How to build a picture guessing game with Twilio
Time to read: 7 minutes
How to build a picture guessing game with Twilio
Twilio is known among developers for having the go-to APIs for delivery notifications, promotional offers, emergency alerts, and account verification – but did you know that Twilio is also used for good old-fashioned fun?
In this blog post, we developers will channel our creativity and artistic flair to build a picture guessing game.
Feel free to build along, or fork the project on GitHub to add your own unique flair. You can play the game by texting “GO” to +1 (844) 905-5079.
Background
To start the game, text “GO” to +1 (844) 905-5079. You’ll receive an animated GIF of a random drawing and have 3 attempts to guess what it is. Some of the images are easier to guess than others, and that’s because they come from real-world drawings through Quick, Draw!
Quick, Draw! is an online guessing game by Google that challenges real players to doodle objects; the goal being to have a neural network AI recognize the drawings. With over 15 million players contributing more than 50 million drawings, it’s the world’s largest doodling data set . This large volume of drawings gives our players a great deal of variety in their playthroughs.
To interact with the data set, we’re using quickdraw , a Python API for accessing the Quick, Draw! data.
Now on to the tutorial!
Step 0: Tools to install
- A supported Python version installed on your computer
- ngrok installed on your machine. ngrok is a useful tool for connecting your local server to a public URL. You can sign up for a free account and learn how to install ngrok
- Access to a phone that can make and receive MMS messages
Step 1: Create a free Twilio account
In order to build this yourself or use other Twilio products , you’ll need a Twilio account. If you haven’t already, sign up for a free Twilio account . The signup process is quick and no credit card is required!
