How to Send SMS using NodeJS and Google Cloud Functions
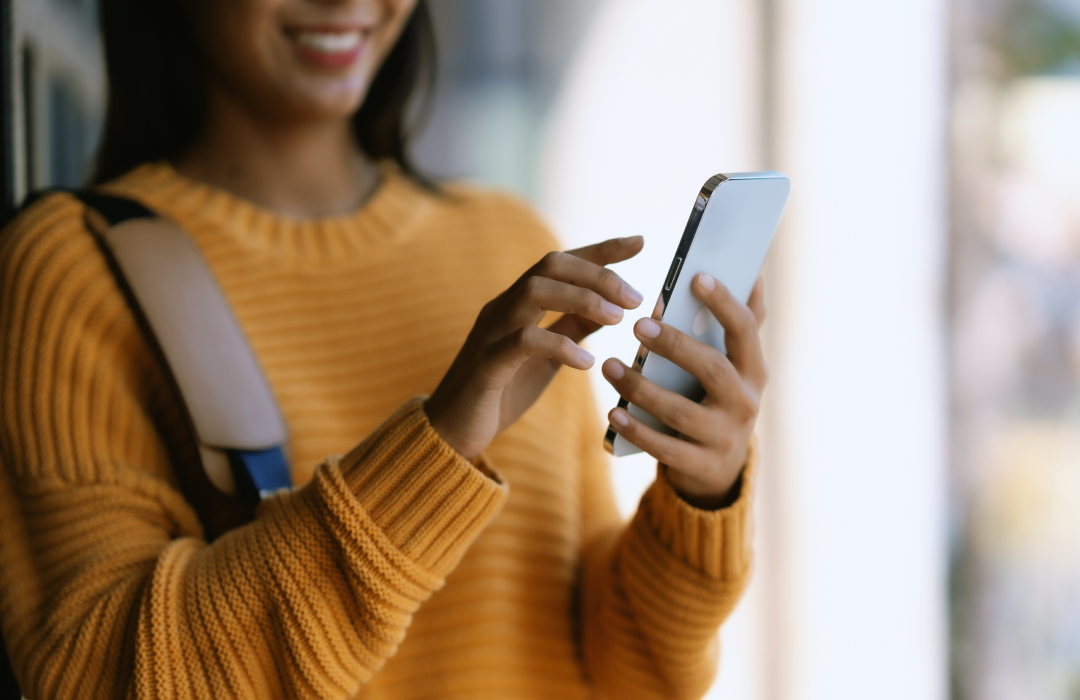
Time to read:
How to Send SMS using NodeJS and Google Cloud Functions
In today's high-speed, interconnected world, the ability to communicate quickly and effectively is paramount. Over the past couple of decades, SMS and messaging apps like WhatsApp have revolutionized the way businesses connect with their customers. They are significant tools for modern-day communication, and harnessing their power could add significant value to your business.
Understanding how to leverage such tools is an essential coding skill in our tech-driven world. This post will guide you on how to send SMS with Node.js using Google Cloud Functions , a light-weight, event-based, asynchronous compute solution that allows you to create small, single-purpose functions that respond to cloud events without the need to manage a server or a runtime environment.
Prerequisites
You need to ensure you have the following before moving forward:
- A Twilio Account: Sign-up here
- A Google Cloud Account: Register at Google Cloud's website to open an account.
- Optional: Install the gcloud CLI
Using Google Cloud Functions to send SMS
By following these steps, you can create your own SMS sending service using Google Cloud Functions. Just imagine all the value and convenience that it puts in your hands.
Leveraging the robust features of Google Cloud Functions to communicate quickly, effectively and efficiently.
Copy your Twilio Credentials
Once you are logged in to the Twilio Console , scroll down to find your Account SID and Auth Token. Copy these values, as you’ll need them later.
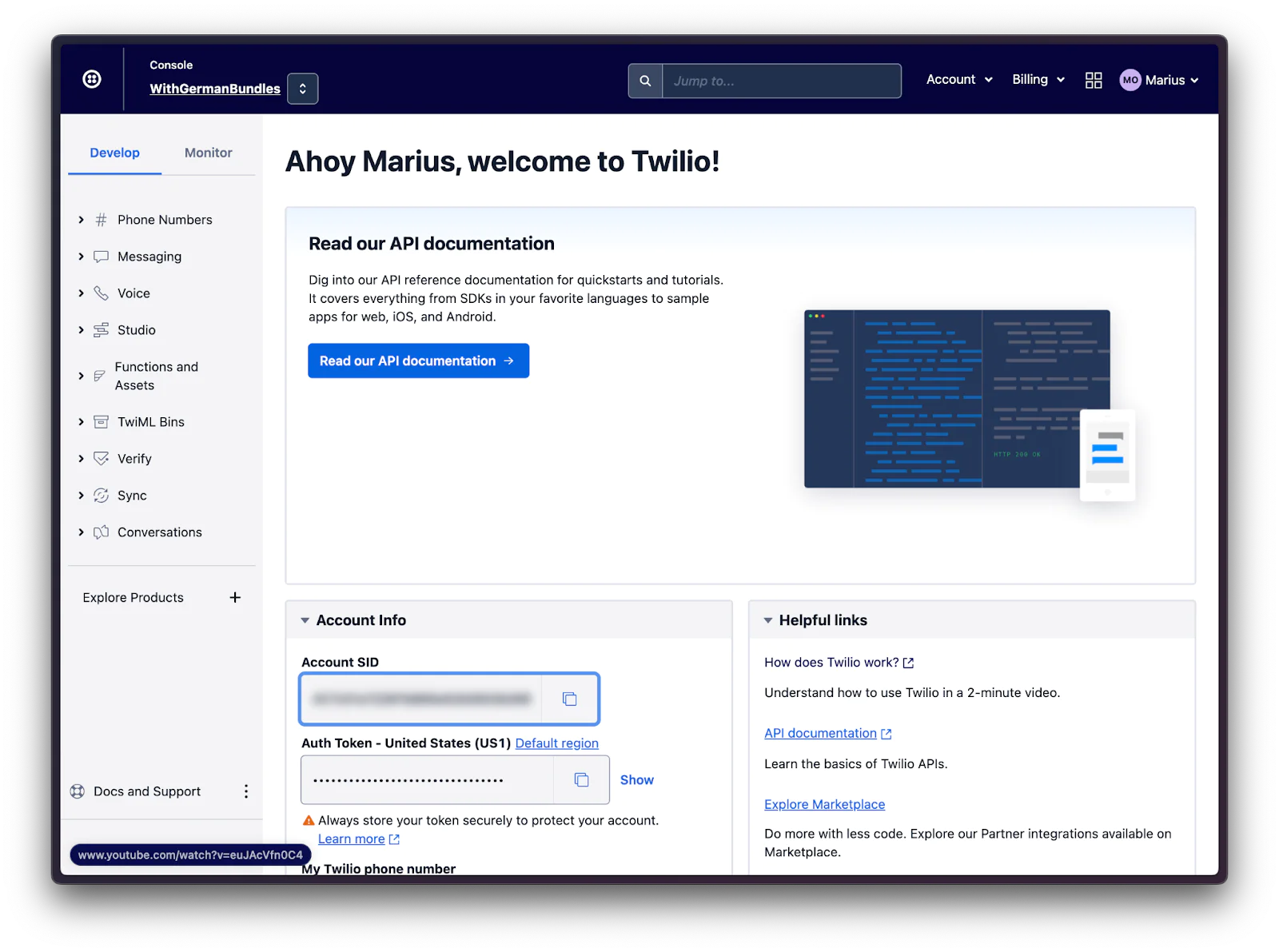
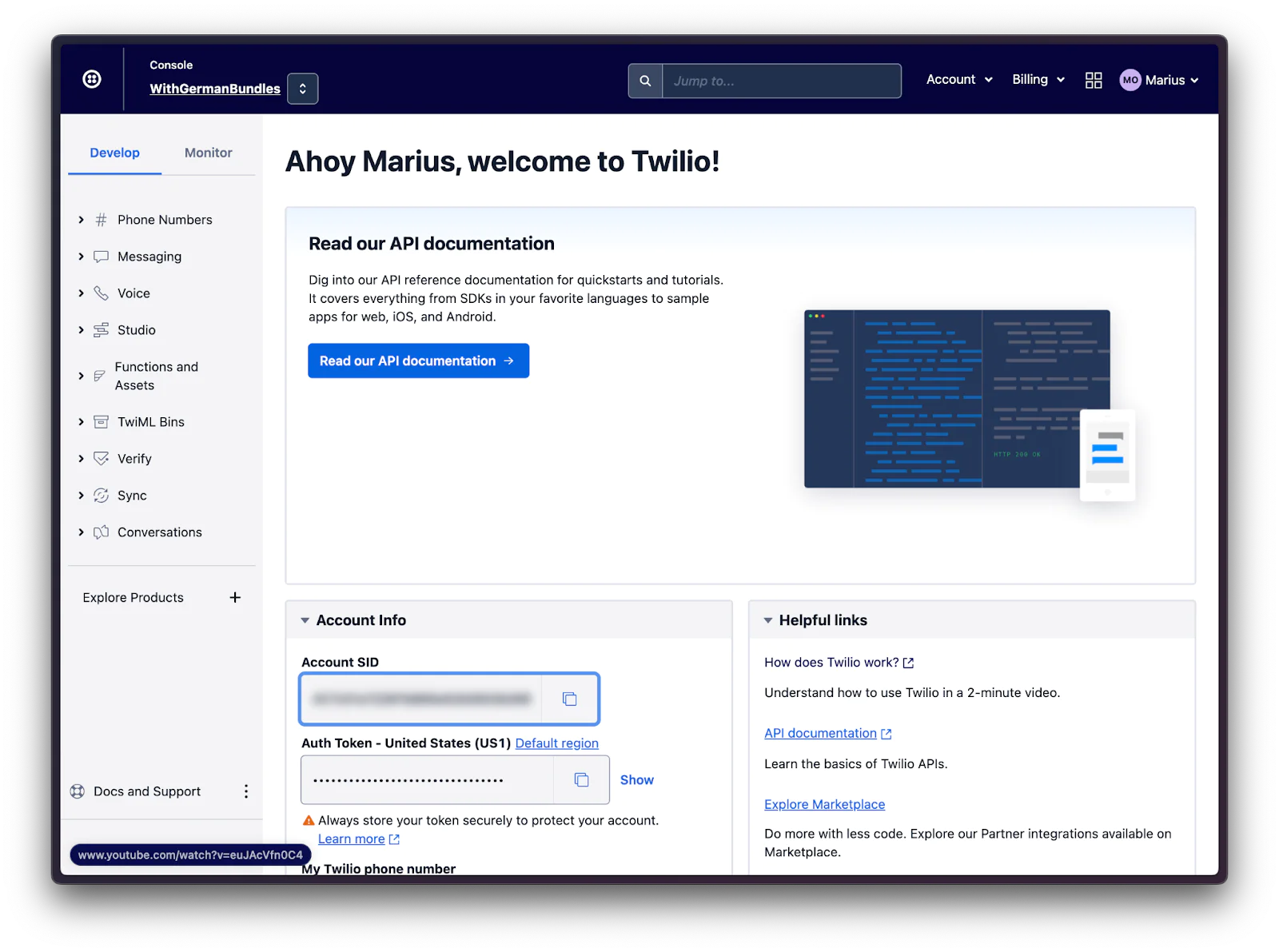
Purchase the phone number you want to use
To send a message, you need to buy a phone number—ideally from the region you are based in—to avoid roaming charges. To accomplish this, go to the Twilio Console and search for a number you’d like to buy.
