Phone Verification with Twilio For Python Developers
Time to read: 3 minutes
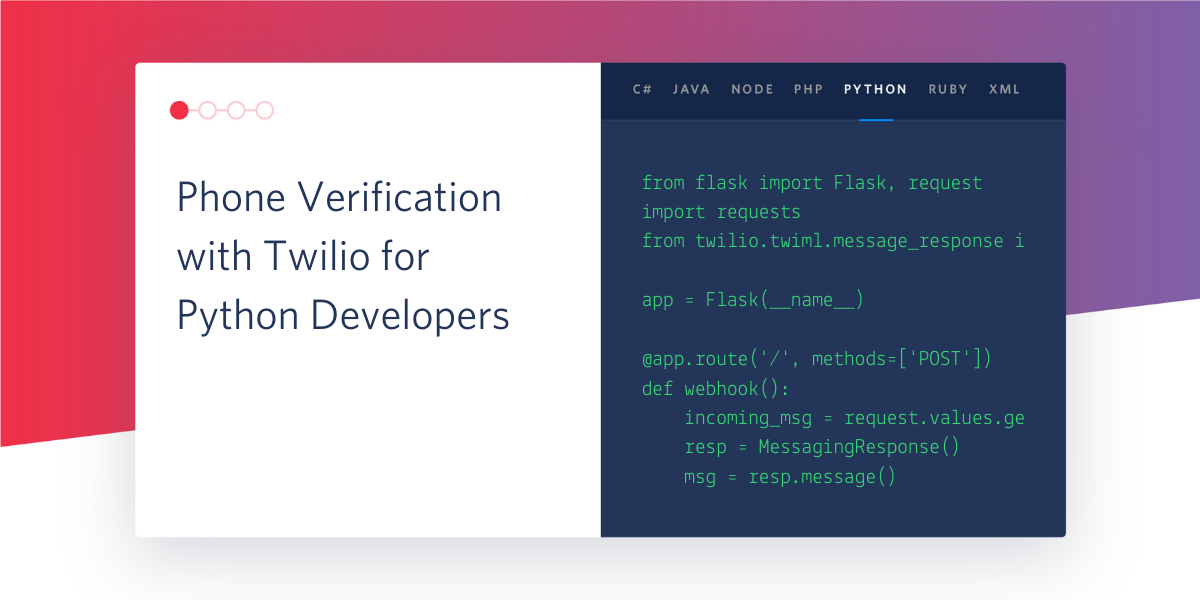
Twilio Verify is a service that allows you to add phone verification to your applications. If you’re curious about the benefits of verifying your users, consider the following two use cases:
- Reduce fake accounts - If you ask users to provide a valid phone number while they create an account, they will be less likely to create phony or duplicate accounts.
- Two-factor authentication - Sending a verification code to your users when they sign-in can help protect their accounts if their credentials are compromised.
In this tutorial, we’ll learn how to work with the Twilio Verify API in Python. Since this is such a simple API, we will be doing all the work from a Python shell!
Tutorial Requirements
To follow this tutorial you need the following items:
- Python 3.5 or newer. If your operating system does not provide a Python 3.5+ interpreter, you can go to python.org to download an installer.
- A phone line. Mobile and landlines are both okay.
- A Twilio account. If you are new to Twilio you can create a trial account and start developing for free. Please review the features and limitations of a free Twilio account.
Service Configuration
The first part of this tutorial is dedicated to configuring the Verify service in your Twilio account.
Log in to your Twilio Console, select the “All Products & Services” button on the left sidebar, and then click on Verify. You will now be staring at an empty list of “services”. In the context of Twilio Verify, a service is an entity that represents an application that requires verification. Click the “Create Service Now” button, and then enter a friendly name for it.
I’m going to use the name Microblog, which is one of my open source projects. The name that you choose for your service will appear in the SMS messages that are sent to users, and will also be spoken through a text-to-speech engine in voice calls.
The Verify service that you create will be assigned a “Service SID”, shown in the settings page for the service:
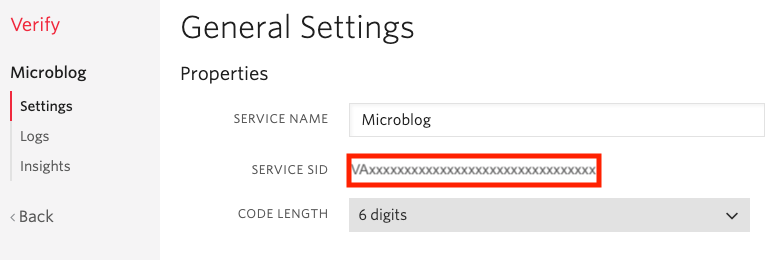
In addition to the Service SID, to work with Verify you also need your Twilio account SID and Auth Token, which you can get from the Twilio Console page:
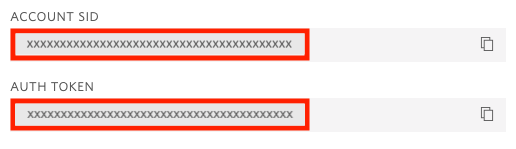
Creating a Python Environment
Now we are ready to see how to run a verification. We’ll begin by making a new directory on your computer called *twilio-verify-tests* or similar, then creating a new Python virtual environment in it.
For Mac and Unix users, the commands are:
For Windows users, the commands are:
Next, install the Twilio Helper library for Python in your virtual environment:
Make sure the version of the Twilio Helper Library is 6.17.0 or newer, as older releases do not support the Twilio Verify API.
Sending a Token to a User
You can send yourself a verification SMS directly from the Python shell by entering the following four statements:
When you run the above code, make sure you insert your Account SID, Auth Token and Service SID in the right places.
Your phone number must be entered in E.164 format. For example, a number from the US must be entered as ’+12345678900’
, where +1
is the country code, 234
is the area code, and 567-8900
is the local number.
If the number that you entered is a mobile number, you will receive a text message on your phone:
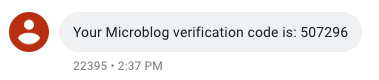
Note how the message includes the friendly name that you gave to the service in the Twilio Verify console.
If the number that you entered is a landline, Twilio will give you an error. That’s because by passing channel=’sms’
you are asking for a verification SMS to be sent to a phone line that cannot receive text messages. You can use channel='call'
to request a voice call where the code is read aloud to you. Verification voice calls work for landlines as well as for mobile phones.
Verifying a Token
The second part of the process is when the user goes to your application and enters the code that was provided to them in the SMS or phone call. To verify the code provided by the user on the application, you have to issue another call to the Twilio Verify API:
Note how in the first verification attempt a wrong token was given, and in that case, the status attribute of the response is marked as pending
. Once the correct token is given, the status changes to approved
, and that is your indication that the verification was successful.
Conclusion
The Twilio Verify API is a simple way to increase the security of your application. I hope you decide to give it a try!
If you are interested in a real-world implementation of two-factor authentication with this API, see my tutorial Add Two-Factor Authentication to a Python Flask Application with Twilio Verify.
I would love to see what you build with the Twilio Verify API!
Miguel Grinberg is a Python Developer for Technical Content at Twilio. Reach out to him at mgrinberg [at] twilio.com if you have a cool Python project you’d like to share on the Twilio blog!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.