Step 2: Buy a Twilio phone number
If you haven’t done so already, buy a Twilio phone number – a phone number purchased through Twilio – to send messages.
After signing up for an account, log in to the Twilio Console . Then, navigate to the Phone Numbers page. Click Buy a Number to purchase a Twilio number.
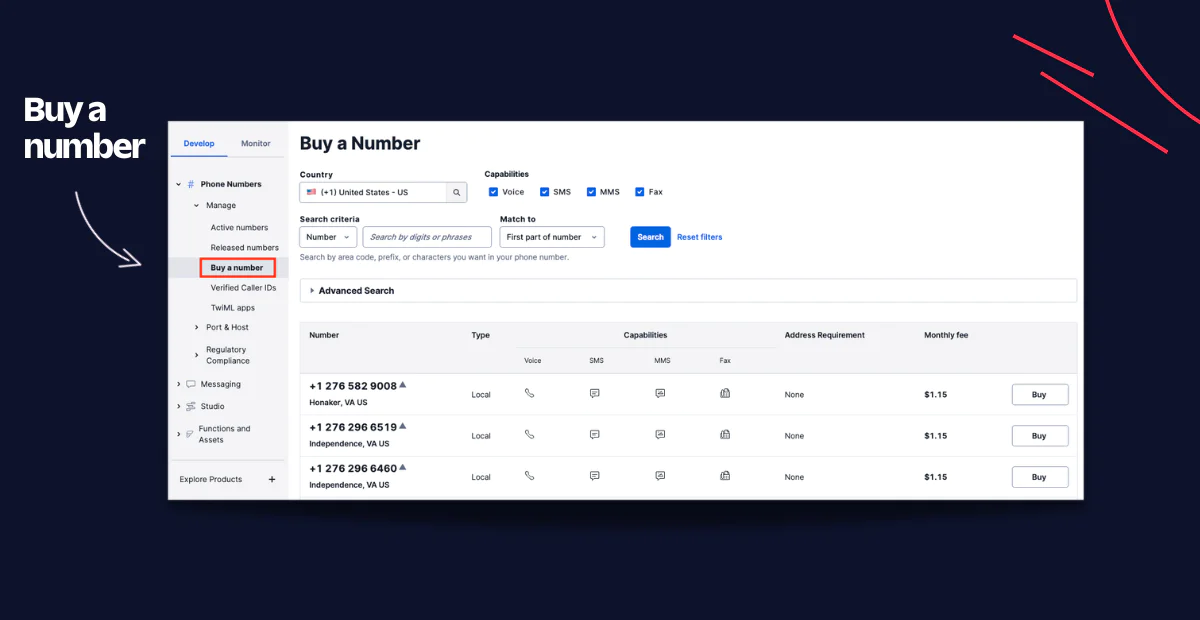
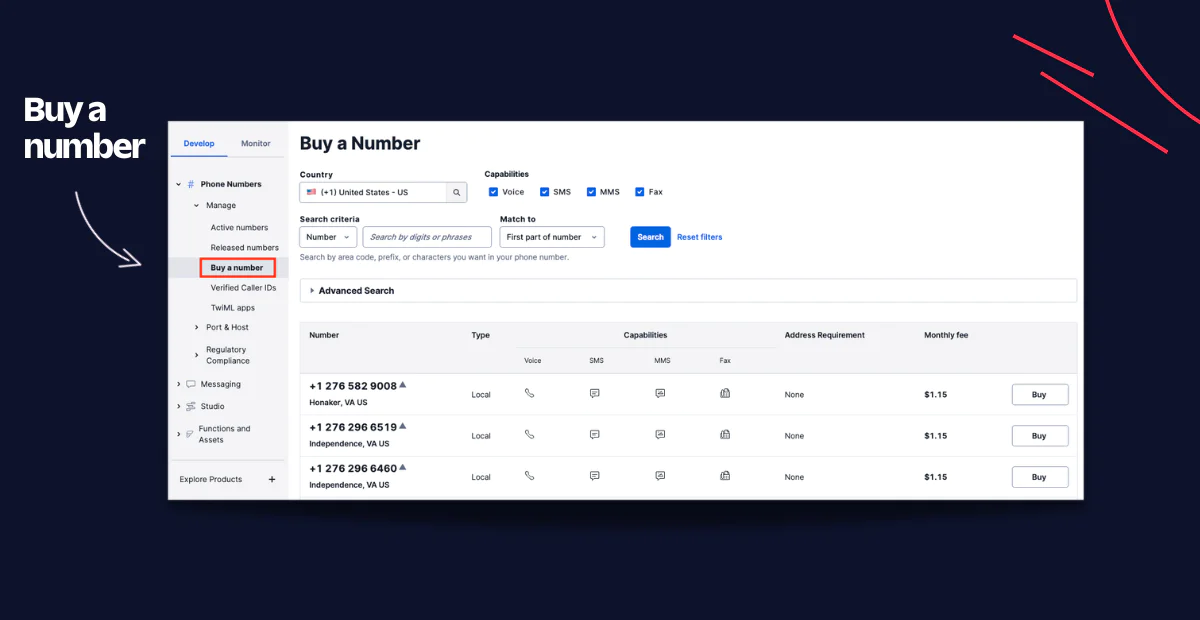
Step 3: Setup local development environment
After creating a Twilio account and obtaining a phone number, this step sets up a project to accommodate your code.
Open a Terminal window and create an empty project directory called twilio-picture-game :
Then change into that directory, as that’s where your code will be.
Since the code for this tutorial will be in Python, create a virtual environment :
Activate your virtual environment:
Then, using the pip package manager, install the required dependencies in your virtual environment:
Step 4: Configure environment variables
With your local development environment set up, it’s time to configure some environment variables so your credentials are hidden. As a best practice when working with sensitive information like API keys and passwords, it’s important to ensure they are secure and not exposed to the public.
Create a file called .env in the project’s root directory (/twilio-picture-game ) to store your API keys.
Within the .env file, create the following environment variables:
Next, paste your credentials within the .env file.
The Account SID , Auth Token , and Twilio Phone Number can be found on the Homepage of your Twilio Console under Account Info.
Your .env file should now look similar to this:
Step 5: Create a Service, Environment, and Asset to host pictures
This application will eventually retrieve a picture for users to guess. However, before fetching the image, create a serverless environment to host them.
