Build SMS Notifications With AngularJS, Firebase, and Twilio
Time to read: 4 minutes
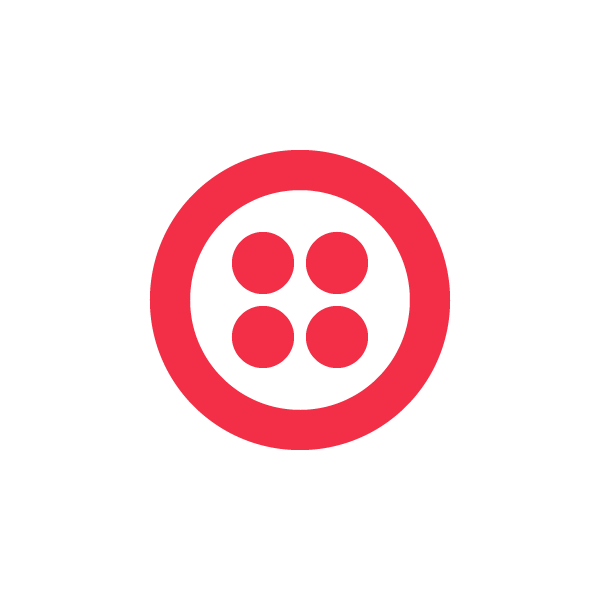
Christoffer Klemming started out programming pacemakers and ended up a Google PMM. Back home in Stockholm, Christoffer was focused on writing code. When he crossed the pond to work at Google, code took a backseat. But, he still used his programming mindset in a less code-intensive job. “Programming is really a mindset in how to break down big problems into smaller chunks that each can be logically solved, rolling back up to the whole,” says Christoffer.
Using AngularJS and Twilio helped him break down and roll up a problem that Bay Area residents know well – waiting in lines. A simple text can cut out the hassle of being stuck standing in line, waiting for your name to be called. Christoffer’s company, Waitwhile, allows businesses like beauty salons or restaurants to text waiting customers when they’re ready to serve.
It’s a simple, powerful solution for an annoying problem. MVC also happens to be an annoying problem for Christoffer. It’s one he solved using AngularJS. “I’m admittedly not an expert in MVC frameworks but I did spend a lot of time upfront researching what technology stack to use, essentially trying to answer: “what is the most future-proof way to build an app in 2015’.”
He landed on a combination of Twilio SMS, AngularJS, Firebase, and Ionic. Christoffer was surprised at how fast he got up and running after watching a tutorial on Watch and Code. Now he’s spreading the good word of AngularJS, showing you how to build SMS notifications with AngularJS and Firebase.
Check out his tutorial below.
Waitwhile – Building SMS Alerts For People In Line
Waitwhile is built with AngularJS and Firebase for storage and authentication, and relies on Twilio for 2-way texting. This post will explore how to juice up your client-side app with realtime text notifications using Twilio together with Zapier and Firebase.
Note: this post assumes you already have a Firebase instance up and running. If not, here’s a great 5 min tutorial.
Once you have a Firebase application configured, here’s how to get SMS with Zapier and Twilio set up in eight steps:
Step 1: Implement your app so that when you want to trigger an SMS, you create a new Firebase child object to a new child node (e.g. /sms/). Make sure to populate the SMS child object with the recipient’s phone number as an attribute:
You can also personalize the SMS with attributes like recipient name, sender or even media links. Make sure to add at least one Firebase object to /sms/ so that Zapier can recognize it later.
Step 2: Create your Twilio account. You get a “free number” from where your Twilio SMS will be sent from. If you want to be able to send SMS to numbers other than your own, you’ll need to load some Twilio credit. Fortunately, each SMS costs as low as $0.0075 and is well worth it.
Step 3: Create your free Zapier account. It’s free up to 100 zaps per month. Select “Create a new Zap” to get started.
Step 4: In the Zap edit mode, select Firebase as the trigger. Choose “New item in Queue” as the trigger setting. This is more reliable than triggering based on Added Child Record, as it reduces risk of duplicate entries and gives you more fine-grained control.
Note: it will remove the Firebase SMS child object once sent out, so treat that path as a temporary message queue in Firebase. If you want to store the SMS object in Firebase you’ll need to also record it under a permanent path, e.g. /smsHistory/).
