Redact or Delete SMS Messages as They Arrive with Node.js
Time to read:
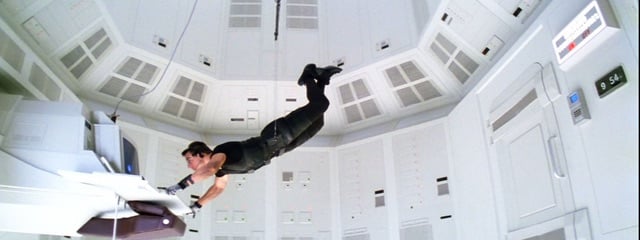
Keeping tabs on who has access to data in your application is hard when they exist in multiple places, like SMS messages in your app and within Twilio. Here’s how to redact or delete messages as soon you receive them with Node.js.
What you’ll need
To build this application to redact or delete SMS messages, you’ll need:
- A Twilio account (you can sign up for a free account if you don’t have one)
- A Twilio phone number that can send and receive SMS messages
- Node.js to build the app (I’m using the latest in the long term support series, version 6.9.2)
- ngrok so we can expose our development server to Twilio’s webhooks
Got all that ready? Let’s see how to delete some messages.
Building a webhook endpoint
In order to delete or redact our messages as they arrive we need to get notified by Twilio that we have received a message. To receive these notifications we use a webhook. When our Twilio number receives a message Twilio will make an HTTP request to a URL we supply with all the details about the message.
On the command line, create a new folder for our application, change into that folder and initialise a new Node.js application.
We need to install a few libraries to get our application started.
- express for creating the web application
- body-parser to read the body of the requests sent to the app from Twilio
- the Node.js Twilio helper for making requests to the Twilio REST API
Now create a file called index.js
and open it in your favourite editor. Enter the following application boilerplate:
We need an endpoint that can receive POST requests from Twilio whenever our Twilio number receives a message. We’ll start simple and create one that logs out the incoming message. The message body is sent as the Body
parameter in Twilio’s request to the application. Add a route for a POST request to /messages
with the following code to log the message.
Start up the server:
In another terminal get ngrok started as well:
Copy your ngrok URL and open the Twilio console to edit your phone number. Enter the ngrok URL with a path to /messages
in the Messaging config.
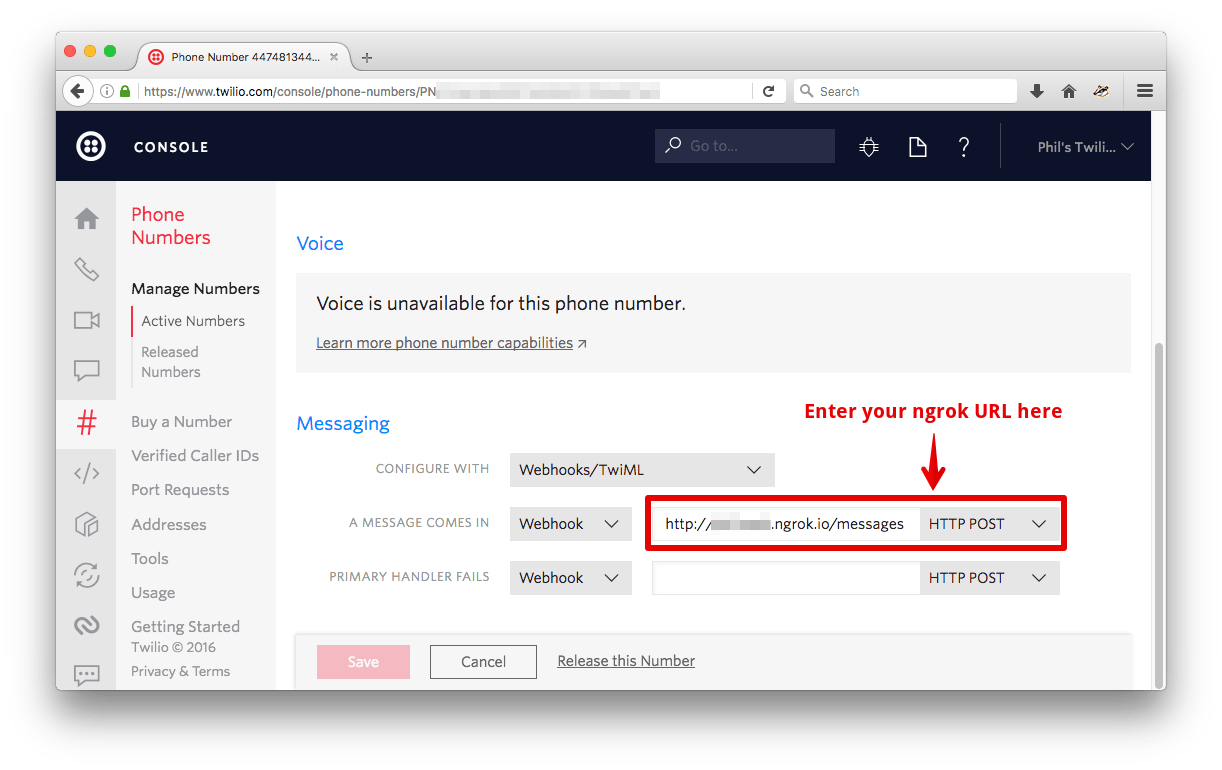
Now send your number a message and watch as the message is logged to the console! I sent myself a message that I knew wouldn’t be deleted.