To quickly build and deploy these images without having to spin up or maintain infrastructure, Serverless is a possible solution. Twilio Serverless API allows you to deploy Assets and Environments programmatically. Assets will be used to host pictures in a development Environment, and a Service is a container for your Assets and Environments.
Create a new file called serverless.py :
Within serverless.py , paste the following:
Executing the command python3 serverless.py will create a Service, Environment, and Asset, and append their corresponding SIDs to additional environment variables in the .env file.
Your .env file should now look similar to this:
Step 6: Quick, Draw! picture
In this step, you’ll interact with the quickdraw Python API to get a random animated drawing.
Within your project directory, create a new file called quick_draw.py and paste the following code into it:
Here’s a breakdown of the code in quick_draw.py :
- Lines 1-3, are module imports to make use of their functionality.
- Line 3, uses the quickdraw module and will be used to interact with the quickdraw API.
- Lines 5-22, create a categories list. Each item represents a category of drawings available in Quick, Draw! Select your own across the 345 available categories .
- Lines 24-31, define a quick_draw function that pulls an image from the data set and saves it.
- Line 27, fetches a random drawing from a random category.
- Line 28, saves the drawing as a GIF file with the filename quickdraw.gif
Executing the command python3 -c "import quick_draw; quick_draw.quick_draw()" will run the function and save a random image, as seen below.
Step 7: Deploy your picture using Serverless API
Next, deploy and host your GIF using the Twilio Serverless API . Create a new file called deploy.py by executing touch deploy.py. Then add the following code:
Here’s a breakdown of the code in deploy .py :
- Lines 1-8, are module imports to make use of their functionality.
- Lines 12-16, define variables taken from the .env file.
- Lines 22-25, define an orchestrator function that invokes functions to create an asset, build, and deployment.
- Lines 27-47, creates an Asset Version by uploading the animated GIF to Serverless.
- Lines 49-56, creates a Build of the asset.
- Lines 58-65, fetches the Build Status .
- Lines 67 & 68, checks if the Build Status is completed.
- Lines 70-84, uses polling to check the Build Status, once completed, it creates a Deployment .
Running the command python3 -c "from deploy import host_asset; host_asset()" will deploy the previously stored image to Twilio Serverless Assets.
Step 8: Send messages with Twilio
Programmable Messaging is Twilio’s API for sending messages using channels like SMS and MMS. Create a new file called app.py and define a function to send text messages using the Programmable Messaging API. Here’s the code for reference:
Here’s a breakdown of app.py :
- Lines 9-10, retrieve the Account SID and Authentication Token from your Twilio account.
- Line 12, creates a client object.
- Lines 14-25, define a function to send an outbound text message using the Programmable Messaging API.
Test the SMS functionality by executing the command python3 -c "from app import send_outbound_text; send_outbound_text('YOUR PHONE NUMBER HERE', 'test message')".
Be sure to replace the function parameters with the recipient's phone number.
Step 9: Game logic
Now that you have successfully procured a random animated drawing and uploaded it to Twilio Serverless, you can create a new file for the game logic. The game will activate on receipt from an incoming message. If the user is not playing, a new game will start (triggered by the keyword “GO”). A random drawing will be fetched and deployed. If the user incorrectly responds with the wrong drawing name, their remaining lives will decrease by one until they either make a correct guess or exhaust their 3 lives.
Modify app.py by inserting the following code:
Here’s a breakdown of the modified code in app.py :
- Lines 1-3, import the previously created functions from quick_draw.py and deploy.py .
- Lines 10-18, set up the web application using Flask.
- Lines 20-23, define a function to initialize the game, resetting the game state.
- Lines 25-34, define a function to start the game, initializing the game and starting a thread to deploy a drawing.
- Lines 36-40, define a function to send a text message with instructions to the game as well as a MMS of the drawing.
- Lines 47-53, define a function to send messages with a countdown, like a loading screen since deploying an image can take tens of seconds.
- Lines 55-65, define a function to handle a player’s incorrect guess, reducing their lives by one.
- Lines 67-69, define a function to handle a player’s correct guess.
