Respond to Incoming SMS with NodeJS and Google Cloud Functions
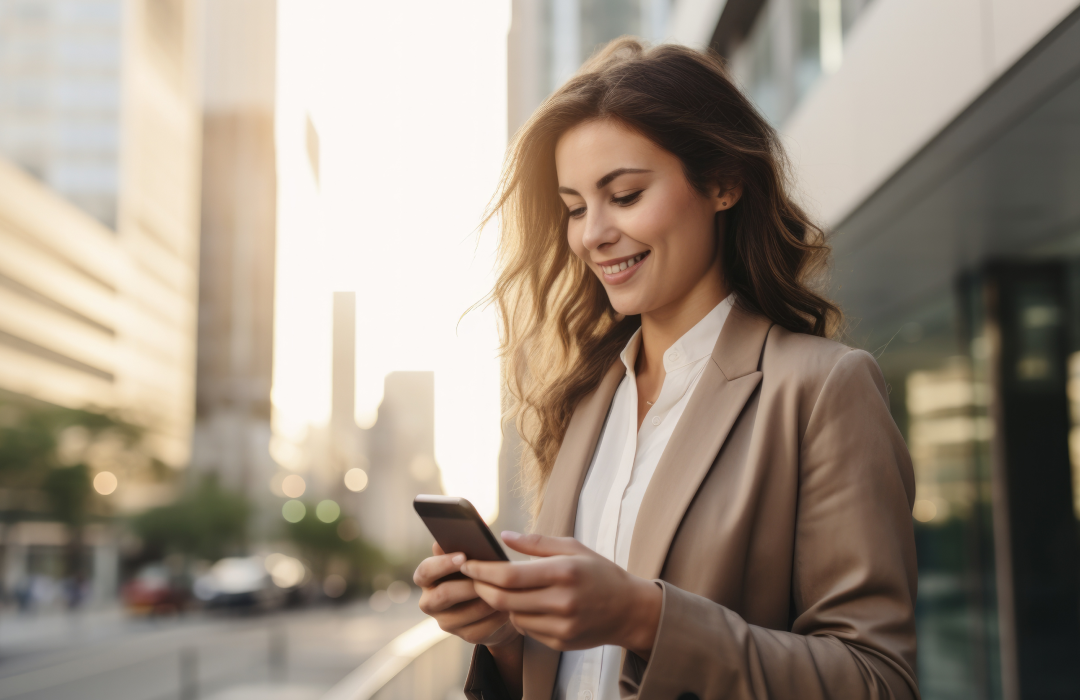
Time to read: 3 minutes
Respond to Incoming SMS with NodeJS and Google Cloud Functions
In today's fast-paced world, businesses have realized the power of instant communication with their customers via SMS and WhatsApp. But just sending out messages doesn’t live up to a good customer experience. Customers also expect to be able to respond to these messages. And your apps should do so with clever messages. If that excites you, you're in the right place!
In this article, you'll explore how to architect a robust mechanism for managing incoming SMS using Google Cloud Functions (Pub/Sub), and Node.js.
Prerequisites
Our journey demands that you come pre-equipped with the following:
- A Twilio Account: Sign-up here
- A Google Cloud Account: Register at Google Cloud's website to open an account.
Let's gear up and embrace the exciting and challenging art of reciprocating incoming SMS messages using Google Cloud Functions and Node.js.
Handling Incoming SMS with Google Cloud Functions
A webhook is a method used by web applications to communicate with each other in real-time when a specific event occurs. They can be part of a traditional monolithic application, but they are especially suitable for serverless functions due to their scaling behavior. Let's put this into action:
Use the Power of Google Cloud Functions
Google Cloud Functions act as building blocks for serverless applications and allow you to run your code without provisioning or managing servers.
Go to the GCP Console to create a new Cloud Function. Click the blue Create Function button.
