How to Send an SMS With Spark and Java
Time to read: 4 minutes
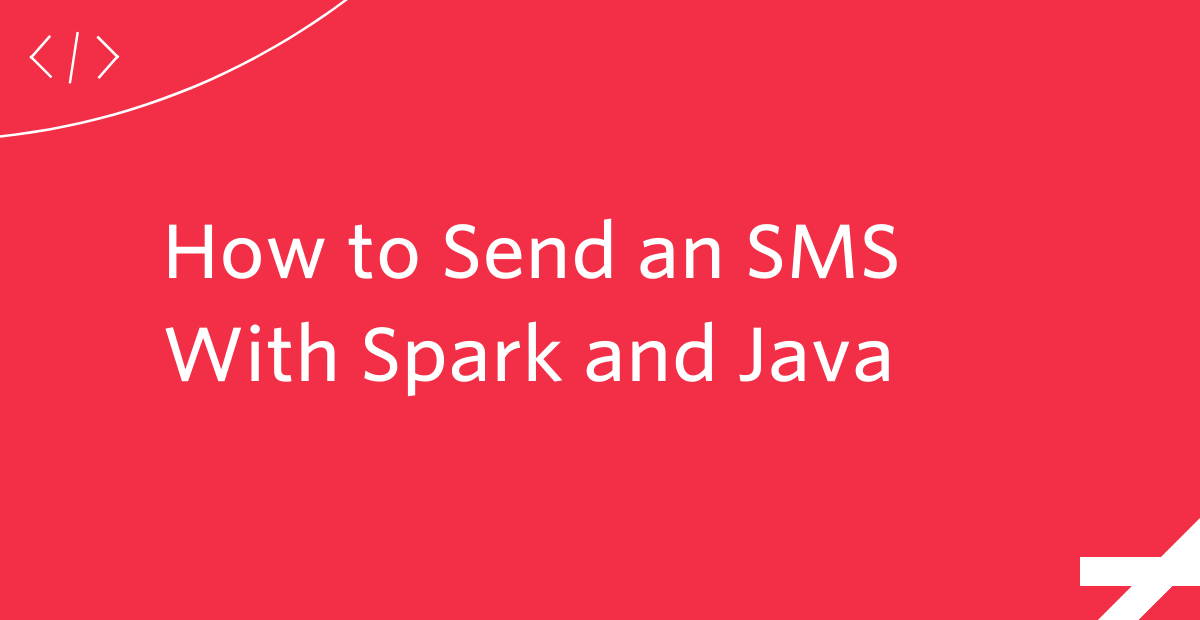
Twilio is all about powering communication and doing it conveniently and quickly in any language.
With the help of the Twilio SMS API, Java, and the Spark framework, you can respond to all incoming SMS with a text message. This article will help you set up a Spark application with a lightweight framework allowing you to be more productive in expanding and developing the project further.
In this article, you will learn how to navigate a Java IDE to set up and build a Java Spark application and send an SMS to your mobile device.
Tutorial requirements
- Java Development Kit (JDK) version 8 or newer.
- IntelliJ IDEA Community Edition for convenient and fast Java project development work. The community edition is sufficient for this tutorial.
- A free or paid Twilio account. If you are new to Twilio get your free account now! (If you sign up through this link, Twilio will give you $10 credit when you upgrade.)
- ngrok, a handy utility to connect the development version of the Java application running on your system to a public URL that Twilio can connect to.
Install ngrok
Ngrok is a great tool because it allows you to create a temporary public domain that redirects HTTP/S requests to a local port, such as 4567
or anything else such as 8080
.
Visit the ngrok website and log into your account. Download the ngrok zip file and follow the instructions on the site for your appropriate operating system. If you downloaded ngrok straight to your desktop, then you will see the executable file icon as seen here near the desktop toolbar.
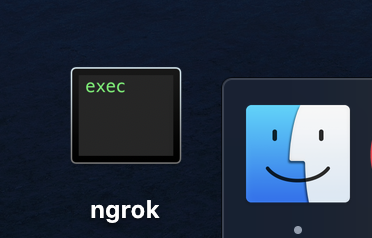
Depending on where you extracted the ngrok zip file, you might need to type ./ngrok
instead of ngrok
into your terminal. If you are a Windows or Linux user, look into how you can set ngrok in your system path for your convenience.
