How to Connect Twilio Programmable Voice With Microsoft Teams
Time to read: 5 minutes
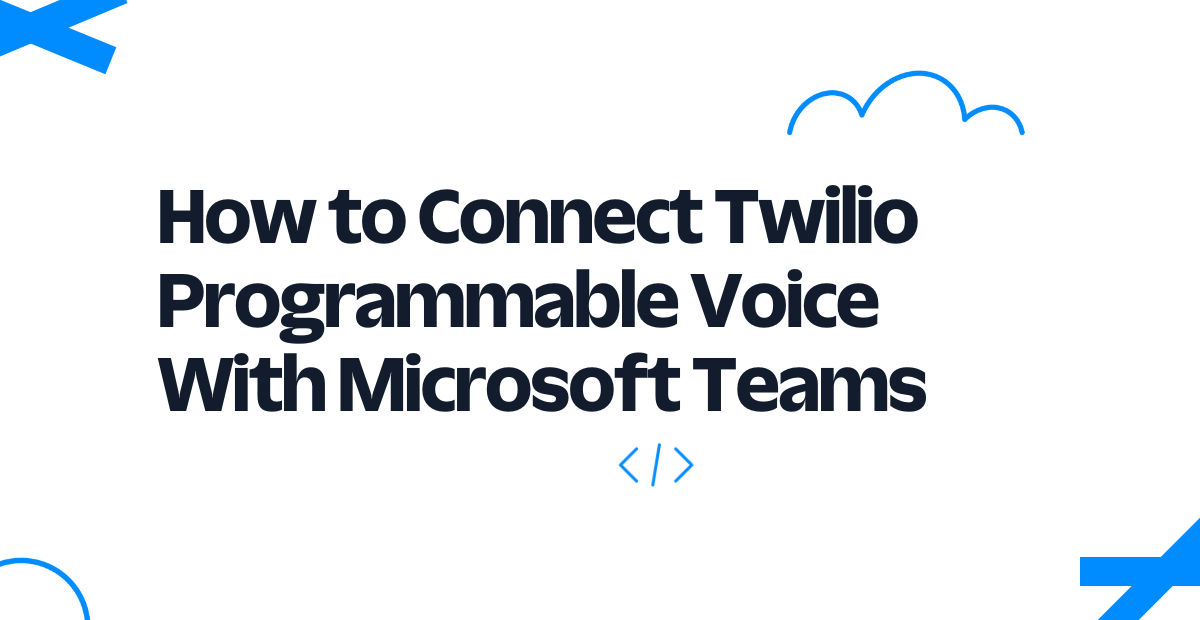
Many organizations rely on Microsoft Teams as an all-in-one tool for team communication. In addition to chat rooms, Microsoft Teams also provides video conferencing and file sharing, helping businesses work better together.
Twilio has also become an essential part of business communications, but from a different angle. Among the many communication channels we support, Twilio can provide users with an SMS number that external users can text through our Programmable SMS product. This unlocks new interactions between businesses and customers, enabling new sales and support workflows.
Rather than relying on Microsoft Teams and Twilio separately, it can be beneficial to integrate both platforms into one message stream. This allows teams to log onto one platform and receive communication from multiple sources.
In this post, we’ll show you how to connect to Microsoft Teams from within Twilio. For our project, we’ll set up a server to wait for a webhook notification from Twilio when our SMS number receives a text message. Once that notification comes in, we’ll forward that message to a Microsoft Teams channel.
Benefits of integrating Twilio and Microsoft Teams
When your organization depends on separate services—such as Twilio and Microsoft Teams—to communicate, integrating these services can bring powerful features with significant benefits. This is particularly the case when integrating Twilio Programmable SMS with Microsoft Teams.
For example, when users perform specific actions within Microsoft Teams—such as uploading a file or adding a new member to a channel—this can trigger an action on the Twilio platform, like sending an SMS message or initiating a video call. The same is true if there’s a message sent to your Twilio SMS number. It can trigger an action within a Microsoft Teams channel.
Additionally, integrating Twilio with Microsoft Teams lets you streamline communication to reach your users through various channels. Building these integrations also allows you to leverage automation, removing the manual steps between one event and triggering an action.
Finally, by integrating Twilio with Microsoft Teams, you expand your toolset for increased functionality for your users. Combining Microsoft Teams’ collaboration tools and Twilio’s multichannel communication tools opens the door for countless new features and possibilities.
Types of integrations
There are different ways to integrate Twilio with Microsoft Teams, but the most common usage is connecting messaging in a Microsoft Teams channel with Twilio SMS messaging or voice calling.
For example, a chat message in a Microsoft Teams channel that meets a certain condition—such as a keyword or post by a specific user—can trigger an SMS message through Twilio. Or, sending an SMS message to a Twilio number can trigger a chat message in a Microsoft Teams channel.