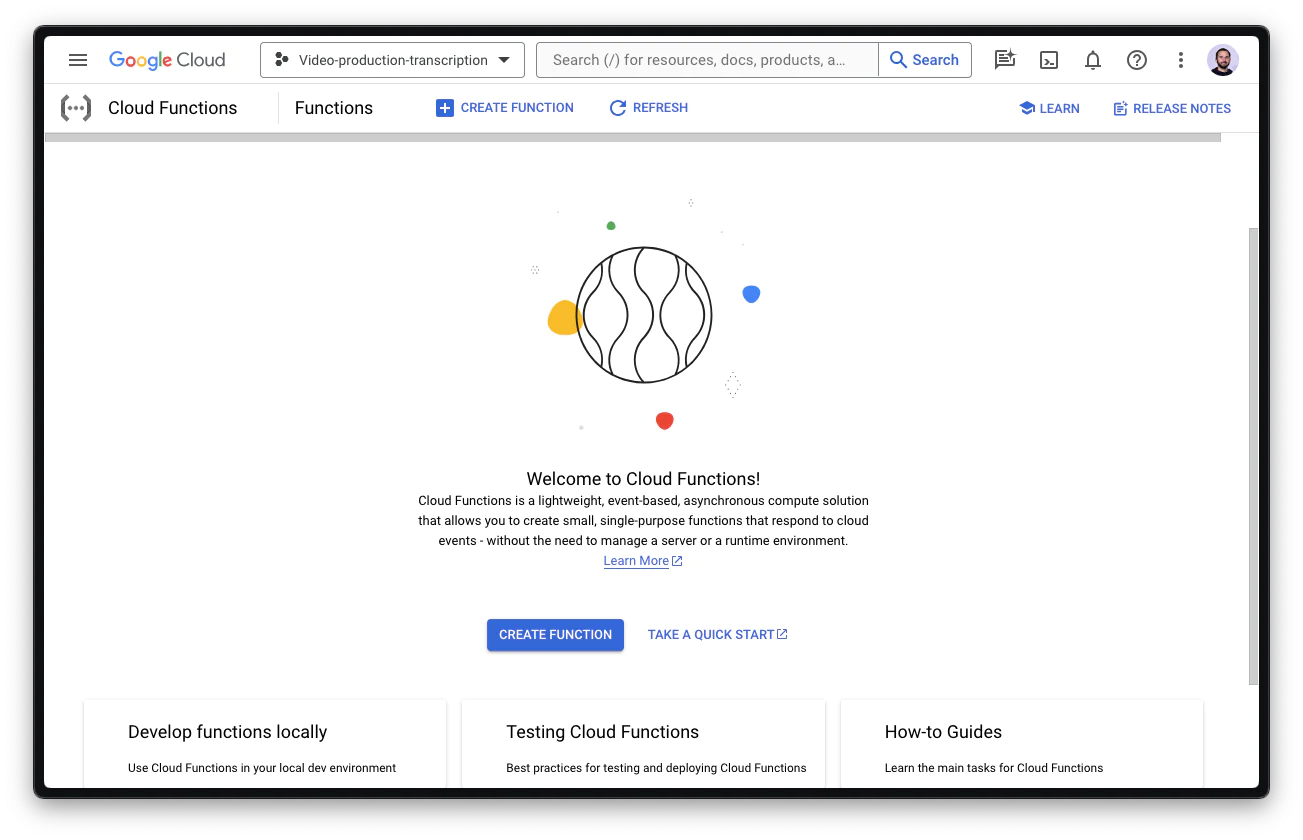
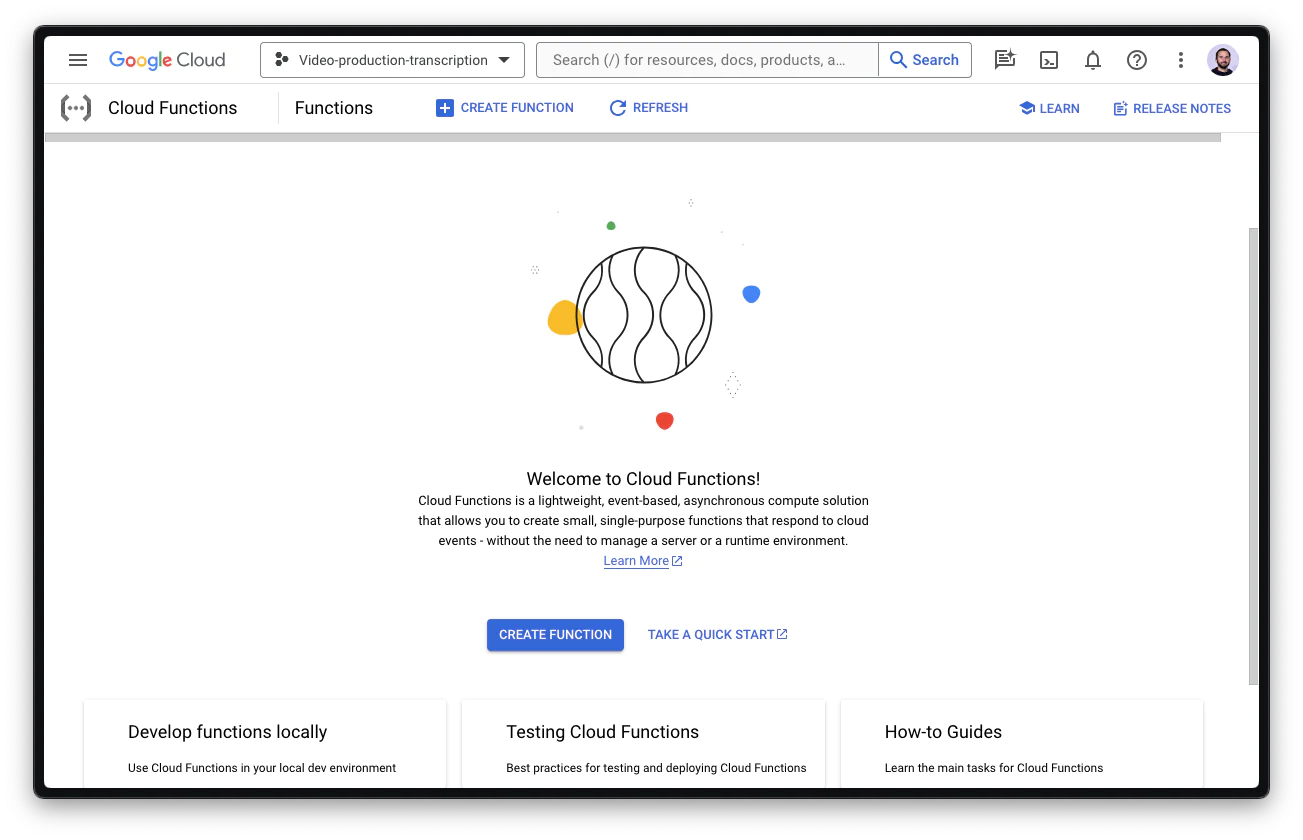
In the next dialog, give the new function a name like receive-sms. Make sure the HTTPS-based trigger is selected, and unauthenticated invocations are allowed. Only this way will Twilio be able to trigger the webhook.