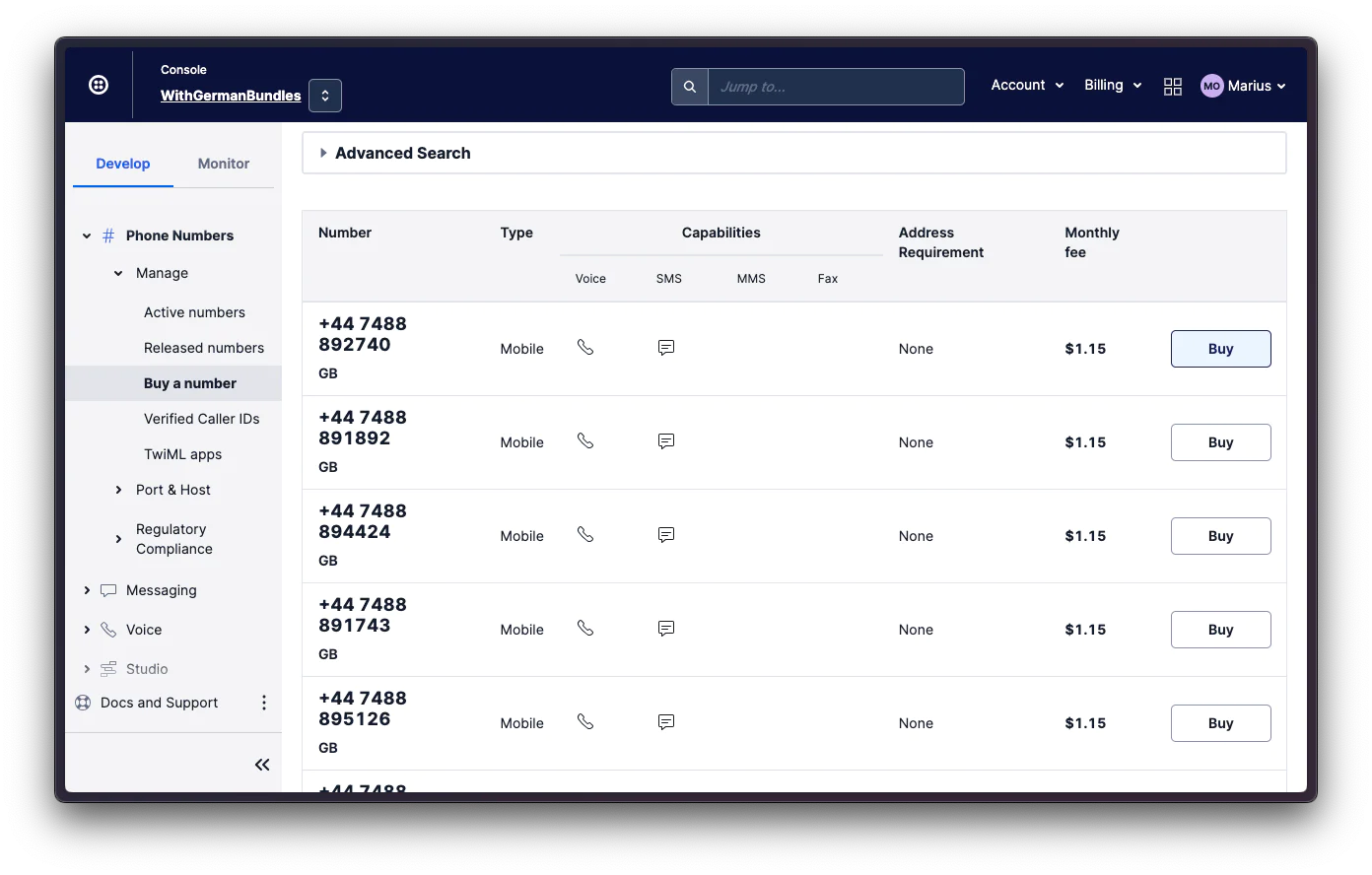
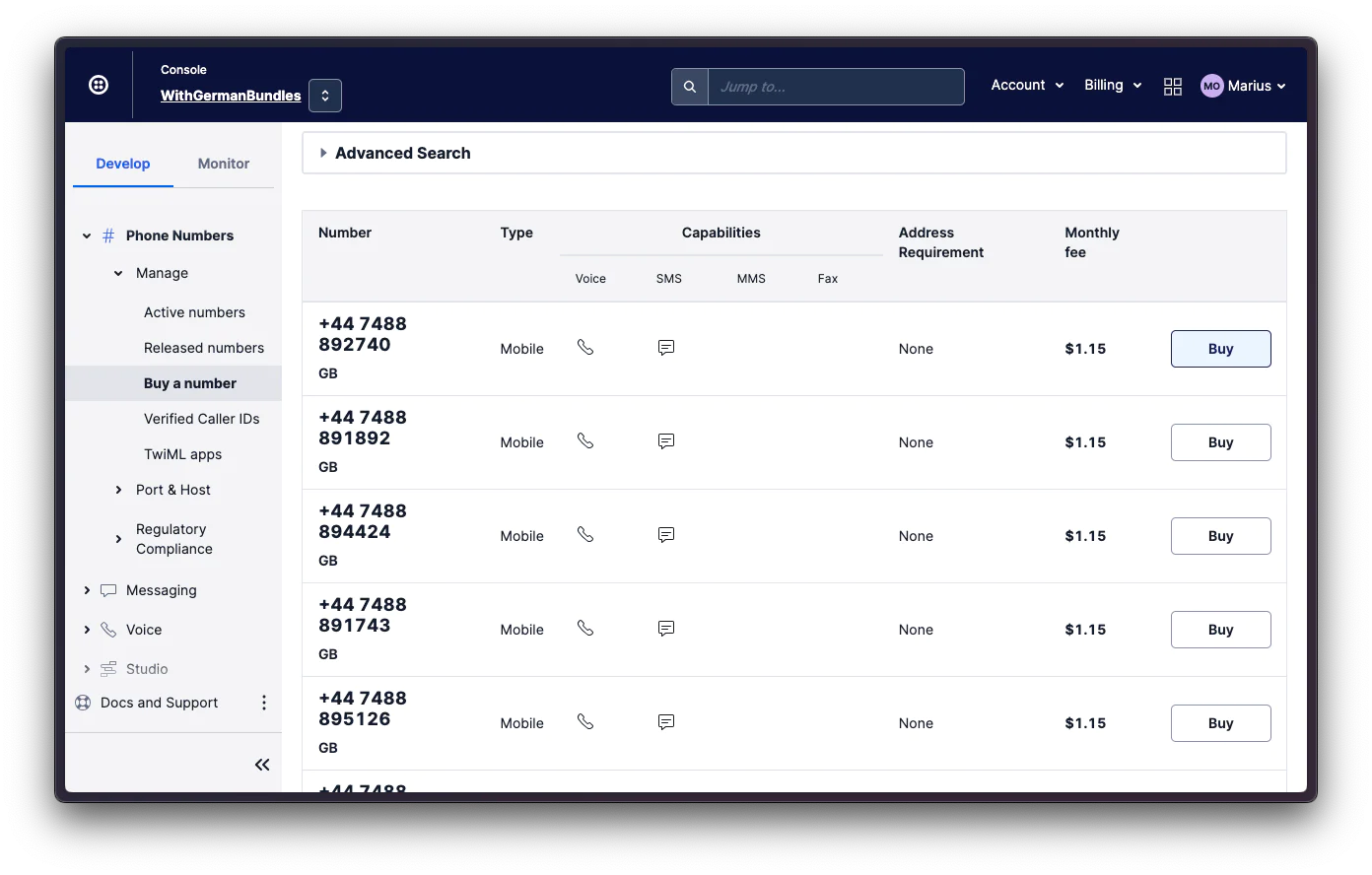
Also copy this number for later usage.