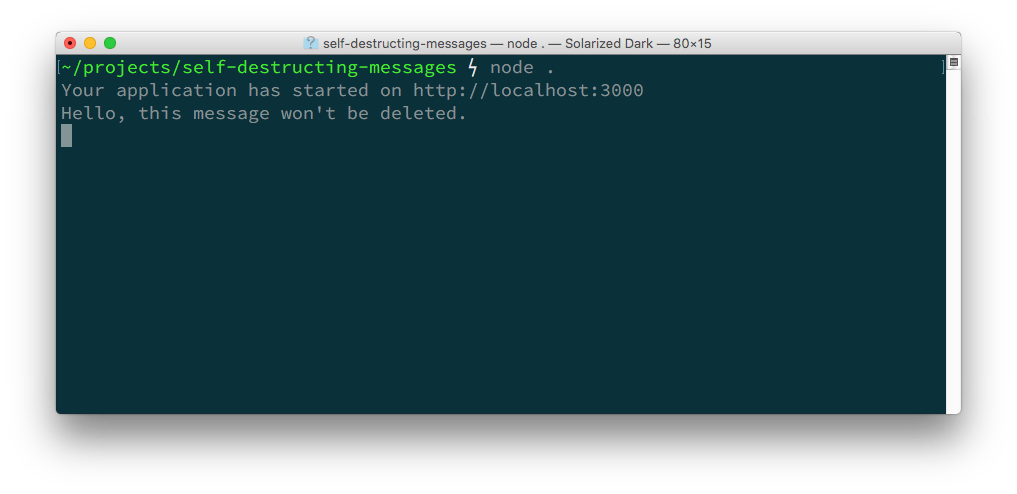
And there’s the message in the Twilio console log.
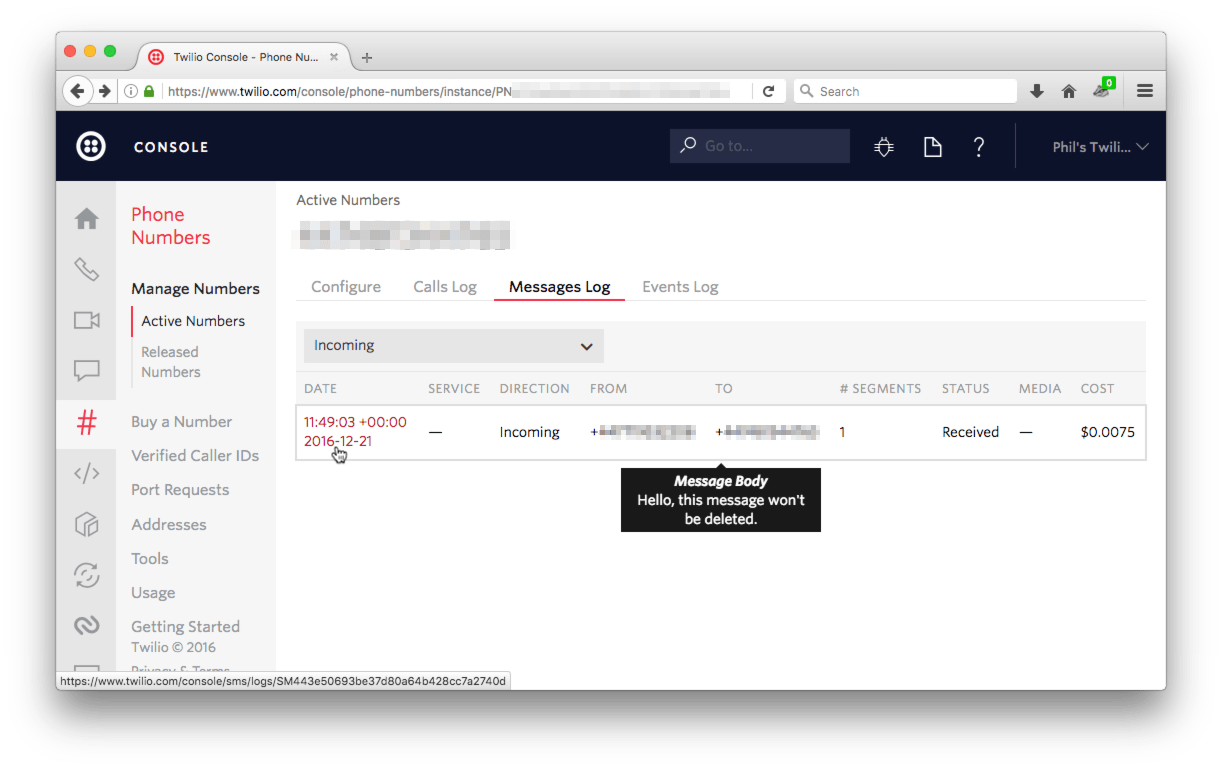
Let’s get down to redacting that message body or deleting the message entirely.
Setting up the Twilio REST Client
To redact the text in a message or delete it completely we’re going to use the Twilio Node.js helper library to access the REST API. The library will need to use your Account SID and Auth Token so set them as the environment variables TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
. If you’re not sure how to set environment variables, take a look at this setup guide.
Now we need to require the Twilio module and set up a REST client to use to access the API.
Redacting message bodies
To delete the text from a message we need to update the message resource by POSTing to it with a blank body. In your /messages
route add the following code:
Restart your server and send another message. You’ll see the body logged in the terminal, but when we inspect the Twilio console the message body will be gone.
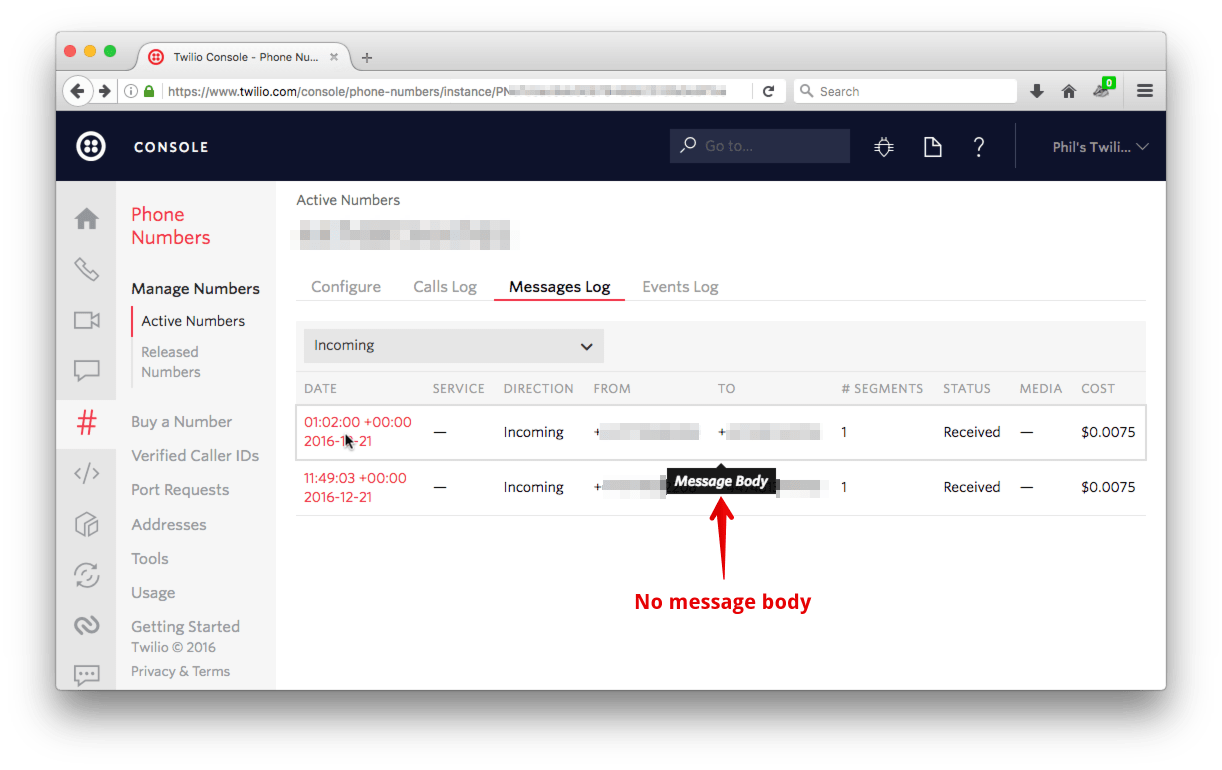
Deleting messages
We’ve managed to redact the body of the message, but what if we want to delete the message completely? We can perform a DELETE through the REST API. There is a slight problem here though. When the message arrives at our webhook, it may not have been marked as “received” within Twilio. We can’t delete the message until it is “received” so we keep checking the message until its status changes to “received”. Then we can delete it.
We first add a function to index.js which checks the message and its status and if it is “received” then deletes it, otherwise the application waits for 1 second and tries again.
We don’t want the web request to be waiting around for the message to get deleted, so we return some empty TwiML first, then call on our tryDelete
function.