- Lines 71-88, define a Flask route, called /game, to handle incoming POST requests and act as the orchestrator of the game.
- Line 90, runs the Flask web application on port 3000.
Execute the command python3 app.py to run the app server.
Step 10: Configure a webhook for responses to an incoming message
With the code for the application complete, you’ll need to configure your Twilio phone number to send requests to a webhook for handling responses to incoming messages. This configuration enables the application to receive and reply to incoming messages, completing the interactivity for the game.
At this point, running python3 app.py will start your local server on http://localhost:3000 . As of now, your application is only running on a server within your computer. But you need a public-facing URL (not http://localhost ). You could deploy your application to a remote host, but a quick way to temporarily make your web application available on the Internet is by using a tool called ngrok .
In another terminal window run the following command:
This will create a “tunnel” from the public Internet to port 3000 on your local machine, where the Flask app is listening for requests. You should see output similar to this:
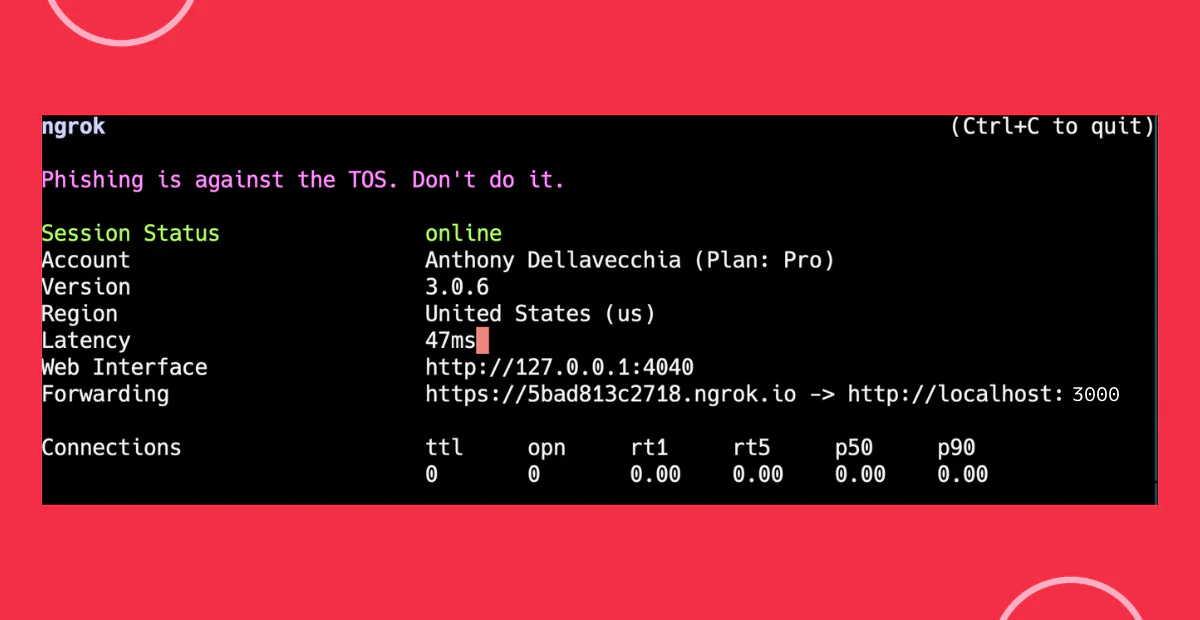
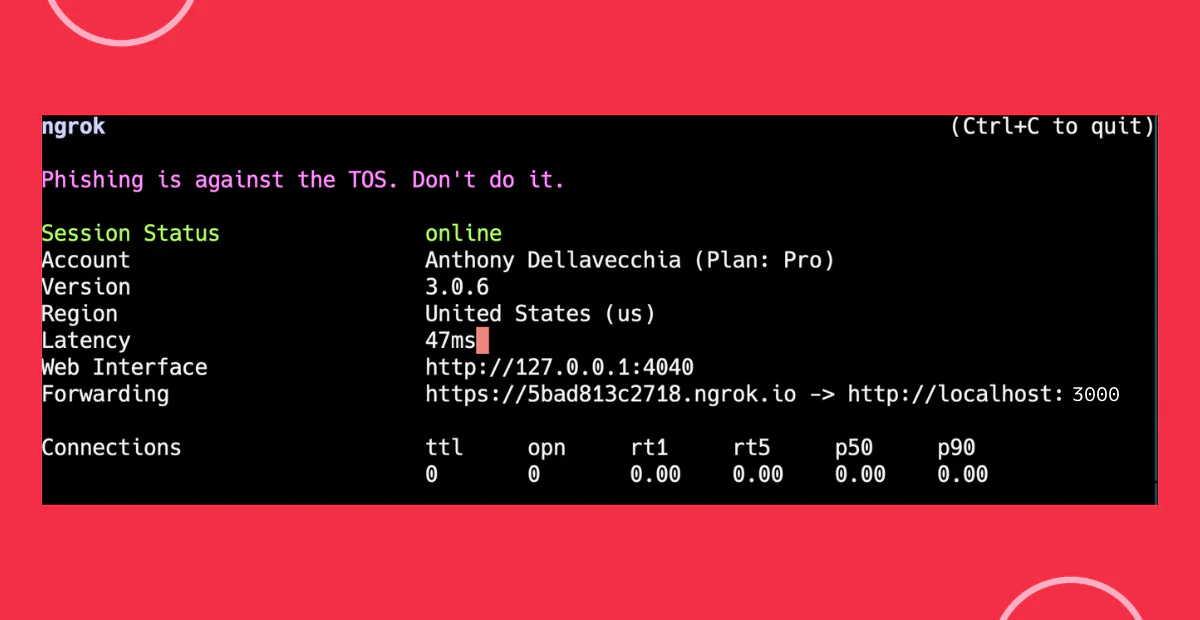
Take note of the line that says “Forwarding”. In the image above, it reads: https://5bad813c2718.ngrok.io -> http://localhost:3000.
This means that your local application is accessible, publicly, on https://5bad813c2718.ngrok.io and your Flask route is accessible on https://5bad813c2718.ngrok.io/game.
In the Twilio Console, do the following:
- Go to the Console’s Number page .
- Click on the Twilio phone number you purchased earlier (or want to modify).
- Scroll down to the Messaging Configuration section.
- Under A Message Comes In , select Webhook .
- Paste in the URL of the application https://5bad813c2718.ngrok.io/game.
- Click Save Configuration .
After completing the configuration and ensuring your web server and ngrok are running, you can give the game a try.
Since it’s triggered by an incoming message, send a text message to your Twilio number to trigger the game. In my case, text “GO” to +1 (844) 905-5079.
Next steps
If you’d like to learn more about this game along with an introduction to Programmable Messaging, I presented it during SIGNAL 2023. You can watch the recording here.
Thanks so much for reading! If you found this tutorial helpful, have any questions, or want to show me what you’ve built, let me know online. And if you want to learn more about me, check out my intro blog post .