Use the Power of Google Cloud Functions
Google Cloud Functions act as building blocks for serverless applications and allow you to run your code without provisioning or managing servers.
Go to the GCP Console to create a new Cloud Function. Click the blue Create Function button.
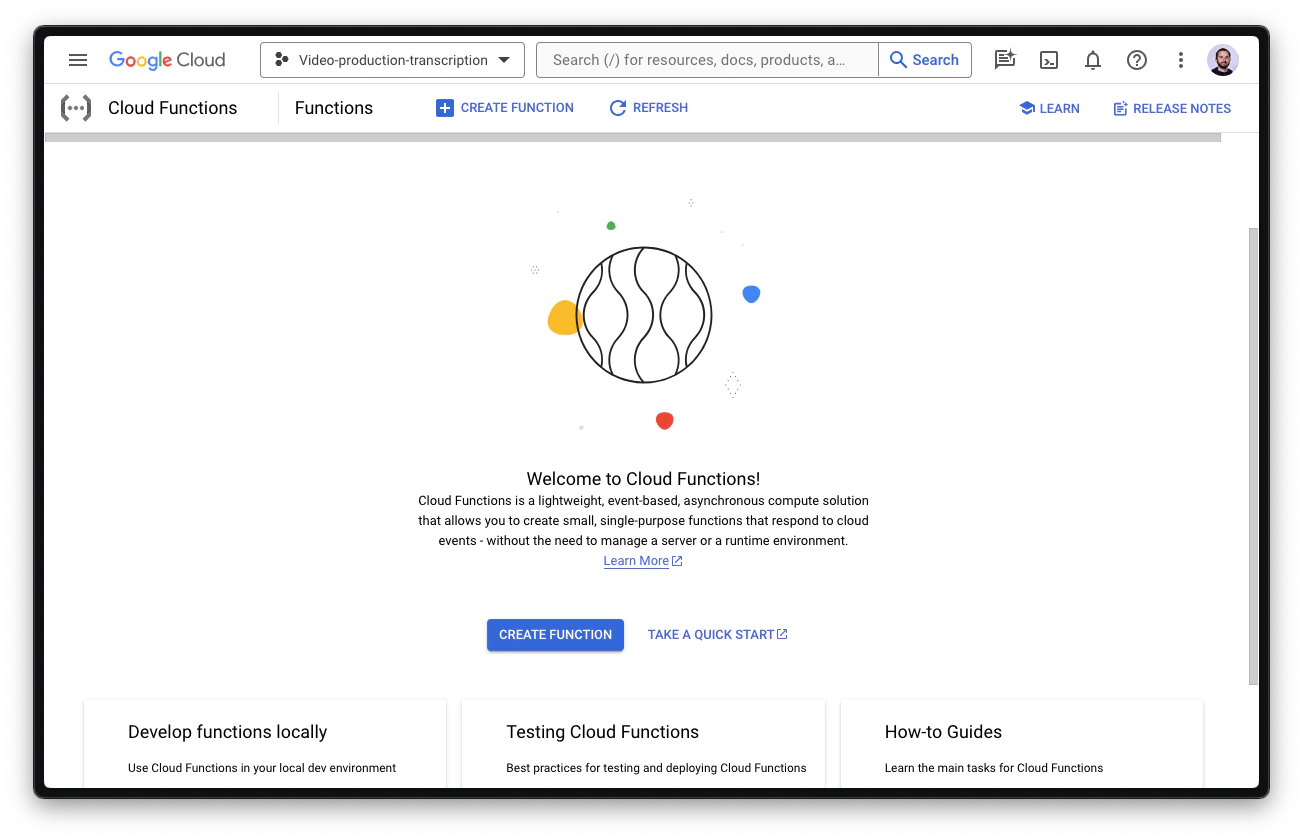
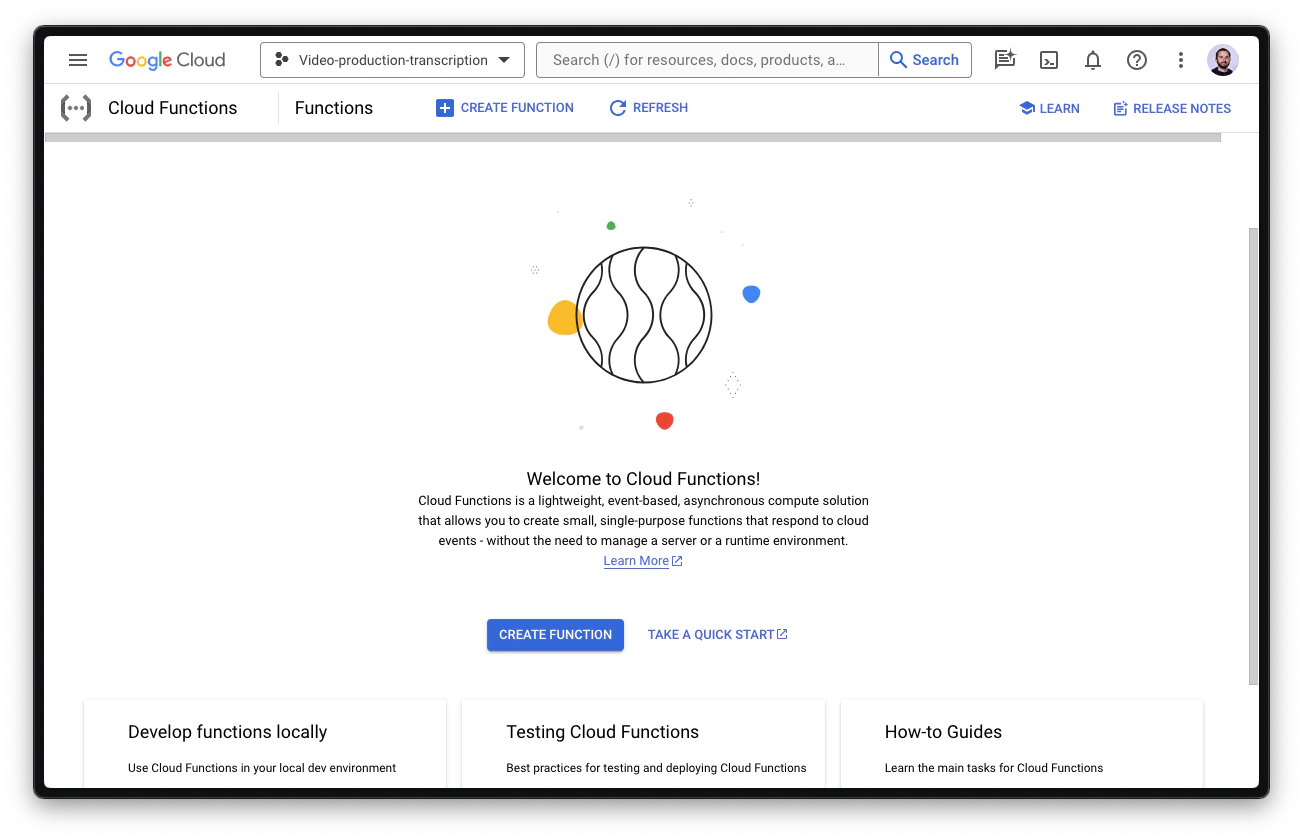
In the next form, give the new function a name like send-sms. For this demo, we’re happy with an HTTPS-based trigger. Only authenticated requests should be able to trigger the function and, hence, send text messages. Make