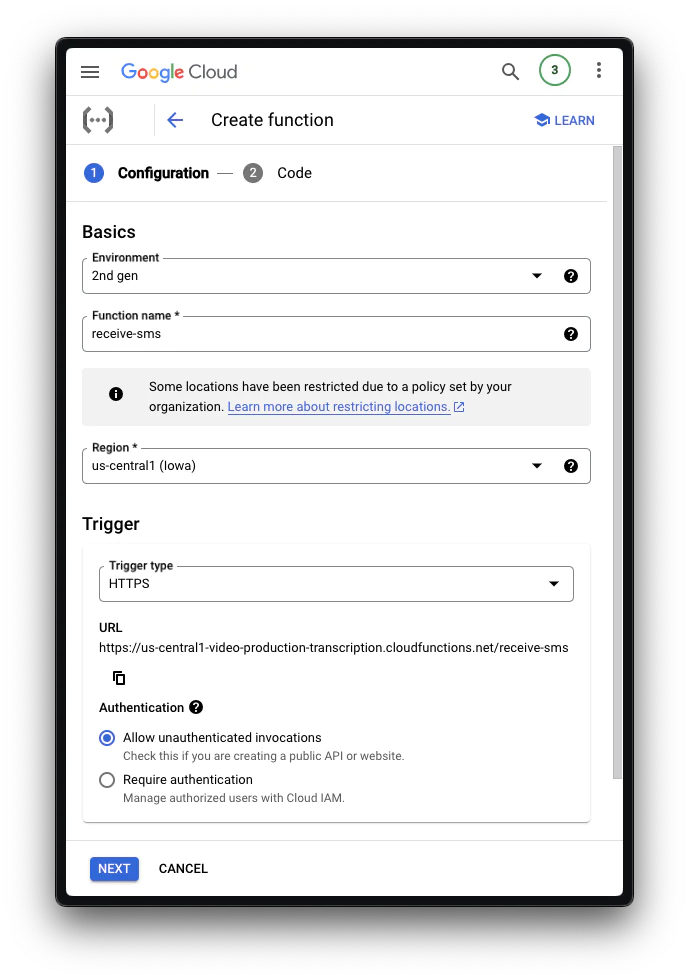
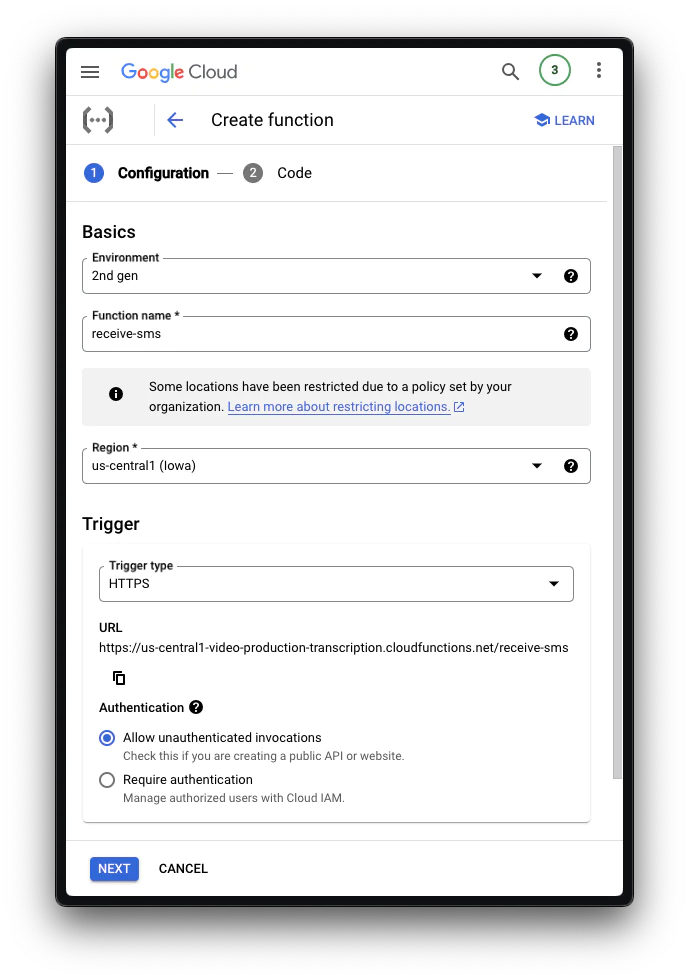
Hit Next to start implementing the Cloud Function. You might also need to enable another set of APIs.