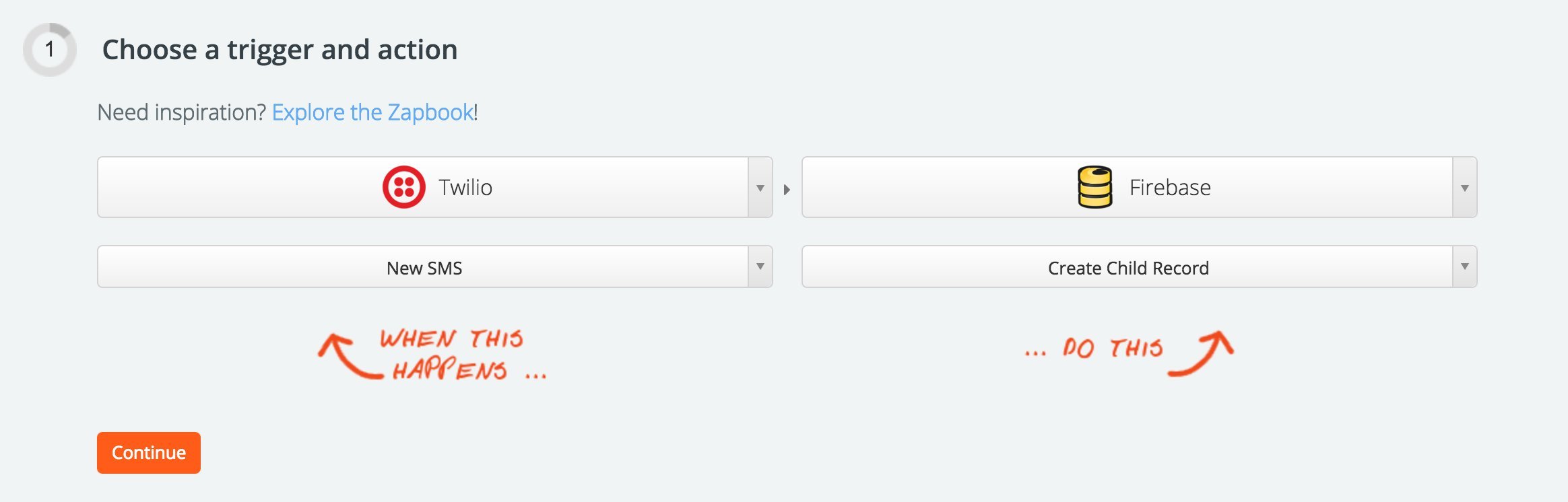
Step 5: Select Twilio “Send SMS” as the Action.
Step 6: Add your Firebase SMS path (e.g /sms/) as the “Path to Data”. Zapier will listen to this path for any new events and then instantly trigger the Zap action.
Step 7: Next, personalize your SMS. For the Phone and Message field, click the “Insert FB fields”. This will load your Firebase data from Step 1 and allow you to insert the appropriate attributes. You can add your own text here as well.
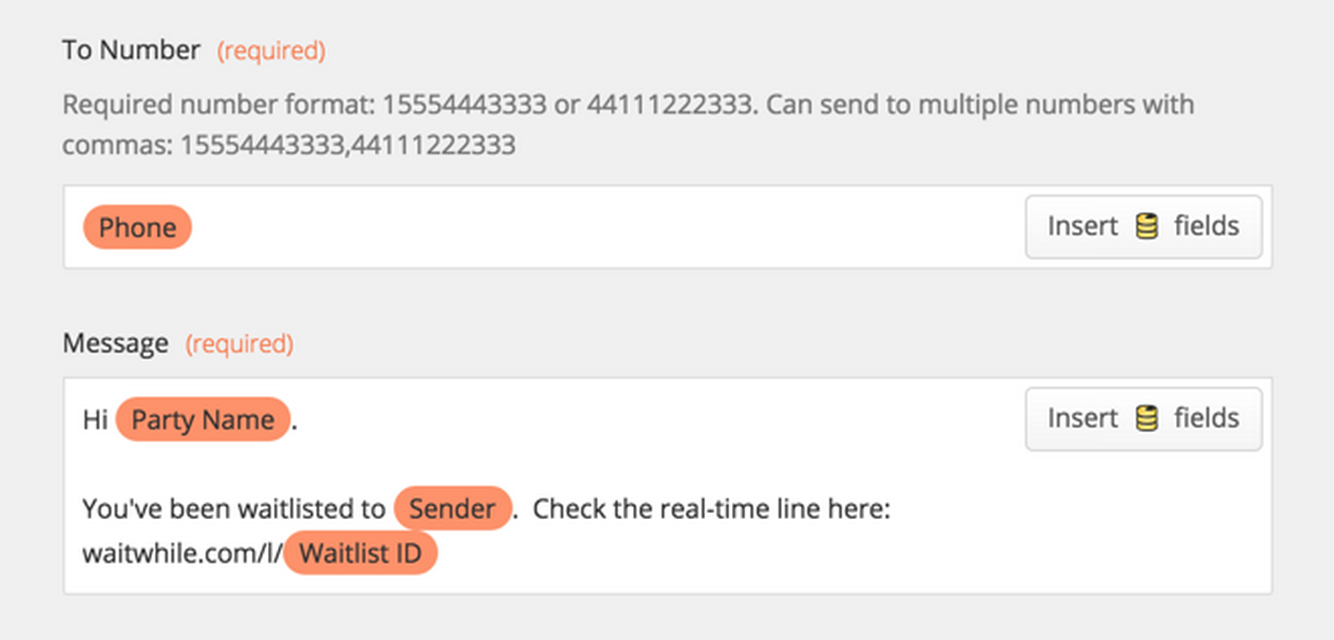
Step 8: While you’re trying it out, make sure to set your own phone number in the “To Number” field. Test the Zap to ensure you receive it.