sure to choose Require Authentication. If you have a larger footprint on GCP, it probably makes sense to use other triggers , like the Pub/Sub Messaging Service. In this interface, you can also define the environment variables for this function. Scroll down to Runtime, build., connections and security settings, and focus on the Runtime tab. Add the three values (TWILIO_ACCOUNT_SID, TWILIO_AUTH_TOKEN, and TWILIO_NUMBER) you copied earlier.
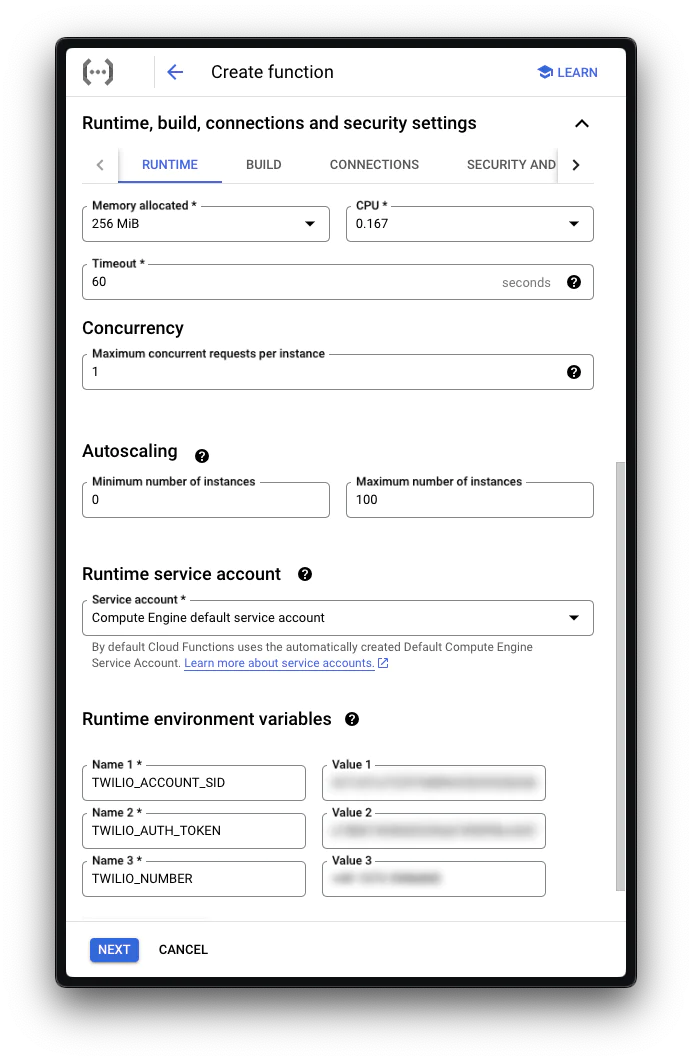
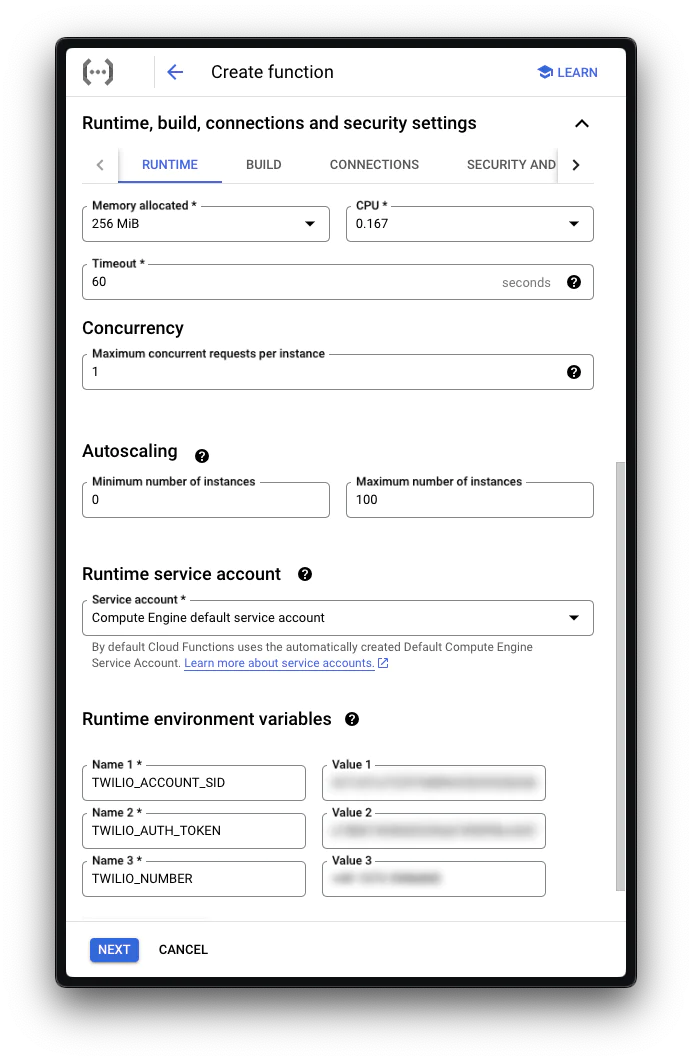
Hit Next to start implementing the Cloud Function.