Go ahead and test out ngrok then kill the process by pressing Ctrl+C to move forward with the tutorial.
Start a new Java project in IntelliJ IDEA
Open IntelliJ IDEA and click on Create New Project.
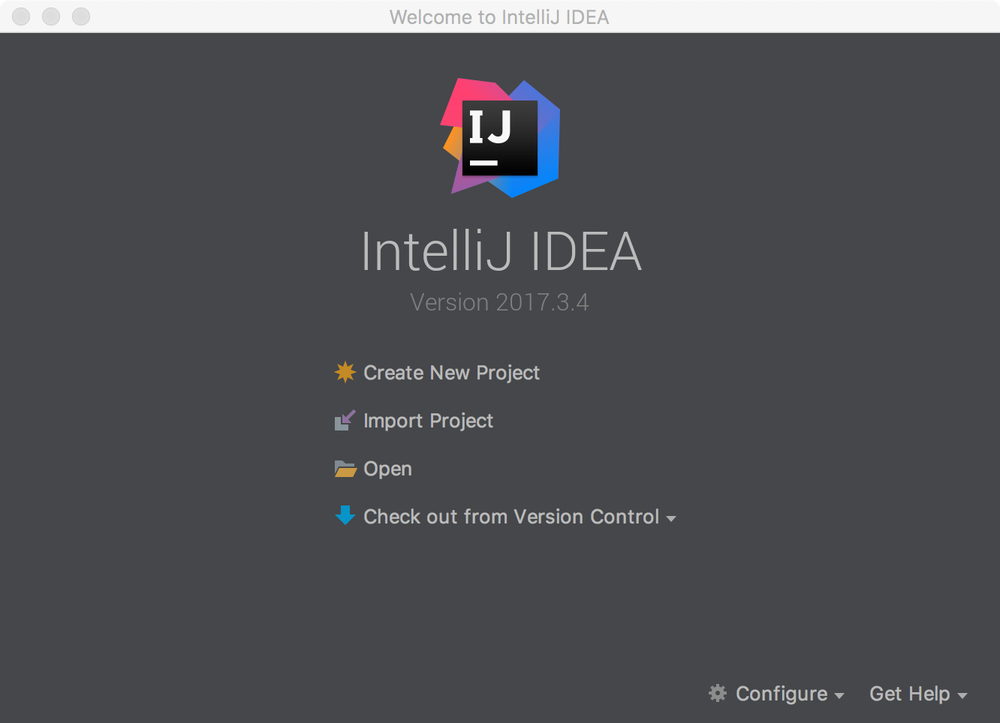
Choose Gradle on the left hand side and check Java in the box on the right hand side.
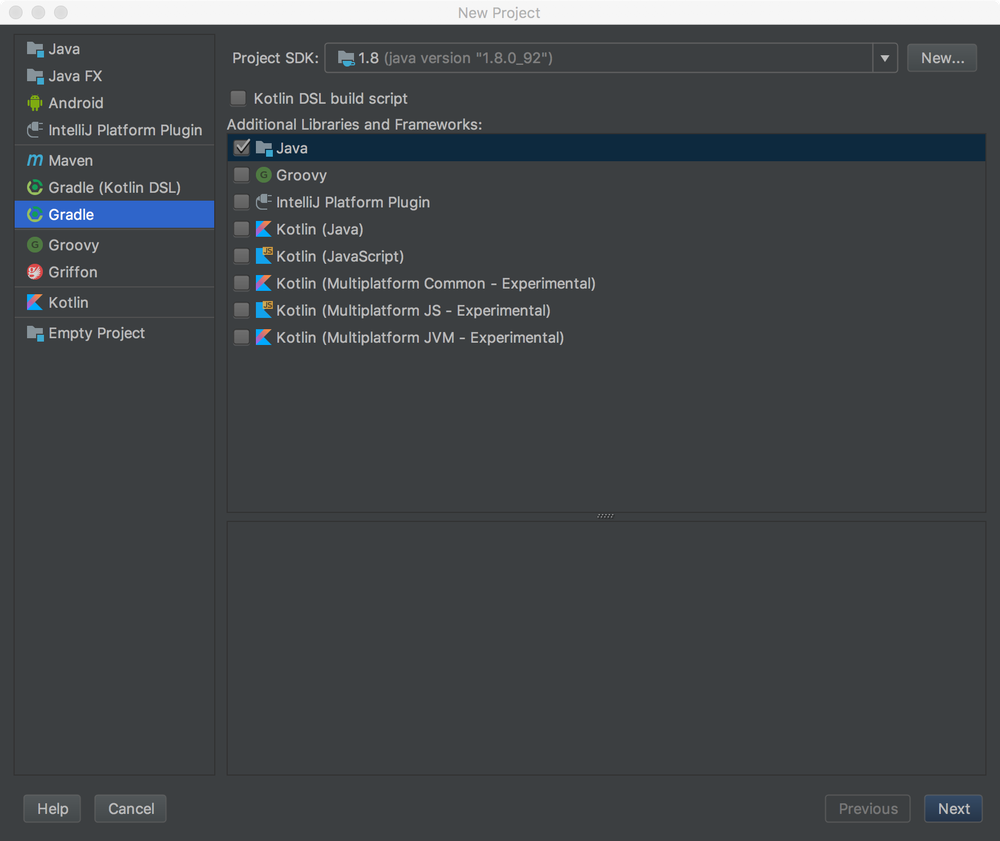
Give your project a name such as "SmsApp" and click the Finish button.
After the project setup is complete, your project directory structure should look like the following image:
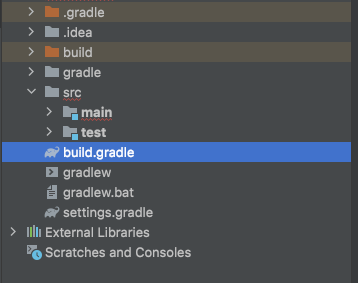
Open the build.gradle file in the IDE and add the following lines inside the dependencies