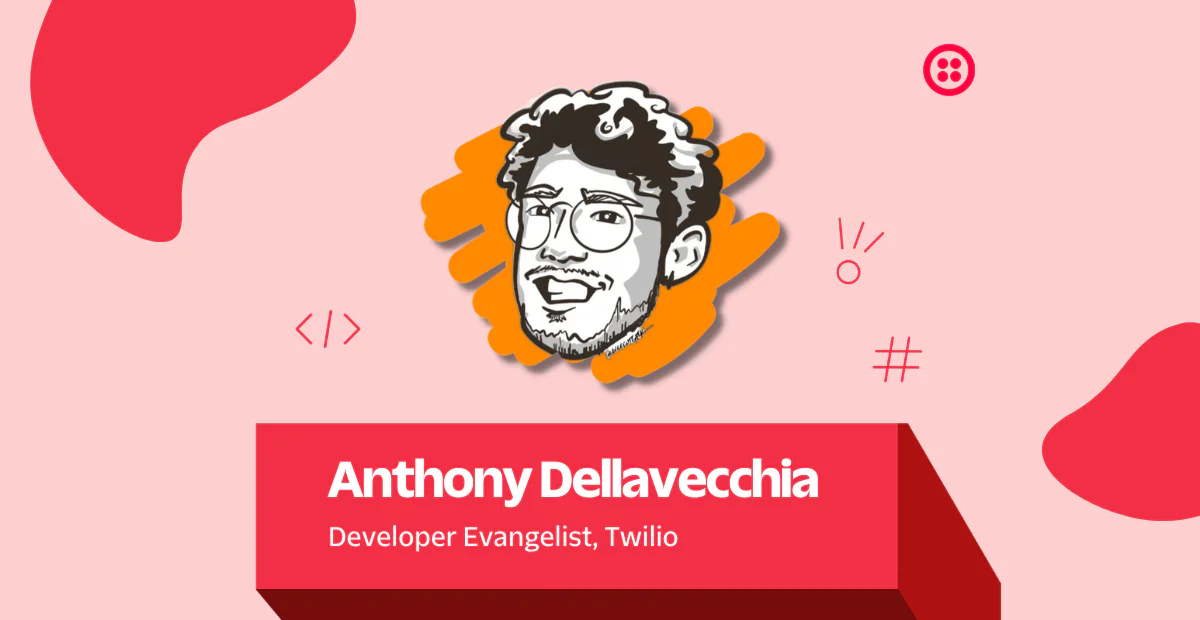
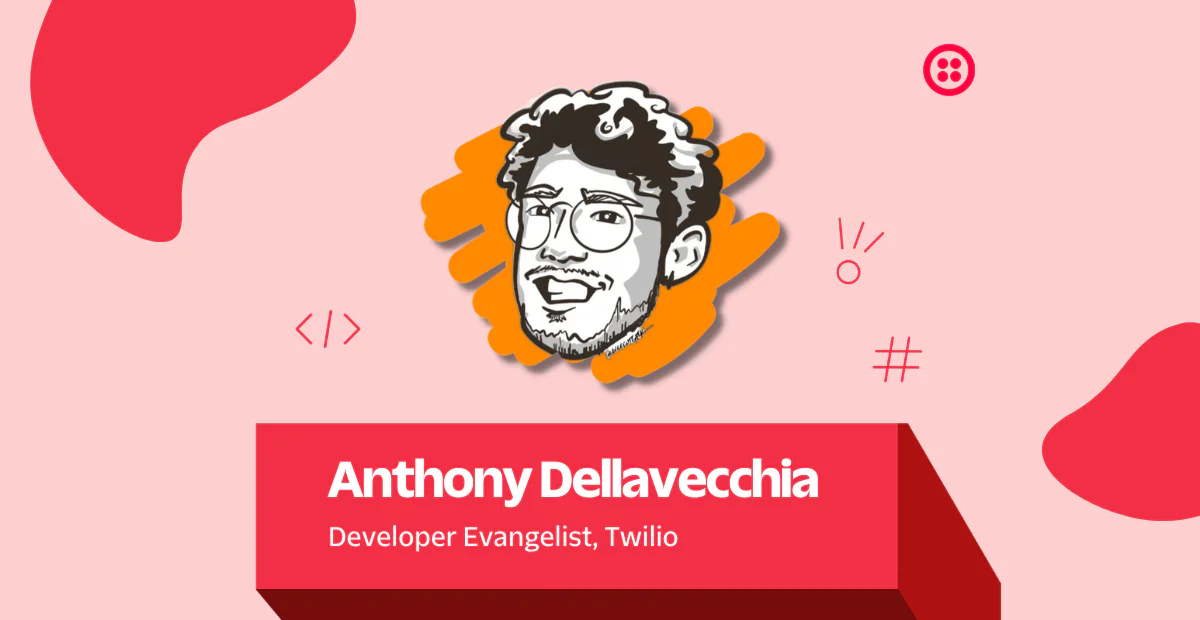
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.