Call Tracking with Python and Django
Time to read:
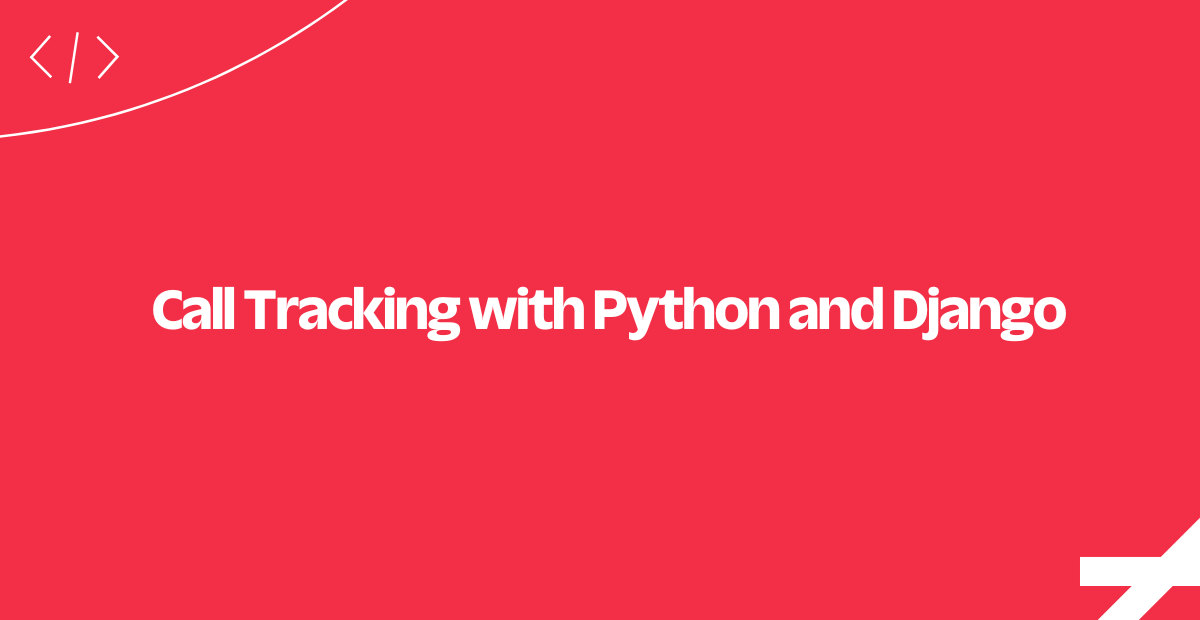
This Django web application shows how you can use Twilio to track the effectiveness of different marketing channels.
This application has three main features:
- It purchases phone numbers from Twilio to use in different marketing campaigns (like a billboard or a bus advertisement)
- It forwards incoming calls for those phone numbers to a salesperson
- It displays charts showing data about the phone numbers and the calls they receive
In this tutorial, we'll point out the key bits of code that make this application work. Check out the project README on GitHub to see how to run the code yourself.
Search for available phone numbers
Call tracking requires us to search for and buy phone numbers on demand, associating a specific phone number with a lead source. This utility module uses the Twilio Python helper library to search for phone numbers by area code and return a list of numbers that are available for purchase.
Now let's see how we will display these numbers for the user to purchase them and enable their campaigns.
Display available phone numbers
We display a form to the user on the app's home page which allows them to search for a new phone number by area code. At the view level, we use the utility module we created earlier to actually search for numbers, and display an HTML page to the user with a list of numbers they can choose to buy.
We've seen how we can display available phone numbers for purchase with the help of the Twilio Python helper library. Now let's look at how we can buy an available phone number.
Buy a phone number
Our purchase_phone_number
utility function takes a phone number as its sole parameter and uses our Twilio API client to actually purchase one of the available phone numbers we searched for earlier.
If you don't know where you can get this application SID
, don't panic, the next step will show you how.
Set webhook URLs in a TwiML Application
When we purchase a phone number, we specify a voice application SID. This is an identifier for a TwiML application, which you can create through the REST API or your Twilio Console.
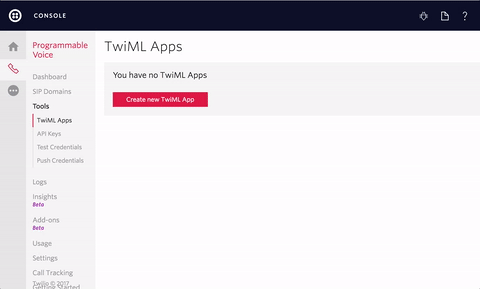
Associate a phone number with a lead source
Once we search for and buy a Twilio number, we need to associate it with a lead source in our database. This is the core of a call tracking application. Any phone calls to our new Twilio number will be attributed to this source.
So far our method for creating a Lead Source and associating a Twilio phone number with it is pretty straightforward. Now let's have a closer look at our Lead Source model which will store this information.
The LeadSource model
The LeadSource
model associates a Twilio number to a named lead source (like "Wall Street Journal Ad" or "Dancing guy with sign"). It also tracks a phone number to which we'd like all the calls redirected, like your sales or support help line.
As the application will be collecting leads and associating them to each LeadSource or campaign, it is necessary to have a Lead model as well to keep track of each Lead
as it comes in and associate it to the LeadSource
.
Define the Lead model
A Lead
represents a phone call generated by a LeadSource
. Each time somebody calls a phone number associated with a LeadSource
, we'll use the Lead
model to record some of the data Twilio gives us about their call.
The backend part of the code which creates a LeadSource
as well as a Twilio Number is complete. The next part of the application will be the webhooks that will handle incoming calls and forward them to the appropriate sales team member. Let's us see the way these webhooks are built.
Forward calls and create leads
Whenever a customer calls one of our Twilio numbers, Twilio will send a POST request to the URL associated with this view function (should be /call-tracking/forward-call
).
We use the incoming call data to create a new Lead
for a LeadSource
, then return TwiML that connects our caller with the forwarding_number
of our LeadSource
.
Once we have forwarded calls and created leads, we will have a lot of incoming calls that will create leads, and that will be data for us but we need to transform that data into information in order to get benefits from it. So, let's see how we get statistics from these sources on the next step.
Get statistics about our lead sources
One useful statistic we can get from our data is how many calls each LeadSource
has received. We use the Django ORM's annotate feature to make a list containing each LeadSource
and a count of its Lead
models. We create a custom model manager so that we can easily access this data in our views.
Up until this point, we have been focusing on the backend code to our application. Which is ready to start handling incoming calls or leads. Next, let's turn our attention to the client side. Which, in this case, is a simple Javascript application, along with Chart.js which will render these stats in an appropriate way.
Visualize our statistics with Chart.js
Back on the home page, we fetch call tracking statistics in JSON from the server using jQuery. We display the stats in colorful pie charts we create with Chart.js.
That's it! Your Python and Django application is now ready to purchase new phone numbers, forward incoming calls, and record some statistics for our business.
Where to next?
That's it! Our Django application is now ready to purchase new phone numbers, forward incoming calls, and record some statistics for our business.
If you're a Python developer working with Twilio, you might also enjoy these tutorials:
Put a button on your web page that connects visitors to live support or sales people via telephone.
Appointment Reminders (Django)
Send your customers automatic reminders ahead of appoiments using Twilio SMS.
Did this help?
Thanks for checking this tutorial out! If you have any feedback to share with us please contact us on Twitter, we'd love to hear it.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.