Click “Turn Zap On” and it’s now up and running!
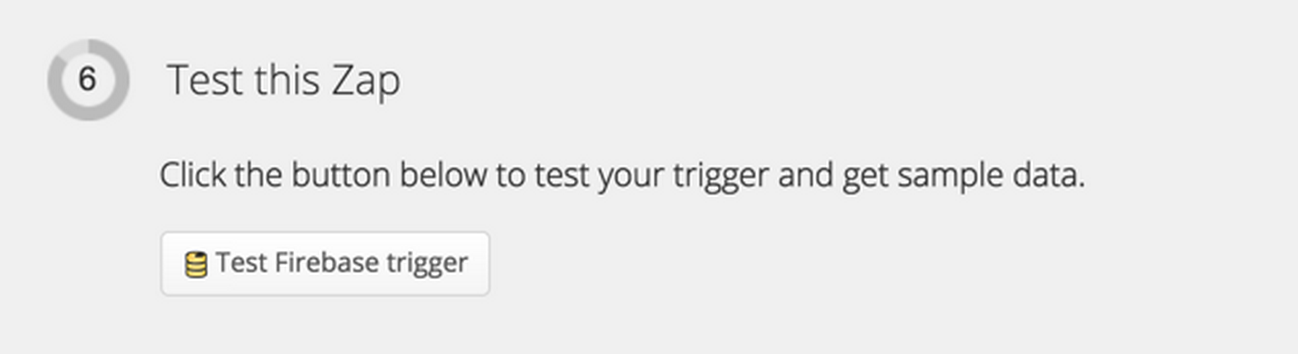
Make It Shine – Personalize SMS
Now that you have the basics set up, you can start making it more interesting by personalizing the SMS object. For Waitwhile, I actually generate an entire “SMS text” attribute which is fully personalized based on app interactions.
Then in Zapier just insert the “text” attribute as the entire Message. This has the added benefit of making SMS text changes more maintainable as you can do it directly in your app code.
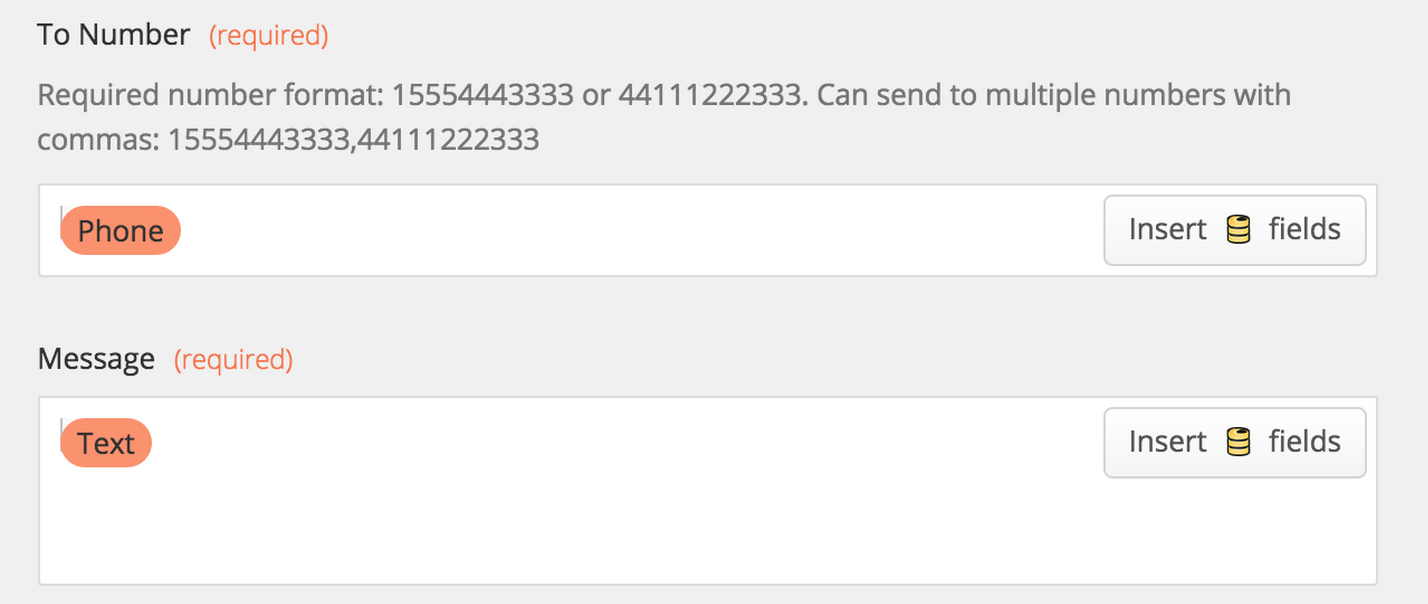
Make It Secure
In Firebase you’ll want to enforce security rules to avoid someone abusing your service to send spam texts and to check that phone numbers conform to international format (E.164), which Twilio requires. For example, a simple rule set might look like:
Support voice mail for incoming calls