block:
Create the SMS app's Java class
Expand the main subfolder under the src folder at the root of the project directory. Notice the empty java folder.
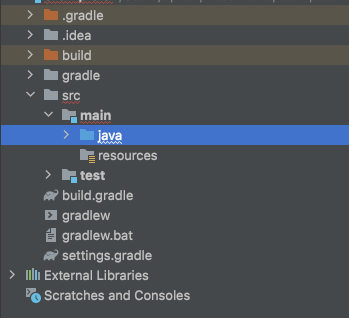
Right click on the Java folder and click on the New option. Select Java Class as seen in the image below. Clicking this option will prompt you to give the class a name. Go ahead and name it "SmsApp".
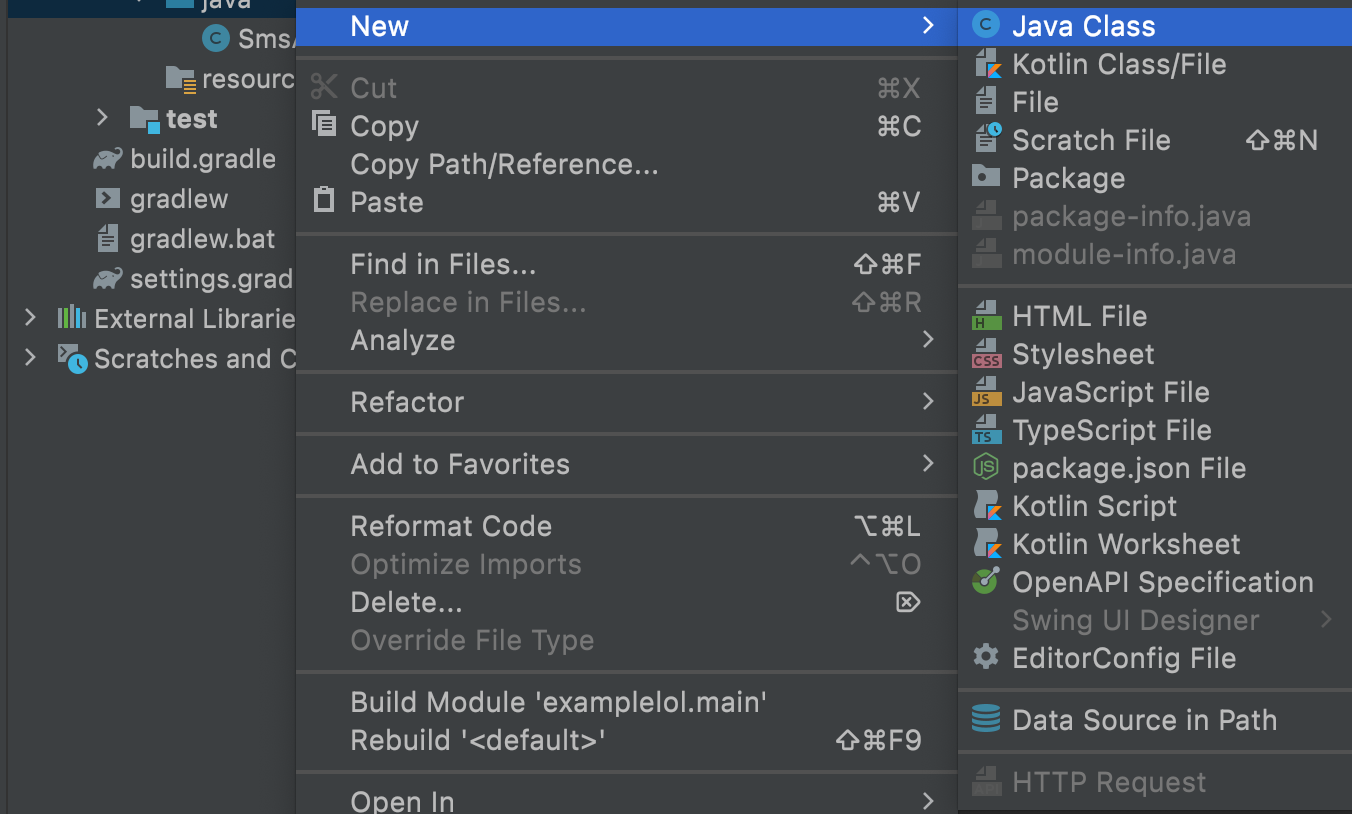
Copy and paste the following code into the newly created file:
In the public SmsApp
class, a main
method is invoked so that the code in the program starts once executed.
The main
method defines two routes:
- A GET route,
/
, which responds to all requests to the root URL"/"
with a string message. - A POST route,
/sms
, which responds with TwiML markup for an SMS message. ThisBody
object is created to hold the string message.
Feel free to change the string message, otherwise, go ahead and save the file.
Run the Java Spark application
Right click on the SmsApp file in the project interface and find the option to Run 'SmsApp.main()'. Wait a few seconds for the project to build, download the project's dependencies, and compile.
Spark automatically runs applications on port 4567 so head over to http://localhost:4567/ in your web browser to see the string message.