Implement the Serverless Function
The next user interface shows you the Inline Editor to implement the function. First, add the twilio dependency in the package.json.
Then, implement the function that uses the `twilio` package to create a TwiML response that includes a random Dad Joke.
Click Deploy to activate the Cloud Function. After deployment, you should see the URL of the function and copy it for later.
Test the Function
You can test this function in a new browser tab, since this function explicitly allows unauthenticated inbound traffic. This is great. Alternatively, you can also use curl:
In production, you would want to verify the signature of the request to make sure it’s sent by Twilio and no one else. It might also make sense to bundle multiple Cloud Functions behind an API Gateway .
Purchase the phone number you want to use
To send a message, you need to buy a phone number—ideally from the region you are based in—to avoid roaming charges. To accomplish this, go to the Twilio Console and search for a number you’d like to buy.
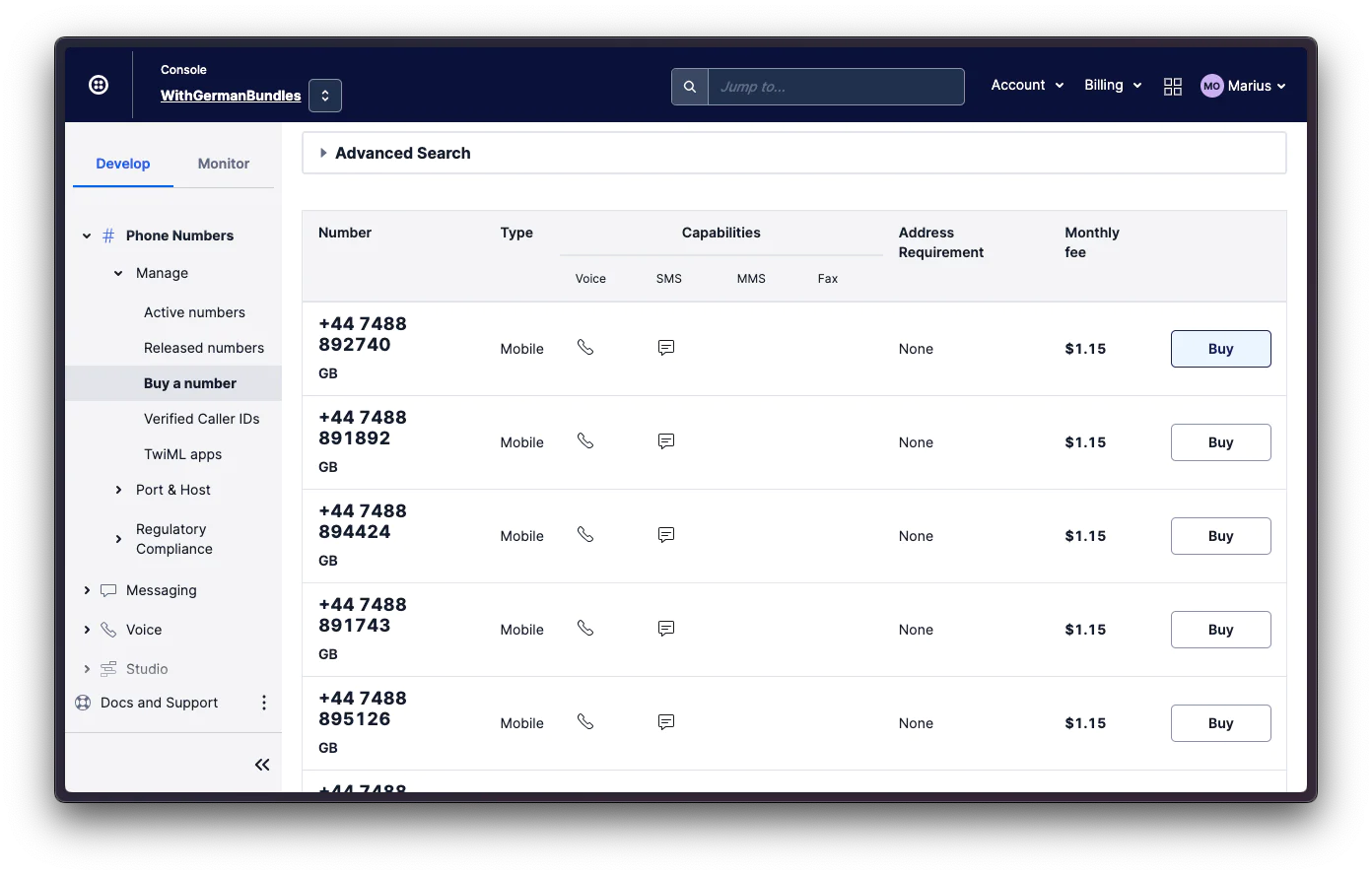
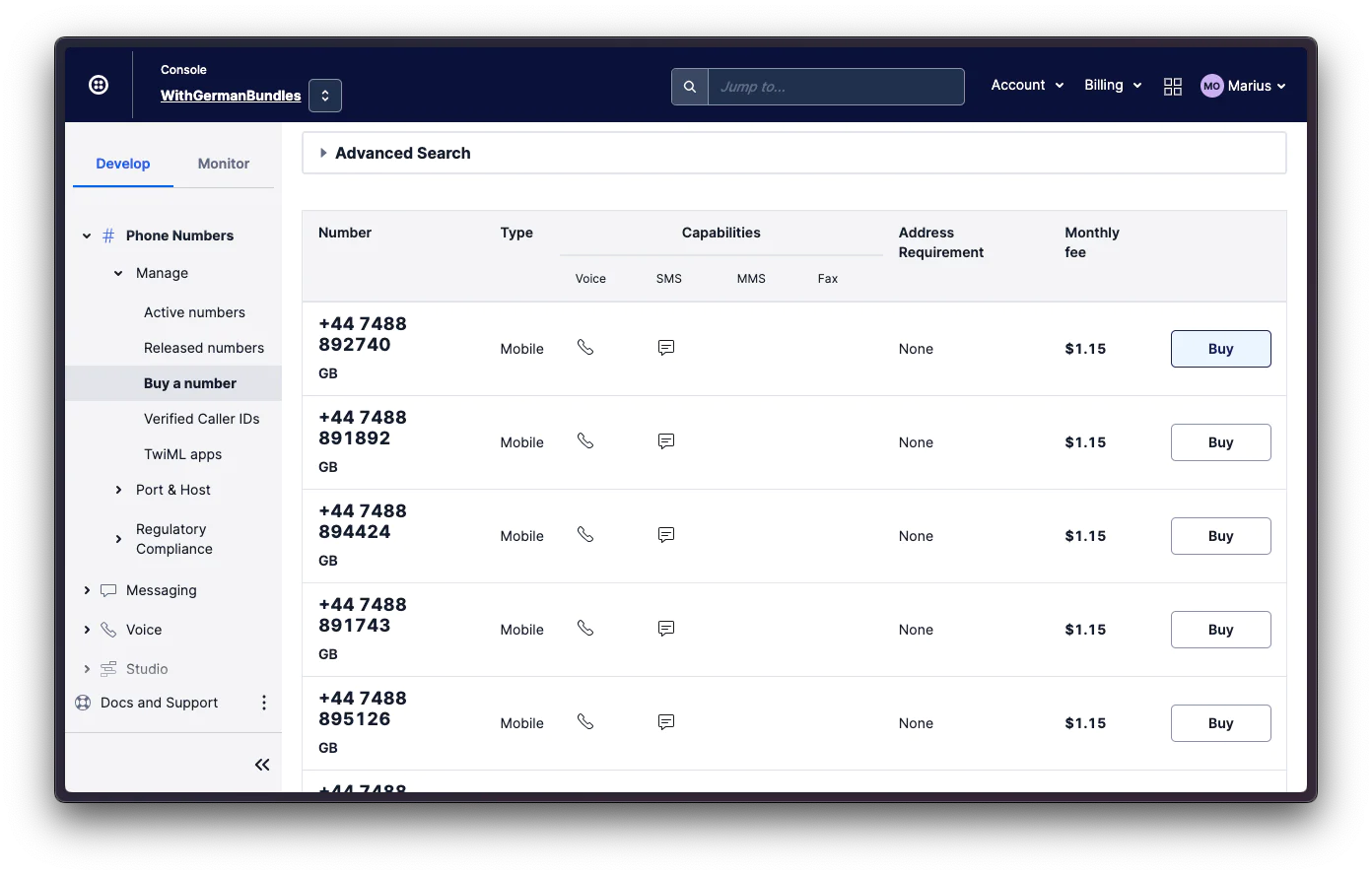
Register the Function as Webhook
Now it’s time to go to the Twilio Console and find the number you purchased earlier. Click on the number, go to the Configure tab, and find the Messaging Configuration box. In this box, there’s a section A message comes in. Make sure you add the URL of your function as a webhook and that it’s using HTTP POST.