More advanced types of integrations could leverage other APIs from Twilio, such as the Phone Numbers API, Video API, or Voice API. For example, you could build chatbots that react to certain actions within a Microsoft Teams channel using Twilio APIs. You can also build integrations that leverage APIs from Twilio SendGrid. For example, an action in a Microsoft Teams channel can trigger email campaigns within SendGrid.
How can I integrate Twilio and Microsoft Teams?
Integrating Twilio with Microsoft Teams is straightforward, and you can adopt your web programming language of choice. Ultimately, however, an integration will require building a handler for a webhook call.
Prerequisites
Before you begin the tutorial, you should be acquainted with the following tools and concepts:
- First, our demo project will use Ruby 3.0, so basic familiarity with that programming language will be helpful. However, the concepts we introduce will apply to any language or development environment.
- Next, we’ll use webhooks to listen for events and create responses to them. Refer to the documentation for Twilio and Microsoft Teams to understand how to configure webhooks for each platform.
- Finally, be sure to install ngrok, a tool that exposes your localhost to the public internet, making testing easier.
Step 1: Set up a server in Ruby
Create a new project folder called twilio_w_ms_teams
. Within that folder, create a file called Gemfile
and paste these lines into it:
We’ll use Sinatra to build our web server. To test it out, create a file called server.rb
with the following contents:
Next, in our terminal, run the bundle install
command to install our packages. Finally, boot up the server:
In another terminal window, run this command:
This exposes port 4567 (Sinatra’s default) to the world. You’ll see some diagnostic information emitted, but the most important piece of information is the resulting public URL. It looks like <alphanumeric_characters>.ngrok.io
. However, in our case, our public URL is https://72bd60d9b4f7.ngrok.io
.
Finally, in yet another terminal window, issue a curl
command to your server’s public URL to ensure that it can respond to HTTP requests:
If your server responds to a GET /hello
request with the string World
, then your setup is complete.
Step 2: Listen to Twilio events
Back in the Gemfile
, add the following line to the bottom:
This installs the Twilio Ruby SDK, which makes interacting with the Twilio API much simpler. Again, run bundle install
to install this package.
Next, we’ll need to define the URL where Twilio will send webhooks. Follow these instructions for setting up a webhook in Twilio. In our case, our webhook URL will be the ngrok public URL, with a path of /sms
. So, it’ll be https://72bd60d9b4f7.ngrok.io/sms
.
Finally, we’ll need to define this new /sms
route in our Sinatra app. To do so, we’ll modify our server.rb
file to look like this:
Twilio has documentation outlining all of the parameters sent when issuing a request to your webhook server. However, for our use case, we’ll only concern ourselves with the sender (From
) and the message contents (Body
).
If you’d like, you can send a test text message to your Twilio number right now. Receiving that message will trigger the webhook, which will call your /sms
route. Your Sinatra server should respond with a 200 OK
, containing a body string of Got it
.
Step 3: Send messages to Microsoft Teams
Now that we’ve connected with Twilio, we need to connect with Microsoft Teams. To do that, we need an SDK that’ll help us simplify communication with the Microsoft Teams API. In the Gemfile
, add this line:
Run bundle install
to install this package.
Our goal now is to take the SMS that Twilio handed to our server and send it over to Microsoft Teams. To do that, first, at the top of our server.rb
file, we’ll need to require
the gem we just installed:
We’ll also need to configure a webhook for the Microsoft Teams channel where we want to send this message.
Next, change the /sms
route in server.rb
to look like the following:
Voilà! Take the SMS from Twilio as before, but this time, you’ll also construct a new MicrosoftTeamsIncomingWebhookRuby::Message
object. Calling #send
delivers it to the webhook URL provided by Microsoft Teams. However, keep in mind the URL that Microsoft Teams uses is not your server’s hostname but rather the URL of the Microsoft Teams channel.
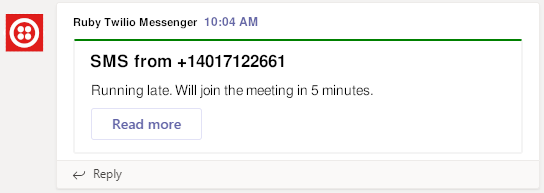
Discover more Twilio integration resources
We’ve only begun to scratch the surface of developing integrations between Twilio and other platforms. Fortunately, you can tap into Twilio’s extensive developer documentation to learn how to integrate with WhatsApp, issue voice calls, and even send emails—all through our API.
Plus, if you’re interested in call center integrations, learn how to integrate Twilio Flex with any customer relationship management, Microsoft Dynamics 365, Telegram, and more. We can’t wait to see what you build!
Alvin Lee is a full-stack developer based in Phoenix, Arizona. He specializes in web development, integrations, technology consulting, and prototype building for startups and small-to-medium businesses.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.