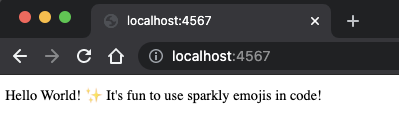
Set up the webhook with Twilio
Open a new terminal window, and start ngrok with the following command, to make the Java application publicly available over the Internet:
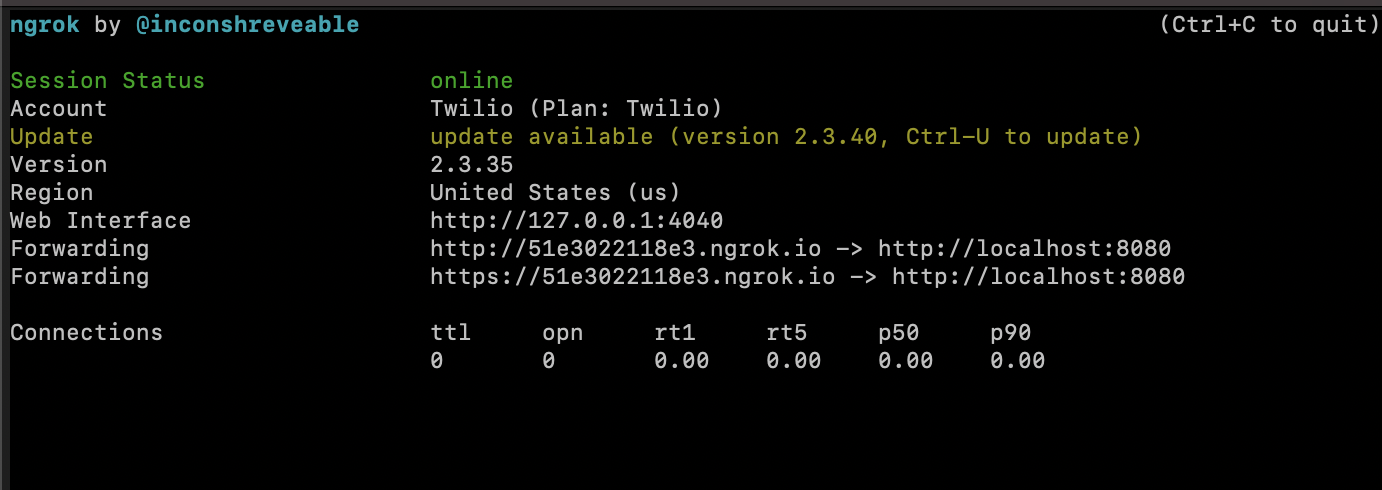
Your ngrok terminal will look similar to the image above. As you can see, there are URLs in the “Forwarding” section. These are public URLs that ngrok uses to redirect requests into our Java servlet.
Let the application run in the background and open the Forwarding HTTPS URL in your web browser. The URL from ngrok in my example is “https://ad7e4814affe.ngrok.io/sms”.
NOTE: Keep in mind that this URL is no longer active and only presented here for demonstration purposes.
You should be able to see the same view as if you were on localhost:4567.
Copy the Forwarding URL starting with https://
and open the Twilio Console, where we will tell Twilio to send incoming message notifications with this URL.
In the Twilio Console, click on the left sidebar to explore products, and find Phone Numbers. Click on the active phone number that you want to use for this project and scroll down to “Messaging”.
There, paste the URL copied from the ngrok session into the A MESSAGE COMES IN field and append /sms
, since that is the endpoint. Before you click on the Save button, make sure that the request method is set to HTTP POST
.
Here is my example for reference:
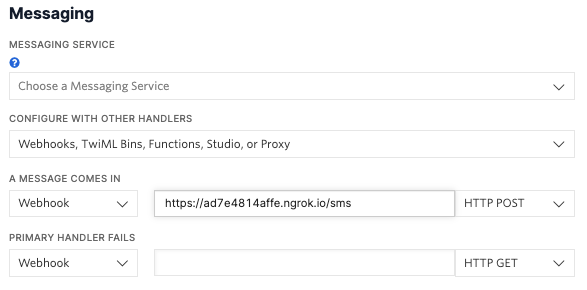
Before you click on the Save button, make sure that the request method is set to HTTP POST
.
Run the Twilio SMS Spark Application
Send a text message to your Twilio phone number on your mobile device. Wait a few seconds and you should see the TwiML message response on your device.
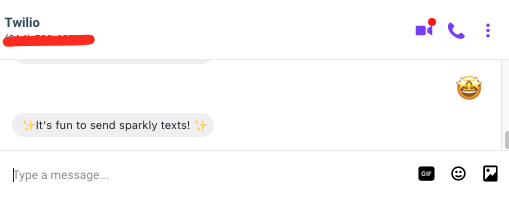
What's next for building Java projects?
Congratulations on sending an SMS text message on your Java Spark application! But don't stop here. Check out these articles on how you can build more with Twilio and Java:
- Create a standalone voicemail using Java and Twilio.
- Make a button that sends a WhatsApp message using Java.
- Allow the button to trigger a voice call with Java and Twilio.
- Explore projects with Java servlets.
- And so much more!
Let me know about the projects you're building with Java and Twilio by reaching out to me over email!
Diane Phan is a software developer on the Developer Voices team. She loves to help programmers tackle difficult challenges that might prevent them from bringing their projects to life. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.