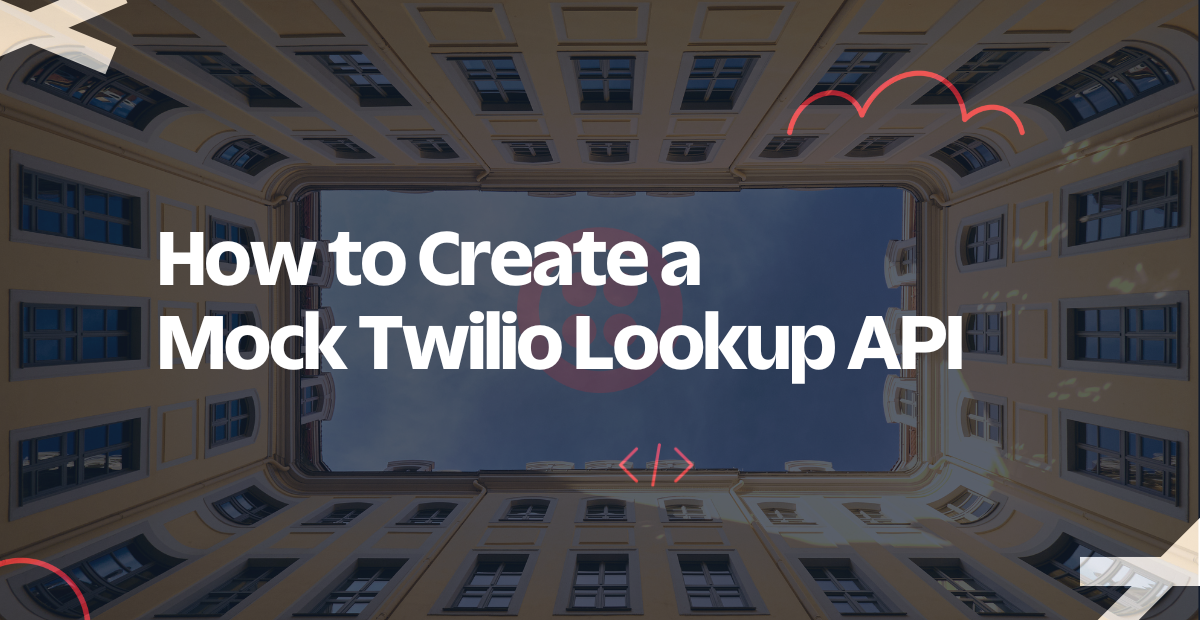
A significant number of web applications depend on external APIs when integrating third-party services. Due to maintenance or ongoing development, they may occasionally be unavailable during development.
In situations like these, mock APIs are helpful in testing, because they allow developers to continue integrating against the service. Alternatively, there may be a price per API request, so simulating API responses during development might be crucial to minimizing cost.
In this short tutorial, you will learn how to mock Twilio's Lookup API.
Prerequisites
- PHP 8.2 or newer
- Composer globally installed
- Your preferred text editor or IDE
- An HTTP client such as curl or Postman
Understanding Twilio's Lookup API
Among other things, the Twilio Lookup API enables customers to access phone number data, carrier details, and caller names attached to phone numbers. It also supports phone number validation and formatting. You can see a sample response from the API is available below.
Create the project directory
The first thing to do is to create the project directory, where you store your PHP projects, and to switch into it. To do that, run the commands below.
The structure of the application is simplistic, consisting of two directories: app and public. The controller class named Lookup.php, which manages the backend logic before passing it to the front end, is located in the app directory. The front end is located in the public directory.
To create the core directory structure, run the command below.
Use the following commands if you're using Microsoft Windows:
Create the application
The next thing to do is to add all of the dependencies that the project needs. The application will run on the Slim framework and PHP’s version of Google’s phone number library. Run the command below to install these dependencies.
You will need to add a PSR-4 Autoloader configuration so that the classes created in this tutorial will be available throughout the application. To do this, in composer.json add the JSON snippet below after the already existing code.
Then, run the following command to update Composer's autoloader file (vendor/autoload.php).
Creating the mock class
Now that all the dependencies are installed, the next step is to create the class that will return the mock data. To do that, create a new file named Lookup.php in the app/Twilio directory. Then, paste the code below into the new file.
The class has a single property, named $data
, which is an array used to hold any data returned from the request. It has two methods: fetch()
and validationResult()
. The fetch()
method returns the data to the user once the method is invoked. The isPossibleNumberWithReason()
method of the phone library is a method that validates the given number and returns integers from 0-3. The validationResult()
method replaces these integer codes with their corresponding human-readable codes since the libphonenumber
package returns the validation result as an integer.
The most crucial part of the application has now been created. All that is left is bootstrapping the Slim application and testing it. To do so, create a new file named index.php in the public directory. Then, paste the code below into the file.
The code imports the required classes and initializes the Slim application with a single route. The route is restricted to handling GET requests. It receives a number which is passed to Lookup
's fetch()
method, then the result is returned. Finally, the application is launched, by calling its run()
method.
Test the application
With the application complete it's time to run it with PHP’s built-in web server and test it.
For testing, run the curl command line below after replacing <<PHONE NUMBER>>
with a valid E.164 formatted phone number.
This should return a response similar to the following.
That’s how to mock the Twilio Lookup API
Mocking plays an essential part in development, and in this tutorial we went through how to mock Twilio's Lookup API. However, given the mockup's numerous limitations, it is important to note that this should only be used in lieu of the actual Lookup API during development, rather than as a replacement.
Happy building!
Prosper is a freelance Laravel web developer and technical writer that enjoys working on innovative projects that use open-source software. When he's not coding, he searches for the ideal startup opportunity to pursue. Find him on Twitter and Linkedin.
"Look Up Klosterhof Leipzig" by Tenzin Peljor is licensed under the CC BY-SA 2.0.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.