Oh, since all your SMS will be sent from your Twilio free number (unless you upgrade), recipients may try to call it. To solve for this you can code your own Twilio voice mail using Twilio XML which a synthetic voice will read out!
Two-Way SMS
Finally, you can set up two-way SMS functionality by essentially reversing the Zapier flow (i.e. have a Firebase child record be creating by a Twilio new incoming SMS):
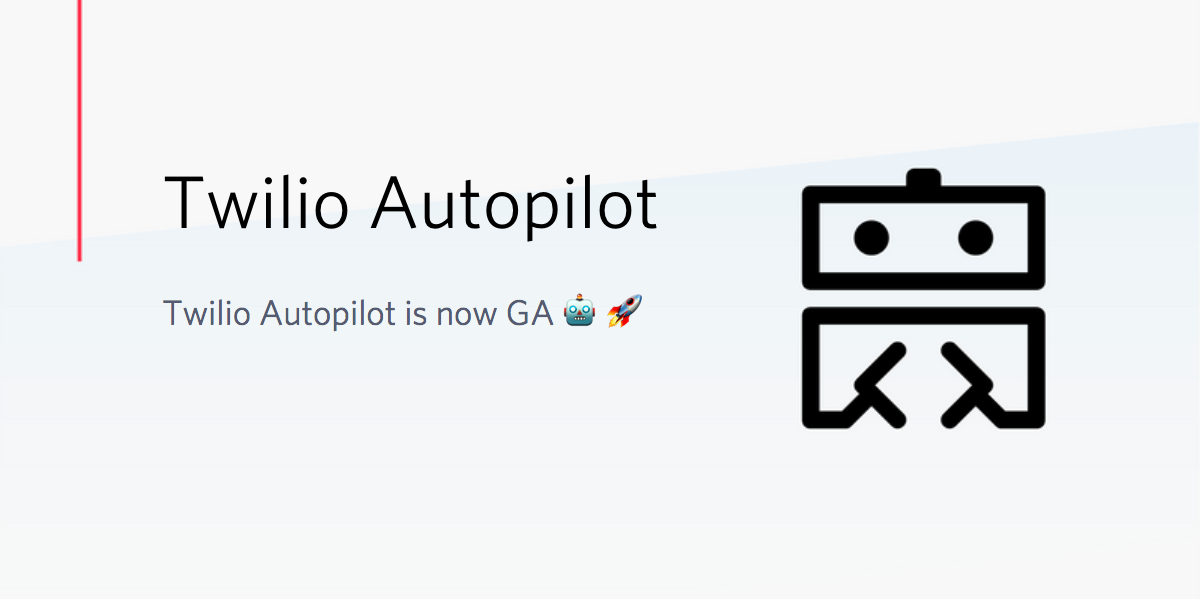
Twilio + Zapier + Firebase = awesome sauce
Twilio is an incredibly flexible and powerful platform. Together with Zapier to glue together different APIs, you can bootstrap advanced and reliable integrations in matter of minutes. Waitwhile has successfully triggered thousands of SMS messages by using Twilio together with Firebase and Zapier, and we’re just getting started!
Christoffer would love to learn if you have feedback on this tutorial. Comment below, email him at christoffer@waitwhile.com, or hit him up on Twitter @waitwhile anytime.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.