Writing a Google Cloud Function in Node.js
The next user interface shows you the Inline Editor to implement the function. First, add the twilio dependency in the package.json.
Then, implement the function that uses the twilio package to send SMS based on the provided request parameters. The account credentials will automatically be retrieved from the corresponding environment variables:
Once you click Deploy the Google Cloud Function will be available under a URL that looks like this one:
Your function is now isolated, autoscaled, and responds to HTTP triggers.
Send a Test SMS with the Google Cloud Function
Go to the Testing tab of the function to define the test event. Make sure to add a valid phone number as recipient and a body. Now you can either test this function straight from the Cloud Shell or from your local machine when you copy the CLI test command.
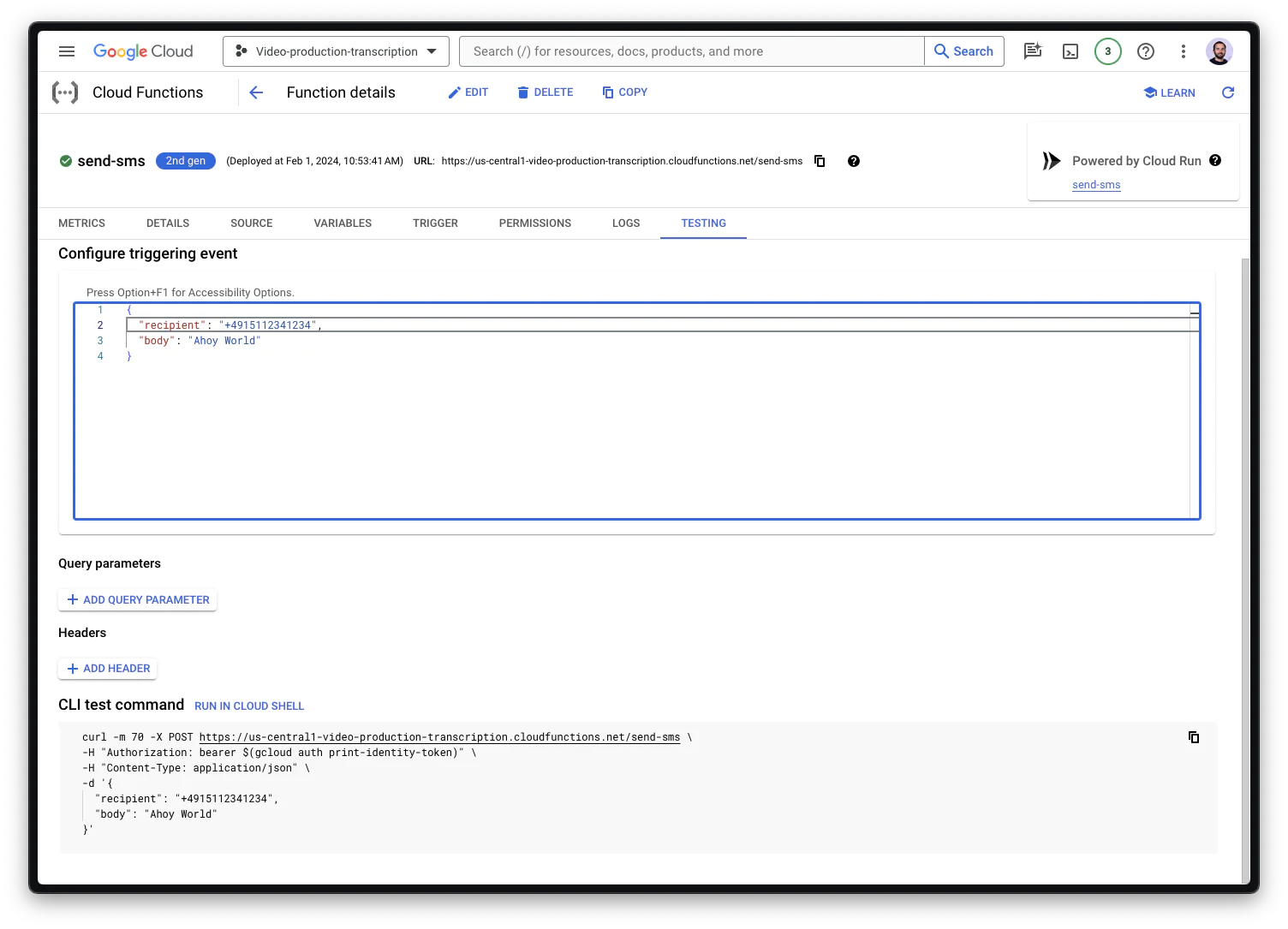
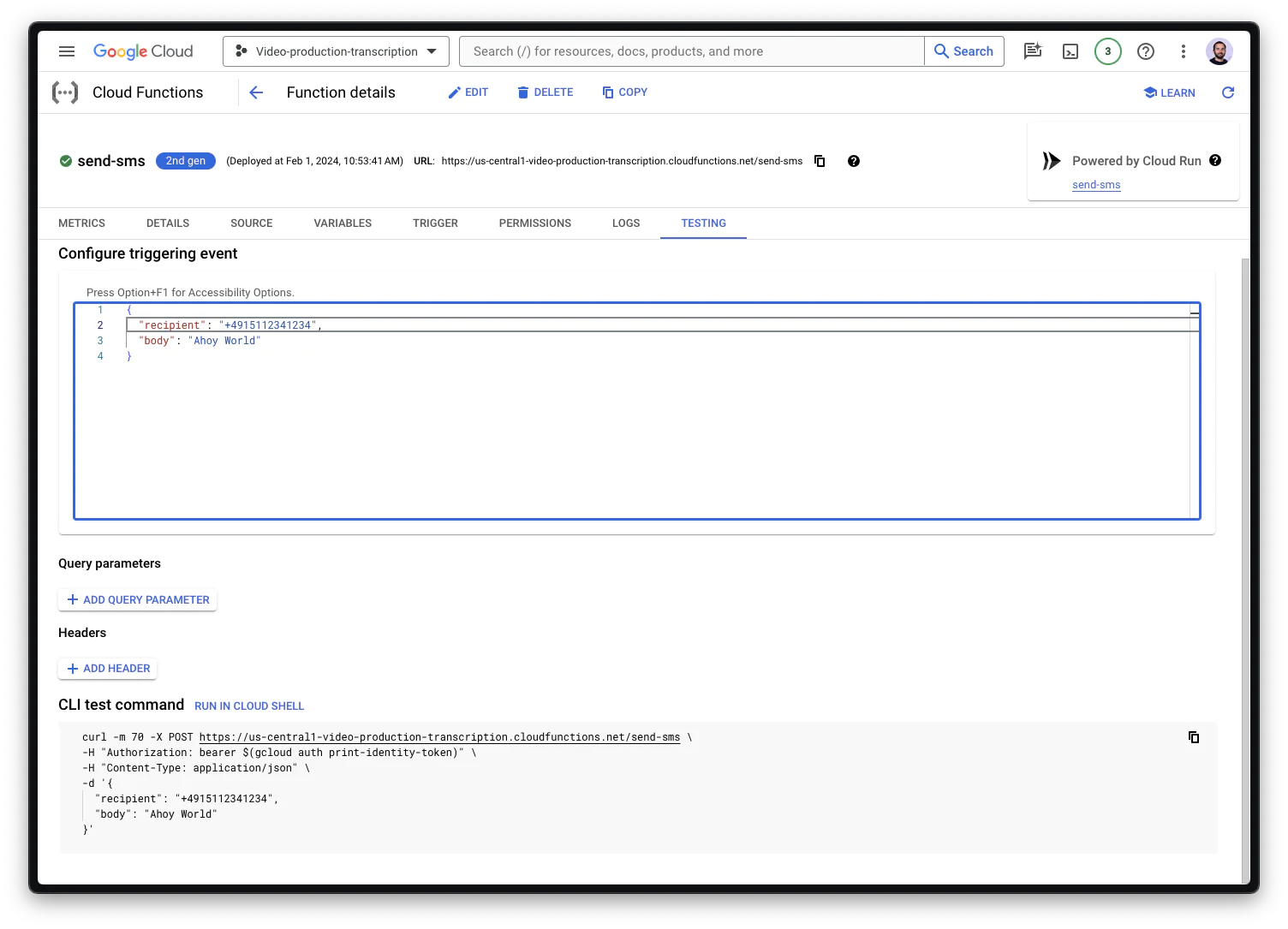
Did you receive your test message?
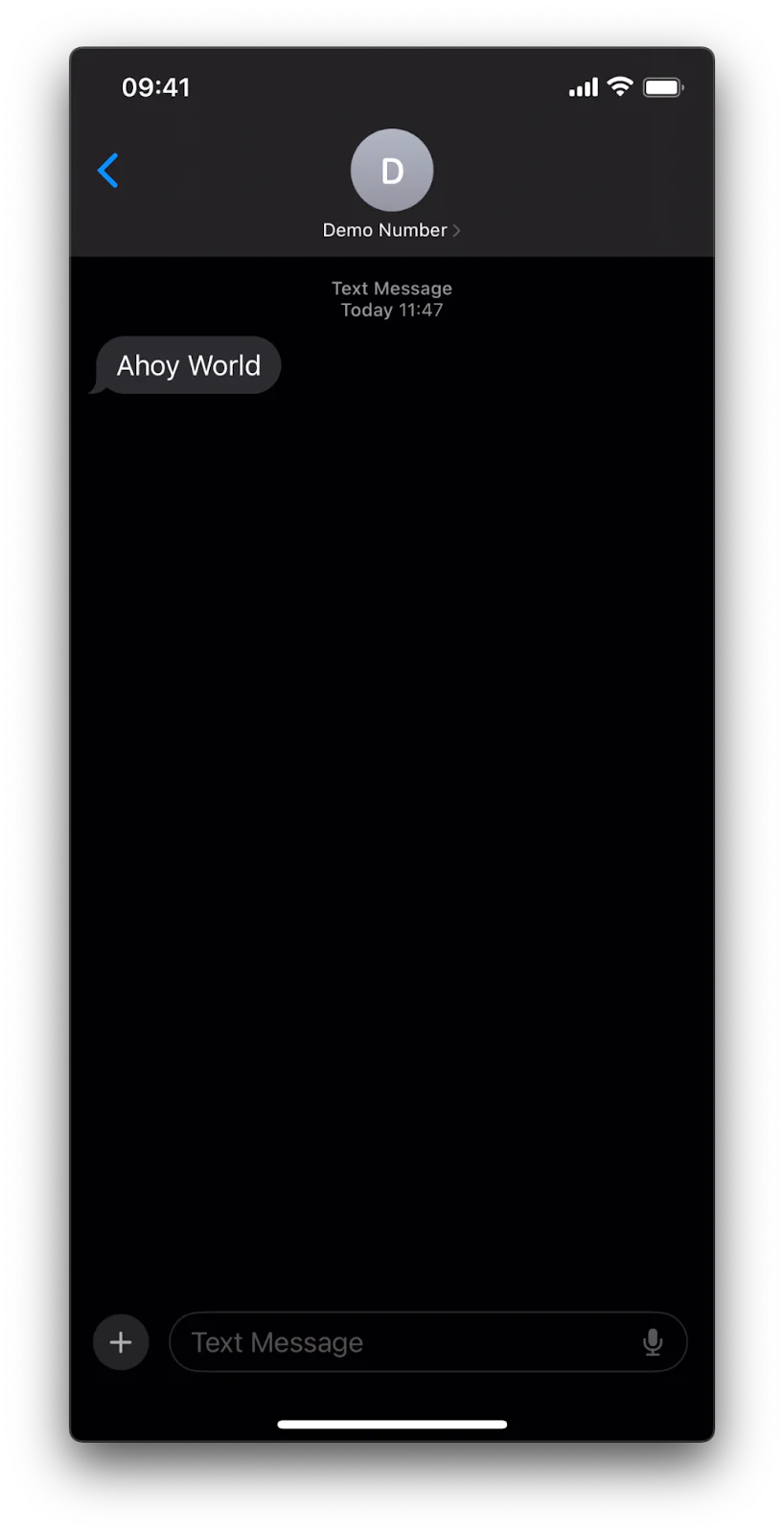
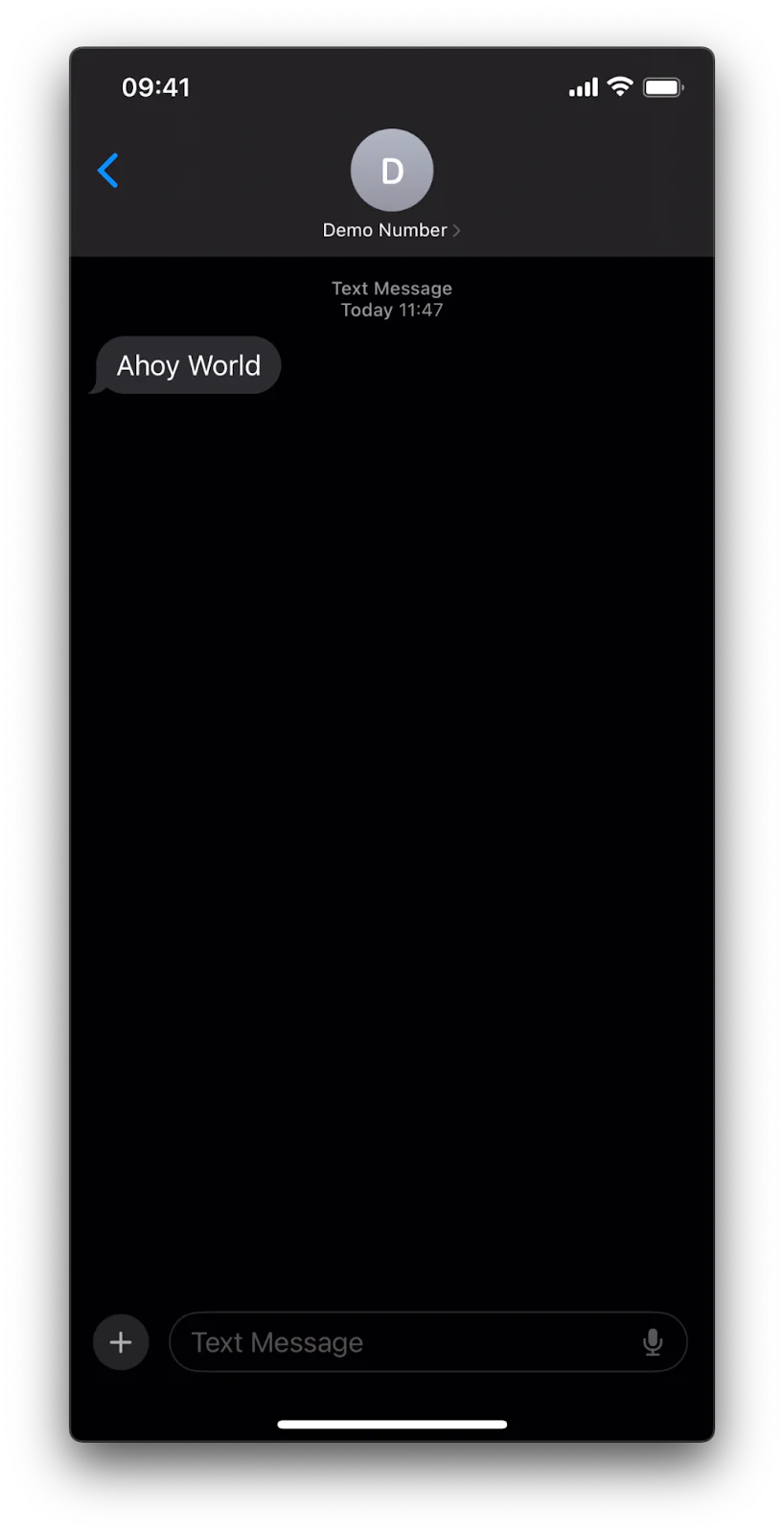
Conclusion
And voilà! You have conquered the art of sending SMS using Node.js and Google Cloud Functions. How cool is that? Not only can you fire off a message to anyone, anytime, but you can do so without breaking a sweat. All this is thanks to the power of Google Cloud and Twilio working hand in hand.
However, this is just the beginning of what's possible with cloud functions and SMS technology. Our next step is to show you how to handle incoming SMS messages, which opens another world of possibilities, such as chatbots that leverage AI.
Never stop exploring, for in the world of coding, every line holds the potential to unlock revolutionary possibilities. Keep experimenting, keep innovating, and stay obsessed with creating better communication with your customers.
I can’t wait to see what you build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.