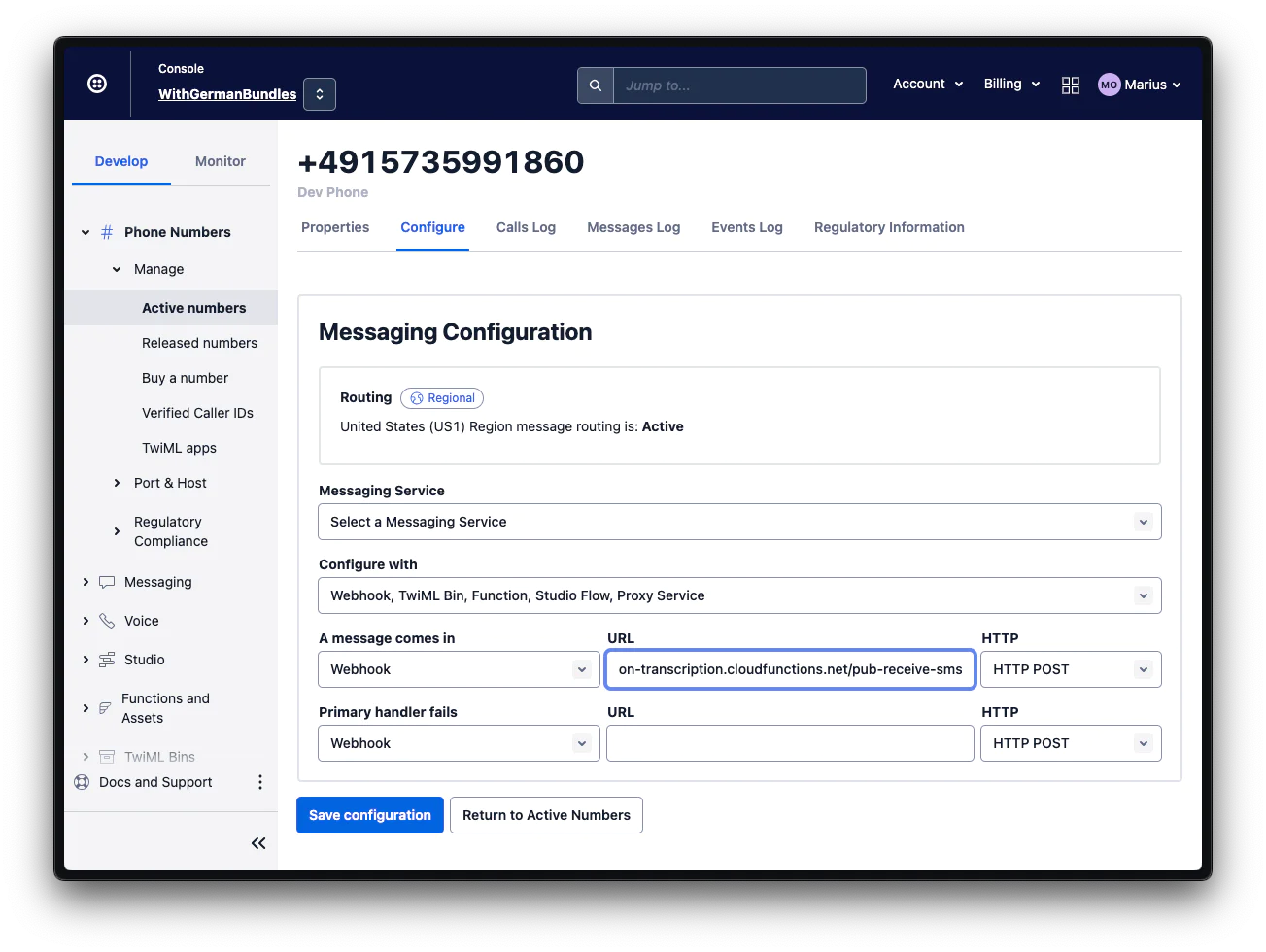
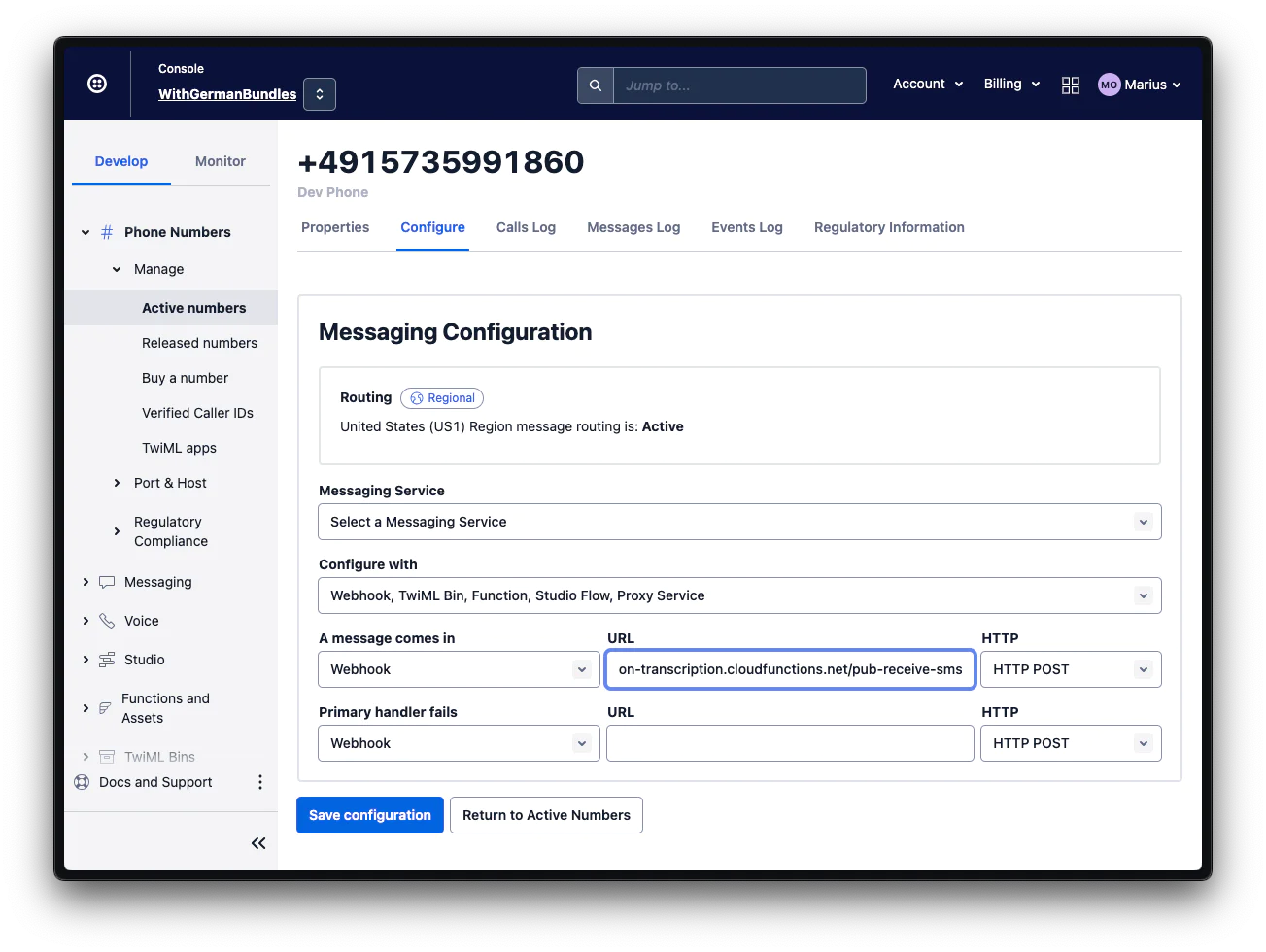
You've just simplified customer communication by automating the complex process of handling incoming messages. Feel the power yet? Maybe you start feeling it when you text that number and receive a “funny” dad joke.
