Using Twilio with Node.js by Dusty Candland of Red27 Consulting
Time to read: 4 minutes
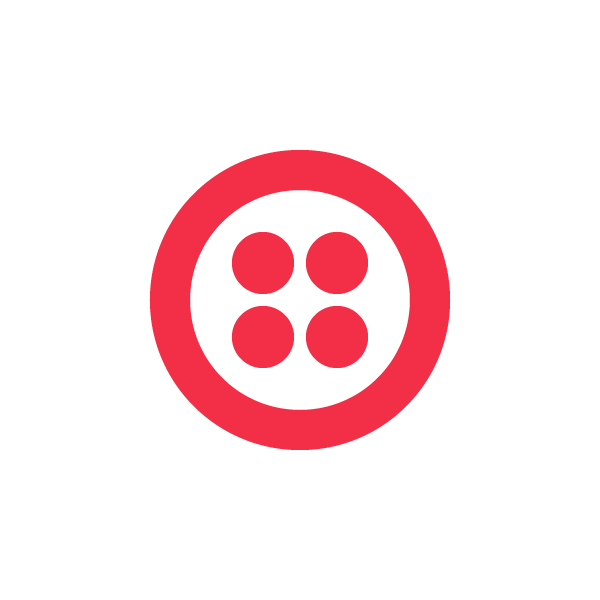

Recently while working on a Functional Mockup for SMSBet.info, we decided to use Node.js and Twilio. Combining these two technologies makes building SMS and telephony apps really easy. Node.js is a super fast, event-based, async server-side JavaScript engine. While the project is relatively young it has a very committed and growing community behind it.
Node.js on its own provides simple evented IO. The first step to building a Twilio app was to find a web framework to help handle the basics of HTTP and View rendering. Express is a popular framework that will work well for our needs. Using Express is enough to be able to receive an SMS message, get it’s content, and respond. That’s what I’m going to demonstrate here.
Setup

You’ll also need a Twilio account for these examples. If you’ve just signed up, you can use the sandbox number on your account or if you’ve upgraded a local number will work as well. First we’ll build the app and deploy it, then later on we’ll configure our SMS URL that gets invoked when a message is sent to our sandbox number.
Lastly we need a place to host this application, I’m going to use Nodester.
The Project
Now lets create our Express project and get the ball rolling.
twiliosample is the name of the Express project we’re creating. The Express command will create and initialize the app in a directory with the same name. If you prefer, you can use any name you want in place of twiliosample.
Inside of our project folder, locate and open app.js in your editor of choice and add the following to the top of the file. This creates a sys object that will allow us to log the incoming SMS data.
Next we’ll create a function to handle our incoming SMS requests. Add the following method under the default index route.
When a POST request comes in to /incoming, this function will be called to handle the request. We first extract the From and Body values from the POST data and log it. Next we return back the TwiML that will send a text message back to the sender with a thank you message.
To test this out you will need a public URL for Twilio to access. To test locally you can simulate the a request using the follow cURL syntax. Start your Node.js server using ‘node app.js’ from the project root. Then from another command prompt issue this curl command.
If everything is working you will see your TwiML returned and your message logged in the Node.js command prompt window.
Going Live
If you have a Nodester account, we can publish our application, set up Twilio to call it, and test it out! Nodester uses Git as for deployment, so we need to set up our project as a Git reposititory.
Nodester provides a CLI toolkit to make it easier to work with your Nodester-hosted apps. You can install the tools using the Node Package Manager:
Once you have the tools installed, follow these instructions to set up your user account. Next we’ll set up an application. Because app names need to be globally unique, you’ll need to use a name other than twiliosample. We’ll use the appnpm command to remotely install the packages we’re using for this project.
The last command will output a Git repository URL. Use that URL in the following command to connect your local Git repository with the remote Nodester repository.
Update the port your app is listening on, default is 3000, in app.js to the port listed by the info command. Commit your changes to git and push to Nodester and start your application.
Browse to your app and see if it’s all working http://[nodesterappname].nodester.com.
The last step is to connect your public Nodester app URL with your phone number. Sign in to Twilio and update the SMS URL for your phone number (or the sandbox number) to http://[nodesterapp].nodester.com/incoming. Send a text to you number and wait for the response. If you’re using the sandbox number remember to prefix your PIN to your text.
You can check the logs on the server to make sure your message was logged with the following command:
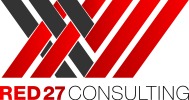
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.