Restart your server again and send in a message. It will get printed to the console, then you will see “Message deleted” printed and when you check the Twilio console it will be gone.
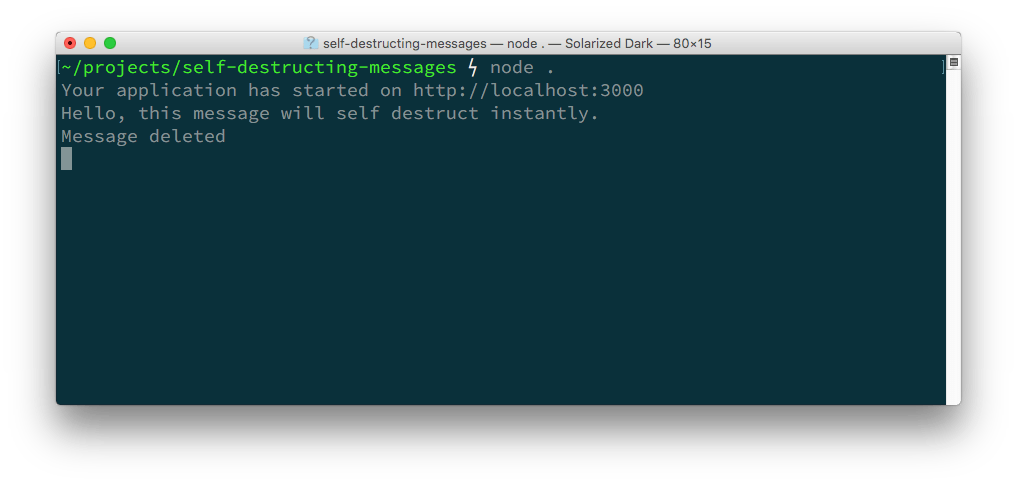
So that’s all you need to do to redact or delete your received messages in Twilio using Node.js. I recommend that rather than just logging the received message, you store it in your own database so that you can control who can and can’t read it.
If you’ve got excited about deleting things from Twilio, there’s more!
- If you also receive media messages, then watch out, you need to delete the media separate from the message text
- If you have call logs, recordings or transcriptions you want to delete you can do that too
Do you use other techniques to keep your messages under the sole control of your system? Let me know in the comments or drop me an email at philnash@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.