Tips for Getting Started with Blazor
Time to read:
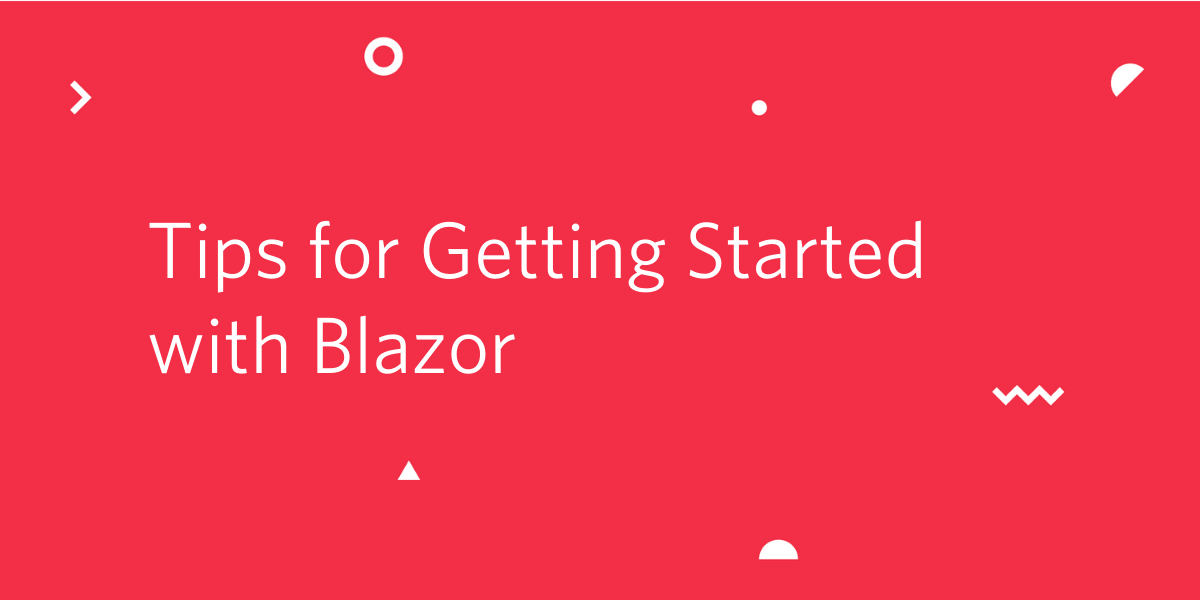
As a .NET developer, I've been excited to explore all of the great things that came with the release of .NET Core 3.0. I have spent time playing with one of the newly launched technologies, Blazor, which allows me to build web applications targeting web assembly using HTML, CSS and C#.
In my excitement, I went to see what the fuss was about. Following the instructions on Blazor.net, I updated Visual Studio 2019, installed the Blazor project templates, created a new Blazor app project, and then realized there were some choices I needed to make in order to build my shiny Blazor web application. As I made some choices and got going with my project I continued to see more and more places where I wished some things had already existed to help make development easier.
As a result, I've put together this list of tips to help sort through making our Blazor applications a bit easier to design, build and test. Enough already let's get to the tips!
Tip 1: Choose Wisely between Blazor WebAssembly & Blazor Server
The first step when getting started with Blazor is to install the tooling and templates. These can be found on blazor.net. If you've installed all of the Blazor templates and then create a new Blazor project, you may find yourself needing to choose between Blazor WebAssembly or Blazor Server.

What's the difference? When should I use one over the other? Why does it matter?
Let's take it from the top. Blazor is a framework used to build interactive web applications with HTML, CSS and C#. It allows developers to build rich web applications with Razor Components that use the Razor syntax and C# code for your application.
It also provides the flexibility to host those components on a server (with Blazor Server) or on a client machine (with Blazor WebAssembly). Check out the table below to see what you get with each:
Blazor WebAssembly | Blazor Server |
|
|
There are a lot of points above but one of the most important factors to consider is while Blazor WebAssembly currently remains in active development, Blazor Server is generally available and supported today.
TIP: Choose your template wisely after considering the differences between the two
Tip 2: Put Your C# Code in a C# Class
Let's presume you have created a new Blazor project using Visual Studio 2019, Visual Studio Code, or the code editor of your choice. The default template gives you a fully functioning demo application that you can run. After typing dotnet run
in the terminal to see the sample application you may want to add new features. Where the tutorial guidance directs you to the Pages folder to create a new component for your new page, this is the moment where you should stop. If you're only using Razor code then this guidance is accurate.
However if you try to wire up page activities using C# code, create a class in a different C# file. You can name it whatever you'd like so long as it derives from ComponentBase. Then in your Razor file, add inherits YOUR_FULLY_QUALIFIED_CLASS_NAME
, replacing with your fully qualified class name to the top of the Razor file. Moving your code into a C# class will make your project more maintainable, a great deal more testable, and help to make your coding experience more modular.
TIP: Put your C# component code in a C# file instead of a Razor file
Tip 3: Don't Be Afraid of TypeScript
Now you're well on the way to building your application and realize that you need to save data to the browser's storage. The quick thing to do is create a scripts folder under wwwroot, create a site.js file and add the Javascript code to do it. Below is a sample of what that code would look like.
Did you know you could use TypeScript and make this code a little easier to manage with type checking, error handling, and great tooling support? It's actually quite easy to do. With some code changes, a filename change, and an additional package, you can begin using TypeScript in your Blazor application.
For example, you can turn the Javascript above into TypeScript by doing the following:
- At the command line type,
dotnet add package Microsoft.TypeScript.MSBuild
- Rename your .js file to a .ts file
- Change your code to accommodate TypeScript by replacing your code with the following
The build process will take care of transpiling your TypeScript into Javascript but now you have the benefits of static typing, easier collaboration and improved productivity.
TIP: Don't be afraid to add some TypeScript in the mix
Tip 4: Find & Fix Errors in Your Blazor Application
There is inevitably the issue where you're running your application and an error occurs. When that happens in a Blazor Server application, it's not unusual to check the browser console and see an error message that says Error: There was an unhandled exception on the current circuit, so this circuit will be terminated. For more details turn on detailed exceptions in 'CircuitOptions.DetailedErrors'.
Don't fret - we can do that with a simple one line change in the Startup.cs file. Head over there to the public void ConfigureServices(IServiceCollection services)
method and change the method to the below:
This change will allow you to see the errors in the browser console. Caution - this change does print your entire stack trace in the console. You should be careful about shipping this sort of change into a production environment.
TIP: Turn on Detailed Errors to find and fix problems quickly
Conclusion
These are just a few tips to help your Blazor application development go well. I took the liberty of putting together a new project template with a few additional folders and packages to make our development a bit easier to manage. Grab it from Nuget by visiting this link or by running dotnet add package Structured.Blazor.Server
in your terminal window. If you have any questions, you can always reach me via email or on Twitter. I can't wait to see what you build. Happy coding 😉
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.