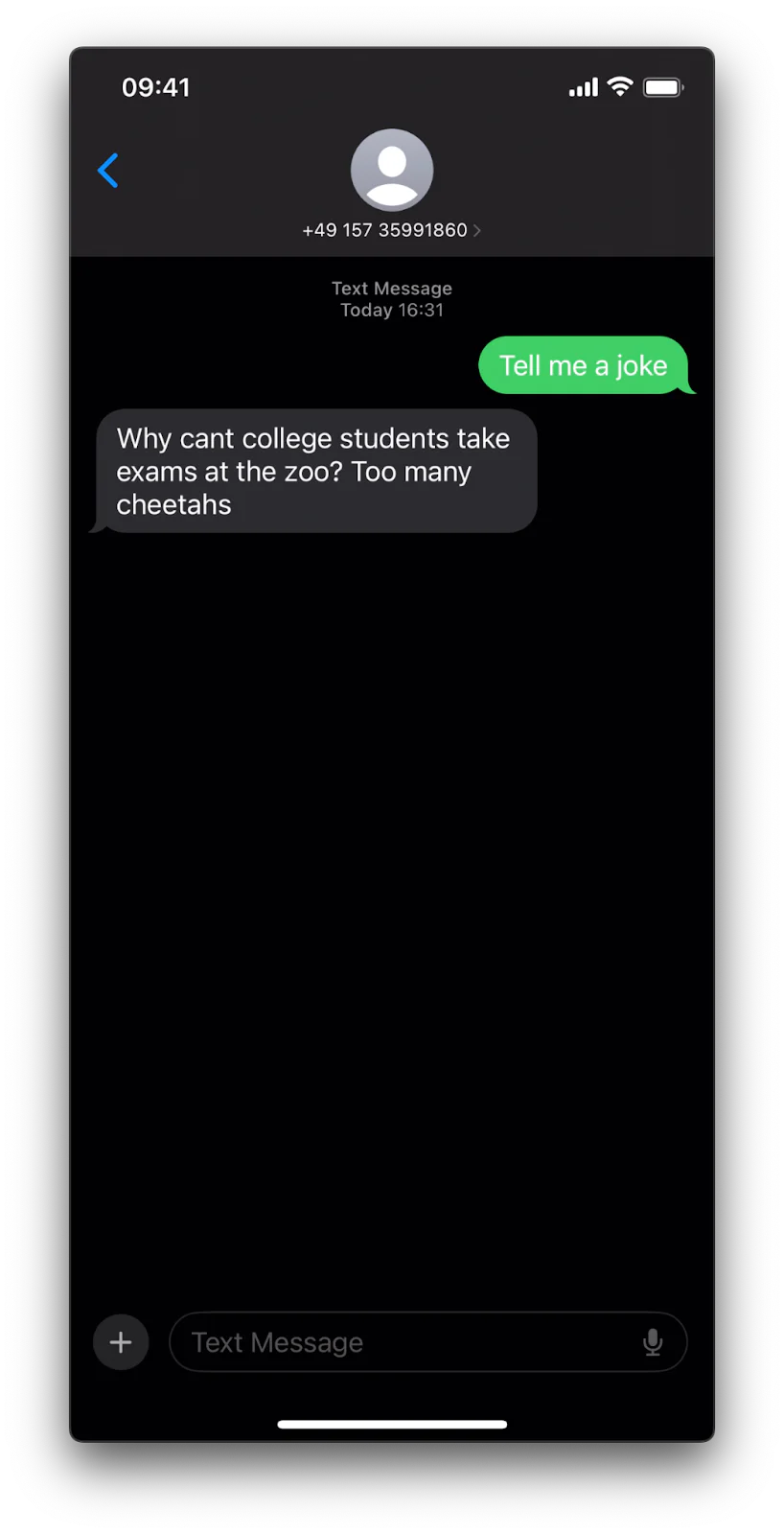
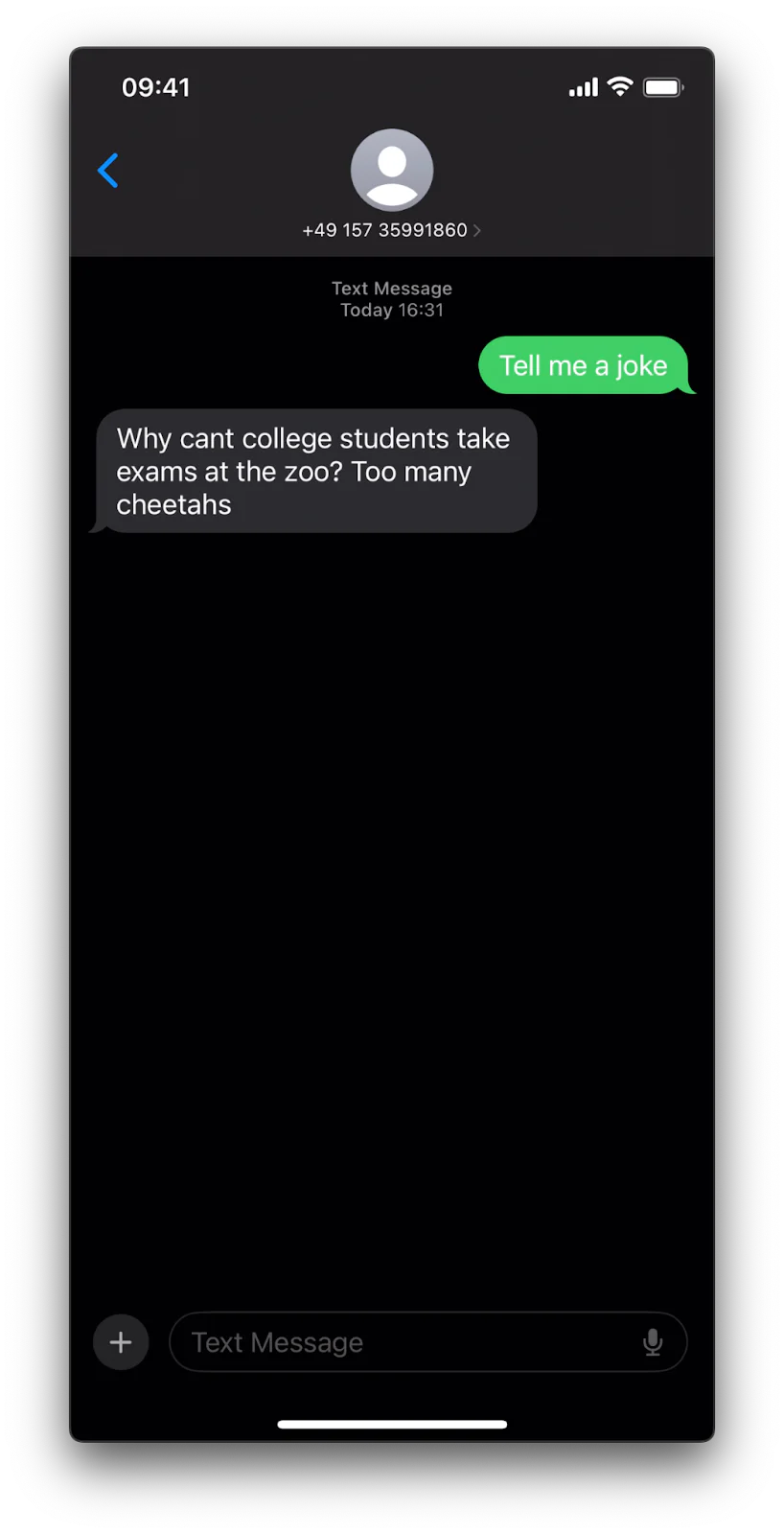
Conclusion
Handling incoming SMS with Google Cloud Functions opens up countless possibilities for better customer interactions. Especially if you have already built on Google Cloud Platform. It adds responsiveness and dynamism to your customer interactions - from quick acknowledgements to instant automated actions.
Let's keep feeding our curiosity, and never stop learning. I can’t wait to see what you build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.